Comprehensive Guide to the Latest C# 13 Latest Features with Examples
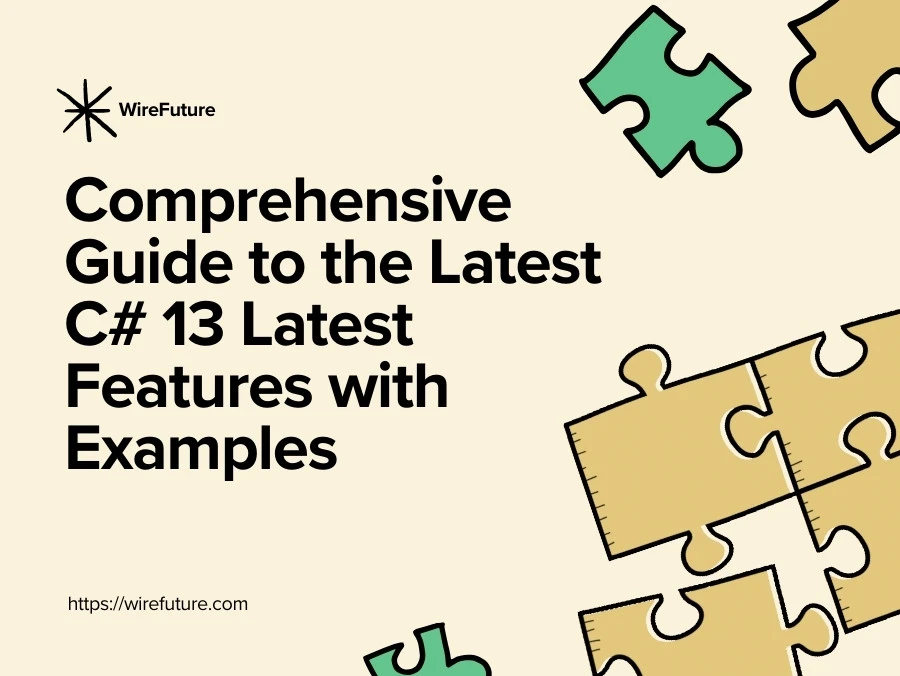
In this blog post we will explore C# 13 latest features that make the language more functional and flexible so that programmers can create more effective and expressive code. This article reviews these features along with practical examples and explanations to help developers understand and leverage the latest developments in C#. If you are looking for C# 12 features, you can find them here: C# 12 Latest Features.
1. params
Collections
Description
Traditionally, the params
keyword in C# allowed methods to accept a variable number of arguments, but it was limited to arrays. In C# 13, params
is extended to support any type that implements IEnumerable<T>
and has an Add
method, such as List<T>
, Span<T>
, and ReadOnlySpan<T>
. This change allows greater flexibility in method design and makes it easier to pass collections without converting them to arrays.
Example
void PrintValues(params IEnumerable<int> values)
{
foreach (var value in values)
Console.WriteLine(value);
}
// Using List<int>
PrintValues(new List<int> { 1, 2, 3 });
// Using ReadOnlySpan<int>
int[] numbers = { 4, 5, 6 };
PrintValues(numbers.AsSpan());
This enhancement reduces the need to manually convert collections to arrays before passing them to methods, streamlining code and improving performance by avoiding unnecessary allocations.
2. New Lock
Object
Description
The System.Threading.Lock
type in C# 13 provides a modern approach to thread synchronization. Unlike the traditional Monitor
class, Lock
offers scoped locking via EnterScope()
, which automatically manages the lock’s lifecycle. This method simplifies resource management and reduces boilerplate code for ensuring thread safety.
Example
var lockObject = new System.Threading.Lock();
using (lockObject.EnterScope())
{
// Critical section
Console.WriteLine("Resource locked safely");
}
Lock
improves synchronization efficiency and clarity by integrating lock acquisition and release with the using
statement. This minimizes the risk of forgetting to release a lock, which can lead to deadlocks or resource contention.
3. \e
Escape Sequence
Description
C# 13 introduces the \e
escape sequence to represent the ESCAPE character (Unicode U+001B
). Previously, this character was represented using \u001b
or \x1b
, which were less intuitive and could lead to errors if followed by valid hexadecimal digits.
Example
string escapeSequence = "Escape character: \e";
Console.WriteLine(escapeSequence);
The \e
escape sequence makes the code more readable and less error-prone by providing a straightforward way to include the ESCAPE character in strings, eliminating confusion with hex values.
4. Method Group Natural Type
Description
C# 13 improves overload resolution involving method groups by pruning inapplicable candidate methods earlier in the process. This enhancement allows the compiler to determine the most suitable method overload more efficiently, especially in complex scenarios involving generics. By optimizing overload resolution, C# 13 provides more precise .NET development solutions, streamlining the development process and enhancing performance. This feature is particularly beneficial for large codebases, reducing ambiguity in method selection and making the code more maintainable and robust.
Example
Func<int, bool> isEven = IsEven;
bool IsEven(int number) => number % 2 == 0;
This optimization reduces compilation time and simplifies the process of resolving method groups to their most appropriate overloads, particularly in complex generic scenarios.
5. Implicit Indexer Access in Object Initializers
Description
The implicit indexer, represented by the ^
operator, allows developers to access array elements from the end. In C# 13, this feature is extended to object initializers, enabling more intuitive and concise array and collection initializations.
Example
var countdown = new TimerRemaining()
{
Buffer =
{
[^1] = 0,
[^2] = 1,
[^3] = 2,
[^4] = 3,
[^5] = 4,
[^6] = 5,
[^7] = 6,
[^8] = 7,
[^9] = 8,
[^10] = 9
}
};
This feature simplifies reverse indexing in initializations, making the code more readable and eliminating the need for additional logic to handle index calculations from the end of a collection.
Conclusion
C# 13 latest features includes improvements that simplify development and improve code readability and performance. The enhanced params collections, modernized synchronization with the Lock object, intuitive \e escape sequence, optimized method group resolution and improved implicit indexing together make a more expressive programming experience. These features reflect how C# is evolving to meet modern development needs while remaining simple and clear. By adopting these advancements, developers can create more succinct and efficient code, keeping their tasks in the forefront of technology.
Additional Resources
Our team of software development experts is here to transform your ideas into reality. Whether it's cutting-edge applications or revamping existing systems, we've got the skills, the passion, and the tech-savvy crew to bring your projects to life. Let's build something amazing together!
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.