Creating a Reusable Toast Component in Angular
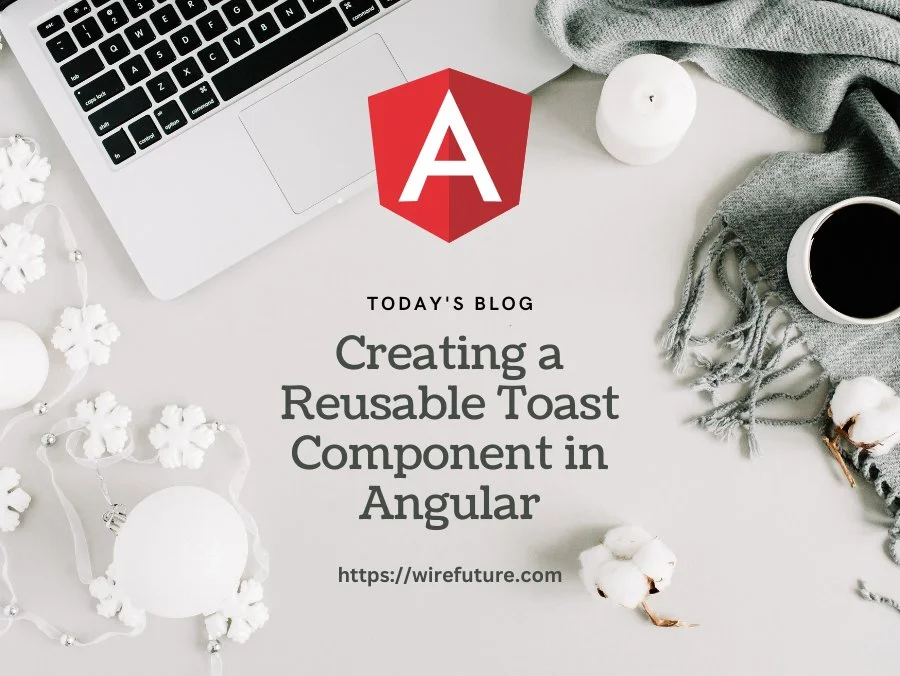
In the quickly transforming world of web development, notifications provide users real-time feedback. Whether it’s to confirm an action, alert users of an error or simply show informational messages, toast notifications can help improve user experience. Reusable components are an absolute necessity for an Angular development company looking to simplify their application development process. This article demonstrates exactly how to build a reusable toast component in Angular using ng-bootstrap. Finally, you have an effective solution that you can embed in any Angular project and use for consistent and efficient notification handling across your applications.
For those interested in learning more about Angular development, check out our Angular Development blogs. Stay updated with the latest insights and best practices!
Prerequisites
Before we begin, ensure you have the following installed:
- Node.js
- Angular CLI
- ng-bootstrap
You can install Angular CLI globally using npm:
npm install -g @angular/cli
To add ng-bootstrap to your Angular project, run:
ng add @ng-bootstrap/ng-bootstrap
Step 1: Setting Up the Angular Project
First, create a new Angular project if you don’t already have one:
ng new angular-toast-demo
cd angular-toast-demo
Step 2: Installing ng-bootstrap
If you haven’t already added ng-bootstrap, you can do so by running the following command:
ng add @ng-bootstrap/ng-bootstrap
Step 3: Creating the Toast Service
Create a service to handle the toasts. This service will manage the list of toasts and provide methods to add and remove toasts.
Generate the service using Angular CLI:
ng generate service toast
Update the generated service (toast.service.ts
) as follows:
import { Injectable } from '@angular/core';
interface Toast {
message: string;
header?: string;
classname?: string;
delay?: number;
}
@Injectable({
providedIn: 'root'
})
export class ToastService {
toasts: Toast[] = [];
show(message: string, options: Partial<Toast> = {}) {
this.toasts.push({ message, ...options });
}
remove(toast: Toast) {
this.toasts = this.toasts.filter(t => t !== toast);
}
}
Step 4: Creating the Toast Component
Generate a new component for the toast:
ng generate component toast
Update the component (toast.component.ts
and toast.component.html
) to display the toasts.
toast.component.ts
import { Component } from '@angular/core';
import { ToastService } from '../toast.service';
@Component({
selector: 'app-toast',
templateUrl: './toast.component.html',
styleUrls: ['./toast.component.css']
})
export class ToastComponent {
constructor(public toastService: ToastService) { }
}
toast.component.html
<ngb-toast
*ngFor="let toast of toastService.toasts"
[class]="toast.classname"
[header]="toast.header"
[autohide]="true"
[delay]="toast.delay || 5000"
(hide)="toastService.remove(toast)">
{{ toast.message }}
</ngb-toast>
toast.component.css
ngb-toast {
position: fixed;
top: 20px;
right: 20px;
z-index: 1200;
}
Step 5: Adding the Toast Component to the Application
To make sure the component is available throughout the application, include it in the AppComponent
.
Update app.component.html
to include the <app-toast>
selector:
app.component.html
<router-outlet></router-outlet>
<app-toast></app-toast>
Step 6: Using the Toast Service
Inject the ToastService
into a component to start showing toasts. For example, let’s use it in app.component.ts
:
app.component.ts
import { Component } from '@angular/core';
import { ToastService } from './toast.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private toastService: ToastService) { }
showToast() {
this.toastService.show('Hello, this is a toast message!', { header: 'Notification', classname: 'bg-success text-light' });
}
}
app.component.html
<button (click)="showToast()">Show Toast</button>
<router-outlet></router-outlet>
<app-toast></app-toast>
Step 7: Customizing the Toasts
You can customize the appearance and behavior of the toasts by passing different options when calling the show
method. For example, you can set different classes, headers, and delays:
this.toastService.show('Success message!', { classname: 'bg-success text-light', delay: 10000 });
this.toastService.show('Error message!', { classname: 'bg-danger text-light', delay: 5000 });
this.toastService.show('Info message!', { header: 'Information', classname: 'bg-info text-light', delay: 7000 });
For those interested in learning more about Angular development, check out our Angular Development blogs. Stay updated with the latest insights and best practices!
Conclusion
With these steps you created a reusable toast component in Angular with ng-bootstrap. This component could be utilized to show various kinds of notifications across your application. You can extend this component to add additional animation styles, icons or buttons to the toasts.
This approach allows code reuse and a consistent look and feel for notifications across your application.
From initial concept to final deployment, WireFuture is your partner in software development. Our holistic approach ensures your project not only launches successfully but also thrives in the competitive digital ecosystem.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.