How To Integrate Stripe in ASP.NET Core?
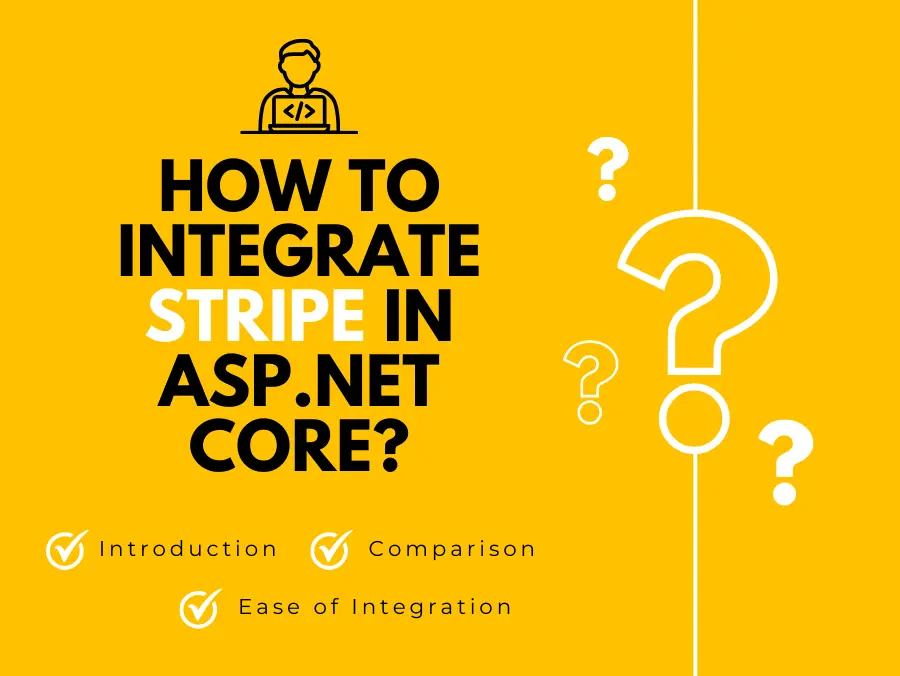
This blog post is your ultimate walkthrough on how to integrate Stripe in ASP.NET Core, making your payment processes smoother and more secure than ever. Stripe has become popular with both developers and businesses, distinguishing itself from other payment gateways by its surprisingly developer friendly approach and robust features set. One of the key reasons for its widespread adoption is the ease of integration. Stripe offers comprehensive documentation, client libraries in various programming languages, and a developer-first API design that makes it straightforward to embed into applications. This ease of use is not only saving time; it enables developers at any skill level to implement complex payment solutions with confidence.
In addition, Stripe’s commitment to remain at the forefront of technology trends means that it supports a broad range of payment methods, e.g. credit cards, bank transfers and more recent digital wallet for an international audience. Its attractiveness is enhanced by its transparent pricing model and reliable customer support, which makes it a go to solution for startups and established enterprises that are trying to scale up without losing user experience.
It’s an invaluable skill for developers to learn how to integrate Stripe, enabling them to open up a range of projects that require payment processing. Understanding Stripe’s API can have a significant impact on project success, regardless of whether it is for online stores, subscription services or marketplace. This knowledge not only adds value to the developer’s toolkit, but it also represents an important asset for any team that aims at providing a seamless payment experience in their application.
So today, we’re going to learn on how to seamlessly integrate Stripe into an ASP.NET Core application. We’ll walk you through everything from installing the necessary packages to configuring your application, and coding the integration. You will have a fully functional ASP.NET Core application that can process payments, ensuring a smooth and secure checkout experience for your users, by the end of this session.
Table of Contents
- Prerequisites
- Step 1: Create Your ASP.NET Core Project to integrate Stripe in ASP.NET Core
- Step 2: Install the Stripe NuGet Package
- Step 3: Configure Stripe in Program.cs to integrate Stripe in ASP.NET Core
- Step 4: Create a Payment Service
- Step 5: Inject and Use Your Payment Service to integrate Stripe in ASP.NET Core
- Step 6: Configure Entity Framework Core
- FAQ on Integrating Stripe with ASP.NET Core
- Final Thoughts
Prerequisites
Before we start, make sure you’ve got the following ready:
- .NET 7.0 SDK or later installed on your machine.
- A Stripe account (it’s free to sign up, and you’ll need it to access your API keys).
- Visual Studio 2022 as your IDE.
Step 1: Create Your ASP.NET Core Project to integrate Stripe in ASP.NET Core
First things first, create a new ASP.NET Core Web Application. Open your terminal or command prompt and run:
dotnet new webapi -n StripeIntegrationDemo
This command scaffolds a new web API project named StripeIntegrationDemo
.
Step 2: Install the Stripe NuGet Package
To interact with Stripe, we need the Stripe .NET library. Install it via NuGet Package Manager or the CLI with:
dotnet add package Stripe.net
This command fetches and installs the Stripe.NET library, which provides a rich set of APIs to communicate with Stripe’s services.
Step 3: Configure Stripe in Program.cs to integrate Stripe in ASP.NET Core
Now, let’s wire up Stripe in our Program.cs
file. You’ll need your Stripe secret key, which you can find in your Stripe Dashboard under Developers > API keys.
Open Program.cs
and add the following code before building the app:
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
// Configure Stripe
builder.Services.Configure<StripeSettings>(builder.Configuration.GetSection("Stripe"));
Stripe.StripeConfiguration.ApiKey = builder.Configuration["Stripe:SecretKey"];
builder.Services.AddSingleton<StripePaymentService>();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
Create a new file StripeSettings.cs
in your project with:
public class StripeSettings
{
public string SecretKey { get; set; }
}
And, add your Stripe settings to appsettings.json
:
"Stripe": {
"SecretKey": "your_stripe_secret_key_here"
}
Step 4: Create a Payment Service
Let’s encapsulate Stripe payment functionalities within a service. Create a new folder named Services
and add a class StripePaymentService.cs
with the following content:
using Stripe;
using Stripe.Checkout;
using Microsoft.Extensions.Options;
public class StripePaymentService
{
public StripePaymentService(IOptions<StripeSettings> stripeSettings)
{
StripeConfiguration.ApiKey = stripeSettings.Value.SecretKey;
}
public Session CreateCheckoutSession(string successUrl, string cancelUrl)
{
var options = new SessionCreateOptions
{
PaymentMethodTypes = new List<string>
{
"card",
},
LineItems = new List<SessionLineItemOptions>
{
new SessionLineItemOptions
{
PriceData = new SessionLineItemPriceDataOptions
{
UnitAmount = 2000, // For example, $20.00 (this value is in cents)
Currency = "usd",
ProductData = new SessionLineItemPriceDataProductDataOptions
{
Name = "T-shirt",
},
},
Quantity = 1,
},
},
Mode = "payment",
SuccessUrl = successUrl,
CancelUrl = cancelUrl,
};
var service = new SessionService();
Session session = service.Create(options);
return session;
}
}
This service includes a method CreateCheckoutSession
to create a Stripe Checkout session with a sample product.
Step 5: Inject and Use Your Payment Service to integrate Stripe in ASP.NET Core
Now, inject your StripePaymentService
into the DI container in Program.cs
:
builder.Services.AddScoped<StripePaymentService>();
Create a new controller PaymentController.cs
in your Controllers
folder to utilize this service:
public class PaymentController : ControllerBase
{
private readonly StripePaymentService _paymentService;
private readonly ApplicationDbContext _context;
public PaymentController(StripePaymentService paymentService, ApplicationDbContext context)
{
_paymentService = paymentService;
_context = context;
}
[HttpPost("create-checkout-session")]
public ActionResult CreateCheckoutSession()
{
var session = _paymentService.CreateCheckoutSession(
"https://yourdomain.com/payment/success?sessionId={CHECKOUT_SESSION_ID}",
"https://yourdomain.com/payment/cancel?sessionId={CHECKOUT_SESSION_ID}"
);
// Record the session in your DB
_context.PaymentRecords.Add(new PaymentRecord
{
StripeSessionId = session.Id,
Created = DateTime.UtcNow,
Status = "Created"
});
_context.SaveChanges();
return Ok(new { sessionId = session.Id });
}
[HttpGet("success")]
public async Task<IActionResult> Success(string sessionId)
{
var paymentRecord = await _context.PaymentRecords.FirstOrDefaultAsync(p => p.StripeSessionId == sessionId);
if (paymentRecord != null)
{
paymentRecord.Status = "Success";
await _context.SaveChangesAsync();
}
// Redirect to a success page or return success response
return Ok("Payment successful.");
}
[HttpGet("cancel")]
public async Task<IActionResult> Cancel(string sessionId)
{
var paymentRecord = await _context.PaymentRecords.FirstOrDefaultAsync(p => p.StripeSessionId == sessionId);
if (paymentRecord != null)
{
paymentRecord.Status = "Cancelled";
await _context.SaveChangesAsync();
}
// Redirect to a cancel page or return cancel response
return Ok("Payment cancelled.");
}
}
Define your PaymentRecord
entity:
public class PaymentRecord
{
public int Id { get; set; }
public string StripeSessionId { get; set; }
public DateTime Created { get; set; }
public string Status { get; set; }
}
Step 6: Configure Entity Framework Core
Ensure you have Entity Framework Core installed and configured. If you haven’t already, add the package:
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
dotnet add package Microsoft.EntityFrameworkCore.Design
Configure your DbContext
in a new file ApplicationDbContext.cs
:
using Microsoft.EntityFrameworkCore;
public class ApplicationDbContext : DbContext
{
public ApplicationDbContext(DbContextOptions<ApplicationDbContext> options) : base(options) {}
public DbSet<PaymentRecord> PaymentRecords { get; set; }
}
Then, add the DbContext
to your services in Program.cs
:
builder.Services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(builder.Configuration.GetConnectionString("DefaultConnection")));
Don’t forget to add your connection string to appsettings.json
:
"ConnectionStrings": {
"DefaultConnection": "Your_SQL_Server_Connection_String"
}
FAQ on Integrating Stripe with ASP.NET Core
Before diving into the integration, make sure you’ve got the basics covered: an ASP.NET Core project set up, a Stripe account (you’ll need those API keys), and the Stripe.NET library installed in your project.
First, store your API keys securely using ASP.NET Core’s configuration system, preferably in appsettings.json or through environment variables. Then, access these keys in your application and initialize the Stripe client with them.
Always use HTTPS to protect data in transit, validate webhook signatures for authenticity, and never, ever log sensitive information like API keys or card details. Storing sensitive data should be left to Stripe; your focus is on making secure API calls and handling responses.
Stripe provides a plethora of test API keys and card numbers that simulate different scenarios (successful payments, declines, etc.). Use these in your development environment to thoroughly test your integration before going live.
Patience and thorough testing are your allies. Payment processing is critical and sensitive, so take your time to understand the flow, handle errors gracefully, and ensure your integration is as secure as possible. And remember, the Stripe and ASP.NET Core communities are incredibly supportive. Don’t hesitate to reach out for help or share your own insights once you’ve mastered the integration. It’s all about learning and growing together in the tech world.
Final Thoughts
These changes now allow your application to process Stripe payments and record results in a database using the Entity Framework Core. This configuration provides a solid basis for developing more complex payment flows, handling webhooks for asynchronous payment events, and integrating additional Stripe features.
Do you need the best development of Stripe ASP.NET Core? Are you looking for a .Net development company that truly stands out? Look no further than WireFuture! We are not just a team; we are a team of passionate, code crunching experts who thrive on turning complex problems into sleek, efficient solutions. We specialize in creating robust, scalable applications that drive business success in ASP.NET Core.
Hire dedicated .NET developers who blend in cutting-edge technology with a touch of creativity, ensuring your project isn’t just completed; it’s transformed into a masterpiece. We are the partners you need to bring your vision to life, whether it is a new start up or an existing firm focused on innovation. With WireFuture, you can explore the future of .Net development, where your goals are in line with our expertise. Together, let’s create something extraordinary!
From initial concept to final deployment, WireFuture is your partner in software development. Our holistic approach ensures your project not only launches successfully but also thrives in the competitive digital ecosystem.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.