Getting Started with Laravel: A Journey to Web Development Excellence
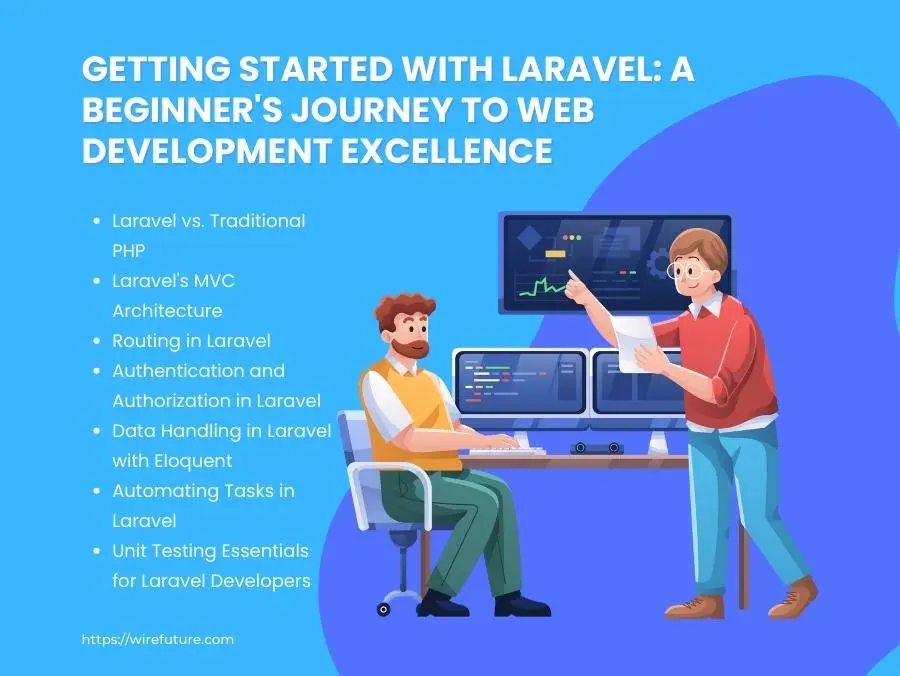
When you dive into web development, it’s easy to find yourself at a crossroads and choose the right framework that will not only simplify our coding process but also enhance functionality and scale for your applications. Enter Laravel, a shining star of the PHP framework universe, which has been praised for its elegance, modern features, and comprehensive ecosystem. Laravel has been a game changer since it was founded in 2011, bringing joy and productivity to developers across the globe.
- Introduction to Laravel
- Laravel vs. Traditional PHP
- Laravel’s MVC Architecture
- Routing in Laravel
- Authentication and Authorization in Laravel
- Data Handling in Laravel with Eloquent
- Introduction to Automating Tasks in Laravel
- Unit Testing Essentials for Laravel Developers
- Conclusion
Introduction to laravel
Laravel is designed from the ground up with simplicity and developer satisfaction in mind. It’s as if you’re always reaching for that one tool in your arsenal, not just because it’s going to do the job, but because it’s going to be fun. Laravel’s expressive, elegant syntax is intuitive enough for first time developers and powerful enough to experienced programmers. This balance makes Laravel an attractive choice for a wide range of projects, from small websites to large-scale enterprise applications.
The robust Laravel ecosystem, which includes everything from lightweight templating engines (Blade) to a complete package for building strong APIs (LUMEN), is one of the most compelling reasons why you should choose Laravel. Laravel’s ecosystem is like a well-oiled machine, designed to work seamlessly together, providing tools like Laravel Mix for front-end asset compilation, and Laravel Nova for administration panel design. This ecosystem ensures that developers have access to an arsenal of tools capable of handling virtually any task, which speeds up and facilitates the development process.
In addition, the Laravel community is a thriving and encouraging one where developers have access to an extensive range of resources, trainings as well as forums for sharing knowledge, troubleshooting problems and working together on projects. In particular, in dealing with difficult problems or new aspects of the framework, such a community dimension is invaluable.
Laravel vs. Traditional PHP
While classic PHP is widely used by developers, it requires them to write more boilerplate code and manual integration of features that are commonly found in the majority of web applications. Laravel, in contrast, has a lot of features built into the box that you can use out of the box like Advanced Routing, Middleware, Templating and an excellent ORM (Object-Relational Mapping). These features make it easier for developers to focus on the unique features of their applications, rather than reinvent the wheel for common tasks.
Moreover, Laravel’s embrace of modern PHP principles, such as object-oriented programming, and its adherence to the MVC (Model-View-Controller) architecture, result in cleaner, more maintainable code. This provides an important advantage over traditional PHP scripts, which can get complicated and difficult to manage as projects are developed.
Laravel also boasts a vibrant ecosystem, including a robust package manager (Composer) and a wealth of packages developed by the community, which can further accelerate development. In essence, compared to traditional PHP programming, Laravel offers a more structured, efficient, and enjoyable development experience. This not only increases the quality of the resulting applications, but also increases the productivity and satisfaction of the developers.
Laravel’s MVC Architecture
Laravel’s adoption of the Model-View-Controller architecture is a testament to its commitment to clean, organized, and modular web development. This architectural approach allows the application logic to be separated by three components, thus providing for a systematic and efficient way of designing applications.
The Model component is responsible for the data and business logic, the View component is responsible for the display of information, and the Controller is connecting the Model and View components to the business logic, responding to user inputs and performing interactions with data model objects.
This separation of interests enables developers to work on each component and not affect the others, which will make a code more usable, maintainable or robust. In other words, developers can update the business logic in a way that does not change the user interface. Since each component can be independently verified, this is also helpful for debugging and testing.
Laravel’s MVC architecture promotes not only productivity and high-quality code, but also best practice in web development. The developers can ensure that their applications are organized, adaptable to changes and able to grow through the use of Laravel’s MVC architecture.
Routing in Laravel
Routing in Laravel is a powerful feature that allows developers to map web requests to specific controller actions or closure functions, enabling a clean and expressive way of organizing web application routes. Laravel makes it easy for developers to choose the best approach to their application by supporting both closure-based and controller-based routes. Closure based Routing is convenient and easy to use, ideal for small applications or simpler paths.
Here’s an example:
Route::get('/', function () {
return 'Welcome to our application!';
});
For more complex applications, controller-based routing offers a structured approach. Routes are defined in the web.php file and linked to controller actions:
Route::get('/user/{id}', 'UserController@show');
RESTful resource controllers, which allow CRUD operations to be executed in a simple manner, are also supported by Laravel. A resource controller is created by the command:
php artisan make:controller UserController –resource
This controller can be registered with a single route:
Route::resource('users', 'UserController');
The Laravel Routing System is very flexible, supporting route parameters, named routes, and middleware, which allows for complex routing needs:
Route::get('/post/{post}', function ($postId) {
// ...
})->middleware('auth');
Route::get('/dashboard', function () {
return view('dashboard');
})->name('dashboard');
Authentication and Authorization in Laravel
Laravel’s authentication and authorization system is designed to ensure that only authenticated users will be able to interact with some parts of the application, allowing them to perform particular tasks. This guide, together with code samples, will examine how authentication and authorization can be implemented in Laravel.
Authentication in Laravel
Laravel makes it easy to use authentication. This includes builtin authentication and user registration, which can be set up with a single command:
php artisan make:auth
To reset login, registration or password, this command will build up the required routes, views and controllers. You can use the Auth facade to configure authentication routes on a manual basis:
Auth::routes();
A user authentication controller can be created after that. For example, to verify the user’s identity:
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
class LoginController extends Controller
{
public function authenticate(Request $request)
{
$credentials = $request->validate([
'email' => 'required|email',
'password' => 'required',
]);
if (Auth::attempt($credentials)) {
$request->session()->regenerate();
return redirect()->intended('dashboard');
}
return back()->withErrors([
'email' => 'The provided credentials do not match our records.',
]);
}
}
Authorization in Laravel
Laravel handles authorization via Gates and Policies. Gates provide a simple closure based approach, whereas policies are class based and ideal for more complex authorization logic.
Using Gates
You define Gates in the AuthServiceProvider
using the Gate
facade. Gates determine if a user can perform an action:
use Illuminate\Support\Facades\Gate;
Gate::define('edit-post', function ($user, $post) {
return $user->id === $post->user_id;
});
You can then use these gates in your controllers:
if (Gate::allows('edit-post', $post)) {
// The user can edit the post...
}
Using Policies
Policies are classes that organize authorization logic around a particular model or resource. You can generate a policy using Artisan:
php artisan make:policy PostPolicy --model=Post
In the generated policy, you can define methods that correspond to different actions a user might perform:
public function update(User $user, Post $post)
{
return $user->id === $post->user_id;
}
You can then use this policy in your controller:
public function update(Request $request, Post $post)
{
$this->authorize('update', $post);
// The current user can update the post...
}
Middleware for Authentication and Authorization
Laravel has a middleware that checks authenticated users and authorises actions. The auth middleware ensures that a route is accessible only by authenticated users:
Route::get('/profile', function () {
// Only authenticated users may enter...
})->middleware('auth');
For authorization, you can use policies directly within routes:
Route::post('/post/{post}', function (Post $post) {
// Authorization logic here...
})->middleware('can:update,post');
Data Handling in Laravel with Eloquent
Laravel’s Eloquent ORM is a shining jewel in the arsenal, which offers an Active Record Implementation that makes database interactions easier. By treating database tables as classes and rows as objects, Eloquent allows for a more intuitive and expressive syntax for querying and manipulating data. A seamless way to manage database schemas and performing CRUD operations is provided by this integration of Eloquent ORM with Laravel’s migration feature.
Using Eloquent ORM with Migrations
You can easily modify and share your application’s database schema with Laravel migration, which is similar to version control for your database. In order to ensure that your database and your application’s data structures remain in sync, they are coupled beautifully with Eloquent models.
Here’s how to create a migration and a corresponding Eloquent model for a posts
table:
// Creating a migration
php artisan make:migration create_posts_table --create=posts
// Creating an Eloquent model
php artisan make:model Post
This migration can be used to define the structure of the posts
table, and the Post
model will interact with this table.
CRUD Operations with Eloquent ORM
Inserting Records
To insert a new record into the posts
table:
$post = new Post;
$post->title = 'My First Post';
$post->content = 'Content of the first post';
$post->save();
Updating Records
To update an existing record:
$post = Post::find(1); // Find the post with ID 1
$post->title = 'Updated Title';
$post->save();
Deleting Records
To delete a record:
$post = Post::find(1);
$post->delete();
Retrieving All Records
To get all records from the posts
table:
$posts = Post::all();
Retrieving a Record by ID
To find a record by its ID:
$post = Post::find(1);
Laravel’s Eloquent ORM and migrations together offer a robust system for database management and data manipulation, ensuring developers can focus on crafting features rather than wrestling with database queries. This combination not only accelerates the development process, but also increases the durability and scale of applications.
Introduction to Automating Tasks in Laravel
To allow developers to focus on building features instead of managing cron jobs, Laravel task scheduling simplifies the management of regular tasks. Laravel’s script is intended to be executed within the UNIX cron daemon, which will execute specified tasks on a regular basis. This elegant solution avoids the need to create multiple cron entries for each task, streamlining the scheduling process.
Setting Up Task Scheduling
First, you need to add a single cron entry on your server that runs the Laravel scheduler every minute:
* * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1
This command ensures that Laravel evaluates your scheduled tasks every minute and runs them if their conditions are met.
Defining Scheduled Tasks
You define your scheduled tasks in the schedule
method of the App\Console\Kernel
class. Here’s how to schedule a closure to run daily:
protected function schedule(Schedule $schedule)
{
$schedule->call(function () {
Log::info("Scheduled task run successfully.");
})->daily();
}
Scheduling Artisan Commands
Laravel makes it incredibly simple to schedule Artisan commands:
$schedule->command('inspire')->hourly();
Scheduling Queue Jobs
You can also schedule queue jobs:
$schedule->job(new HeartbeatJob)->everyFiveMinutes();
Scheduling Shell Commands
Even external shell commands can be scheduled directly:
$schedule->exec('node /home/forge/script.js')->dailyAt('02:00');
Unit Testing Essentials for Laravel Developers
Testing is not only a feature of Laravel; it’s an integral part of the framework. Laravel has been packed with support for PHPUnit, which makes it easy to test in the unit. Moreover, for browser testing, Laravel Dusk provides an expressive, easy-to-use API for testing your application’s user interface.
Unit Testing
Laravel’s built-in PHPUnit support allows you to test your application thoroughly. Here’s a simple unit test example:
/** @test */
public function it_can_add_two_numbers()
{
$this->assertEquals(4, 2 + 2);
}
To test a user model, you might have:
/** @test */
public function a_user_has_a_name()
{
$user = new User(['name' => 'John']);
$this->assertEquals('John', $user->name);
}
Browser Testing with Laravel Dusk
Laravel Dusk enables browser testing, allowing you to interact with your application as a user would:
/** @test */
public function a_user_can_login()
{
$this->browse(function (Browser $browser) {
$browser->visit('/login')
->type('email', 'user@example.com')
->type('password', 'password')
->press('Login')
->assertPathIs('/home');
});
Laravel’s testing tools ensure that your applications are robust, reliable, and ready for production.
Conclusion
In conclusion, Laravel stands out as a comprehensive framework that elegantly simplifies web development challenges. Laravel ensures that your development process is efficient and enjoyable, thanks to its robust MVC architecture, the seamless ORM of Eloquent, and the sophisticated authentication and authorization capabilities. Laravel gives developers the confidence needed to create robust, reliable Web applications by combining powerful unit testing and task scheduling features.
Laravel provides you with the tools and community support to increase your web projects to new heights, whether you are an experienced developer or just starting out. Get to know Laravel and realize the full potential of your web development endeavors.
Are you looking to hire Laravel developers? With unparalleled expertise and commitment to excellence, WireFuture stands out as your ideal Laravel partner. You don’t only get a service provider with WireFuture, you get someone devoted to bringing your vision to life using Laravel.
Trust WireFuture for hiring dedicated Laravel developers to build the foundation of your web development journey, making sure that projects are not only finished but created perfectly.
WireFuture stands for precision in every line of code. Whether you're a startup or an established enterprise, our bespoke software solutions are tailored to fit your exact requirements.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.