How to Implement gRPC in .NET: A Quick Guide
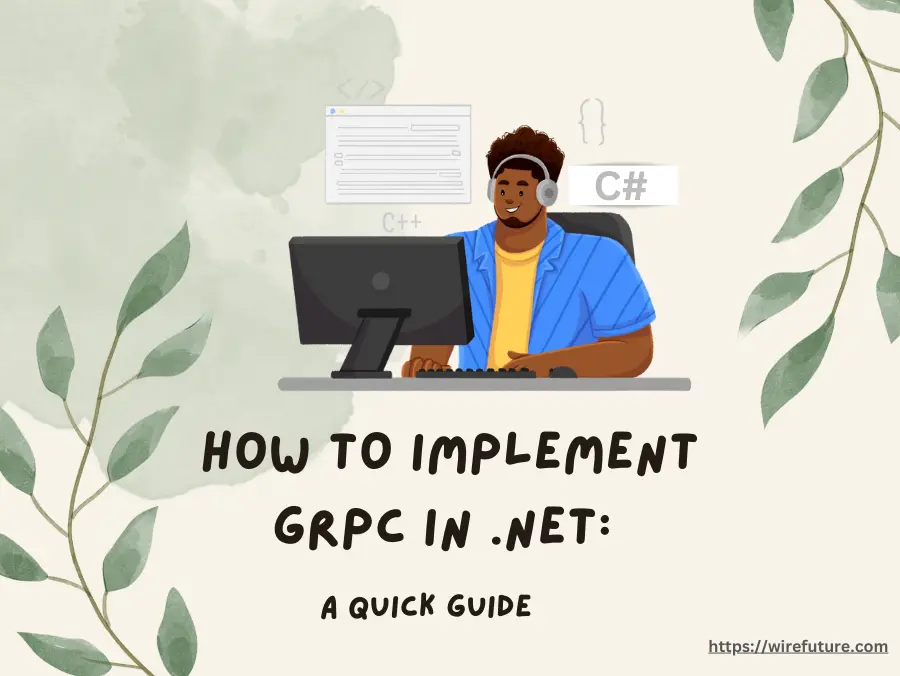
In this post, we’re diving deep into implementing gRPC in .NET, offering a quick and practical guide to seamlessly implementing this powerful system in your applications.. gRPC, short for Google Remote Procedure Call, emerges as a high-performance, open-source framework championed by Google. It aims at facilitating lightning-fast communication between microservices and applications, ensuring that the exchange of data is not merely fast but also robust. The use of HTTP/2 as the main transport protocol is at the heart of GRPC’s effectiveness.
To define both the service interface and the structure of the messages to be sent, gRPC uses Protocol Buffers, commonly known as ProtoBuf. ProtoBuf offers a compact and efficient way to represent your data, making it a powerful binary serialization tool. This efficiency is not just in terms of size, but also by making gRPC extremely flexible across various programming environments due to the ease of cross language serialization and deserialization.
The language’s gnostic nature really makes gRPC stand out. This allows for a wide variety of programming languages to be supported, so that services written in various languages can be integrated and communicated easily. This universality makes gRPC an indispensable tool in the development of complex, scalable, and diverse systems.
In essence, gRPC stands as a robust solution for efficient, cross-language service-to-service communication. It is a testament to the modern technological progress of distributed computing and provides developers with a powerful tool to build interconnected systems that are both fast and reliable.
Table of Contents
- Lightning-fast data exchange and low latency
- gRPC in .NET: Installing the necessary tools and creating a GRPC Service
- gRPC in .NET: Crafting a client to consume the gRPC service
- Tools and strategies for testing gRPC services
- gRPC in .NET: Best practices for deploying gRPC services
- Troubleshooting common Issues
- Conclusion
- Pointers to community resources
Lightning-fast data exchange and low latency
The ability of gRPC to facilitate lightning fast data exchange with exceptionally low latency, a critical aspect for modern applications that requires high performance and real-time responsiveness, is one of the key characteristics of this technology.
This capability is primarily rooted in gRPC’s use of HTTP/2 as its underlying transport protocol, a significant leap forward from the older HTTP/1.1. The HTTP/2 protocol introduces a multiplexing of requests over a single connection, allowing multiple requests and responses to be launched at the same time, which significantly reduces the overhead associated with setting up multiple connections and the latency associated with them.
Moreover, gRPC employs Protocol Buffers (ProtoBuf) for data serialization, which is inherently more efficient than traditional JSON or XML serialization. ProtoBuf is generating compact, binary messages that are both smaller and faster for serialize and deserialize operations. The key to reducing the size of the load and reducing network latency and improving the speed of data transmission between services is this binary format.
In addition, HTTP/2’s ability to further enhance real bidirectional data exchange is supported by GRPC support for streaming and its advanced flow control mechanisms. This enables applications which require continuous data updates or real-time interaction, e.g. for streaming video services, games and Internet of Things device management, to communicate continuously and simultaneously via a send receive communication pattern that is suitable for them.
gRPC in .NET: Installing the necessary tools and creating a GRPC Service
The step-by-step guide is intended to provide the first-time user with a clearly defined roadmap for developing their environment, covering installation of the necessary tools and frameworks as well as demonstrating how they can use Protocol Buffers in order to generate service codes.
Step 1: Install .NET 8 SDK
First and foremost, ensure you have the .NET 8 SDK installed. This software development kit is your toolbox, containing all the necessary components to create, run, and test .NET applications. Download it from the official .NET website.
Verify the installation by opening a command prompt or terminal and running:
dotnet –version
This command should display the version number, confirming the successful installation of the .NET 8 SDK.
Step 2: Create Your gRPC Project
With the .NET SDK ready, it’s time to create a new gRPC project. Open your terminal and execute the following command:
dotnet new grpc -n MyGrpcProject -o MyGrpcProject
This command scaffolds a new gRPC project named MyGrpcProject
in a directory with the same name. Navigate to your project directory:
cd MyGrpcProject
Step 3: Understanding the Project Structure
Upon creation, your project includes a sample protobuf file (greet.proto) located in the Protos directory. This file defines a simple gRPC service. Open greet.proto to explore the predefined service:
syntax = "proto3";
package Greet;
service Greeter {
rpc SayHello (HelloRequest) returns (HelloReply) {}
}
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}
Step 4: Auto-Generate Service Code
ASP.NET Core automatically generates C# code from your protobuf files. This magic happens thanks to the <Protobuf> item group in your project file (MyGrpcProject.csproj). Ensure your .proto file is included:
<ItemGroup>
<Protobuf Include="Protos\greet.proto" GrpcServices="Server" />
</ItemGroup>
This configuration tells the SDK to generate the server-side gRPC code from greet.proto
.
Step 5: Implement the Service
Navigate to the Services directory. Here, you’ll find a C# file named after your service (GreeterService.cs), containing a class that extends the base class generated from your protobuf definition. Here’s how you might implement the SayHello method:
using Grpc.Core;
using System.Threading.Tasks;
public class GreeterService : Greet.Greeter.GreeterBase
{
public override Task<HelloReply> SayHello(HelloRequest request, ServerCallContext context)
{
var reply = new HelloReply
{
Message = $"Hello, {request.Name}"
};
return Task.FromResult(reply);
}
}
Step 6: Run Your Service
To see your gRPC service in action, run it using the .NET CLI:
dotnet run
This command starts a server on localhost, listening for gRPC calls to your GreeterService
.
gRPC in .NET: Crafting a client to consume the gRPC service
A series of simple yet critical steps is required to create a client that can consume gRPC services, establish connections, execute service methods and test your gRPC servers in an.NET 8 console application. This guide provides a clear understanding of how to work effectively with the gRPC service, so that you can understand these processes in detail.
Step 1: Create a .NET Console Application
First, create a new .NET 8 console application. Open your terminal or command prompt and run the following command:
dotnet new console -n GrpcClientProject
cd GrpcClientProject
This command creates a new console application project named GrpcClientProject
and then changes the directory to the project’s folder.
Step 2: Add gRPC Client Packages
To consume a gRPC service, you need to add the necessary NuGet packages to your client project. Run the following commands to install the Grpc.Net.Client and Google.Protobuf packages, along with the Grpc.Tools package for code generation:
dotnet add package Grpc.Net.Client
dotnet add package Google.Protobuf
dotnet add package Grpc.Tools
Step 3: Define the Protobuf Service Contract
Copy the .proto
file from your gRPC service project to your client project, preferably into a folder named Protos
. Then, edit the .csproj
file to include the .proto
file for code generation:
<ItemGroup>
<Protobuf Include="Protos\greet.proto" GrpcServices="Client" />
</ItemGroup>
This instructs the SDK to generate the client-side stubs from your protobuf file during build time.
Step 4: Crafting the Client
In your Program.cs or a new client class, instantiate the gRPC client and call the service methods. First, ensure your client is using the necessary namespaces:
using Grpc.Net.Client;
using Greet; // The namespace declared in your .proto file
Then, establish a connection to your gRPC service and execute a method:
static async Task Main(string[] args)
{
// Set up the channel
using var channel = GrpcChannel.ForAddress("https://localhost:5001");
// Instantiate the client
var client = new Greeter.GreeterClient(channel);
// Create a request
var request = new HelloRequest { Name = "World" };
// Call the service method
var reply = await client.SayHelloAsync(request);
Console.WriteLine($"Greeting: {reply.Message}");
// Keep the console window open
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
}
Step 5: Testing Your gRPC Service
Ensure that your gRPC service is running to test the gRPC client. Next, you’ll be running your client application. If this is done correctly, your client will send a request to the service, which in turn will respond, and you will see a greeting message printed on the console.
This test confirms that the client can communicate with your gRPC service in a successful manner. Use Unit Testing Frameworks, such as xUnit with the GrPC, for more detailed testing. Core. Testing package to mock gRPC services and test various scenarios and edge cases.
Tools and strategies for testing gRPC services
Testing gRPC services effectively ensures that your application communicates as expected, underpinning both the reliability and robustness of your system. The use of a mix of unit and integration tests, in addition to security priorities, entails this. This is a comprehensive overview of the tools, strategies and practices to test gRPC services using NUnit, which is one of the most popular.NET application benchmarking frameworks.
To test gRPC services, you’ll primarily use the Grpc. Core and GrPC.Net.Client libraries that make it easy to create and run gRPC calls in test environments. NUnit is a framework for testing, giving an extensive set of assertions and attributes which can be used to specify and run tests.
Setting Up NUnit
First, ensure your project is set up for NUnit testing. Add the necessary packages to your test project:
dotnet add package NUnit
dotnet add package Microsoft.NET.Test.Sdk
dotnet add package NUnit3TestAdapter
dotnet add package Grpc.Core.Testing
Unit Testing gRPC Services
Unit tests focus on individual components. For gRPC, this means testing service implementations in isolation. Utilize the Grpc.Core.Testing
package to mock gRPC service calls.
using NUnit.Framework;
using Grpc.Core;
using Grpc.Core.Testing;
using System.Threading.Tasks;
[TestFixture]
public class GreeterServiceTests
{
[Test]
public async Task SayHello_ReturnsExpectedMessage()
{
// Arrange
var service = new GreeterService();
var mockCall = TestCalls.AsyncUnaryCall(
Task.FromResult(new HelloReply { Message = "Hello, Test" }),
Task.FromResult(new Metadata()),
() => Status.DefaultSuccess,
() => new Metadata(),
() => { });
// Use Moq or similar to mock the Greeter.GreeterClient if needed
// Act
var response = await service.SayHello(new HelloRequest { Name = "Test" }, mockCall);
// Assert
Assert.AreEqual("Hello, Test", response.Message);
}
}
Integration Testing
Integration tests evaluate the service’s behavior in a more realistic scenario, often involving the actual server and client.
using NUnit.Framework;
using Grpc.Net.Client;
using System;
using System.Threading.Tasks;
[TestFixture]
public class GreeterServiceIntegrationTests
{
private GrpcChannel channel;
private Greeter.GreeterClient client;
[SetUp]
public void SetUp()
{
// Assuming the gRPC service is running locally
channel = GrpcChannel.ForAddress("https://localhost:5001");
client = new Greeter.GreeterClient(channel);
}
[Test]
public async Task SayHello_GreetsWithName()
{
// Act
var reply = await client.SayHelloAsync(new HelloRequest { Name = "IntegrationTest" });
// Assert
Assert.IsTrue(reply.Message.Contains("IntegrationTest"));
}
[TearDown]
public void TearDown()
{
channel?.Dispose();
}
}
gRPC in .NET: Best practices for deploying gRPC services
Implement Health Checks:
ASP.NET Core supports health checks that can be used to assess the health of your gRPC service.
public void ConfigureServices(IServiceCollection services)
{
services.AddGrpc();
services.AddHealthChecks(); // Add health checks
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapGrpcService<MyGrpcService>();
endpoints.MapHealthChecks("/health"); // Map health checks endpoint
});
}
Monitoring and Logging Techniques
1. Application Insights for Monitoring:
Integrate Application Insights into your ASP.NET Core application for comprehensive monitoring. Add the Application Insights SDK to your project:
dotnet add package Microsoft.ApplicationInsights.AspNetCore
Configure Application Insights in Startup.cs
:
public void ConfigureServices(IServiceCollection services)
{
services.AddApplicationInsightsTelemetry();
// Other services
}
2. Logging with Serilog:
Serilog provides rich logging capabilities. Install Serilog and its ASP.NET Core integration:
dotnet add package Serilog.AspNetCore
Configure Serilog in Program.cs
:
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.UseSerilog((context, services, configuration) => configuration
.ReadFrom.Configuration(context.Configuration)
.ReadFrom.Services(services)
.Enrich.FromLogContext()
.WriteTo.Console())
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
Troubleshooting common Issues
1. Connectivity Issues:
Ensure your service is accessible and listening on the expected ports. Use logging to capture and examine any connectivity errors.
2. SSL/TLS Configuration:
SSL/TLS misconfigurations can prevent clients from establishing secure connections. Verify your certificates and ensure they are correctly configured in your application.
3. Performance Bottlenecks:
Identify performance bottlenecks by monitoring CPU, memory usage, and response times. Tools like Application Insights and Prometheus can be instrumental.
services.AddGrpc(options =>
{
options.EnableDetailedErrors = true; // Enable detailed errors for development
});
Note: Enable detailed errors only in development environments to avoid exposing sensitive information.
You can ensure the reliability and robustness of your gRPC services by following these deployment best practices, using sophisticated monitoring and logging techniques, and preparing for common problems. This comprehensive approach does not merely facilitate deployment, but also ensures that your services are healthy and functioning in the production environment.
Conclusion
Finally, navigating the intricacies of gRPC within the ASP.NET Core ecosystem reveals a powerful way to build high performance, scalable microservices. The journey will enrich your technical knowledge significantly, whether you are a newcomer to the gRPC world or an experienced developer who wants to improve their skills.
gRPC provides the tools necessary to increase your application’s performance, including creating an effective communication between microservices and understanding advanced features and best practice. To remain at the forefront of the .NET development landscape, you must seek the adventure of learning, experimenting, and integrating these technologies.
Are you looking for top-tier .NET development expertise?
Look no further than WireFuture, your premier .net development team. At WireFuture, we’re not just developers; we’re innovators and problem-solvers dedicated to pushing the boundaries of what’s possible with .NET and gRPC technologies. We have a great deal of experience in developing tailormade, scalable solutions that drive success and innovation.
Our experts are ready to bring your vision to life, whether you want to experiment with a new microservices architecture or optimize the current systems. Partner with WireFuture, and let’s build something extraordinary together.
Pointers to community resources
- gRPC.io Official Documentation: The official gRPC documentation (grpc.io) is a comprehensive resource covering everything from introductory concepts to advanced features.
- Stack Overflow: The Stack Overflow community is an excellent place to find answers to specific questions or challenges you might encounter.
- ASP.NET Core Documentation: Microsoft’s official documentation on ASP.NET Core (docs.microsoft.com) provides insights into integrating gRPC with ASP.NET Core applications.
At WireFuture, we believe every idea has the potential to disrupt markets. Join us, and let's create software that speaks volumes, engages users, and drives growth.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.