Complete Guide to Chatbot Development in C# for Beginners
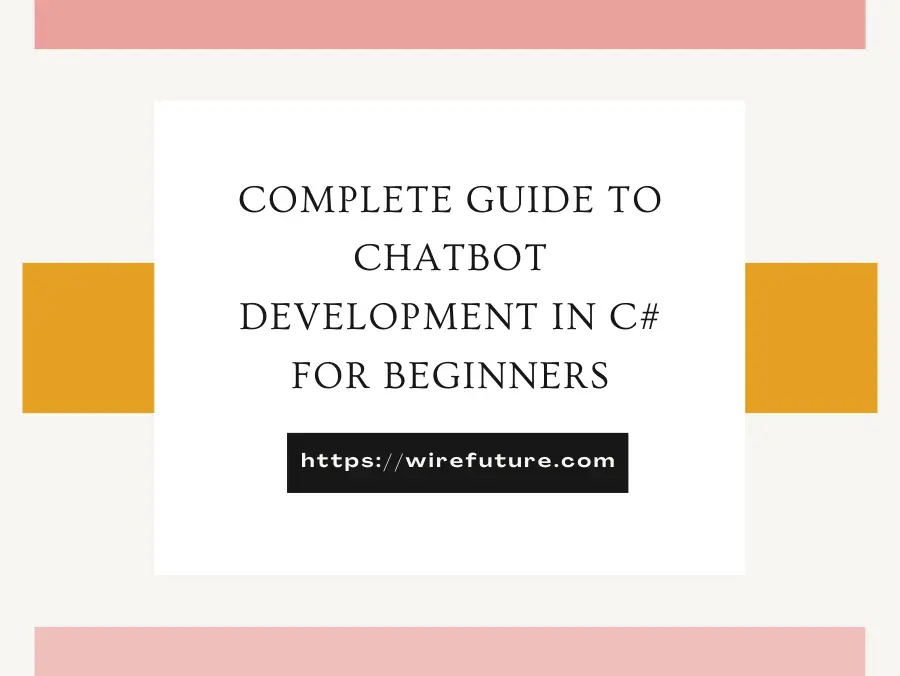
Chatbot development in C# is a great way to explore the world of artificial intelligence and automated customer service tools. This guide will walk through the entire development of a chatbot from scratch, designed for people with a background in .NET frameworks. Regardless of whether you wish to enhance user experience for business applications or simply want to add advanced tech to your tasks, chatbot development can offer excellent value for functionality and user experience.
First things first, define the core goals and design of the chatbot, and choose the tools and frameworks that best fit the powerful C# features. We will integrate NLP to help your chatbot understand and answer user questions step-by-step. We will also walk you through the basics of programming, testing and deployment of your chatbot – while keeping it strong, flexible and secure. We’re off to begin!
Table of Contents
- Choose the Environment For Chatbot Development
- Design the Chatbot’s Architecture
- Integrate Natural Language Processing (NLP)
- Develop the Core Functionality
- Incorporate Data Storage
- Testing and Deployment
- Conclusion
Choose the Environment For Chatbot Development
Chatbot development using C# requires choosing the right development environment. This prepares the groundwork for a smooth building process. Here is a broader view on how you choose and prepare your development environment:
Select Development Environment
Choose an appropriate IDE: The integrated development environment (IDE) is where you primarily code, debug and test your chatbot. Visual Studio is recommended for C# due to its robust features, support for .NET applications, and integration with most Microsoft services and tools. Visual Studio also includes a user interface, strong debugging capabilities, and numerous extensions to assist you in your development.
Install the Necessary Frameworks: Having the correct .NET framework or .NET Core installed is crucial for backend development. The .NET Framework is suited for a number of uses, and between .NET framework and .NET Core it is determined by everything you require -.NET Core is ideal for cross platform applications & microservices, whereas the .NET framework is perfect for Windows applications. Download the final stable release for the most recent features and security fixes.
Design the Chatbot’s Architecture
Designing your chatbot’s architecture is a crucial step that sets the groundwork for how your chatbot will function and interact with users. This involves choosing the right approach and tools for your development process. Here’s a detailed expansion on this step:
Design the Chatbot’s Architecture
- Choose the Chatbot Type: Begin by deciding between a rule-based or AI-based approach. Rule-based chatbots are simpler and respond to user inputs based on predefined rules. They work well for tasks that require straightforward, predictable interactions. On the other hand, AI-based chatbots use machine learning and natural language processing to understand and respond to user inputs more dynamically. This type is more suitable for complex tasks where user inputs are less predictable and can evolve over time.
- Select a Development Framework: For structuring the development process, consider using frameworks that provide foundational tools and functionalities. The Microsoft Bot Framework is an excellent choice for building chatbots in C#. It offers rich features, including support for multiple communication channels (like Microsoft Teams, Slack, Facebook Messenger), and built-in AI capabilities. To get started with Microsoft Bot Framework, you can download it from the official Microsoft Bot Framework page. This page provides all the necessary resources and documentation to help beginners set up and start developing their chatbots.
- Downloading and Setting Up the Framework: To download and set up the Microsoft Bot Framework, follow these steps:
- Visit the Microsoft Bot Framework website at the link provided above.
- Click on the “Start free” button to sign up or log in with your Microsoft account.
- Once logged in, navigate to the “Download” section to find the SDK and tools. Select the version compatible with your development environment (ensure you have Visual Studio installed as mentioned in the previous point).
- Follow the installation instructions provided on the website to set up the SDK on your machine.
Integrate Natural Language Processing (NLP)
In this example we will do a chatbot development for a STD health care website. Natural Language Processing (NLP) is an important starting point for developing a chatbot, especially for more niche applications such as a STD health website, where nuanced user queries are essential. With Microsoft LUIS (Language Understanding Intelligent Service), you can improve the context and accuracy of user input for your chatbot. Practical guide to NLP implementation with Microsoft LUIS, including examples for STD health chatbot:
Integrate Natural Language Processing (NLP):
- Utilize Microsoft LUIS:
- Setup: First, visit the Microsoft LUIS website. You’ll need to sign in using your Microsoft account. If you don’t have one, you’ll need to create it.
- Create a New LUIS App: Once logged in, go to the “My Apps” section and click on the “Create new app” button. Provide a name for your app, such as “STDHealthBot”, and a description that helps identify the app’s purpose.
- Set the Culture: Choose the appropriate culture/language for your chatbot based on the geographical location and language preferences of your users.
- Train Your NLP Model:
- Define Intents: Intents are purposes or goals that users have when interacting with the chatbot. For a STD health chatbot, examples of intents could be “SymptomCheck”, “TreatmentOptions”, and “ClinicLocations”.
- Click on “Build” in the top menu of your LUIS dashboard.
- Navigate to “Intents” in the left sidebar and click “Create new intent”. Enter the name for each intent and save.
- Define Entities: Entities represent specific details that the chatbot can extract from user input. For your STD chatbot, relevant entities might include “STDType” (e.g., HIV, herpes), “Symptoms” (e.g., rash, fever), and “Location”.
- Under the “Entities” menu on the left, click “Create new entity”. Choose a suitable type for each entity, like simple, hierarchical, or composite based on your needs, and define them.
- Add Utterances: Utterances are sample phrases that users might say related to each intent. This helps LUIS understand and recognize similar phrases during real interactions.
- For each intent, click on it, then add examples of user input in the “Utterances” section. For instance, under “SymptomCheck”, you might add phrases like “What are the symptoms of herpes?” or “I feel a burning sensation, what STD could it be?”
- Train the Model: After setting up intents and entities, train your model by clicking the “Train” button at the top right of the dashboard. This teaches LUIS how to recognize the intents and entities in user conversations.
- Test the Model: Use the “Test” panel on the right side of the dashboard to input sample queries and observe how well LUIS identifies intents and entities. Adjust your utterances and train again as needed.
- Define Intents: Intents are purposes or goals that users have when interacting with the chatbot. For a STD health chatbot, examples of intents could be “SymptomCheck”, “TreatmentOptions”, and “ClinicLocations”.
- Integrate LUIS with Your Chatbot:
- Once your model performs satisfactorily, publish it by navigating to the “Publish” section and selecting a production or staging slot. This action generates an endpoint URL.
- Use this endpoint in your C# chatbot application to send user input to LUIS and receive analyzed results, which guide how your chatbot responds.
These steps will enable your STD health website’s chatbot to process user queries with a greater level of understanding, making interactions more intuitive and effective.
Develop the Core Functionality
Developing the core functionality of your chatbot involves programming the necessary components to manage dialogues, handle user interactions, and maintain conversation context. This is why it is crucial to partner with an expert .NET development company who can understand the chatbot needs and develop in a robust manner. Here’s a detailed look at how you can develop these key features for an STD chatbot using C#, complete with code samples to illustrate the process:
Develop the Core Functionality:
Program the Chatbot Using C#: To begin programming your chatbot, you will need to handle basic interactions such as greeting the user, responding to queries, and managing dialogue flow. Below are some sample code snippets using C# that demonstrate how to implement these interactions within the Microsoft Bot Framework:
Setting Up the Bot Framework: Before you start coding, ensure you have the Bot Framework SDK installed. This can be done through NuGet in Visual Studio:
Install-Package Microsoft.Bot.Builder
Creating the Chatbot’s Main Handler: This handler will process incoming messages and generate responses. Here’s a simple example of a bot handler:
using Microsoft.Bot.Builder;
using Microsoft.Bot.Schema;
using System.Threading.Tasks;
public class STDHealthBot : ActivityHandler
{
protected override async Task OnMessageActivityAsync(ITurnContext<IMessageActivity> turnContext, CancellationToken cancellationToken)
{
string userMessage = turnContext.Activity.Text;
await turnContext.SendActivityAsync(MessageFactory.Text($"You said: {userMessage}"), cancellationToken);
}
}
Implement State Management: State management is crucial for tracking conversation context and ensuring that the chatbot can follow along with the user’s needs, especially in complex dialogues about health issues like STDs. Here’s how to implement basic state management:
Setting Up State Management: Use the Bot Builder SDK’s state management features to track and store conversation data.
using Microsoft.Bot.Builder;
using Microsoft.Bot.Builder.Dialogs;
Creating Conversation State Objects: You will store user data and dialogue information in these state objects.
public class STDHealthBotAccessors
{
public ConversationState ConversationState { get; }
public IStatePropertyAccessor<DialogState> DialogStateAccessor { get; }
public STDHealthBotAccessors(ConversationState conversationState)
{
ConversationState = conversationState ?? throw new ArgumentNullException(nameof(conversationState));
DialogStateAccessor = conversationState.CreateProperty<DialogState>("DialogState");
}
}
Using Dialogs to Manage Conversations: Dialogs help manage complex flows such as symptom checking or locating clinics. Here’s how you can set up a dialog for checking symptoms:
public class SymptomDialog : ComponentDialog
{
public SymptomDialog() : base(nameof(SymptomDialog))
{
var waterfallSteps = new WaterfallStep[]
{
PromptForSymptom,
AnalyzeSymptom,
};
AddDialog(new WaterfallDialog(nameof(WaterfallDialog), waterfallSteps));
AddDialog(new TextPrompt(nameof(TextPrompt)));
}
private async Task<DialogTurnResult> PromptForSymptom(WaterfallStepContext stepContext, CancellationToken cancellationToken)
{
return await stepContext.PromptAsync(nameof(TextPrompt), new PromptOptions { Prompt = MessageFactory.Text("Please enter your symptoms:") }, cancellationToken);
}
private async Task<DialogTurnResult> AnalyzeSymptom(WaterfallStepContext stepContext, CancellationToken cancellationToken)
{
var symptoms = (string)stepContext.Result;
// Logic to analyze symptoms
await stepContext.Context.SendActivityAsync(MessageFactory.Text($"Analyzing your symptoms for: {symptoms}"), cancellationToken);
// Proceed based on analysis
return await stepContext.NextAsync(null, cancellationToken);
}
}
These code examples provide a basic framework for developing an STD health chatbot using C#. By programming responses, managing dialogues, and maintaining state, your chatbot will be well-equipped to handle user interactions effectively and provide meaningful assistance.
Incorporate Data Storage
Incorporating data storage into your chatbot is essential for maintaining user data, session information, and for enabling robust logging mechanisms that aid in debugging and future enhancements. Here, I’ll expand on how to implement these features within a C# chatbot, specifically for a STD health website, providing detailed code samples.
Use Databases to Store User Data and Session Information: Storing user data and session information helps personalize interactions and maintain continuity in conversations. For our purposes, we can use Azure Cosmos DB, a globally distributed, multi-model database service that integrates well with C# applications and the Microsoft Bot Framework.
Setting Up Azure Cosmos DB: First, you need an Azure account and a Cosmos DB instance. Once set up, you can use the following NuGet package to integrate Cosmos DB with your C# project:
Install-Package Microsoft.Azure.Cosmos
Configuring Cosmos DB in C#: Configure the Cosmos client, database, and container in your C# application. Here’s how you might initialize these components:
using Microsoft.Azure.Cosmos;
public class CosmosDbService
{
private CosmosClient cosmosClient;
private Database database;
private Container container;
public async Task InitializeAsync(string databaseName, string containerName)
{
this.cosmosClient = new CosmosClient("YourConnectionStringHere");
this.database = await this.cosmosClient.CreateDatabaseIfNotExistsAsync(databaseName);
this.container = await this.database.CreateContainerIfNotExistsAsync(containerName, "/partitionKeyPath");
}
}
Storing and Retrieving Data: Implement methods to store and retrieve user session data and other relevant information.
public async Task AddItemAsync(MyItem item)
{
await this.container.CreateItemAsync<MyItem>(item, new PartitionKey(item.Id));
}
public async Task<MyItem> GetItemAsync(string id)
{
try
{
ItemResponse<MyItem> response = await this.container.ReadItemAsync<MyItem>(id, new PartitionKey(id));
return response.Resource;
}
catch (CosmosException) // For example, handle not found, etc.
{
return null; // Or handle errors appropriately
}
}
Implement Logging for Debugging and Future Improvements: Logging is critical for debugging issues and for understanding how users interact with your chatbot. Using Serilog with ASP.NET Core is a robust way to implement logging.
Setting Up Serilog: Add the Serilog packages to your project via NuGet:
Install-Package Serilog.AspNetCore
Install-Package Serilog.Sinks.AzureAnalytics
Configuring Serilog in Your Startup: Configure Serilog in the Startup.cs
of your ASP.NET Core application to log to both the console and Azure Analytics.
public class Startup
{
public Startup(IConfiguration configuration)
{
Log.Logger = new LoggerConfiguration()
.ReadFrom.Configuration(configuration)
.WriteTo.Console()
.WriteTo.AzureAnalytics("YourWorkspaceId", "YourPrimaryKey")
.CreateLogger();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseSerilogRequestLogging(); // Simple middleware for logging HTTP requests.
}
}
These code samples provide a foundation for integrating robust data storage and logging capabilities into your STD health chatbot.
Testing and Deployment
Thoroughly Test the Chatbot: Testing your chatbot involves checking its responses, understanding capabilities, and overall performance across a variety of scenarios. Here’s how to approach this:
Unit Testing: Begin with unit tests to ensure each component of your chatbot functions as expected. Using a framework like xUnit for C#, you can automate testing of the logical units within your application.
using Xunit;
using Microsoft.Bot.Builder;
using Microsoft.Bot.Builder.Adapters;
public class ChatbotTests
{
[Fact]
public async Task TestGreetingIntent()
{
var adapter = new TestAdapter();
var bot = new STDHealthBot();
await new TestFlow(adapter, async (turnContext, cancellationToken) =>
{
await bot.OnTurnAsync(turnContext, cancellationToken);
})
.Send("hello")
.AssertReply("Welcome to the STD Health Assistant! How can I help you today?")
.StartTestAsync();
}
}
Integration Testing: Test how different components of your chatbot work together, such as interaction with the backend database and third-party APIs. This ensures the end-to-end workflow is functioning correctly.
User Acceptance Testing (UAT): Simulate real user interactions to see how the chatbot handles various queries and dialogues. Tools like Bot Framework Emulator can be very helpful here, allowing you to interact with your bot in a controlled environment.
Deploy the Chatbot on Servers or Platforms Like Azure: Once your chatbot is thoroughly tested, you can deploy it to a server or a cloud platform such as Azure to make it accessible to users. Here’s a breakdown of these steps:
- Prepare the Bot for Deployment: Ensure your bot is ready for deployment by setting environment-specific configurations like database connections and API keys in your application settings.
- Deploy to Azure: Azure provides a seamless integration environment for bots developed with the Microsoft Bot Framework.
- Create an Azure Bot Service: Go to the Azure portal and create a new Bot Service. Follow the setup wizard, selecting the appropriate settings for your bot.
- Publish Your Bot to Azure: You can use Visual Studio to publish your chatbot directly to Azure. Right-click on your project, select ‘Publish’, and then follow the prompts to select your Azure Bot Service.
az login
az account set --subscription "<your-subscription-id>"
az bot publish --name "<your-bot-name>" --resource-group "<your-resource-group>"
Conclusion
Whether you’re building chatbots for dynamic customer support, business processes optimisation, or immersive user experiences, the chatbot development process is filled with opportunities for innovation and improvement. Recall that a chatbot that works well is able to listen and respond to users’ needs, so how you choose tools and how you develop is crucial.
If you want to create a chatbot but are unsure where to start, or need a specialist to create your digital assistant, hire chatbot developers at WireFuture. Our chatbot developers are happy to design, develop and deploy a chatbot to your specifications. Choosing WireFuture as your chatbot developers means you get the best expertise along with quality and innovation.
Take the next step in enhancing your digital strategy by incorporating a smart, efficient chatbot. WireFuture has chatbot experts who are experts in their field and ready to change how you interact with your audience. Let’s make something awesome together.
WireFuture's team spans the globe, bringing diverse perspectives and skills to the table. This global expertise means your software is designed to compete—and win—on the world stage.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.