Angular vs. React: What Every New Developer Needs to Know
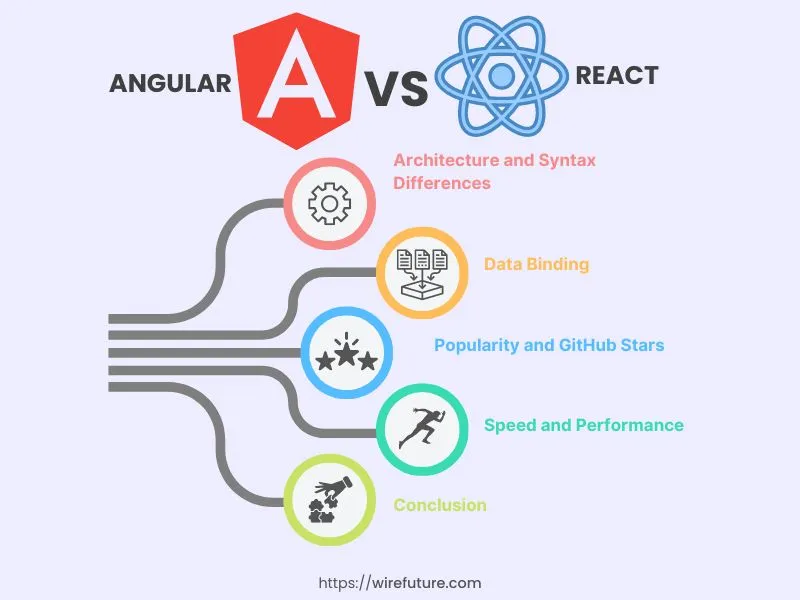
When new developers start exploring JavaScript frameworks, they typically compare Angular and React first. Angular offers a comprehensive package, ideal for developers looking for a structured approach to building large-scale applications. On the other hand, React, with its focus on building dynamic user interfaces, appeals to those who appreciate flexibility and simplicity in their projects. Choosing between Angular’s all-encompassing framework and React’s component-based library lays the groundwork for their project’s future and ultimately their career goals and preferences.
Angular is a full-fledged MVC (Model-View-Controller) framework developed by Google whereas React is a library for building user interfaces developed by Facebook. They both have their unique strengths and cater to different types of web development projects.
- Architecture and Syntax Differences Between Angular and React
- Popularity and GitHub Stars
- Real-World Usage
- Speed and Performance
- Conclusion
Architecture and Syntax Differences Between Angular and React
People like Angular because it takes a comprehensive approach and comes with a lot of features ready to use, such as form handling, routing, and state management. It uses TypeScript, which is a more advanced version of JavaScript. TypeScript adds static typing to the code, which makes it easier to change and fix bugs.
But React, on the other hand is more focused on the view layer and gives you more options for how the design is set up. It uses JSX, a syntax extension that lets you write HTML inside of JavaScript.
Let us discuss with the help of several concepts and fundamental differences that distinguish Angular from React.
1. Component Structure
Angular components are defined with a decorator that specifies template, styles, and selector metadata.
import { Component } from '@angular/core';
@Component({
selector: 'app-greeting',
template: `<h1>{{ greeting }}</h1>`,
styleUrls: ['./greeting.component.css']
})
export class GreetingComponent {
greeting: string = 'Hello, Angular!';
}
React components can be created as classes or functions and use JSX for templating.
import React, { useState } from 'react';
function Greeting() {
const [greeting] = useState('Hello, React!');
return <h1>{greeting}</h1>;
}
2. Data Binding
Angular supports two-way data binding, allowing for automatic synchronization between the model and the view.
<!-- Angular template -->
<input [(ngModel)]="user.name" type="text">
<p>Hello, {{user.name}}!</p>
React achieves similar functionality through controlled components, with state management and explicit event handling.
// React component
import React, { useState } from 'react';
function UserGreeting() {
const [name, setName] = useState('');
return (
<div>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<p>Hello, {name}!</p>
</div>
);
}
3. State Management
Angular manages state within components or through services for more complex scenarios, often using libraries like NgRx for global state management.
import { Injectable } from '@angular/core';
import { BehaviorSubject } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class UserService {
private userSource = new BehaviorSubject<string>('Angular User');
currentUser = this.userSource.asObservable();
constructor() { }
changeUser(name: string) {
this.userSource.next(name);
}
}
React uses hooks like useState
for local state management within components and Context API or libraries like Redux for global state.
import React, { createContext, useContext, useState } from 'react';
const UserContext = createContext();
function App() {
const [user, setUser] = useState('React User');
return (
<UserContext.Provider value={{ user, setUser }}>
<ChildComponent />
</UserContext.Provider>
);
}
function ChildComponent() {
const { user, setUser } = useContext(UserContext);
return (
<div>
<p>{user}</p>
<button onClick={() => setUser('New React User')}>Change User</button>
</div>
);
}
4. Lifecycle Methods
Angular provides lifecycle hooks for key moments in a component’s lifecycle, like creation and destruction.
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-lifecycle-example',
template: `<p>Lifecycle example</p>`
})
export class LifecycleExampleComponent implements OnInit {
constructor() { }
ngOnInit() {
console.log('Component initialized');
}
}
React class components offer lifecycle methods, while functional components use hooks like useEffect
for similar purposes.
import React, { useEffect } from 'react';
function LifecycleExample() {
useEffect(() => {
console.log('Component mounted');
return () => {
console.log('Component will unmount');
};
}, []);
return <p>Lifecycle example</p>;
}
Popularity and GitHub Stars
React often leads in popularity and GitHub stars, reflecting its wide adoption among developers for both simple and complex web applications. Angular, while having a significant following, usually trails behind React in these metrics, majorly due to its steep learning curve and more opinionated nature. Below is the position of both the frameworks as in Feb, 2024.
React Watches And Stars

Angular Watches And Stars

Real-World Usage
Angular’s use of TypeScript adds an extra layer of efficiency and safety, making it easier to manage large codebases and maintain high standards of quality. Financial institutions, e-commerce sites, and dynamic content platforms often utilize Angular to build their applications. Angular is preferred for enterprise-level applications, thanks to its structured framework and robust features. For instance, companies like Microsoft, Autodesk, PayPal, Delta Airlines and McDonald’s often use Angular for their web applications.
React’s versatility shines in the development of single-page applications (SPAs), where users expect smooth interactions without page reloads. It’s also the foundation for many modern web applications and sites that prioritize user experience and interactivity. Major companies like Facebook, Instagram, Netflix and Airbnb are among the notable examples of React in action.
Speed and Performance
Both Angular and React deliver high performance for web applications, but their approaches differ.
Angular
Angular uses real DOM, which can slow down the app’s performance when handling a large number of elements and complex data binding. However, it compensates with ahead-of-time (AOT) compilation, optimizing the application during the build process.
If you are facing performance issues in your Angular applications you should consider implementing few of the many techniques. Discussing each technique in detail is outside the scope of this article as each method deserves a separate blog post of its own.
Ahead-of-Time (AoT) Compilation
Angular applications can be compiled in two ways: Just-in-Time (JiT) and Ahead-of-Time (AoT). AoT compilation converts your Angular HTML and TypeScript code into efficient JavaScript code during the build phase before the browser downloads and runs the code. This process significantly reduces the application’s load time by eliminating the need to compile the application in the browser, leading to quicker rendering and improved performance.
Lazy Loading
Lazy loading is a method for loading programme modules, resources, or parts only when they are needed. By breaking the app up into multiple bundles and loading them one at a time, this method can greatly shorten the time it takes for the app to load for the first time. You can easily use lazy loading in Angular with the Angular Router, which makes setting up route-based code splitting simple.
Tree Shaking
Tree shaking gets rid of code that isn’t being used in your app, which makes the bundle smaller and the start time faster. By only including the code that is used in your app, you can get rid of library code that isn’t needed and make your app smaller overall. When using AoT compilation and ES2015 modules, Angular’s build tools, such as Webpack, allow tree shaking right out of the box.
Optimizing Change Detection
The change detection system in Angular can be tweaked to make applications run faster. By default, Angular uses a change detection technique based on zones. This can cause performance problems in complex apps. By telling Angular to only run change detection when certain input properties change, the OnPush change detection approach in components can cut down on the number of checks it needs to do by a large amount.
Minimizing Use of Third-Party Libraries
Third-party tools can improve your app’s useful features, but they may also make it the app’s size larger and slower. Check if each library is really needed, and think about lighter alternatives or custom versions for functions that aren’t too complicated. Always see how adding a new library affects the size of the bundle and how it affects speed.
Server-Side Rendering (SSR)
By using Angular Universal’s Server-Side Rendering, you can make your app seem faster, especially when it first loads. SSR makes sure that users see the content before the whole Angular app becomes dynamic by rendering Angular apps on the server and sending static HTML to the browser. This method works especially well for SEO and for people whose internet connections are slow.
Efficient Use of Observables
Angular’s RxJS Observables are a useful way to handle data flows that happen at different times. However, improper use of observables can cause memory leaks and speed problems. Make sure you unsubscribe from observables in components that are removed, or use operators like takeUntil to finish observables automatically and stop memory leaks.
React
React uses a virtual DOM, allowing for efficient updates and rendering by comparing the current state with the new state and updating only what’s necessary. This leads to faster performance, especially in dynamic applications with frequent UI updates.
If you are facing performance bottleneck in a React application you should focus on rendering performance, bundle size reduction, and efficient data handling. Here are some key strategies that you should consider:
Code Splitting
Splitting up the code is one of the best ways to make React apps run faster. With this method, your app is broken up into smaller pieces that are only loaded when they are needed. The whole pack is not loaded at once. The dynamic import() syntax in React lets you split code right out of the box. This syntax can be used with React.lazy to split code at the component level and with React Router to split code based on routes.
Memoization
Memoization is a way to speed things up by caching expensive function calls so that subsequent renders don’t have to do extra math. React gives you React.memo to remember components and useMemo to remember values inside functional components. These tools are especially helpful for parts that render often with the same props or for calculations that are expensive and don’t need to be done again and again for each render.
Optimizing Renders with shouldComponentUpdate and React.PureComponent
You can control the re-rendering process in class components by defining the shouldComponentUpdate lifecycle method. This method checks to see if a component should update when a state or prop changes. Alternatively, adding to React.PureComponent instantly compares props and state in a shallow way, which stops renders that aren’t needed. These methods help lower the drawing work, especially for parts that don’t change very often.
Efficient State Management
State handling is a very important part of how well a React app works. It can be inefficient to load parts with state that can be moved or doesn’t need to be in that state at all. Carefully choose which global state management tools to use, like Redux or Context API, and make sure that only the necessary parts are linked to the state and re-rendered when it changes.
Server-Side Rendering (SSR)
Similar to Angular, Server-Side Rendering in React renders parts of apps on the server and sends the HTML to the client, which speeds up the app’s initial start time. Although it takes more work to set up and take into account for client-side interactivity, this method is better for SEO and can get information to users faster.
FAQ on Angular vs. React
The fundamental distinction between Angular and React lies in their nature and architecture. Angular is a comprehensive framework that provides a robust set of features for building complex web applications, including two-way data binding, a comprehensive component-based structure, and a suite of development tools.
React, by contrast, is a JavaScript library that focuses exclusively on building user interfaces and encourages the development of UI components that are both reusable and stateful.
The learning curve for both technologies depends significantly on the individual’s background in web development. React is often perceived as more straightforward to learn due to its focused scope and simplicity in building interactive user interfaces.
Angular, conversely, encompasses a wider array of concepts and features, including dependency injection, templates, and RxJS for handling asynchronous operations, which may require a steeper learning curve.
Performance in Angular and React applications can be highly optimized and is largely dependent on the implementation strategy adopted by the developer. React’s virtual DOM provides an efficient way of updating the user interface by only re-rendering components that have changed. Angular employs ahead-of-time compilation, which optimizes the application at build time, leading to improved runtime performance. Both technologies, when utilized effectively, can achieve high performance in web applications.
Yes, both Angular and React can be utilized for developing mobile applications. React has a dedicated framework, React Native, which allows for the creation of native mobile applications using React’s component-based architecture. Angular can be used in conjunction with frameworks such as Ionic or NativeScript to build mobile applications that leverage Angular’s powerful features and development practices.
Community support is a vital aspect of any technology’s ecosystem. React, developed and maintained by Facebook, boasts a vast and active community that contributes to a wealth of resources, including libraries, tools, and tutorials. Angular, developed by Google, similarly benefits from strong community support, with a wealth of resources available for developers, including comprehensive documentation, community forums, and third-party tools. Both technologies are well-supported and continually evolving through community contributions.
The demand for developers skilled in either Angular or React is substantial and varies according to industry trends, geographic location, and specific company requirements. React, owing to its popularity for front-end development, has seen a significant demand in the job market. Angular, with its robust framework suitable for enterprise-level applications, also maintains a strong presence in job listings, particularly in companies that value structured development practices and scalability.
The decision to use Angular or React for a project should be based on several factors, including the project’s requirements, the development team’s familiarity with the technology, and the specific features needed for the application. Angular offers a full-fledged framework with a wide range of built-in functionalities, making it suitable for large-scale, complex applications. React, being a lightweight library focused on UI components, offers greater flexibility and is ideal for projects that require a dynamic and interactive user interface. Ultimately, the choice between Angular and React should align with the project’s goals and the development team’s expertise.
Conclusion
Angular and React are both used by web developers, but they meet different needs. Angular is a full framework that has a steep learning curve but strong features for building large-scale apps. Because React is more adaptable and simple to use, it can be used for a wide range of projects, from easy ones to hard ones. Angular or React should be chosen based on the needs of the project, how familiar the team is with the technology, and the amount of flexibility and functionality that is needed.
Dive into the digital age with WireFuture, your go-to hub for top-tier web development talent! Are you on the hunt to hire Angular developers or hire React developers? Our team at WireFuture is a melting pot of innovation and expertise, ready to bring your web projects to life with the latest in Angular and React technologies. We’re proud of creating dynamic, responsive and user friendly applications that not only satisfy your expectations but also exceed them. Our developers are here to turn your vision into a stunning reality, whether it’s a single page application, a complex enterprise grade solution, or an interactive social media platform.
WireFuture stands for precision in every line of code. Whether you're a startup or an established enterprise, our bespoke software solutions are tailored to fit your exact requirements.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.