How to Send a Message to a Microsoft Teams Channel in C#
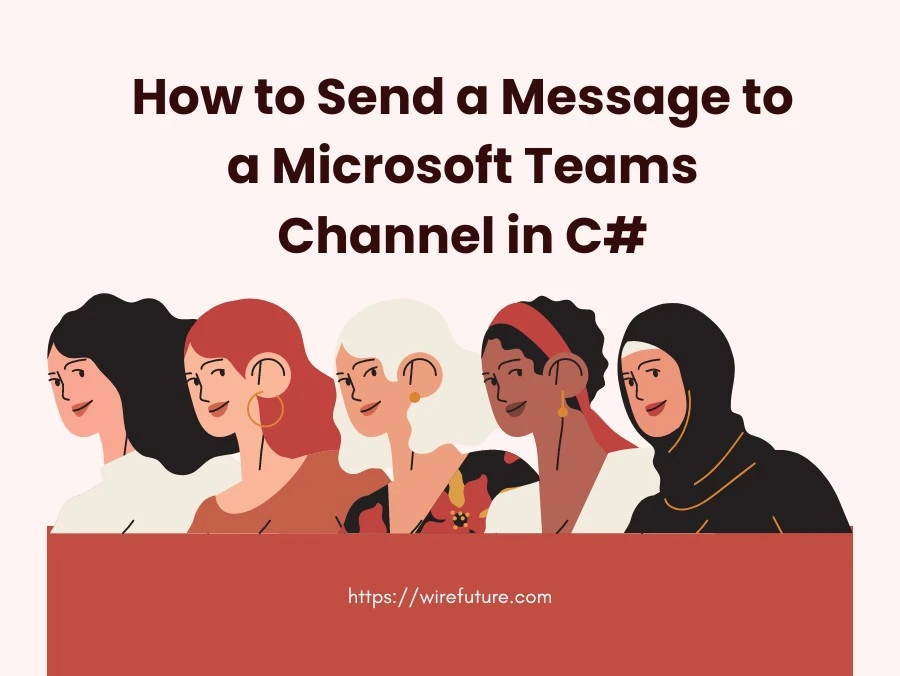
Microsoft Teams is a popular collaboration tool used extensively in organizations for communication and teamwork. Webhooks are one way to integrate and automate tasks in Teams. Webhooks let you send a message to a Microsoft Teams channel from within your application. In this article, we will explore how to send messages to a Teams channel using a webhook in C#, utilizing JSON.NET for JSON handling. We will cover the introduction, use cases, detailed code examples, limitations and a summary.
Table of Contents
Introduction
A webhook in Microsoft Teams allows external services to send messages to a Teams channel. This integration is particularly useful for automating notifications, alerts, or updates from your application or service. Webhooks provide a simple way to push data to Teams without requiring a full-fledged bot or a complex API integration.
To send a message via a webhook, you need to:
- Create an Incoming Webhook in Teams: Obtain a webhook URL for the desired channel.
- Send a POST Request: Use the webhook URL to send JSON payloads from your C# application
Sample Use Cases
- Alert Notifications: Automatically send alerts to a Teams channel when specific events occur, such as server downtime, failed builds, or exceeded thresholds.
- Daily Reports: Post daily or periodic reports, like sales summaries or usage statistics, directly into a Teams channel.
- Task Updates: Notify a team about updates to tasks or issues in project management tools.
- Deployment Messages: Inform the team about successful or failed deployments of applications or services.
Code Sample
Here’s a step-by-step guide to sending messages to a Teams channel using a webhook in C# with .NET 8:
Step 1: Create an Incoming Webhook in Teams
- Go to the Microsoft Teams app.
- Navigate to the channel where you want to send messages.
- Click on the ellipsis (
...
) next to the channel name, then selectConnectors
. - Find and configure the
Incoming Webhook
connector, providing it a name and an image if desired. - Copy the webhook URL provided by Teams.
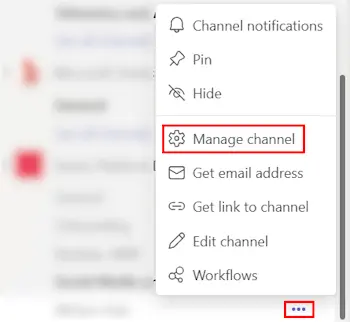
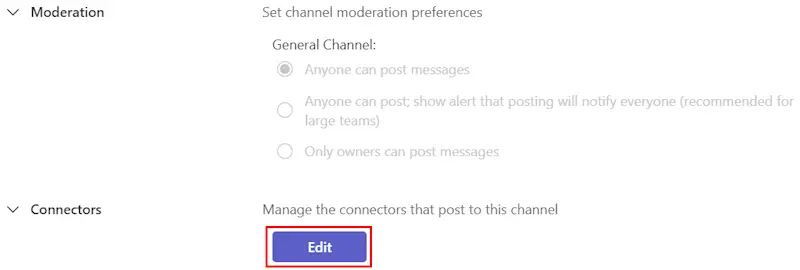
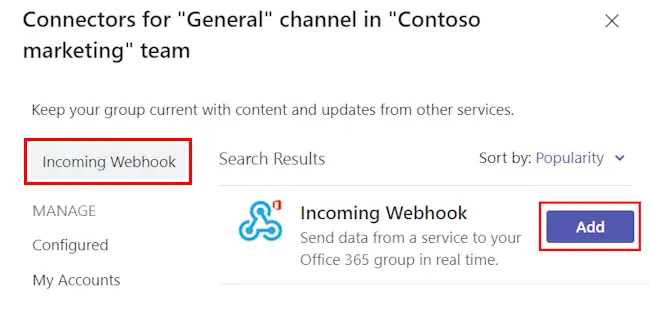
Step 2: Send a POST Request from C#
We’ll use HttpClient
in .NET 8 to send a POST request to the webhook URL. JSON.NET will handle the JSON serialization.
Install JSON.NET
Add the JSON.NET package to your project using NuGet:
dotnet add package Newtonsoft.Json
C# Code Example
Here’s a complete example of sending a message:
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
public class TeamsWebhookClient
{
private static readonly HttpClient httpClient = new HttpClient();
private readonly string webhookUrl;
public TeamsWebhookClient(string webhookUrl)
{
this.webhookUrl = webhookUrl;
}
public async Task SendMessageAsync(string title, string message)
{
var payload = new
{
text = $"**{title}**\n{message}"
};
var jsonPayload = JsonConvert.SerializeObject(payload);
var content = new StringContent(jsonPayload, Encoding.UTF8, "application/json");
var response = await httpClient.PostAsync(webhookUrl, content);
response.EnsureSuccessStatusCode();
Console.WriteLine("Message sent successfully!");
}
}
class Program
{
static async Task Main(string[] args)
{
string webhookUrl = "YOUR_WEBHOOK_URL";
var client = new TeamsWebhookClient(webhookUrl);
await client.SendMessageAsync("Deployment Status", "The application was deployed successfully.");
}
}
Explanation
- TeamsWebhookClient Class: Manages the HTTP request to the Teams webhook.
- SendMessageAsync Method: Creates a JSON payload and sends it as a POST request.
- Program Class: Demonstrates how to use the
TeamsWebhookClient
to send a message.
Replace "YOUR_WEBHOOK_URL"
with the actual webhook URL obtained from Teams.
Limitations
While webhooks are a powerful tool, they come with certain limitations:
- No Attachments: You cannot send files, images, or attachments. Webhooks support only plain text and card formatting. For this you should upload your files somewhere on Azure Blob storage or likewise and then include the URL in the message that you want to send.
- Limited Formatting: Messages can be formatted using basic Markdown, but advanced formatting or embedding multimedia is not supported.
- No Response Handling: Webhooks are one-way communication. You cannot receive or handle responses from Teams using a webhook.
- Rate Limits: There may be rate limits imposed by Teams on the number of messages sent via webhooks to prevent abuse.
Conclusion
To send a message to a Microsoft Teams channel via webhooks is a simple way to integrate external apps into Teams. With minimal setup, you can push notifications, updates, and reports directly into your team’s communication flow. However, keep in mind the limitations of attachments and response handling when designing your integration.
This approach, using .NET 8 and JSON.NET for JSON processing, gives a modern and robust way to programmatically interact with Teams. As your integration needs grow, you can build Teams bots or use the Microsoft Graph API for richer interactions. For tailored solutions, consider leveraging .NET software development company to meet your specific integration requirements.
WireFuture's team spans the globe, bringing diverse perspectives and skills to the table. This global expertise means your software is designed to compete—and win—on the world stage.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.