How To Integrate Azure OpenID in ASP.NET Core
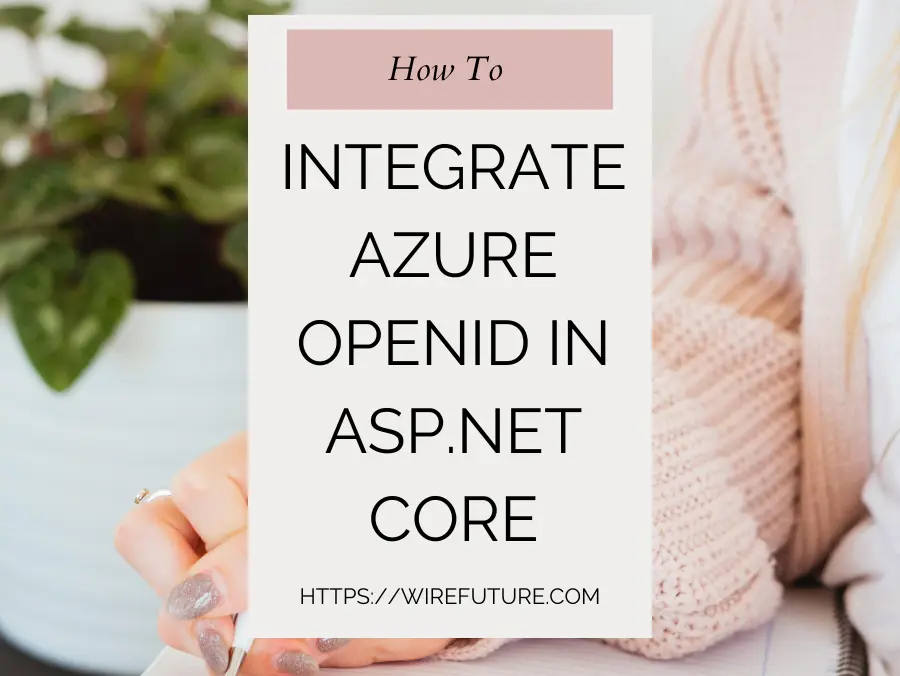
In this blog post we will try to understand how to integrate Azure OpenID in ASP.NET Core. When building secure ASP.NET Core apps, Azure OpenID Connect (OIDC) is a foundation for strong authentication. Azure OIDC is more than an enhancement; it’s a solution. it’s an identity verification powered user authentication solution that leverages OAuth 2.0’s strengths. This ensures your application knows who is accessing it – not just whether they are authorised.
What’s OpenID Connect (OIDC) and OAuth 2.0?
OIDC is user authentication – focused extension of OAuth 2.0. Whereas OAuth 2.0 is all about authorization, OIDC adds an identity layer that enables applications to know the ‘who’ behind the access request. Integrating Azure OpenID with ASP.NET Core allows your application to use this advanced authentication method for user authentication while using Microsoft’s Azure Active Directory (AD) for user identity management.
Concepts of Integration
Tenants: These are your instance in Azure AD for your organization. Using Azure OpenID with ASP.NET Core, you set up authentication for your app against a tenant.
Applications: ASP.NET Core app must be registered as an application in Azure AD. This registration is critical for integrating Azure OpenID, as it defines how your app authenticates with the protocol.
Tokens: Tokens issued by OIDC are central to the integration process:
- ID Tokens store user profile information that is used for authentication in your ASP.NET Core app.
- Access Tokens let your application make authenticated API calls on the user’s behalf.
The Advantages of Integration
The benefits of integrating Azure OpenID Connect in your ASP.NET Core application are numerous:
- Streamlined Authentication: By using Azure OIDC, you can leverage Microsoft’s best security practices for authentication.
- Enhanced Security: The integration makes sure your authentication method is based on secure, industry-standard protocols.
- Scalability: Azure OIDC is built for scalable authentication that scales with your application user base.
- User Management: Azure AD provides tools to manage users and accesses for an easier user experience in your application.
- More Advanced Security Features: Azure AD gives you access to features including multi-factor authentication and conditional access to improve your app security.
Table of Contents
- Integrate Azure OpenID in ASP.NET Core
- Securely Managing User Sessions
- Troubleshooting Common Issues
- Integrate Azure OpenID In ASP.NET Core: Conclusion
- Additional Resources
- Frequently Asked Questions (FAQ)
Integrate Azure OpenID in ASP.NET Core
This process involves a few key steps: installing necessary packages, tweaking your Startup.cs
to use Azure OpenID Connect, and setting up the middleware for handling authentication processes including sign-in and sign-out. Here’s how you can do it, complete with code samples to guide you through.
Step 1: Installing Necessary NuGet Packages
To get started, you need to add the appropriate NuGet packages to your ASP.NET Core project. The primary package for integrating Azure OpenID Connect is Microsoft.AspNetCore.Authentication.OpenIdConnect
. You can install this package using the .NET CLI:
dotnet add package Microsoft.AspNetCore.Authentication.OpenIdConnect
dotnet add package Microsoft.Identity.Web
These commands add the OpenID Connect middleware and Microsoft Identity Web to your project, which simplifies the integration with Azure AD.
Step 2: Configuring the Startup.cs
In your Startup.cs
, you’ll need to configure services and the app’s middleware to use Azure OpenID Connect. This involves setting up the authentication services in the ConfigureServices
method and then ensuring the app uses authentication middleware in the Configure
method.
ConfigureServices Method:
Here’s how you can configure the services for Azure OpenID Connect:
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication(options =>
{
options.DefaultScheme = CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = OpenIdConnectDefaults.AuthenticationScheme;
})
.AddCookie()
.AddOpenIdConnect(options =>
{
options.Authority = "https://login.microsoftonline.com/{TenantID}";
options.ClientId = "{ClientID}";
options.ClientSecret = "{ClientSecret}"; // Required for web apps
options.ResponseType = "code";
options.SaveTokens = true;
options.Scope.Add("openid");
options.Scope.Add("profile");
// Add additional scopes as needed
});
services.AddControllersWithViews();
}
Replace {TenantID}
, {ClientID}
, and {ClientSecret}
with your Azure AD tenant ID, client ID, and client secret, respectively.
To create a Tenant ID, Client ID and a secret for your Azure AD application, log into the Azure portal. Open Azure Active Directory and click “App registrations” to create a new application registration. Once completed, Azure gives you a unique Application (Client) ID. For the Tenant ID, check the Azure Active Directory overview page, and look for Directory (Tenant) ID. Lastly, click your application, go to “Certificates and secrets” and click “New client secret” Write a description and expiration for the secret, then save; be sure to copy the value after saving; it can not be retrieved once you leave the page.
Configure Method:
Ensure your application uses authentication by adding it to the middleware pipeline in the Configure
method:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// Other configurations like error handling, static files, etc.
app.UseRouting();
// Use authentication and authorization middleware
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
Step 3: Setting Up the Middleware for Authentication
With the above setup, your application is now configured to use Azure OpenID Connect for authentication. When a user attempts to access a protected resource, they will be redirected to Azure AD for sign-in. Upon successful sign-in, Azure AD redirects back to your application, where the OpenID Connect middleware processes the sign-in response, creates a session for the user, and redirects them to their originally requested resource.
Handling Sign-In and Sign-Out:
To facilitate user sign-in and sign-out in your application, you can add actions in a controller, like so:
public class AccountController : Controller
{
public IActionResult SignIn()
{
var redirectUrl = Url.Action(nameof(HomeController.Index), "Home");
return Challenge(
new AuthenticationProperties { RedirectUri = redirectUrl },
OpenIdConnectDefaults.AuthenticationScheme);
}
public async Task<IActionResult> SignOut()
{
var callbackUrl = Url.Action(nameof(SignedOut), "Account", values: null, protocol: Request.Scheme);
return SignOut(
new AuthenticationProperties { RedirectUri = callbackUrl },
CookieAuthenticationDefaults.AuthenticationScheme,
OpenIdConnectDefaults.AuthenticationScheme);
}
public IActionResult SignedOut()
{
// Handle post-sign-out flow here (e.g., display a "You've been signed out" page)
return RedirectToAction(nameof(HomeController.Index), "Home");
}
}
This setup handles the basic flow of signing users in and out of your application using Azure OpenID Connect. Each part of this process is customizable, allowing you to tailor the authentication experience to meet your application’s needs.
Accessing tokens within a controller action
public async Task<IActionResult> Index()
{
// Access tokens stored in the authentication cookie
var accessToken = await HttpContext.GetTokenAsync("access_token");
var idToken = await HttpContext.GetTokenAsync("id_token");
// Use the access token to call a protected resource...
return View();
}
Validating tokens manually is typically not required for general use cases, as the middleware handles this based on the configuration you provide. However, if you need to validate tokens for specific reasons, you can use the System.IdentityModel.Tokens.Jwt
library to inspect and manually validate tokens.
Securely Managing User Sessions
After authentication, managing user sessions securely involves several key considerations. This is where top-tier ASP.NET development company shine, delivering cutting-edge solutions and unparalleled expertise for your project needs.:
- Cookie Security: Use secure cookies that are HTTP-only and configure them to be sent only over HTTPS connections. This helps prevent session hijacking and man-in-the-middle attacks.
- Session Expiration: Configure a reasonable expiration time for sessions to reduce the risk of session fixation attacks. Use sliding expiration to keep users active as long as they’re interacting with the application.
- Validate Sessions: Regularly validate the session against the user’s authentication state. This can be handled automatically by the middleware but consider your application’s specific needs for re-validation.
Best Practices for Session Security and Token Storage
- Secure Session Storage: If you need to store session data on the server, ensure it’s secured and encrypted. For storing tokens and sensitive data, consider secure mechanisms like ASP.NET Core’s Data Protection API.
- Token Handling: When storing tokens (like the ID token or access token), ensure they’re stored securely. Avoid storing sensitive tokens in local storage or cookies accessible via JavaScript.
- Use HTTPS: Always use HTTPS to encrypt the data transmitted between the client and server. This protects the authentication tokens and cookies from being intercepted.
- Regularly Update Authentication Libraries: Keep your authentication libraries up to date to mitigate known vulnerabilities.
- Monitor Session Activity: Implement monitoring to detect unusual session activity, which could indicate session hijacking or token theft.
services.ConfigureApplicationCookie(options =>
{
options.Cookie.HttpOnly = true;
options.Events.OnRedirectToLogin = context =>
{
// Redirect handling for unauthorized access...
};
});
Troubleshooting Common Issues
Troubleshooting and resolving common integration issues with Azure OpenID and ASP.NET Core can save you a lot of headaches. From debugging challenges to ensuring you have proper logging and monitoring for authentication-related activities, let’s dive into some effective strategies and code samples to keep your application running smoothly.
Debugging Common Integration Issues
Issue 1: Users Can’t Sign In
- Cause: Incorrect setup in Azure AD or misconfiguration in
Startup.cs
. - Resolution: Verify that the Client ID, Tenant ID, and Redirect URIs in your Azure AD app registration match exactly with those in your
Startup.cs
configuration. Also, ensure that you’ve correctly configured the application as either single-tenant or multi-tenant in both the Azure portal and your code.
services.AddAuthentication(OpenIdConnectDefaults.AuthenticationScheme)
.AddOpenIdConnect(options =>
{
options.ClientId = "Your-Client-Id";
options.Authority = "https://login.microsoftonline.com/Your-Tenant-Id";
options.CallbackPath = "/signin-oidc"; // Ensure this matches the redirect URI in Azure AD
});
Issue 2: Access Denied or Insufficient Permissions
- Cause: The application is not requesting the correct scopes or roles.
- Resolution: Ensure that your application requests appropriate scopes during authentication and that your Azure AD app has necessary permissions granted.
services.AddAuthentication().AddOpenIdConnect(options =>
{
// Other options...
options.Scope.Add("openid");
options.Scope.Add("profile");
options.Scope.Add("email");
// Add additional scopes as needed
});
Tips for Logging and Monitoring Authentication-Related Activities
Effective logging and monitoring are crucial for diagnosing and resolving issues quickly. ASP.NET Core and Azure provide tools to help with this.
Enable Detailed Logging in ASP.NET Core
Configure logging in appsettings.json
to include detailed information for troubleshooting:
"Logging": {
"LogLevel": {
"Default": "Warning",
"Microsoft": "Information",
"Microsoft.Hosting.Lifetime": "Information",
"Microsoft.AspNetCore.Authentication": "Debug"
}
}
This setting will provide detailed logs from the authentication process, helping you spot where things might be going wrong.
Using Application Insights for Monitoring
Azure Application Insights is a powerful tool for monitoring your web applications. To use it for monitoring authentication-related activities:
Add Application Insights to your project:
dotnet add package Microsoft.ApplicationInsights.AspNetCore
Configure Application Insights in Startup.cs
:
public void ConfigureServices(IServiceCollection services)
{
// Add application insights
services.AddApplicationInsightsTelemetry();
// Other service configurations...
}
Custom Logging:
You can also add custom logging around authentication events in Startup.cs
to track specific issues:
services.AddAuthentication(OpenIdConnectDefaults.AuthenticationScheme)
.AddOpenIdConnect(options =>
{
// Configure options...
options.Events = new OpenIdConnectEvents
{
OnTokenValidated = context =>
{
// Log success
context.HttpContext.RequestServices.GetRequiredService<ILogger<Startup>>()
.LogInformation("Token validated for {UserName}", context.Principal.Identity.Name);
return Task.CompletedTask;
},
OnRemoteFailure = context =>
{
// Log failure
context.HttpContext.RequestServices.GetRequiredService<ILogger<Startup>>()
.LogError(context.Failure, "Authentication failed.");
return Task.CompletedTask;
}
};
});
By applying these troubleshooting techniques and monitoring strategies, you can more effectively identify and resolve common integration issues with Azure OpenID and ASP.NET Core, ensuring a smoother authentication experience for your users.
Integrate Azure OpenID In ASP.NET Core: Conclusion
Integrating Azure OpenID Connect with ASP.NET Core ensures greater protection for your apps while simultaneously enhancing the user experience. With Azure AD for authentication, you can deliver single sign-on (SSO) experiences to users while lowering login friction and increasing security with multi factor authentication (MFA) and conditional access policies. This integration helps you protect your users’ information and your resources from attack.
WireFuture knows how complex and challenging it is to integrate Azure OpenID in ASP.NET Core. Our team of ASP.NET Core specialists & Azure professionals can enable you to navigate these obstacles and unleash the potential of your apps. When you hire ASP.NET Core developers and Azure experts from WireFuture, you get more than technical skills: you get people with experience. you’re joining a team that’s driven by innovation, excellence & solutions for business success. Looking to enhance your web applications with secure authentication or explore new possibilities with Azure? WireFuture is here to bring your vision to life. Let’s create something amazing together.
Additional Resources
Official Documentation and Tutorials
- Microsoft Docs: Start with the ASP.NET Core Documentation for comprehensive guides on ASP.NET Core fundamentals, security, and advanced scenarios. For Azure AD and OpenID Connect specifics, explore the Azure Active Directory documentation.
- Microsoft Learn: Engage with interactive learning paths on Microsoft Learn, such as Integrate your app with an Azure Active Directory for hands-on experience with Azure AD.
Community Forums and Platforms
- Stack Overflow: A treasure trove of Q&A, Stack Overflow hosts a vibrant community for ASP.NET Core and Azure Active Directory. It’s a great place to seek advice, share knowledge, and troubleshoot issues.
- GitHub Issues: For specific challenges or bugs, the ASP.NET Core GitHub Issues page is an invaluable resource. You can report issues, contribute to discussions, and track the resolution of problems.
Frequently Asked Questions (FAQ)
Azure OpenID Connect (OIDC) is a authentication protocol that extends OAuth 2.0, providing an additional identity layer that allows applications to verify a user’s identity based on authentication performed by an authorization server, in this case, Azure Active Directory (Azure AD).
Integrating Azure OpenID Connect with ASP.NET Core allows developers to leverage Microsoft’s secure and scalable identity service, providing users with a seamless authentication experience, supporting single sign-on (SSO), and simplifying the management of user identities.
You can register your application in the Azure portal by navigating to Azure Active Directory > App registrations > New registration. Here, you’ll provide your application’s name, supported account types, and the redirect URI that Azure AD will use to return responses to your application.
The main NuGet package required is Microsoft.AspNetCore.Authentication.OpenIdConnect
. You might also need Microsoft.Identity.Web
for more advanced scenarios and integrations.
You configure your application in the Startup.cs
file, specifically in the ConfigureServices
method, where you’ll set up the authentication services with .AddAuthentication()
and .AddOpenIdConnect()
, providing necessary options such as the Client ID, Tenant ID, and Callback Path.
User sessions are managed through cookie authentication, configured alongside OpenID Connect in the Startup.cs
. This involves issuing a cookie upon successful authentication, which is then used to maintain the user’s session.
Best practices include using HTTPS for all communications, setting secure and HTTPOnly flags on cookies, implementing appropriate session expiration, and using Azure AD’s built-in security features like multi-factor authentication (MFA).
Common troubleshooting steps include ensuring correct application registration settings in Azure AD, verifying matching redirect URIs, and enabling detailed logging in ASP.NET Core to investigate authentication flow issues.
Imagine a team that sees beyond code—a team like WireFuture. We blend art and technology to develop software that is as beautiful as it is functional. Let's redefine what software can do for you.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.