How to Implement Server Side Rendering In React
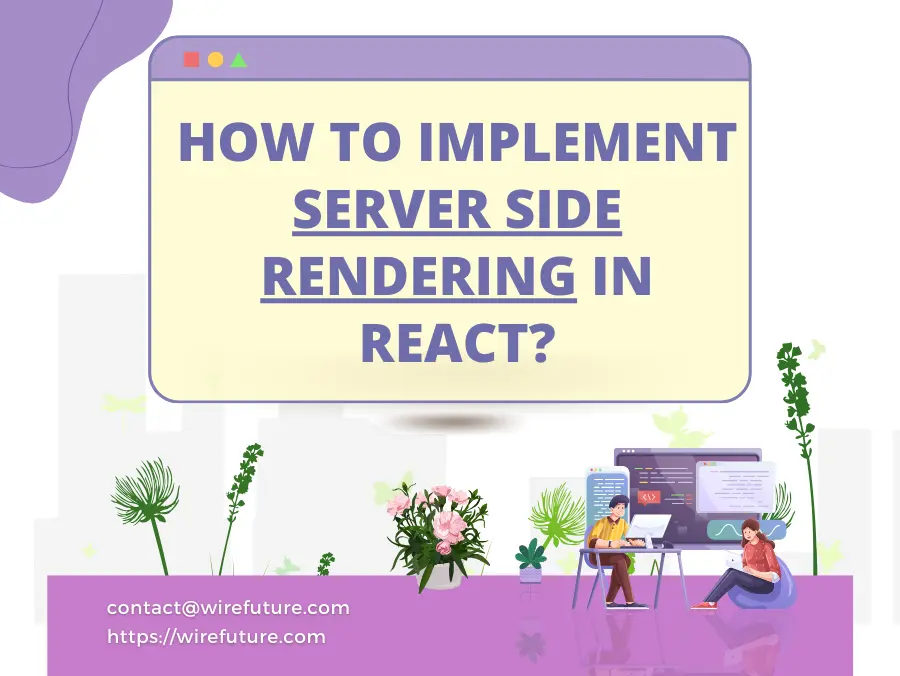
The strategic role of Server Side Rendering in React Applications is becoming increasingly important as web development evolves at a rapid pace. This approach focuses on the fundamental aspects of optimizing web performance, by improving loading times and enhancing search engine optimization (SEO). The discussion provides a detailed explanation of the benefits and substantial impact of SSR, showing their essential role in modern web applications.
Table of Contents
- The Necessity of Server Side Rendering for Modern Web Development
- Server Side Rendering In React: A Complete Example
- Wrapping It Up
The Necessity of Server Side Rendering for Modern Web Development
The primary advantage of SSR lies in its capability to render the initial page on the server, thereby sending a fully populated HTML page to the client. This significantly shortens the time it takes for the content to appear on the user’s screen, thus directly improving the perceived performance of the application. In an era where the immediacy of content delivery can dictate user retention, the strategic implementation of SSR can be the differentiating factor that keeps a user engaged rather than navigating away.
In addition, SSR’s contribution to Search Engine Optimization cannot be underestimated. The content that is easy to access and easily understood shall be the first priority in search engines. Through server-side rendering, React applications ensure that their content is immediately available for search engine crawlers. This direct availability is essential for improving the rank of a page and, moreover, leading to increased Organic Traffic. It’s not just an advantage to be seen on the results of search engines, it’s also a necessity for survival at a very competitive digital market.
In Conclusion, SSR is not only a technological decision to integrate with the web development practices but also a strategy for developing faster, easier and more easily available web applications. As the digital landscape becomes more competitive, SSR is becoming a key element in the development of web solutions that meet and exceed the expectations of today’s users and search engines.
Server Side Rendering In React: A Complete Example
Here’s how you set up an SSR-powered React app that sings to the tune of efficiency and speed.
First, you’ll need Express.js, a Node.js framework, to create your server. This server will pre-render your React components into HTML, ensuring they’re ready for their performance before they even reach the browser. Also, we have to understand a bit about ReactDOMServer so let’s get started.
👉 To explore what’s new in React 19, check out: React 19 Features and Migration Guide
What is Express and How to Install It?
For Node.js developers, Express.js is like a Swiss Army knife. This framework provides a strong set of features to develop Web and Mobile Applications. It facilitates the rapid development of server side logic and routes, making it a popular option for handling HTTP requests.
To get Express set up in your project, you’ll need Node.js installed on your machine. Once you have Node, installing Express is as simple as running a command in your terminal. Open your command line tool and enter:
npm install express
This command fetches the Express package from npm (Node Package Manager) and adds it to your project, ready for use.
What is ReactDOMServer?
The ReactDOMServer is a component of the React library, which can be sent to your client’s browser on an initial request for components that are rendered in HTML. This is important for SSR because it enables your React app to be seen by search engines and users before any JavaScript has been loaded or interpreted on the client side.
This server-side rendering capability is essential for SEO and improving the load time of your pages. You are ensuring that HTML is fully developed when it comes to the client, which makes your app more accessible and efficient if you render React components on a server.
Implementing SSR with Express and ReactDOMServer
Now, let’s expand on how to set everything up with a bit more context:
1. Initializing Your Project:
Before installing Express, you’ll want to initialize a new Node.js project if you haven’t already. In your project directory, run:
npm init -y
This command creates a package.json
file in your project, which will track your dependencies and scripts.
2. Installing Express:
With your project initialized, install Express using npm:
npm install express
3. Setting Up Your Server File:
We need to create a simple server using Express that serves our React application. Here is the example to do that:
// server.js
import express from 'express';
import React from 'react';
import ReactDOMServer from 'react-dom/server';
import App from './src/App';
const PORT = process.env.PORT || 3000;
const server = express();
server.use(express.static('build'));
server.get('/*', (req, res) => {
const app = ReactDOMServer.renderToString(<App />);
const html = `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>React App with SSR</title>
</head>
<body>
<div id="root">${app}</div>
<script src="/bundle.js"></script>
</body>
</html>
`;
res.send(html);
});
server.listen(PORT, () => {
console.log(`Server is listening on port ${PORT}`);
});
Let’s break down the key parts:
- Importing Dependencies: We import express to handle HTTP requests, React for our components, and ReactDOMServer to render our components to HTML on the server.
- Creating an Express Server: We initialize an Express application using express().
- Serving Static Files: server.use(express.static(‘build’)) tells Express to serve static files (like your JavaScript bundle) from the build directory.
- Server-Side Rendering Route: The server has a catch-all route that uses ReactDOMServer.renderToString(<App />) to convert your React app into a string of HTML. This HTML is then sent back to the client inside a basic HTML document structure.
To tie it all together, ensure your Webpack configuration bundles your server-side code correctly. You’ll want to output a server bundle (e.g., server.js
) that Node can run to serve your SSR’d app.
// webpack.server.js
const path = require('path');
const nodeExternals = require('webpack-node-externals');
module.exports = {
entry: './server.js',
target: 'node',
externals: [nodeExternals()],
output: {
path: path.resolve('server-build'),
filename: 'server.js'
},
module: {
rules: [
{
test: /\.js$/,
use: 'babel-loader'
}
]
}
};
4. Running Your Server:
To run your server, you’ll need to add a start script to your package.json
or run the server file directly with Node. For example:
"scripts": {
"start": "node server.js"
}
Then, run your server with:
npm start
This command starts your Express server, which listens for requests and serves your SSR React application.
Wrapping It Up
In wrapping up our exploration of Server Side Rendering (SSR) in React applications, it’s clear that SSR is more than just a technical strategy; it’s a comprehensive approach to optimizing web performance, improving SEO, and ensuring a superior user experience.
The strategic implementation of SSR in React applications represents a significant step forward in developing web applications that are not only fast and accessible but also rank well on search engine results pages. This is testimony to the evolving nature of web development, where performance and user satisfaction are most important.
Are you looking to hire React developers? This is where WireFuture positions itself as an important partner. Specializing in .Net / PHP / Python based applications and with a keen focus on cutting-edge web technologies like React, WireFuture offers expertise in crafting SSR-enabled React applications that stand out for their speed, efficiency, and accessibility.
Choosing WireFuture means working with a team of experienced React developers who bring a wealth of experience and a proven track record of success in modern web development. WireFuture’s developers are equipped to provide solutions that meet your specific needs and exceed expectations, whether you want to build a new project from scratch or optimize an existing application with SSR.
Step into the future with WireFuture at your side. Our developers harness the latest technologies to deliver solutions that are agile, robust, and ready to make an impact in the digital world.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.