How to Improve Performance of ASP.NET Core Web Applications
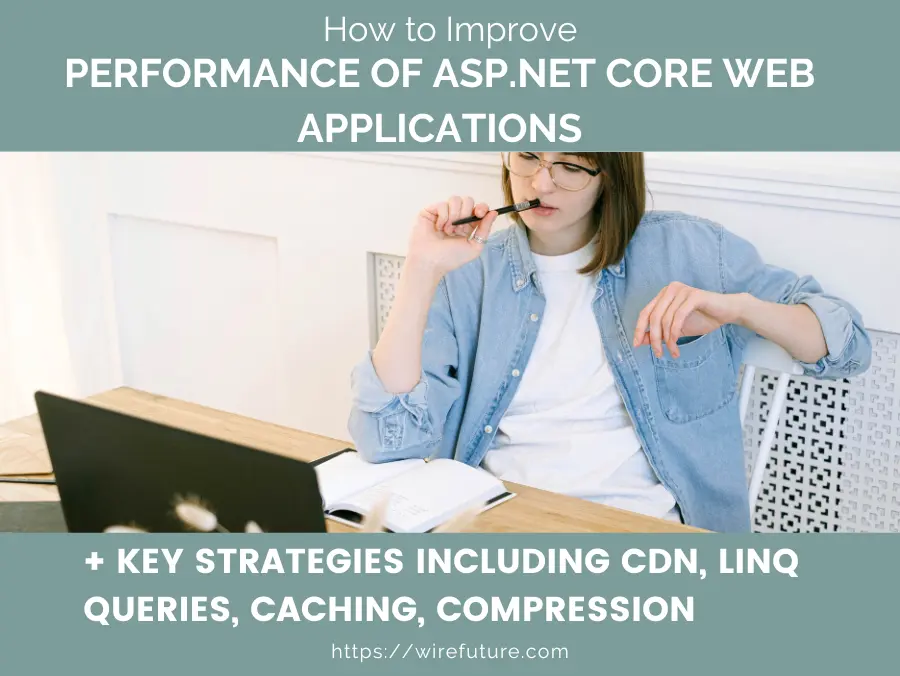
In the bustling world of web development, standing out requires not just innovative ideas but also the seamless performance of your applications. ASP.NET Core is an adaptable and feature rich platform which developers use to develop performant, scalable, and robust websites. However to genuinely make use of this framework, knowing how to improve performance of ASP.NET Core web applications is crucial. Optimization contributes to a faster and more responsive application for users while also reducing management resources and costs. This blog discusses optimization of ASP.NET Core web applications in detail. We cover everything from the fundamentals behind ASP.NET core performance to real techniques that can dramatically boost your app speed and efficiency. Whether you’re a pro developer or a newbie, these insights can help you to improve performance of ASP.NET Core applications. Let’s go optimization – where every millisecond saved is worth it to your users.
Table of Contents
- improve performance of ASP.NET Core Web Applications By Profiling
- Response Compression
- Efficient Data Access
- improve performance of ASP.NET Core Web Applications By Caching
- Minimize Middleware
- Static Files and CDN
- improve performance of ASP.NET Core Web Applications with Asynchronous Programming
- Health Checks and Monitoring
- Optimize Client-Side Performance
- improve performance of ASP.NET Core Web Applications With Regular Updates and Refactoring
- Conclusion
improve performance of ASP.NET Core Web Applications By Profiling
Profiling is a critical first optimization step for ASP.NET Core web applications. Imagine it as an app health check-up for your application that identifies where the performance issues are, like a detective sifting through code to find the meat in the nut shell. This is a crucial step since just before you can optimize your application performance you must understand the place that the bottlenecks are placed. These bottlenecks can range from memory leaks, overly CPU utilization, inefficient database queries, to badly executed HTTP requests.
Visual Studio Diagnostic Tools
This is an integrated suite inside Visual Studio that includes powerful profiling tools to diagnose performance issues. Whether you’re testing memory usage or investigating CPU spikes, Visual Studio Diagnostic Tools provides an intuitive experience that works directly with your development environment. It is like having a ‘magnifying glass ‘that reveals the inner workings of your application – you can see exactly where there is room for improvement without leaving your coding interface.
JetBrains dotTrace
dotTrace by JetBrains is a profiler that focuses on application performance analysis – determining the time spent in each part of your code. It’s intuitive and integrates seamlessly with other JetBrains tools — making it a favorite among developers who work within the JetBrains ecosystem already. dotTrace visualizes call trees, hot spots, and threading issues to help you fine tune your application.
Redgate ANTS Performance Profiler
Redgate’s offering is another powerful tool to make profiling easier. It’s best at profiling SQL queries, HTTP requests, and.NET code – giving you the inside scoop on where your application is falling behind. ANTS performance Profiler provides an intuitive user interface for drilling down into the data to find the root cause of Performance problems. It is particularly helpful for programmers on database-intensive tasks where optimizing SQL queries can yield substantial performance improvements.
Use of these tools effectively requires a methodical approach. Start by defining what you want to achieve from your profiling session. Is it reducing memory usage, reducing response time, or detecting slow running database queries? Once you have a target in mind, run your application with these tools to gather performance metrics. Read the results to analyze what issues are causing the most harm to your application. Such an analysis may point out unexpected places where optimizations can make a significant contribution.
Recall that profiling is not about finding the problems but understanding them. This understanding lets you prioritize your optimization efforts to the areas that matter most to your application’s performance. Profiling is thus the baseline for all subsequent optimization efforts, leading you through the minefield of performance improvement with clarity and clarity.
Response Compression
Response compression for ASP.NET Core web apps is one way to boost performance by compressing HTTP responses delivered out of your server to the client. This technique may result in reduced transmission times resulting in a superior user experience, especially on slower network links. It’s like optimizing how your web content is delivered to the user so it reaches him quickly.
Enabling Response Compression Middleware
ASP.NET Core provides built-in middleware for response compression. To enable it, you must first add the middleware to your project dependencies.
Step 1: Add Package Dependency
Ensure that your project file (*.csproj) includes a reference to the Microsoft.AspNetCore.ResponseCompression
package. If it’s not included, you can add it via NuGet package manager or manually by editing the csproj file:
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.ResponseCompression" Version="X.X.X" />
</ItemGroup>
Replace X.X.X
with the latest version of the package.
Step 2: Configure Middleware
In your Startup.cs
file, update the ConfigureServices
method to include the response compression services:
public void ConfigureServices(IServiceCollection services)
{
// Add response compression services
services.AddResponseCompression(options =>
{
options.Providers.Add<GzipCompressionProvider>();
options.EnableForHttps = true; // Optional: To enable compression for HTTPS responses
});
// Add framework services.
services.AddControllersWithViews();
}
Then, in the Configure
method, ensure that the response compression middleware is added before any middleware that might compress responses:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseResponseCompression();
// The rest of your middleware configuration...
}
Step 3: Configure Compression Provider
Optionally, you can further configure the compression provider. For instance, setting the compression level:
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCompression(options =>
{
options.Providers.Add<GzipCompressionProvider>();
options.EnableForHttps = true;
});
services.Configure<GzipCompressionProviderOptions>(options =>
{
options.Level = CompressionLevel.Fastest;
});
// Add framework services.
services.AddControllersWithViews();
}
Testing Compression
After enabling response compression, you should test your application to ensure that responses are indeed being compressed. This can be done using tools like Postman or CURL:
curl -i -H "Accept-Encoding: gzip" https://yourapplication.com
In the response headers, look for Content-Encoding: gzip
which indicates that the response was compressed.
Caveats and Best Practices
- Content Type: By default, not all content types are compressed. You may need to add or remove content types based on your application’s needs.
- Compression Level: Choosing the compression level (
Fastest
,Optimal
, or a custom level) can impact CPU usage. Test to find the best balance between compression efficiency and server performance. - Security: Be aware of security implications, such as BREACH attacks, when enabling compression for sensitive content over HTTPS.
Follow these steps and considerations and you can implement response compression in your ASP.NET Core web applications to minimize response sizes for faster transmission times just as you would choose the most efficient delivery method for your web content. This tuning improves the UI and results in efficient use of resources on the server and client side.
Efficient Data Access
Database interaction optimization is a critical component to improve performance of ASP.NET Core web applications. Although Entity Framework Core (EF Core) provides a lightweight and extensible ORM solution for.NET applications that lets developers interact with a database through.NET objects, it should be used wisely to avoid performance issues. This chapter will walk you through LINQ usage considerations, using raw SQL for extremely complex queries, and indexing your database tables.
Optimizing LINQ Queries
LINQ queries can sometimes generate inefficient SQL, especially with complex operations. Mastering these optimizations requires a deep understanding of both LINQ and Entity Framework Core. If you’re aiming to enhance your application’s data access layer but lack the in-house expertise, consider the benefits of partnering with specialized talent. At WireFuture, we offer you the opportunity to hire expert Entity Framework Core developers who bring a wealth of experience in optimizing LINQ queries and leveraging the full potential of Entity Framework. Here are some tips to optimize your LINQ queries:
Avoid N+1 Queries
N+1 queries are a common performance issue where an initial query is followed by a separate query for each result of the first query.
Example – Problematic N+1 Query:
var users = context.Users.ToList();
foreach (var user in users)
{
// This will execute a separate query for each user
var orders = context.Orders.Where(o => o.UserId == user.Id).ToList();
}
Solution – Use .Include()
to Avoid N+1 Queries:
var usersWithOrders = context.Users
.Include(u => u.Orders)
.ToList();
Select Only Required Columns
Retrieving only the necessary columns can significantly reduce the amount of data transferred.
Example – Selecting Specific Columns:
var userNames = context.Users.Select(u => new { u.Id, u.Name }).ToList();
Using Raw SQL for Complex Queries
For scenarios where LINQ might not be the most efficient way to query your data, EF Core allows you to execute raw SQL queries, which can be particularly useful for complex queries.
Example – Executing a Raw SQL Query:
var userOrders = context.Orders
.FromSqlRaw("SELECT * FROM Orders WHERE UserId = @userId", new SqlParameter("@userId", userId))
.ToList();
Indexing Your Tables
Proper indexing of your database tables is crucial for optimizing query performance thererby contributing to improve performance of ASP.NET Core web applications. An index can dramatically speed up data retrieval operations, though it’s important to use them judiciously to avoid slowing down write operations. If you’re struggling to work with complex database queries, consider to hire expert SQL developer from WireFuture.
SQL Example – Creating an Index:
CREATE INDEX IX_Orders_UserId ON Orders (UserId);
Entity Framework Core Migrations for Indexes
You can also manage indexes through EF Core migrations.
Example – Adding an Index with a Migration:
First, annotate your model with the Index
attribute:
using System.ComponentModel.DataAnnotations.Schema;
public class Order
{
public int Id { get; set; }
[Index]
public int UserId { get; set; }
// Other properties
}
Then, generate and apply a migration:
dotnet ef migrations add AddOrderUserIdIndex
dotnet ef database update
Best Practices and Considerations
- Profile Your Queries: Always profile and review the actual SQL generated by EF Core to ensure it’s efficient and optimized for your specific database.
- Be Cautious with Raw SQL: While raw SQL can be more efficient for complex queries, it bypasses the safety and abstraction provided by EF Core, making your application more susceptible to SQL injection attacks if not handled carefully.
- Use Indexes Wisely: While indexes can improve read operations, they can also slow down writes because the index must be updated on every insert, update, or delete operation. Balance the number of indexes with the read and write needs of your application.
improve performance of ASP.NET Core Web Applications By Caching
Caching is a key optimization technique used in web development to store expensive data (in terms of time, CPU or database resources) for later requests. ASP.NET Core supports numerous caching mechanisms, among which MemoryCache is the simplest and most efficient in many cases. We’ll see how to use MemoryCache in your ASP.NET Core applications like a super-fast access drawer for your most frequently used tools and data. If you’re looking to enhance your web application’s performance through advanced caching techniques but need expert guidance, hiring seasoned ASP.NET developers can make all the difference. Hire ASP.NET developers from WireFuture today and ensure your web applications run faster and smoother than ever.
Setting Up MemoryCache in ASP.NET Core
To utilize MemoryCache
, you first need to add it to your services in the Startup.cs
file. This makes it available via dependency injection throughout your application.
Step 1: Register MemoryCache Services
public void ConfigureServices(IServiceCollection services)
{
services.AddMemoryCache(); // Adds MemoryCache services to the DI container
services.AddControllersWithViews();
}
Using MemoryCache
With MemoryCache
services registered, you can now inject and use IMemoryCache
in your controllers or services to cache data.
Step 2: Inject IMemoryCache
public class SampleController : Controller
{
private readonly IMemoryCache _memoryCache;
public SampleController(IMemoryCache memoryCache)
{
_memoryCache = memoryCache;
}
}
Step 3: Store and Retrieve Data from the Cache
public IActionResult GetUserData(int userId)
{
// Define a cache key
var cacheKey = $"UserData_{userId}";
// Try to get data from cache
if (!_memoryCache.TryGetValue(cacheKey, out User user))
{
// Key not in cache, so get data from the database
user = GetUserFromDatabase(userId);
// Set cache options
var cacheEntryOptions = new MemoryCacheEntryOptions()
.SetSlidingExpiration(TimeSpan.FromMinutes(5)) // Expire if not accessed for 5 minutes
.SetAbsoluteExpiration(TimeSpan.FromHours(1)); // Expire after 1 hour regardless of access
// Save data in cache
_memoryCache.Set(cacheKey, user, cacheEntryOptions);
}
return View(user); // Return data (from cache or database)
}
In this example, we attempt to retrieve user data from the cache. If it’s not available (cache miss), we fetch the data from the database and store it in the cache with specific options. The SetSlidingExpiration
method ensures the cache entry expires if it hasn’t been accessed in a set period, while SetAbsoluteExpiration
specifies a fixed time at which the cache entry should expire.
Advanced Caching Strategies
- Cache Dependencies: Use cache dependencies to invalidate cache entries when related data changes. This is particularly useful for maintaining consistency between cached data and your database.
- Distributed Cache: For applications running on multiple servers, consider using a distributed cache (like Redis or SQL Server) that can be shared across instances. ASP.NET Core provides built-in support for several distributed caching solutions.
- Varying Cache by Parameters: When caching data that varies based on input parameters (such as user IDs in the example above), ensure that the cache key uniquely identifies each variant to prevent data mixing.
- Monitoring and Eviction Policies: Monitor cache utilization and configure eviction policies wisely to balance memory usage with performance benefits. ASP.NET Core’s caching infrastructure provides events and hooks to help manage this.
Caching can significantly improve performance of ASP.NET Core web applications – by minimizing overhead operations such as database calls. Like a super-fast access drawer, caching lets you access commonly used data quickly, making your application faster and more responsive.
Minimize Middleware
Minimizing middleware is akin to cleaning out your home. it entails going through what you have, deciding what you want and throwing away what’s not necessary. Each middleware piece you add to your application’s request pipeline adds a performance hit because every request must go through that middleware. By reducing this, you simplify your application and potentially lower latency/response time.
Understanding Middleware in ASP.NET Core
Middleware components in ASP.NET Core are software components that are assembled into an application pipeline to handle requests and responses. Each component can perform operations before and after the next component in the pipeline.
Reviewing Your Middleware Configuration
The configuration of your middleware typically takes place in the Configure
method of the Startup.cs
file. Here’s an example of what this might look like:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
Minimizing Middleware
To minimize middleware, you need to evaluate each piece you’ve added to your pipeline and determine if it’s necessary for every request.
Step 1: Identify Middleware That Is Not Required
For example, consider if your application benefits from app.UseHttpsRedirection();
in every environment, or could it be limited to just production?
Step 2: Conditionally Apply Middleware
You can conditionally apply middleware based on the environment or other conditions:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
app.UseHttpsRedirection(); // Only use HTTPS redirection in non-development environments
}
app.UseStaticFiles();
if (MyCustomCondition)
{
app.UseMyCustomMiddleware(); // Conditionally use custom middleware
}
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
In this adjusted configuration, UseHttpsRedirection
is only applied outside of the development environment, and a hypothetical custom middleware is only added if a specific condition (MyCustomCondition
) is true.
Step 3: Avoid Redundant Middleware
Ensure you’re not adding middleware that serves the same purpose or could be combined for efficiency. For instance, if you have custom logging middleware, ensure it doesn’t duplicate the logging provided by ASP.NET Core’s built-in services.
Best Practices
- Benchmark: Use performance testing and benchmarking to understand the impact of each middleware on your application’s performance. This empirical data will guide your decisions on what to keep, remove, or optimize.
- Understand Middleware Order: The order in which middleware components are added to the pipeline matters. Some middleware depends on others to have been executed first. Be mindful of this when adding or removing middleware.
- Keep Up with Updates: New versions of ASP.NET Core might introduce more efficient ways to use or configure middleware. Stay updated to leverage these improvements.
Static Files and CDN
Static file serving is an important optimization factor for static assets such as images, JavaScript and CSS files, which are static assets. Delivering these files effectively can dramatically reduce the load time and UX of your application. With a content delivery Network (CDN) you can even lengthen these benefits by distributing your Content across multiple, geographically distributed servers so users get it faster wherever they are in the world. This is like putting your static content in the fast lane of the internet, avoiding congestion and reducing latency.
Optimizing Static File Serving in ASP.NET Core
ASP.NET Core applications serve static files using the Static File Middleware, configured in the Startup.cs
file. Here’s how to optimize this process:
Step 1: Configure Static File Middleware
Ensure the middleware is correctly configured to serve static files. In Startup.cs
, the UseStaticFiles()
method enables static file serving from the wwwroot
folder.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseStaticFiles(); // Enables static file serving
// Remaining middleware configuration...
}
Step 2: Enable Response Caching
To optimize performance, enable response caching for static files. This reduces the need for repeat requests to your server for the same content.
app.UseStaticFiles(new StaticFileOptions
{
OnPrepareResponse = ctx =>
{
const int durationInSeconds = 60 * 60 * 24; // 1 day
ctx.Context.Response.Headers[HeaderNames.CacheControl] =
"public,max-age=" + durationInSeconds;
}
});
Using a CDN for Global Content Distribution
A CDN can significantly accelerate the delivery of static content by caching it closer to your users. This section explains how to integrate a CDN with your ASP.NET Core application.
Step 1: Choose a CDN Provider
Select a CDN provider that fits your needs. Popular options include Cloudflare, AWS CloudFront, and Microsoft Azure CDN. These services distribute your static content across a network of servers worldwide.
Step 2: Upload Your Static Files to the CDN
Follow your CDN provider’s instructions to upload your static assets or configure your CDN to pull assets directly from your server.
Step 3: Update Your Application to Reference CDN URLs
Modify your application to reference the CDN URLs for your static files instead of local paths. This can be done in your HTML, CSS, or JavaScript files.
Example – Referencing a CDN URL in HTML:
<link href="https://yourcdn.example.com/css/style.css" rel="stylesheet">
Example – Referencing a CDN URL in ASP.NET Core Razor View:
<img src="@Url.Content("https://yourcdn.example.com/images/logo.png")" alt="Logo">
Best Practices for Using CDNs
- Select the Right CDN: Consider factors like geographic coverage, cost, and ease of integration when choosing a CDN provider.
- Use Versioning for Cache Busting: When you update a static file, change its version in the URL to ensure users get the latest version.
- Secure Your Content: Use HTTPS for your CDN URLs to prevent man-in-the-middle attacks and ensure data integrity.
- Monitor CDN Performance: Regularly monitor your CDN’s performance to ensure it meets your expectations and adjust configurations as necessary.
improve performance of ASP.NET Core Web Applications with Asynchronous Programming
Asynchronous coding utilizing async and await keywords within ASP.NET Core will help improve responsiveness and scaleability of your web applications. With asynchronous operations (particularly for I / O-bound tasks like database access, file I / O, and network request) your application can do other work while waiting for these operations to finish. This non-blocking behavior is like putting a multitasking superhero in your code so your application can handle more concurrent requests with less friction – and overall deliver a better user experience which contributes significantly to improve performance of ASP.NET Core web applications.
Understanding Async and Await
The async
modifier indicates that a method is asynchronous and can contain one or more await
expressions. The await
keyword is applied to an asynchronous operation, and it yields control to the calling method until the awaited task is complete. This mechanism helps keep your app responsive by freeing up the current thread to execute other tasks.
Implementing Asynchronous Methods
Here’s a step-by-step guide to implementing asynchronous methods in an ASP.NET Core application, with examples:
Step 1: Marking the Method as Async
Add the async
keyword to the method signature, and return a Task
or Task<T>
.
public async Task<IActionResult> GetFileAsync()
{
var filePath = "path/to/file.txt";
var fileContent = await File.ReadAllTextAsync(filePath);
return Content(fileContent);
}
In this example, File.ReadAllTextAsync
is an asynchronous method that reads all text from a file without blocking the current thread. The await
keyword waits for the operation to complete before proceeding, without stalling the thread.
Step 2: Asynchronous Database Operations
When working with Entity Framework Core or other ORM libraries that support async operations, leverage their asynchronous methods to access the database.
public async Task<IActionResult> GetUsersAsync()
{
var users = await _context.Users.ToListAsync();
return View(users);
}
This snippet asynchronously retrieves all users from the database and passes them to the view. The ToListAsync
method is a part of Entity Framework Core’s asynchronous API.
Step 3: Async Actions in Controllers
ASP.NET Core supports asynchronous actions in controllers, allowing your web application to handle more requests by not blocking threads on long-running tasks.
public async Task<IActionResult> DownloadFileAsync(string fileName)
{
var path = Path.Combine(_hostingEnvironment.WebRootPath, "files", fileName);
var memoryStream = new MemoryStream();
using (var stream = new FileStream(path, FileMode.Open))
{
await stream.CopyToAsync(memoryStream);
}
memoryStream.Seek(0, SeekOrigin.Begin);
return File(memoryStream, GetContentType(path), Path.GetFileName(path));
}
This action asynchronously reads a file from the server’s file system and returns it to the user for download. The use of CopyToAsync
for file operations ensures that the I/O operation does not block the thread. Implementing such optimizations requires a deep understanding of .NET Core’s capabilities and best practices. If you’re looking to leverage these advanced techniques but need more expertise in-house, consider partnering with WireFuture. Our team of expert .NET developers can help you harness the full power of ASP.NET Core, ensuring your application is not only high-performing but also scalable and secure.
Best Practices for Asynchronous Programming
- Avoid Blocking Calls: Replace blocking calls like
.Result
or.Wait()
withawait
to prevent deadlocks. - Use Async All the Way: Once you start an async method, continue using async methods up the call chain. Mixing synchronous and asynchronous code can lead to performance issues and deadlocks.
- Exception Handling: Use try-catch blocks around asynchronous code to handle exceptions properly.
- ConfigureAwait(false): When you don’t need to marshal the continuation back to the original context, use
ConfigureAwait(false)
to potentially improve performance by avoiding unnecessary context switches.
Health Checks and Monitoring
Health checks and monitoring for the ASP.NET Core web applications are important for ensuring availability, performance, and reliability. Health checks let you monitor your application and its dependencies – including databases, file systems and external services – in real time. Application Insights and Serilog are monitoring tools that extend this capability by collecting, analyzing and displaying detailed telemetry data to diagnose issues, analyze Application behavior, and get alerts when performance anomalies are detected. This combination constitutes an integrated health and performance monitoring system, which is essential for preventive maintenance and optimization.
Implementing Health Checks in ASP.NET Core
ASP.NET Core comes with built-in support for health checks, making it easy to set up endpoints that check the health of your application and its critical dependencies.
Step 1: Configure Health Checks
First, you need to add health check services to your application’s service collection in the Startup.cs
file:
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks()
.AddDbContextCheck<ApplicationDbContext>() // Checks EF Core DbContext
.AddUrlGroup(new Uri("http://example.com"), name: "External API check"); // Checks external API availability
}
This code snippet adds health checks to the service collection and configures checks for an Entity Framework Core DbContext
and an external API.
Step 2: Expose Health Check Endpoint
Next, configure a health check endpoint in the Configure
method:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseEndpoints(endpoints =>
{
endpoints.MapHealthChecks("/health");
});
}
This creates a /health
endpoint that returns the health status of your application.
Monitoring with Application Insights and Serilog
While health checks give you a snapshot of the current health status, tools like Application Insights and Serilog provide deeper insights into your application’s behavior and performance over time.
Application Insights
Application Insights, a feature of Azure Monitor, is an extensible Application Performance Management (APM) service for web developers. It monitors live applications, automatically detecting performance anomalies, and includes powerful analytics tools.
Configuring Application Insights:
- Add the Application Insights SDK to your ASP.NET Core application via NuGet.
- Configure the Application Insights service in the
Startup.cs
file:
public void ConfigureServices(IServiceCollection services)
{
services.AddApplicationInsightsTelemetry();
}
Set the Instrumentation Key in your appsettings.json
file or through environment variables.
Configuring Serilog:
- Install the Serilog.AspNetCore NuGet package.
- Configure Serilog in the
Program.cs
file:
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.UseSerilog((hostingContext, loggerConfiguration) => loggerConfiguration
.ReadFrom.Configuration(hostingContext.Configuration)
.Enrich.FromLogContext()
.WriteTo.Console()
.WriteTo.File("logs/myapp.txt"))
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
This configures Serilog to read settings from the application’s configuration file and write logs to both the console and a file. There are many other popular logging libraries like Serilog, log4Net and NLog. Checkout our blog post on implementing logging in ASP.NET Core to supercharge your web applications.
Best Practices for Health Checks and Monitoring
- Comprehensive Coverage: Ensure your health checks cover all critical components of your application, including databases, file systems, and external services.
- Secure Health Check Endpoints: Protect your health check endpoints to prevent unauthorized access.
- Alerting: Configure alerts based on specific metrics or log events to be notified of issues in real-time.
- Log Analysis: Regularly review and analyze log data to identify patterns, trends, and potential issues.
Optimize Client-Side Performance
To improve performance of ASP.NET Core Web Applications, client-side performance optimization is equally as vital as server side optimization for a responsive user experience. A correctly optimized front-end could considerably cut load times, deliver higher responsiveness and deliver an excellent user experience.
Minify and Bundle JavaScript and CSS
Minification removes unnecessary characters from code (like whitespace and comments) without changing its functionality, while bundling combines multiple files into a single file. Both processes reduce the number of requests that a browser needs to make and the size of the files to be downloaded.
Using ASP.NET Core’s Built-In Bundling and Minification
ASP.NET Core offers built-in support for bundling and minification via the Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation package.
- Configure BundleOptions in
Startup.cs
:
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
services.AddRazorPages()
.AddRazorRuntimeCompilation();
services.Configure<BundleOptions>(options =>
{
options.EnableBundling = true;
options.EnableMinification = true;
options.UseContentRootSourcePath = true;
});
}
- Define Bundles in a Configuration File:
Create a bundleconfig.json
file in your project root to define the bundles.
[
{
"outputFileName": "wwwroot/css/site.min.css",
"inputFiles": [
"wwwroot/css/bootstrap.css",
"wwwroot/css/site.css"
]
},
{
"outputFileName": "wwwroot/js/site.min.js",
"inputFiles": [
"wwwroot/js/jquery.js",
"wwwroot/js/bootstrap.js"
],
"minify": { "enabled": true },
"sourceMap": false
}
]
Optimize Images
Optimizing images involves reducing their file size without significantly affecting their visual quality. This can drastically reduce the amount of data transferred to the client, speeding up page loads.
- Use Image Compression Tools: Tools like TinyPNG or ImageOptim can compress images efficiently. For automated workflows, consider using build tools like Gulp or webpack with image optimization plugins.
- Serve Images in Modern Formats: Formats like WebP offer superior compression and quality characteristics compared to traditional formats like JPEG and PNG. Convert images to these formats where browser support allows.
- Implement Responsive Images: Use the
<picture>
element orsrcset
attribute to serve different image sizes based on the client’s screen size and resolution, ensuring that images are not larger than necessary.
Use Content Delivery Networks (CDNs) for Static Assets
CDNs can serve static assets (like CSS, JavaScript, and images) from servers located closer to the user, reducing latency and improving load times.
- Configure your application to use CDN URLs for static resources. This might involve updating the links in your HTML or Razor views to point to the CDN-hosted versions of your assets.
Implement Lazy Loading
Lazy loading defers the loading of non-critical resources at page load time. Instead, these resources are loaded at the moment they are needed.
- Use the
loading="lazy"
Attribute for Images and Iframes:
<img src="image-to-lazy-load.jpg" loading="lazy" alt="Description">
- For JavaScript, consider dynamic imports with promises or the async attribute for scripts:
import(/* webpackChunkName: "myChunkName" */ './myModule').then((module) => {
// Use module which is loaded dynamically
});
improve performance of ASP.NET Core Web Applications With Regular Updates and Refactoring
Updating your ASP.NET Core web applications and dependencies is a vital exercise for performance, safety measures, and compatibility. Regular updates can add optimizations, patches for vulnerabilities and new features that can improve your application. In addition to changes, refactoring your codebase is vital for maintainability, efficiency, and code readability. How do you manage updates and refactor your code for performance?
Keeping Applications and Dependencies Up-to-Date
Update ASP.NET Core and Its Dependencies
- Use Visual Studio or the .NET CLI to Check for Updates: Visual Studio users can use the NuGet Package Manager to check for available updates for all packages. .NET CLI users can use commands like
dotnet list package --outdated
to find anddotnet add package <PACKAGE_NAME> --version <VERSION>
to update. - Read Release Notes: Before updating, review the release notes for each package. They can provide valuable insights into the changes, including performance improvements, breaking changes, or new features that could impact your application.
- Test After Updates: Ensure your application functions as expected after updates by running tests. Automated unit and integration tests can be particularly useful here.
Example Command to Update a Package with .NET CLI:
dotnet add package Microsoft.EntityFrameworkCore --version <NEW_VERSION>
Regular Code Refactoring
Refactoring is the process of restructuring existing code without changing its external behavior. It’s crucial for keeping the codebase clean, efficient, and easy to maintain.
Identify Refactoring Opportunities
- Code Smells: Look for “code smells” such as duplicate code, long methods, large classes, or complex conditional statements. These are indicators that your code might benefit from refactoring.
- Performance Bottlenecks: Use profiling tools to identify parts of your application that are slow or inefficient and target these areas for refactoring.
Implement Refactoring Techniques
- Break Down Large Methods into Smaller, Reusable Methods: Large methods can be difficult to read, test, and maintain. Breaking them into smaller methods can improve readability and reusability.
// Before refactoring
public void ProcessData()
{
// Code to validate data
// Code to process data
// Code to save data
}
// After refactoring
public void ProcessData()
{
ValidateData();
ProcessValidData();
SaveData();
}
- Use Design Patterns Where Appropriate: Design patterns like Repository for data access or Strategy for selecting algorithms can make your code more modular and easier to maintain.
- Optimize Data Access: Review and optimize your database access. Use efficient queries, and ensure you’re not over-fetching data.
- Remove Unused Code and Dependencies: Dead code and unused dependencies can clutter your project, making it harder to maintain. Regularly review your codebase and project references to remove these.
Schedule Regular Code Reviews
- Peer Reviews: Regularly review code with peers to identify potential refactoring opportunities or to discuss possible performance improvements.
- Automated Code Analysis Tools: Tools like ReSharper, Roslyn Analyzer, or SonarQube can automatically identify code quality issues and suggest improvements.
Best Practices
- Stay Informed: Subscribe to newsletters, follow technology blogs, and participate in developer communities to stay informed about the latest updates and best practices for ASP.NET Core development.
- Document Changes: Keep a changelog or use version control commit messages to document updates and refactoring efforts. This can be invaluable for understanding the evolution of your application and for troubleshooting.
- Balance Performance and Readability: While optimizing for performance, ensure your code remains readable and maintainable. Sometimes, ultra-optimized code can become difficult to understand and maintain, defeating the purpose of refactoring.
Conclusion
In conclusion, to improve performance of ASP.NET Core web applications is a continual process that calls for attention, a proactive approach towards maintenance and updates, along with a willingness to continuously refine & improve code. Whether it’s optimized data access, caching, or ensuring your application’s security posture is robust without sacrificing performance, each optimization step enhances the end-user experience and application performance.
WireFuture is a leader in ASP.NET development. As a dedicated ASP.NET development company, we understand the art of creating performant, secure and scalable web apps. Whether you need to optimize your web application or start a new project, hire ASP.NET Core developers from WireFuture. Our experience and quality control ensure that we deliver solutions that exceed your expectations. Let us turn your vision into reality with custom ASP.NET Core solutions for your needs.
WireFuture stands for precision in every line of code. Whether you're a startup or an established enterprise, our bespoke software solutions are tailored to fit your exact requirements.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.