How to Setup Azure OBO in ASP.NET Core & Angular
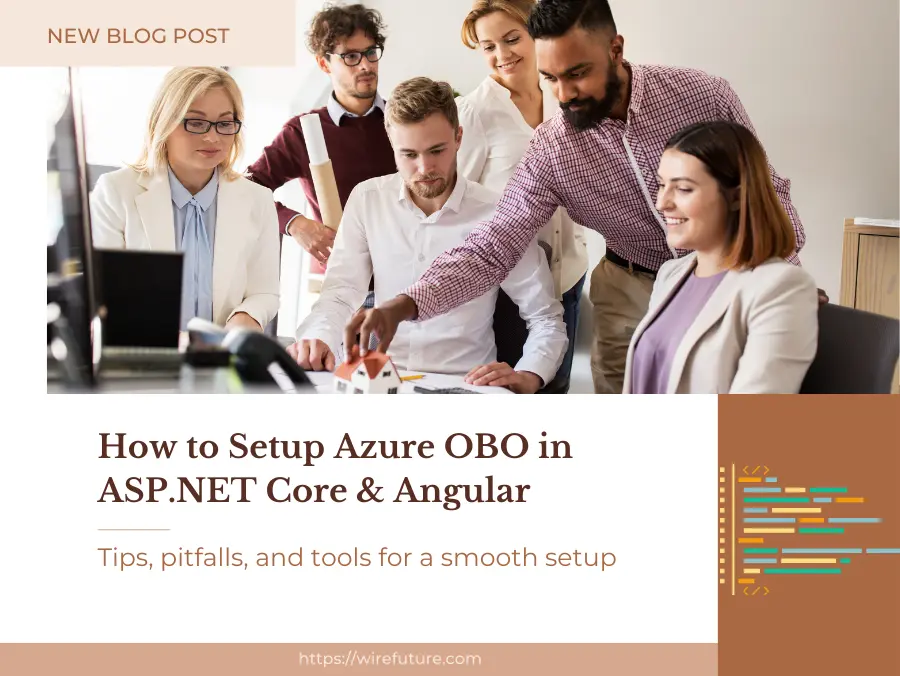
Basically, the Azure OBO flow is an authentication/authorization dance – where your application requests access to resources or APIs on behalf of a user. Now imagine this: Your Angular application is much more than an application itself – it is a portal where your visitors get access to a range of services along with information. Your ASP.NET Core backend serves as the middleman, the trusted envoy that routes requests from your Angular front to the world of APIs and resources. But how does this envoy demonstrate that it is legitimate to have those resources? How does it validate the request, which the user gave permission for it to act on their behalf? Here is where the OBO flow really shines: it gives your backend a standardized way to get access tokens for other resources while keeping the user’s credentials safe.
Secure access delegation is indispensable. In an age when data breaches are as common as rain during rainy season, you have to ensure that backend services can speak to your application securely. It’s not just data protection – it’s about protecting trust – the digital currency. When your Angular frontend needs to communicate with your ASP.NET Core backend, which in turn needs to connect to other resources or APIs, the Azure OBO flow guarantees these conversations are not whispers in the dark but authenticated conversations.
Why is the OBO flow the secret sauce for your ASP.NET Core and Angular app security? It’s very straightforward: it demonstrates a trusted path for delegation of access, ensuring that every request is validated and approved, defending your users and your application from digital smears.
Table of Contents
- Prerequisites
- Setting Up Active Directory for Azure OBO Flow
- Configuring the ASP.NET Core Backend
- Angular App Authentication Setup
- Implementing the Azure OBO Flow in Angular
- Wrapping Up
- Additional Resources
Prerequisites
Several prerequisites must be prepared before starting the intricate process of implementing the On-Behalf-Of (OBO) flow in your ASP.NET Core and Angular applications. These are foundational elements for a successful implementation. The requirements are described below:
Azure Subscriptions: An active Azure subscription is essential. This is your gateway to Microsoft cloud services, and where you can control Azure resources. It is a prerequisite for creating and managing Azure AD tenant(s) and registering apps in the Azure portal. In case you do not have an Azure subscription yet, you are able to begin by registering for a no cost trial or choosing the right payment schedule on the Azure site.
Knowledge of ASP.NET Core and Angular: A fundamental understanding and working knowledge of ASP.NET Core and Angular are mandatory. ASP.NET Core is the backbone of your backend service, it processes requests and provides security settings, and Angular is the fluid front-end. Familiarity with these technologies is crucial, as the OBO flow requires nuanced setups and changes in the backend as well as the frontend of your application.
Azure AD (AD) Tenant: An Azure AD tenant is an instance of Azure AD with accounts and groups. It’s important for managing and securing your apps and resources. You need an Azure AD tenant to register your apps and set up authentication and authorization. In case you have not yet configured an Azure AD tenant, do it within the Azure portal under Azure Active Directory.
Versions of Tools or Libraries that are specific: Using the right versions of tools and libraries is essential. ASP.NET Core development requires the.NET SDK for the target framework version of your project. For Angular development, ensure that Node.js along with Angular CLI versions meet your project needs. Also, the Microsoft.Identity.Web Library for ASP.NET Core and the MSAL (Microsoft Authentication library) for Angular are required to implement the OBO flow. Verify you are using the latest versions of these libraries, or of specific versions recommended by current best practices, to benefit from the most recent security features along with improvements.
Setting Up Active Directory for Azure OBO Flow
To facilitate a secure and efficient authentication process using the On-Behalf-Of (OBO) flow in your applications, the initial step involves the meticulous setup of Azure Active Directory (AD). It includes registering your ASP.NET Core API and Angular application in the Azure portal and then configuring permissions and API access to your specific needs. These steps are critical to enabling your applications to securely request resource access on the user’s behalf. This setup is described below:
Registering the ASP.NET Core API and Angular Application in the Azure Portal
- Access the Azure Portal: Navigate to the Azure Portal (portal.azure.com) and log in with your credentials. Ensure that you have the necessary permissions to create new registrations within your Azure AD tenant.
- Register the ASP.NET Core API:
- Within the Azure Portal, locate and select the “Azure Active Directory” service.
- Navigate to the “App registrations” section and select “New registration”.
- Provide a meaningful name for your ASP.NET Core API registration, specify supported account types, and optionally define a Redirect URI (this is more relevant for web applications that require a reply URL).
- Upon completion, Azure AD assigns a unique Application (client) ID to your API. Note this ID, as it will be used in your ASP.NET Core application’s configuration.
- Register the Angular Application:
- Repeat the registration process for your Angular application, ensuring that you provide distinct names and settings appropriate for a client application. This includes setting up a Redirect URI that matches your Angular application’s login and logout redirect patterns.
- Azure AD will assign a unique Application (client) ID to your Angular application as well. This ID is crucial for configuring the MSAL library in your Angular application.
Setting Up Permissions and API Access for Both Applications
- Configure API Permissions for the Angular Application:
- Within the App registration for your Angular application, navigate to the “API permissions” section.
- Click “Add a permission”, then select “My APIs” and find your previously registered ASP.NET Core API.
- Add the required permissions that your Angular application needs to request on behalf of the user. These permissions should match the scopes defined in your ASP.NET Core API.
- Grant Admin Consent:
- Still within the “API permissions” section, an administrator must grant consent for the permissions requested by your applications. This step is necessary for permissions that require admin consent and ensures that your application can access the API on behalf of users without individual consent prompts.
- Specify Authorized Client Applications:
- For the ASP.NET Core API registration, you need to specify the Angular application as an authorized client application. This involves adding the Application (client) ID of your Angular application to the manifest of the ASP.NET Core API registration.
- Navigate to the “Manifest” section of your API registration and locate the
knownClientApplications
array. Add the Application (client) ID of your Angular application here. This step is crucial for enabling the Azure OBO flow between your Angular application and ASP.NET Core API.
For the OBO flow to function correctly, it’s essential to configure specific delegated permissions in your API that support this flow. This is where the expertise of a .NET development company can transform your project, ensuring secure, efficient, and innovative solutions. This often involves permissions like User.Read
, Mail.Read
, or other scopes that allow your application to access resources or perform actions on behalf of the user. Ensure these permissions are defined in your ASP.NET Core API’s registration and requested by your Angular application.
Configuring the ASP.NET Core Backend
Firstly, ensure that your ASP.NET Core project is utilizing the Microsoft.Identity.Web library, which greatly simplifies the process of integrating Azure AD authentication. If you haven’t already added this package to your project, you can do so by running the following command in your terminal:
dotnet add package Microsoft.Identity.Web
Configuring Azure AD Authentication in .NET 7
.NET 7 continues to streamline and enhance the way developers can configure and work with services and middleware. Here’s how you can set up Azure AD authentication in your .NET 7 application:
Update Program.cs for Azure AD Authentication
In .NET 7, the Program.cs
file is where you configure services and middleware. Here’s how to set up Azure AD authentication:
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddMicrosoftIdentityWebApi(builder.Configuration.GetSection("AzureAd"));
builder.Services.AddControllers();
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthentication();
app.UseAuthorization();
app.MapControllers();
app.Run();
In your appsettings.json
, include your Azure AD configuration settings:
{
"AzureAd": {
"Instance": "https://login.microsoftonline.com/",
"Domain": "your-tenant-name.onmicrosoft.com",
"TenantId": "your-tenant-id",
"ClientId": "your-api-client-id",
"Audience": "api://your-api-client-id"
}
}
Implementing the Azure OBO Flow in Your ASP.NET Core Application
With your application now capable of authenticating users via Azure AD, let’s implement the OBO flow to allow your backend to call downstream APIs on behalf of the user.
Injecting and Using ITokenAcquisition
First, ensure your controllers or services are capable of acquiring tokens for downstream API calls:
using Microsoft.Identity.Web;
public class YourService
{
private readonly ITokenAcquisition _tokenAcquisition;
private readonly HttpClient _httpClient;
public YourService(ITokenAcquisition tokenAcquisition, HttpClient httpClient)
{
_tokenAcquisition = tokenAcquisition;
_httpClient = httpClient;
}
public async Task<string> CallDownstreamApiAsync()
{
var scopes = new[] { "api://downstream-api-client-id/.default" };
var accessToken = await _tokenAcquisition.GetAccessTokenForUserAsync(scopes);
_httpClient.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Bearer", accessToken);
var response = await _httpClient.GetStringAsync("https://downstream-api-url");
return response;
}
}
In this code, ITokenAcquisition
is used to acquire an access token for the specified scopes, which is then used to authenticate the request to the downstream API.
Angular Integration
For the latest Angular version, ensure you’re using MSAL (Microsoft Authentication Library) for Angular to handle authentication on the client side. The MSAL library provides easy integration with Azure AD.
- Install MSAL for Angular:
npm install @azure/msal-angular @azure/msal-browser
- Configure MSAL in Your Angular App:
Update your app.module.ts
to include MSAL configuration that aligns with your Azure AD setup:
import { MsalModule, MSAL_INSTANCE, MsalService, MSAL_GUARD_CONFIG, MsalGuardConfiguration, MsalGuard, MsalRedirectComponent } from '@azure/msal-angular';
import { PublicClientApplication } from '@azure/msal-browser';
export function MSALInstanceFactory(): PublicClientApplication {
return new PublicClientApplication({
auth: {
clientId: 'your-angular-app-client-id',
authority: 'https://login.microsoftonline.com/your-tenant-id',
redirectUri: 'http://localhost:4200'
}
});
}
export function MSALGuardConfigFactory(): MsalGuardConfiguration {
return {
interactionType: InteractionType.Redirect,
authRequest: {
scopes: ['user.read']
}
};
}
@NgModule({
declarations: [
// Your components
],
imports: [
// Other modules
MsalModule.forRoot(MSALInstanceFactory, MSALGuardConfigFactory)
],
providers: [
{
provide: MSAL_INSTANCE,
useFactory: MSALInstanceFactory
},
{
provide: MSAL_GUARD_CONFIG,
useFactory: MSALGuardConfigFactory
},
MsalService,
MsalGuard
],
bootstrap: [AppComponent, MsalRedirectComponent]
})
export class AppModule { }
In this configuration, replace 'your-angular-app-client-id'
and 'your-tenant-id'
with your actual Azure AD application client ID and tenant ID. This setup enables your Angular application to authenticate users and request access tokens for accessing secured endpoints in your ASP.NET Core backend.
Angular App Authentication Setup
Setting up authentication in your Angular application using the Microsoft Authentication Library (MSAL) allows for secure sign-in and access to protected resources in your ASP.NET Core backend, leveraging Azure Active Directory (AD). This setup ensures that your Angular application can authenticate users and acquire tokens for accessing resources securely. Hire ASP.NET Core developers thet offer the specialized expertise needed to drive innovation and security in your projects.
Below is a detailed guide to integrating MSAL into your Angular application.
Implementing MSAL for User Authentication
MSAL for Angular is designed to facilitate authentication with Azure AD, enabling your Angular application to authenticate users and request access tokens from Azure AD to access protected resources.
- Install MSAL for Angular: First, add MSAL to your Angular project. This library includes the authentication service and components required for user sign-in and token acquisition. Run the following command in your project directory:
npm install @azure/msal-angular @azure/msal-browser
This command installs the MSAL Angular library along with its dependency, @azure/msal-browser
, which is the core library for JavaScript applications.
- Register Your Application in Azure AD: Before configuring MSAL in your Angular app, ensure your application is registered in Azure AD and has the necessary permissions to access the ASP.NET Core backend. Note the Application (client) ID and configure the redirect URIs to match your Angular application’s URL.
Configuring Your app.module.ts
to Include MSAL Modules and Services
After installing MSAL, integrate it into your Angular application by configuring the app.module.ts
file. This involves importing MSAL modules and providing the configuration details for your Azure AD application.
- Import MSAL Modules: Modify your
app.module.ts
to include the MSAL modules and configure the MSAL service with your Azure AD application’s details.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { MsalModule, MSAL_INSTANCE, MsalService } from '@azure/msal-angular';
import { PublicClientApplication } from '@azure/msal-browser';
import { AppComponent } from './app.component';
export function MSALInstanceFactory() {
return new PublicClientApplication({
auth: {
clientId: 'Your-Azure-AD-Application-Client-ID',
authority: 'https://login.microsoftonline.com/Your-Tenant-ID',
redirectUri: 'Your-Redirect-URI'
}
});
}
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
MsalModule
],
providers: [
{
provide: MSAL_INSTANCE,
useFactory: MSALInstanceFactory
},
MsalService
],
bootstrap: [AppComponent]
})
export class AppModule { }
Replace 'Your-Azure-AD-Application-Client-ID'
, 'Your-Tenant-ID'
, and 'Your-Redirect-URI'
with your actual Azure AD registration details.
Using MSAL to Acquire Tokens for Accessing the ASP.NET Core Backend
With MSAL configured, your Angular application can authenticate users and acquire access tokens to call protected endpoints in your ASP.NET Core backend.
- Inject
MsalService
and Use It to Acquire Tokens: Inject theMsalService
into your components or services where you need to acquire tokens to call your backend.
import { Component, OnInit } from '@angular/core';
import { MsalService } from '@azure/msal-angular';
@Component({
selector: 'app-secure-component',
templateUrl: './secure-component.component.html',
styleUrls: ['./secure-component.component.css']
})
export class SecureComponent implements OnInit {
constructor(private authService: MsalService) { }
ngOnInit() {
this.authService.loginPopup()
.subscribe((response: AuthenticationResult) => {
this.authService.acquireTokenSilent({
scopes: ['api://your-backend-app-id-uri/user_impersonation'],
account: response.account
}).subscribe(tokenResponse => {
// You now have the token to make requests to your ASP.NET Core backend
console.log(tokenResponse.accessToken);
});
});
}
}
This sample demonstrates a simple login flow initiating a popup for user authentication, followed by a silent token acquisition for accessing the backend. The scopes specified should match those configured in your ASP.NET Core application’s Azure AD app registration.
Implementing the Azure OBO Flow in Angular
First, ensure you have a service in your Angular application dedicated to interacting with your ASP.NET Core backend. This service will be responsible for making HTTP requests to your backend, and it needs to include the Authorization header with the access token acquired from Azure AD.
- Create or Update an Angular Service: Ensure you have a service in your Angular application for making HTTP requests. Here’s a basic example:
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { MsalService } from '@azure/msal-angular';
import { from, Observable } from 'rxjs';
import { mergeMap } from 'rxjs/operators';
@Injectable({
providedIn: 'root'
})
export class BackendService {
private readonly apiUrl = 'https://your-backend-api-url';
constructor(private http: HttpClient, private msalService: MsalService) { }
getProtectedData(): Observable<any> {
// Define the scopes your API requires
const scopes = ['api://your-backend-app-id-uri/.default'];
return from(this.msalService.acquireTokenSilent({ scopes })).pipe(
mergeMap(tokenResponse => {
const headers = new HttpHeaders().set('Authorization', `Bearer ${tokenResponse.accessToken}`);
return this.http.get(`${this.apiUrl}/protected-endpoint`, { headers });
})
);
}
}
This service demonstrates how to acquire a token silently using MSAL’s acquireTokenSilent
method, specifying the necessary scopes for your backend. Once the token is acquired, it’s used to set the Authorization header for the HTTP request to your backend’s protected endpoint.
Handling Token Acquisition Silently
The key to a smooth user experience is acquiring tokens silently without requiring the user to re-authenticate or interrupting their workflow. The acquireTokenSilent
method provided by MSAL Angular attempts to acquire a token from the cache or silently from Azure AD if the cache entry is expired.
- Fallback to Interactive Method if Needed: In cases where the silent token acquisition fails (for example, if the user’s login session has expired), you should gracefully fallback to an interactive method (like a popup or redirect) to acquire the token. Ensure your application handles these scenarios to avoid unhandled exceptions and maintain a seamless user experience.
Here’s how you can extend the service to include a fallback mechanism:
getProtectedDataWithFallback(): Observable<any> {
const scopes = ['api://your-backend-app-id-uri/.default'];
return from(this.msalService.acquireTokenSilent({ scopes })).pipe(
mergeMap(tokenResponse => {
const headers = new HttpHeaders().set('Authorization', `Bearer ${tokenResponse.accessToken}`);
return this.http.get(`${this.apiUrl}/protected-endpoint`, { headers });
}),
catchError(error => {
// Handle specific errors, e.g., interaction required
if (this.isInteractionRequired(error)) {
// Fallback to an interactive method
return from(this.msalService.acquireTokenPopup({ scopes })).pipe(
mergeMap(tokenResponse => {
const headers = new HttpHeaders().set('Authorization', `Bearer ${tokenResponse.accessToken}`);
return this.http.get(`${this.apiUrl}/protected-endpoint`, { headers });
})
);
}
throw error;
})
);
}
private isInteractionRequired(error: any): boolean {
// Implement logic to determine if interaction is required based on the error
return true; // Simplified for example purposes
}
In this enhanced service method, getProtectedDataWithFallback
, a fallback to acquireTokenPopup
is triggered if silent acquisition fails, ensuring that the user can continue their workflow with minimal interruption.
Wrapping Up
Ending our On-Behalf-Of (OBO) flow with ASP.NET Core and Angular, we went from setup to secure deployment – all to secure our apps while ensuring they run on user behalf. This exploration highlights the need for secure access delegation for enhanced application functionality and user experience. Now is your turn to apply these insights to your projects and see the change. Explore the Azure OBO flow, and let us know your stories, struggles, or questions. Each feedback contributes to our knowledge base and helps build a developer community.
Would like to unleash ASP.NET in your next project? WireFuture is an ASP.NET Development Company that can help you realize your vision. Our focus on innovation and security makes sure your projects are innovative yet secure. Team up with WireFuture and turn your concepts into reality – setting new digital standards. Let’s create effective, secure and future-proof solutions together.
Additional Resources
Official Microsoft Documentation
- OBO Flow with Microsoft Identity Platform: Start with the foundational knowledge directly from the source. Microsoft’s official documentation on the OBO flow provides a comprehensive overview, including scenarios, protocol diagrams, and step-by-step guidance. Explore it at Microsoft Identity Platform and the OAuth 2.0 On-Behalf-Of flow.
- MSAL Libraries: To effectively implement authentication in your applications, understanding MSAL is crucial. MSAL simplifies the process of working with Microsoft Identity Platform. For detailed documentation on MSAL, including code samples for various platforms, visit Microsoft Authentication Library (MSAL) documentation.
Tutorials and Hands-On Learning
- Integrating MSAL in Angular Applications: For Angular developers, integrating MSAL to manage authentication can seem daunting at first. The Microsoft Identity Platform docs provide a tutorial on integrating MSAL with Angular, guiding you through creating a sample application that uses MSAL Angular to authenticate users.
- Secure ASP.NET Core Web API using the Azure OBO Flow: If you’re developing an ASP.NET Core Web API and looking to secure it using the OBO flow, Microsoft offers a step-by-step tutorial that walks you through the configuration and coding required to secure your API and call downstream APIs securely.
Our team of software development experts is here to transform your ideas into reality. Whether it's cutting-edge applications or revamping existing systems, we've got the skills, the passion, and the tech-savvy crew to bring your projects to life. Let's build something amazing together!
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.