Exploring Latest Features in Angular 17
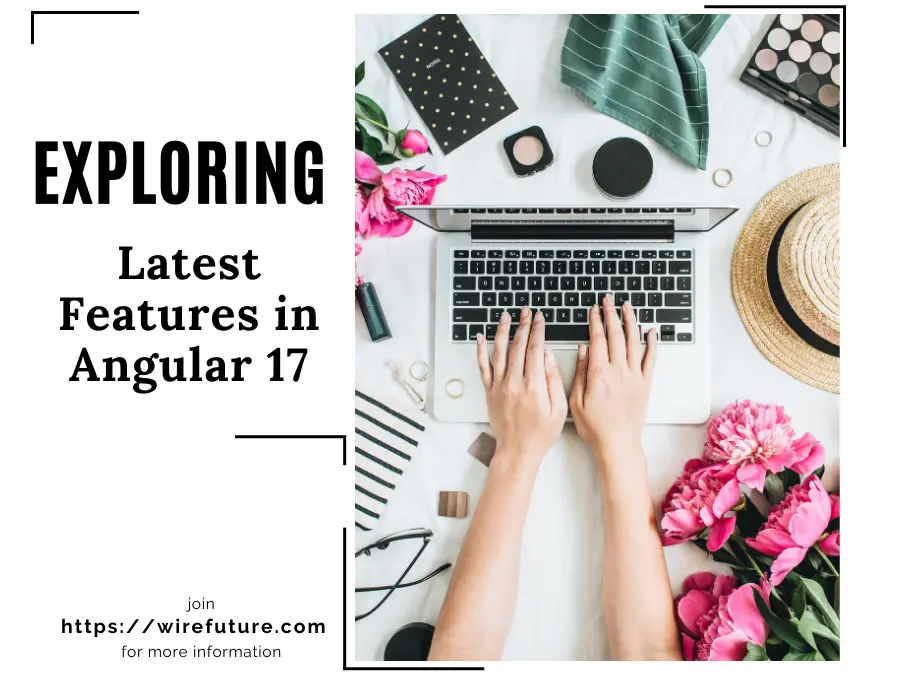
With the quickly transforming world of web development, staying abreast of new capabilities and tools is crucial for developing effective modern programs. Angular 17, the platform famous for its robust ecosystem and developer toolset, has raised the bar once again. This new build is infused with performance refinements, productivity gains, and development process simplifications.
From standalone components to TypeScript 5.2 integration, Angular 17 was built to meet the demands of today’s web development landscape while delivering fast, responsive yet easy-to-build and maintain applications. This article discusses the numerous latest features in Angular 17 and how each release can improve your development process and your projects. Whether you are an Angular veteran or a fresher, understanding these new capabilities is vital to unleashing Angular to your next project. So let’s get started.
Table of Contents
- Performance Enhancements for Control Flow
- Deferrable Views for Lazy Loading
- Standalone Components
- Server-Side Rendering (SSR) Improvements
- Dependency Injection Debugging Tools
- Support for TypeScript 5.2
- Advanced Routing and Lazy Loading
- Material 3 Support
- Conclusion
- FAQ on Latest Features in Angular 17
Performance Enhancements for Control Flow
As the weekend draws near, enjoy a quick 100-second recap of @Angular 17's newest features. 🚀
— ng-news (@_ng_news) November 17, 2023
Happy weekend! pic.twitter.com/KecQw3zrdN
To dive deeper into the Performance Enhancements for Control Flow in Angular 17, let’s explore how these improvements can significantly boost the speed for initial page loads and updates, particularly focusing on the display of lists and dynamic content. Angular 17 introduces a new syntax and optimizations for iterating over collections, which is a common task in web development. This makes rendering lists and managing dynamic content more efficient.
Simplifying Lists with @for
The @for
directive is a new addition that simplifies looping over arrays and collections. This directive not only makes the syntax more intuitive but also enhances performance by optimizing the way Angular tracks changes and updates the DOM. Let’s compare the new syntax with the traditional *ngFor
.
Traditional *ngFor
Example:
<ul>
<li *ngFor="let item of items; index as i">
{{ i }}: {{ item.name }}
</li>
</ul>
New @for
Syntax:
<ul @for="let item of items; trackBy: trackItem">
<li>
{{ item.index }}: {{ item.name }}
</li>
</ul>
In the new syntax, trackBy
is used to specify a tracking function, which improves performance by minimizing DOM manipulations when the list changes.
Optimizing Conditional Rendering with @if
Angular 17 enhances the way conditional content is rendered, making it more efficient and straightforward.
Traditional Conditional Rendering:
<div *ngIf="isLoading; else notLoading">
Loading...
</div>
<ng-template #notLoading>
<div>
Content is loaded.
</div>
</ng-template>
New @if
Syntax:
<div @if="isLoading">
Loading...
</div>
<div @else>
Content is loaded.
</div>
The new @if
syntax eliminates the need for ng-template
and else
template variables, streamlining conditional rendering.
Dynamic Content with Improved Performance
For dynamic content that changes based on user interaction or asynchronous operations, Angular 17’s new control flow directives and optimizations ensure that updates are faster and consume less memory.
Example of Dynamic Content Update:
@Component({
selector: 'my-app',
template: `
<button (click)="addItem()">Add Item</button>
<ul @for="let item of items; trackBy: trackItem">
<li>
{{ item.index }}: {{ item.name }}
</li>
</ul>
`
})
export class AppComponent {
items = [];
addItem() {
this.items.push({ index: this.items.length, name: `Item ${this.items.length}` });
}
trackItem(index, item) {
return item.index; // Use a unique property for tracking
}
}
This example demonstrates adding items to a list with a button click. Using the trackBy
function, Angular can minimize DOM updates, which is especially beneficial for lists that change frequently.
These enhancements in Angular 17 significantly improve the efficiency of rendering lists and dynamic content, leading to faster initial page loads and updates. The simplified syntax for loops and conditional rendering not only makes your code cleaner but also enhances its performance. As you start incorporating these features into your Angular projects, you’ll find your applications becoming noticeably faster and more responsive.
Deferrable Views for Lazy Loading
Deferrable Views for Lazy loading in Angular 17 is a new way of Loading content in web applications. This is a content load management feature that optimizes application performance and user experience by intelligently scheduling content loading steps. Instead of loading all components and data in one go, deferrable views load parts of the application only when they are needed – for example when they enter the viewport or under certain conditions. This method significantly reduces initial load times and enhances resource management.
Using @defer
for Lazy Loading
Angular 17 introduces the @defer
directive, which enables lazy loading of components or content sections in a straightforward and declarative manner. Here’s how you can use it:
Basic Example of @defer
:
<div @defer>
<!-- Content that should be lazy-loaded -->
</div>
In the above example, the content within the div
will be loaded lazily, improving the initial load time of your application.
Conditional Lazy Loading
You can also conditionally load content based on certain criteria, such as when an element comes into the viewport:
<div @defer="isVisible">
<!-- Content that should be lazy-loaded when isVisible is true -->
</div>
In this scenario, the content is only loaded when the isVisible
condition evaluates to true, allowing for more granular control over resource loading.
Using Placeholders with @defer
Angular 17 allows you to specify placeholder content that is displayed while the lazy-loaded content is being prepared. This is particularly useful for improving user experience by providing immediate feedback that content is on its way.
Example with Placeholder:
<div @defer @placeholder="<div>Loading...</div>">
<!-- Lazy-loaded content goes here -->
</div>
This setup displays a loading message until the actual content is ready to be shown.
Combining @defer
with Observables
For even finer control, you can combine @defer
with Angular Observables. This allows you to trigger the loading of content based on events, user actions, or any complex logic defined in your component class.
Observable Example:
// In your component
isVisible$ = new BehaviorSubject(false);
toggleVisibility() {
this.isVisible$.next(!this.isVisible$.value);
}
<!-- In your template -->
<div @defer="isVisible$ | async">
<!-- Content to be loaded based on observable state -->
</div>
By toggling isVisible$
, you can control when the content is lazy-loaded with precision, catering to complex application scenarios.
Advanced Lazy Loading Strategies
Angular 17’s deferrable views support various loading strategies, enabling developers to optimize resource loading further based on user interaction or other criteria:
<div @defer="on hover">
<!-- Content to be lazy-loaded when the user hovers over the element -->
</div>
In this example, content is lazy-loaded when the mouse pointer hovers over the element, which can be particularly useful for elements like dropdown menus or tooltips that don’t need to be loaded immediately.
Through these examples, it’s clear that Angular 17’s deferrable views for lazy loading offer a powerful and flexible way to enhance application performance and user experience. By loading content only when necessary, applications become more responsive and efficient, providing a smoother experience for the end-user.
Standalone Components
Standalone Components in Angular 17 are a new way of building and structuring Angular applications. This makes it incredibly easy to build, test and reuse components – without needing Angular modules (NgModules) in some cases. Standalone components enable developers to declare components, directives, and pipes directly in the component metadata, facilitating the maintenance and scaling of Angular applications.
Basic Example of Standalone Component
In traditional Angular applications, components are declared inside a module, which acts as a container. With Angular 17, you can create a component that is independent of any module.
import { Component } from '@angular/core';
@Component({
selector: 'app-standalone',
standalone: true,
template: `<p>Standalone component works!</p>`,
})
export class StandaloneComponent {}
In the example above, setting standalone: true
in the @Component
decorator marks the component as standalone, meaning it doesn’t need to be declared in an @NgModule
.
Importing Other Components, Directives, and Pipes
Standalone components can import other standalone components, directives, and pipes directly, without needing an Angular module to tie them together.
import { CommonModule } from '@angular/common';
import { Component } from '@angular/core';
import { OtherComponent } from './other.component';
@Component({
selector: 'app-standalone-imports',
standalone: true,
imports: [CommonModule, OtherComponent],
template: `
<p>Standalone component with imports works!</p>
<app-other></app-other>
`,
})
export class StandaloneImportsComponent {}
This approach enhances modularity and reusability, allowing components to encapsulate their dependencies clearly.
Simplifying Angular Applications
Standalone components encourage a more modular and decoupled architecture for Angular applications. They can be particularly beneficial for:
- Small to Medium Projects: Reducing boilerplate and simplifying the project structure.
- Large Projects: Improving maintainability and modularity by encouraging more self-contained components.
- Reusable Libraries and Components: Making it easier to share and reuse components across different projects without worrying about the NgModule dependencies.
Testing Standalone Components
Testing standalone components is more straightforward since there’s no need to configure the TestBed with a module that includes the component, its dependencies, and any required Angular features like routing or forms.
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { StandaloneComponent } from './standalone.component';
describe('StandaloneComponent', () => {
let component: StandaloneComponent;
let fixture: ComponentFixture<StandaloneComponent>;
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [StandaloneComponent],
}).compileComponents();
fixture = TestBed.createComponent(StandaloneComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
This simplified approach can make unit tests easier to write, understand, and maintain.
Standalone components represent a significant step forward in Angular’s evolution, offering a more flexible and straightforward way to create and manage components. This feature aligns with modern development practices, focusing on modularity and ease of use, and it has the potential to make Angular development more accessible and efficient.
Server-Side Rendering (SSR) Improvements
Angular 17 has improved Server-Side Rendering (SSR), which is critical to Angular performance and SE Optimization. With these enhancements, Angular projects can now configure SSR automatically for developers to have a hassle-free setup experience. This improvement not only improves SEO (since crawling and indexing content makes content crawlable and indexable by search engines) but also improves load times for users (since content is rendered pre-loaded on the server reducing the time to first contentful paint).
Automated SSR Configuration
In Angular 17, configuring SSR is much easier with automated configuration tools. In the past, SSR configuration involved time-consuming and error-prone manual adjustments and configurations. These days when creating a brand new Angular project or adding SSR to an existing project, Angular CLI automates much of this.
Lower Load Times & SEO
Angular 17 apps can serve fully rendered pages to clients by pre-rendering content on the server, reducing wait time for first content impressions. This is especially useful for users with slow connections or devices. SSR is also important for SEO as the pre-rendered content can be indexing more efficiently by search engines thereby making Angular applications more visible in search results.
Optimized Tools for SSR
Angular 17 releases new tools and libraries for developers interested in exploring SSR capabilities. These tools aim to give more control and freedom over the rendering process to enable application-specific configurations and optimizations.
For instance, developers can now more easily control the state of transfer between client and server, so that information retrieved during server side rendering is made available to client-side application without being repeated requests. This optimization decreases bandwidth usage and increases performance of the application.
Code Example: Adding SSR to Angular Project
Although the specific commands and settings might vary according to project setup, adding SSR to an Angular project usually has a few key steps:
- Installing the necessary packages: Angular Universal is the technology behind SSR in Angular. To add it to your project, you would use Angular CLI commands that handle the setup and configuration automatically.
- Modifying your application code: To take full advantage of SSR, you might need to make some adjustments to your application code. This could involve ensuring that your application is platform-agnostic, so it can run on both the server and the client.
- Running the SSR server: Once SSR is configured, you can run your Angular application on a server that renders your application to HTML on the fly before sending it to the client.
# Example command to add SSR to an Angular project
ng add @nguniversal/express-engine
This command sets up your Angular app with Angular Universal using an Express.js server. It automatically updates your application with the necessary dependencies, scripts, and configuration files to start using SSR.
Conclusion
Server-Side Rendering improvements in Angular 17 provide a significant performance and SEO boost to Angular applications. With automated configuration, improved tools and overall SSR support, Angular remains a powerful framework for developing responsive web applications. These enhancements make Angular a lot more appealing to programmers who would like feature rich, performant and discoverable applications.
Dependency Injection Debugging Tools
Angular 17 is packed with lots of new features – including its Dependency Injection (DI) debugging tools. Angular’s DI system is one of the core components, allowing developers to clearly declare dependencies for their classes in an easily manageable way. However, DI-related debugging issues such as missing providers, wrong scope configurations, or circular dependencies have sometimes proven challenging. The new builds greatly simplify debugging, making it much easier for developers to find and fix component connection problems.
Enhanced Debugging Experience
Angular 17 developers can expect a much simpler debugging interface. The framework now provides more accurate diagnostics and clearer error messages to make diagnosing DI-related problems easier. These improvements clarify the nature of DI errors and direct developers to the source of the problem – whether it’s a missing dependency, incorrect provider scope, or another configuration oversight.
Tools and Utilities for DI Debugging
Angular 17 introduces new tools and utilities designed specifically for diagnosing and fixing DI problems. These tools allow developers to:
- Inspect the Injector Hierarchy: Developers can now easily view the structure of Angular’s injector hierarchy at runtime. This is crucial for understanding how dependencies are provided and resolved in complex applications.
- Analyze Component Dependencies: Enhanced tooling provides a clearer overview of a component’s dependencies, including which dependencies are missing or improperly configured. This makes it easier to verify that components are correctly set up to receive the services and values they require.
- Visualize Dependency Graphs: Some of the new debugging tools offer visual representations of dependency relationships, helping developers intuitively grasp how components and services are interconnected within their application.
Support for TypeScript 5.2
One the prominent latest features in Angular 17 is its adoption toTypeScript 5.2, further enhancing its development capabilities with performance, error handling, and code management improvements. TypeScript 5.2 introduces a number of key features and enhancements that Angular 17 uses to offer a cleaner and more efficient development environment.
More Quickly Change Detection
TypeScript 5.2 introduces optimizations for faster change detection in Angular applications. This is especially helpful for big apps where detecting changes quickly could make a huge difference to performance. With the latest TypeScript compiler optimizations, Angular 17 can compile and update applications much faster resulting in faster user experiences.
Smarter Error Prevention Software
Perhaps the most prominent characteristic of TypeScript is its ability to identify errors at compile time which could usually be discovered solely at runtime in average JavaScript. TypeScript 5.2 adds even more advanced type-checking and error prevention features. This means Angular developers can write more stable code with fewer bugs. Angular 17 fully utilizes these improvements, allowing developers to quickly catch issues early on in the development cycle, and minimizing debugging time.
More Sensible Code Shorthand Techniques
New shorthand techniques and syntax enhancements also make code more readable and concise, and TypeScript 5.2 also includes new shorthand methods. These include improvements to tuple types, template string types, and more. These features let Angular 17 developers write expressive, cleaner and easier to maintain code.
For instance, TypeScript 5.2 introduces more lenient control of tuple types, which can be particularly useful when using Angular’s reactive forms or HTTP client services. It also allows developers to set exact types for array responses from APIs or form values, further enhancing code accuracy and security.
// Example using TypeScript 5.2 features in Angular
function getApiResponse(): [number, string] {
return [200, 'OK'];
}
const [status, message] = getApiResponse();
console.log(status); // 200
console.log(message); // 'OK'
In this example, the function getApiResponse
is explicitly typed to return a tuple of a number and a string. TypeScript 5.2 ensures that the destructured assignment matches the expected types, providing compile-time safety and clarity.
Conclusion
Angular 17 introduces TypeScript 5.2, which improves the performance and reliability of the framework as well as the developer experience with stronger typing capabilities and syntax enhancements. These improvements result in a faster and error-free development process, allowing developers to create high – complexity applications. With Angular growing alongside TypeScript, developers can expect even more robust and feature-rich web development tools.
Advanced Routing and Lazy Loading
Angular 17 introduces advanced routing and lazy loading features that significantly change the way applications load resources. This new design makes resources and components load only when needed, making Angular apps faster and the user experience more pleasant.
Intelligent Resource Loading
The smarter use of resources is at the heart of Angular’s advanced routing and lazy loading. Angular supported lazy loading in previous versions, but Angular 17 adds support for lazy loading via syntax enhancements and mechanisms for specifying loadable routes and modules. This allows the framework to know your application’s structure more effectively and know when to load specific pieces of code.
Angular Routing for Lazy Loading: Example
Lazy loading using the Angular Router is simplified in Angular 17. Routes can be defined to load modules only when navigated to, lowering the initial load time of the application.
const routes: Routes = [
{
path: 'feature',
loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule)
}
];
In this example, the FeatureModule
is not loaded until the user navigates to the /feature
path. This on-demand loading significantly reduces the initial bundle size, leading to faster application startup times.
Preloading Strategies
Angular 17 enhances the framework’s routing capabilities by providing more control over preloading strategies. Developers can now fine-tune how and when modules are preloaded, ensuring that resources are loaded in the most efficient manner possible.
Angular provides several out-of-the-box preloading strategies, but developers can also create custom strategies to meet specific performance or user experience goals.
RouterModule.forRoot(routes, {
preloadingStrategy: PreloadAllModules
});
This snippet demonstrates how to configure the router to preload all lazy-loaded modules after the initial load, balancing the need for quick initial load times with the desire to have other parts of the application loaded and ready to go.
Granular Loading with Guards and Resolvers
To further optimize the loading process, Angular 17 makes use of guards and resolvers within the routing framework. Guards can be used to prevent modules from loading until certain conditions are met, such as user authentication. Resolvers, on the other hand, can preload data for a component before it’s rendered, ensuring that all necessary data is available upfront.
{
path: 'dashboard',
component: DashboardComponent,
resolve: { data: DashboardResolver }
}
This configuration ensures that the DashboardComponent
only loads once the DashboardResolver
has fetched the necessary data, improving the user experience by preventing the display of incomplete views.
Angular 17 adds advanced routing and lazy loading, providing Angular apps With faster load times and a smoother user experience – not only making Angular faster but also scalable for large/diverse apps – As developers, take advantage of These new features to improve your Angular apps ‘performance and user experience.
Material 3 Support
Material 3 support in Angular 17 is a significant step forward in the design and functionality of Angular applications. Material design has long been an essential element for creating intuitive and pleasing user interfaces, and with Material 3 the Design possibilities are radically expanded. Material 3 is an extensible design system that enables greater customization and a more universal experience across platforms and devices.
Accepting Material 3 Design Principles
Material 3, or Material You, redefines the user experience design with a personal touch that emphasizes adaptability, comfort and expression. With Angular 17 incorporating these principles developers can now build apps that are beautiful while also being expressive to users’ tastes and preferences.
Improved Theming Capabilities
Material 3 is notable for its theming capabilities, which can now be further customized and dynamically theming according to the user preference or system settings. Angular 17 apps can use these to create a more personalised user experience, with themes that can adapt to users’ individual needs – like light or dark mode preferences.
Robustness of Functionality Across Devices and Platforms
Material 3 design principles ensure that applications are not only beautiful but also cross-device and cross-platform compatible. This is particularly crucial in a multi-device world where users demand an identical experience on their mobile, tablet or even desktop.
Example of Using Material 3 in Angular 17
Material 3 is easy to integrate with Angular 17, and with Material 3 you only need a project. The framework also supports Material 3, allowing developers to easily integrate Material components and theming, creating an integrated design language across the application. For example, installing Material 3 theme requires configuring your Angular project to use Material 3 library and then configuring the theme to your needs.
// Importing Material modules in your Angular module
import { MatSliderModule } from '@angular/material/slider';
@NgModule({
imports: [MatSliderModule],
})
export class AppModule {}
This snippet shows how to import a Material component into an Angular module. From there, you can utilize the full range of Material 3 components and theming options to design your application.
Conclusion
Angular 17 is a significant advancement for the framework that features several enhancements and new features to simplify development, accelerate software performance and deliver a much better user experience. From standalone components that simplify app architecture to server-side rendering for faster load times and better SEO, Angular 17 is set to redefine web application development. TypeScript 5.2 integration gives developers greater tools to write clean, error-free code and the new build system promises to make the development cycle even faster and more efficient.
For enterprises and development teams aiming to take advantage of these improvements, upgrading to Angular 17 is a smart move. But a successful migration requires planning, understanding the latest features in Angular 17, and a team with experience moving the changes without disrupting existing operations. And this is where we comes in. Migrating to Angular 17 with the help of a seasoned web application development company like WireFuture not only ensures that you can fully capitalize on these new features but also positions your applications at the forefront of modern web development practices. Whether you’re looking to hire Angular developers or seeking comprehensive support for your Angular projects, WireFuture is your partner in navigating the future of web development with Angular 17.
FAQ on Latest Features in Angular 17
Standalone components are a new feature in Angular 17 that allows developers to create components that don’t require an Angular module (NgModule
) for declaration. This simplifies app architecture and makes components easier to reuse and manage.
Angular 17 introduces enhancements to SSR, including automatic configuration for new projects, which improves SEO and load times. It offers better tools for developers diving deep into SSR functionalities, making websites faster and more accessible to search engines.
The new build system in Angular 17 aims to provide faster build times, improve developer experience, and efficiently manage project assets and dependencies. It leverages modern tooling to optimize the development and deployment process.
Angular 17 embraces TypeScript 5.2, offering developers faster change detection, smarter error prevention, and more intuitive code shorthand methods. This results in cleaner, more reliable code.
Yes, Angular provides tools and guidelines to help developers migrate existing applications to Angular 17. The process involves updating dependencies and making necessary adjustments to take advantage of the new features and improvements.
Deferrable views introduce a new approach to lazy loading in Angular 17, allowing content to be loaded only when necessary. This feature enhances app performance and user experience by intelligently managing resource loading.
With Material 3 support, Angular 17 allows developers to utilize the latest design principles and components from Google’s Material Design. This ensures apps are both aesthetically pleasing and functionally robust across devices.
Angular 17 introduces advanced routing and lazy loading features, providing more intelligent handling of application loading processes. This ensures resources are only loaded when necessary, offering a smoother user experience.
Angular 17 includes new tools for debugging dependency injection issues, making it easier for developers to identify and resolve component connection problems. This improves the development workflow and application stability.
The official Angular documentation (https://angular.io/docs) is the best place to start for detailed information on Angular 17’s features, migration guides, and more. It provides comprehensive resources to help developers utilize the full capabilities of Angular 17.
WireFuture's team spans the globe, bringing diverse perspectives and skills to the table. This global expertise means your software is designed to compete—and win—on the world stage.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.