Fixing 500 Internal Server Error in ASP.NET Core: Practical Guide
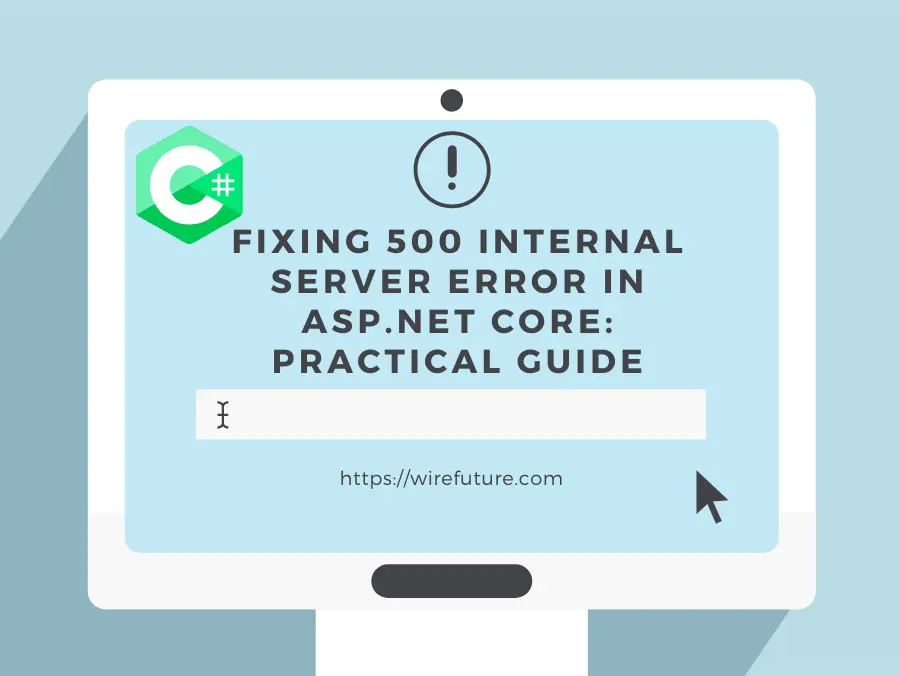
The dreaded 500 Internal Server Error in ASP.NET Core can be a headache for developers and users alike. This article will guide you through the steps to diagnose, understand, and fix this common error. We’ll cover various scenarios, provide code snippets, and include images to make the process easier.
Note: This post is to debug the 500 internal server in ASP.NET Core. If you want to instead debug for ASP.NET framework you can read this article instead: https://wirefuture.com/post/how-to-fix-500-internal-server-error-in-asp-net
Table of Contents
- What is a 500 Internal Server Error?
- Common Causes of 500 Internal Server Error
- How to Fix 500 Internal Server Error
- Conclusion
What is a 500 Internal Server Error?
A 500 Internal Server Error indicates that something has gone wrong on the server side, but the server cannot be more specific about what the exact problem is. This could be due to a variety of issues such as misconfigurations, runtime errors, or even server resource limitations. As an ASP.NET development company, we frequently encounter and resolve these issues, ensuring that your applications run smoothly and efficiently.
Common Causes of 500 Internal Server Error
1. Misconfigured Application Settings
ASP.NET Core applications rely on configuration settings defined in appsettings.json
or environment variables. Incorrect settings can cause the application to fail at runtime.
2. Unhandled Exceptions
Unhandled exceptions in your code can result in a 500 error. These might be due to null references, invalid operations, or other unexpected conditions.
3. Database Connection Issues
Problems with the database connection, such as incorrect connection strings or database server downtime, can also lead to this error.
4. Deployment Issues
Incorrect deployment settings or missing dependencies on the server can cause the application to crash.
How to Fix 500 Internal Server Error
1. Enable Detailed Error Messages
First, enable detailed error messages in the Startup.cs
file to get more information about what’s going wrong. During development, this helps you see the stack trace and error details.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
In production, you should use custom error pages to avoid exposing detailed error information to users.
2. Check Application Configuration
Ensure that all configuration settings are correct. Verify your appsettings.json
file and any environment variables.
appsettings.json
{
"ConnectionStrings": {
"DefaultConnection": "Server=your_server;Database=your_db;User Id=your_user;Password=your_password;"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning"
}
},
"AllowedHosts": "*"
}
Environment Variables
Make sure environment variables are set correctly, especially for sensitive data like connection strings. In your terminal or deployment environment, set these variables.
export ASPNETCORE_ENVIRONMENT=Development
export DefaultConnection="Server=your_server;Database=your_db;User Id=your_user;Password=your_password;"
3. Handle Exceptions Properly
Implement global exception handling to catch unhandled exceptions and log them. This helps in identifying the root cause of errors.
ConfigureServices in Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
services.AddLogging();
}
Configure in Startup.cs
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILogger<Startup> logger)
{
app.UseExceptionHandler(a => a.Run(async context =>
{
var exceptionHandlerPathFeature = context.Features.Get<IExceptionHandlerPathFeature>();
var exception = exceptionHandlerPathFeature.Error;
logger.LogError(exception, "Unhandled exception occurred");
context.Response.StatusCode = 500;
await context.Response.WriteAsync("An unexpected error occurred. Please try again later.");
}));
// other middleware registrations...
}
4. Check Database Connectivity
Ensure your application can connect to the database. Verify the connection string and test the connection.
DbContext Configuration in Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
}
Test Database Connection
Create a simple test to check database connectivity.
public class HomeController : Controller
{
private readonly ApplicationDbContext _context;
public HomeController(ApplicationDbContext context)
{
_context = context;
}
public IActionResult TestDb()
{
try
{
_context.Database.CanConnect();
return Ok("Database connection successful");
}
catch (Exception ex)
{
return StatusCode(500, "Database connection failed: " + ex.Message);
}
}
}
5. Verify Deployment Settings
Ensure all necessary files and dependencies are included in your deployment package. Double-check the deployment settings in your hosting environment.
Publish Your Application
Use the following command to publish your application.
dotnet publish --configuration Release
Verify Deployment Package
Ensure the necessary files are present in the deployment package, such as the appsettings.json
, binaries, and dependencies.
Configure Web Server
Ensure your web server (IIS, Kestrel, Nginx, etc.) is configured correctly to host your ASP.NET Core application.
dotnet yourapp.dll
Conclusion
Fixing a 500 Internal Server Error in ASP.NET Core involves systematically checking your application’s configuration, handling exceptions properly, ensuring database connectivity, and verifying deployment settings. By following these steps, you can diagnose and resolve the issue, ensuring a smoother experience for your users. If you need expert assistance, consider to hire ASP.NET developers who can efficiently troubleshoot and resolve such errors, ensuring your applications remain reliable and performant.
Remember to regularly log and monitor your application to catch and resolve issues early. Happy coding!
Success in the digital age requires strategy, and that's WireFuture's forte. We engineer software solutions that align with your business goals, driving growth and innovation.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.