How to Run Dynamic SQL Using sp_executesql in SQL Server
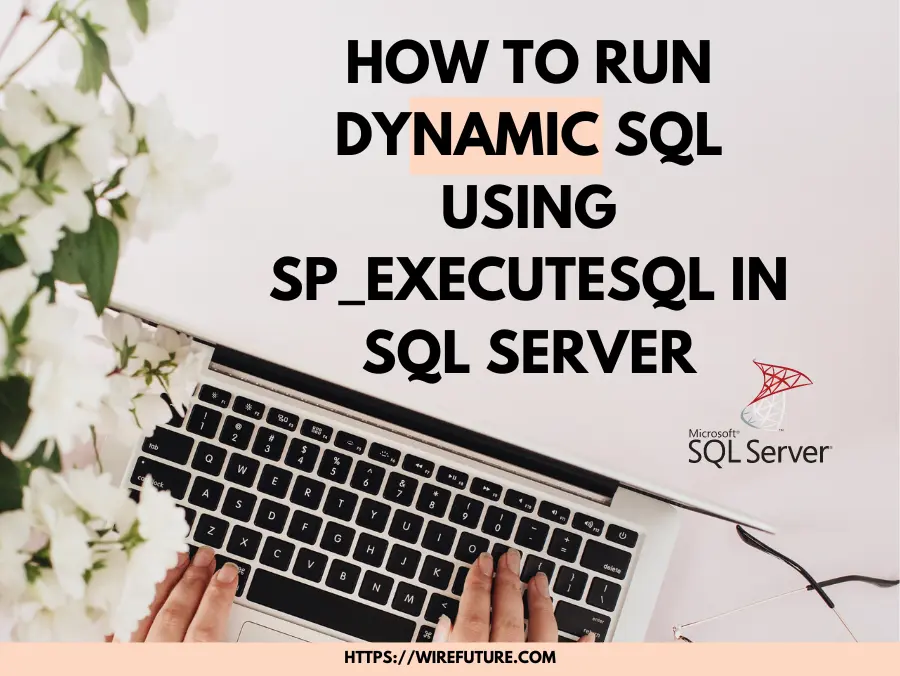
Dynamic SQL is a powerful tool in SQL Server that allows you to build SQL queries dynamically at runtime. This can be particularly useful in situations where the structure of a query depends on variable parameters. In this article, we’ll delve into the use of dynamic SQL using sp_executesql
, exploring its benefits, and demonstrating its usage with a complex example.
Table of Contents
- Introduction to Dynamic SQL
- Why Use sp_executesql For Dynamic SQL?
- Basic Usage of sp_executesql
- Complex Example with sp_executesql
- Conclusion
Introduction to Dynamic SQL
Dynamic SQL refers to SQL statements that are constructed and executed at runtime rather than being hardcoded in the application. This approach offers flexibility in executing queries with varying conditions, columns, and tables based on user input or other runtime variables.
There are two primary methods to execute dynamic SQL in SQL Server:
- Using the
EXEC
command. - Using the
sp_executesql
system stored procedure.
While both methods can execute dynamic SQL, sp_executesql
is generally preferred due to its ability to accept parameters, which helps in preventing SQL injection attacks and improving performance through parameterization and query plan reuse.
Why Use sp_executesql
For Dynamic SQL?
There are numerous situations where using sp_executesql
becomes essential, particularly in dynamic and data-driven applications. Consider a practical example of an e-commerce website. Such a site often needs to display products based on various user-selected filters like category, price range, brand, and rating. Hardcoding each possible query combination would be impractical due to the vast number of possible filter combinations. Instead, sp_executesql
allows for the dynamic construction of SQL queries that adapt to the user’s filter selections in real-time.
If you are developing an e-commerce site or any data-driven application, collaborating with an ASP.NET development company can further enhance your project’s success. They can integrate sp_executesql
effectively, ensuring your application handles dynamic queries efficiently and securely, providing a seamless user experience.
For instance, if a user filters products by a price range of $50 to $150, selects a specific brand, and sorts by highest rating, sp_executesql
can dynamically build a query that incorporates these conditions efficiently. This approach ensures that the query is both optimized for performance and secure from SQL injection attacks, providing a seamless and responsive user experience.
sp_executesql
also offers several advantages over the EXEC
command:
- Parameterization: Allows the use of parameters, which helps in preventing SQL injection.
- Plan Reuse: Enables SQL Server to reuse execution plans for queries with different parameters, improving performance.
- Flexibility: Can handle more complex and dynamic queries with varying parameters and conditions.
Basic Usage of sp_executesql
Before diving into a complex example, let’s start with a basic usage of sp_executesql
.
DECLARE @SQL NVARCHAR(MAX);
DECLARE @ParameterDefinition NVARCHAR(MAX);
DECLARE @EmployeeID INT = 1;
SET @SQL = N'SELECT FirstName, LastName FROM Employees WHERE EmployeeID = @EmpID';
SET @ParameterDefinition = N'@EmpID INT';
EXEC sp_executesql @SQL, @ParameterDefinition, @EmpID = @EmployeeID;
In this example:
- We declare a variable
@SQL
to hold the SQL query. - We define a parameter definition string
@ParameterDefinition
to specify the parameters used in the query. - We set the value of
@EmployeeID
and assign the dynamic SQL query to@SQL
. - Finally, we execute the dynamic SQL using
sp_executesql
, passing the query, parameter definition, and parameter values.
Complex Example with sp_executesql
Now, let’s explore a more complex scenario. Suppose we have a sales database with the following schema:
Sales
table with columns:SaleID
,SaleDate
,CustomerID
,TotalAmount
.Customers
table with columns:CustomerID
,FirstName
,LastName
,City
.
We want to build a dynamic SQL query that allows filtering sales data based on optional parameters such as date range, customer city, and minimum total amount.
Step-by-Step Solution
Declare Variables: We’ll declare variables to hold the dynamic SQL, parameter definitions, and input parameters.
DECLARE @SQL NVARCHAR(MAX);
DECLARE @ParameterDefinition NVARCHAR(MAX);
DECLARE @StartDate DATE = '2023-01-01';
DECLARE @EndDate DATE = '2023-12-31';
DECLARE @City NVARCHAR(50) = 'New York';
DECLARE @MinTotalAmount DECIMAL(10, 2) = 100.00;
Construct Dynamic SQL: We’ll construct the base query and append conditions based on the input parameters.
SET @SQL = N'
SELECT s.SaleID, s.SaleDate, c.FirstName, c.LastName, s.TotalAmount
FROM Sales s
JOIN Customers c ON s.CustomerID = c.CustomerID
WHERE 1 = 1';
IF @StartDate IS NOT NULL
SET @SQL = @SQL + ' AND s.SaleDate >= @StartDate';
IF @EndDate IS NOT NULL
SET @SQL = @SQL + ' AND s.SaleDate <= @EndDate';
IF @City IS NOT NULL
SET @SQL = @SQL + ' AND c.City = @City';
IF @MinTotalAmount IS NOT NULL
SET @SQL = @SQL + ' AND s.TotalAmount >= @MinTotalAmount';
Define Parameters: We’ll define the parameter definition string.
SET @ParameterDefinition = N'
@StartDate DATE,
@EndDate DATE,
@City NVARCHAR(50),
@MinTotalAmount DECIMAL(10, 2)';
Complete Script
Putting it all together, here’s the complete script:
DECLARE @SQL NVARCHAR(MAX);
DECLARE @ParameterDefinition NVARCHAR(MAX);
DECLARE @StartDate DATE = '2023-01-01';
DECLARE @EndDate DATE = '2023-12-31';
DECLARE @City NVARCHAR(50) = 'New York';
DECLARE @MinTotalAmount DECIMAL(10, 2) = 100.00;
SET @SQL = N'
SELECT s.SaleID, s.SaleDate, c.FirstName, c.LastName, s.TotalAmount
FROM Sales s
JOIN Customers c ON s.CustomerID = c.CustomerID
WHERE 1 = 1';
IF @StartDate IS NOT NULL
SET @SQL = @SQL + ' AND s.SaleDate >= @StartDate';
IF @EndDate IS NOT NULL
SET @SQL = @SQL + ' AND s.SaleDate <= @EndDate';
IF @City IS NOT NULL
SET @SQL = @SQL + ' AND c.City = @City';
IF @MinTotalAmount IS NOT NULL
SET @SQL = @SQL + ' AND s.TotalAmount >= @MinTotalAmount';
SET @ParameterDefinition = N'
@StartDate DATE,
@EndDate DATE,
@City NVARCHAR(50),
@MinTotalAmount DECIMAL(10, 2)';
EXEC sp_executesql
@SQL,
@ParameterDefinition,
@StartDate = @StartDate,
@EndDate = @EndDate,
@City = @City,
@MinTotalAmount = @MinTotalAmount;
Conclusion
Using sp_executesql
for dynamic SQL in SQL Server provides a robust and secure way to execute dynamically constructed queries. By leveraging parameterization, we can enhance performance and security, particularly in complex scenarios requiring flexible query construction based on varying input parameters.
In this article, we demonstrated the use of sp_executesql
with a complex example, showcasing how to dynamically build and execute SQL queries with multiple optional filters. This approach can be adapted and extended to fit a wide range of use cases, making your SQL Server applications more dynamic and responsive to user inputs. If you’re looking to implement such advanced techniques in your projects, consider to hire SQL Server developers. They can bring the expertise needed to optimize your database performance, enhance security, and ensure the scalability of your applications.
WireFuture's team spans the globe, bringing diverse perspectives and skills to the table. This global expertise means your software is designed to compete—and win—on the world stage.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.