How To Use Entity Framework Core in .NET 8 Efficiently
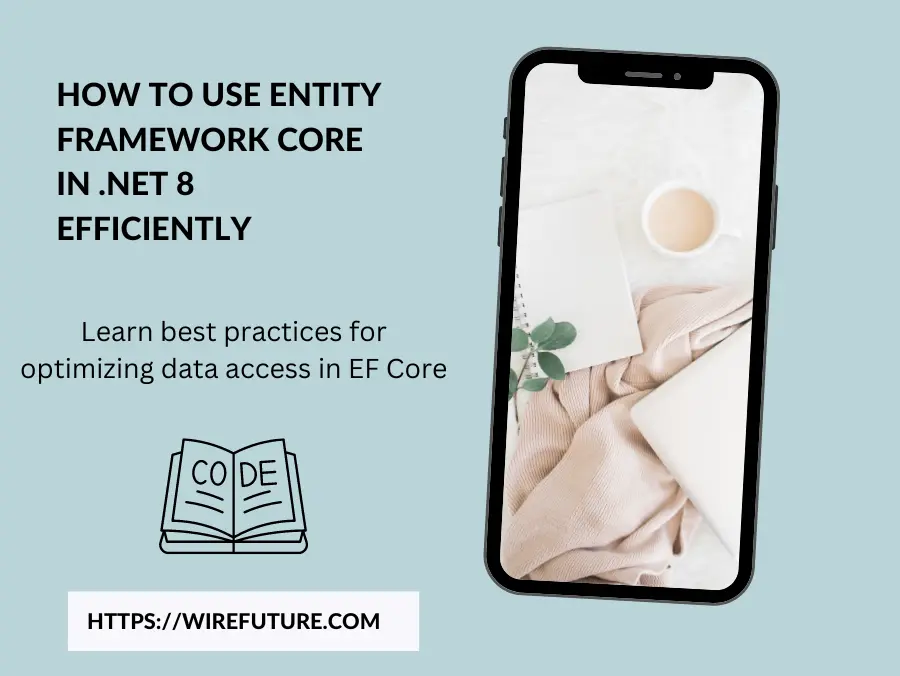
Entity Framework Core (EF Core) in .NET 8 is a powerful data access tool that makes It straightforward to work with databases using .NET; It enables developers to interact with a database directly using .NET objects, without writing most of the data-access code that developers typically write. – This version is even more efficient and flexible with numerous improvements and new features.
In today’s applications, efficient data access is critical. As data volume grows and real-time processing becomes possible, applications must accommodate data access and manipulation quickly and effectively. Bad data access practices may lead to sluggish performance, higher latency, and a bad user experience. Properly using EF Core can avoid these issues while making your applications faster and more responsive. Partnering with a .NET development company ensures you leverage the full potential of EF Core, implementing best practices to optimize data access and enhance your application’s performance.
This article aims to provide tips for using EF Core with .NET 8. Use these tricks and tips to optimize your data access layer, handle complex queries, and raise the performance of your applications overall. Regardless if you’re a brand-new user or even an experienced user of EF Core, these best practices can help you improve your experience with the tool.
Table of Contents
- Best Practices for Using Entity Framework Core in .NET 8
- Configuring DbContext Properly
- Optimizing Queries for Performance
- Efficient Change Tracking
- Batching and Transactions
- Managing Relationships and Navigations In Entity Framework Core
- Handling Migrations and Schema Changes In Entity Framework Core
- Performance Profiling and Tuning
- Dealing with Complex Queries In Entity Framework Core
- Error Handling and Logging In Entity Framework Core
- Conclusion
- Additional Resources
Best Practices for Using Entity Framework Core in .NET 8
Configuring DbContext Properly
Proper configuration of the DbContext
is crucial for ensuring optimal performance and preventing issues such as memory leaks. Here are some best practices for configuring DbContext
in .NET 8:
1. Use a Single DbContext Instance per Request
Using a single DbContext
instance per request helps to avoid memory leaks and ensures better performance. In an ASP.NET Core application, you can achieve this by registering the DbContext
with a scoped lifetime in the Startup
class or using the new minimal API approach in .NET 8.
// Program.cs in .NET 8 minimal API
var builder = WebApplication.CreateBuilder(args);
// Register DbContext with scoped lifetime
builder.Services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(builder.Configuration.GetConnectionString("DefaultConnection")));
var app = builder.Build();
app.MapGet("/", async (MyDbContext context) =>
{
var data = await context.MyEntities.ToListAsync();
return Results.Ok(data);
});
app.Run();
2. Securely Configure Connection Strings
Store connection strings securely using environment variables or configuration settings. This ensures that sensitive information, like database credentials, is not hard-coded in the application.
// appsettings.json
{
"ConnectionStrings": {
"DefaultConnection": "Server=.;Database=MyDatabase;User Id=myUsername;Password=myPassword;"
}
}
// Program.cs in .NET 8 minimal API
var builder = WebApplication.CreateBuilder(args);
// Read connection string from configuration
var connectionString = builder.Configuration.GetConnectionString("DefaultConnection");
builder.Services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(connectionString));
var app = builder.Build();
app.MapGet("/", async (MyDbContext context) =>
{
var data = await context.MyEntities.ToListAsync();
return Results.Ok(data);
});
app.Run();
3. Enable and Use Lazy Loading Judiciously
Lazy loading can be useful but should be used carefully to prevent unnecessary data fetching. It can be enabled by installing the Microsoft.EntityFrameworkCore.Proxies
package and configuring it in your DbContext
.
// Program.cs in .NET 8 minimal API
var builder = WebApplication.CreateBuilder(args);
// Register DbContext with lazy loading enabled
builder.Services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(builder.Configuration.GetConnectionString("DefaultConnection"))
.UseLazyLoadingProxies());
var app = builder.Build();
app.MapGet("/", async (MyDbContext context) =>
{
var data = await context.MyEntities.ToListAsync();
return Results.Ok(data);
});
app.Run();
// MyDbContext.cs
using Microsoft.EntityFrameworkCore;
using Microsoft.EntityFrameworkCore.Proxies;
public class MyDbContext : DbContext
{
public MyDbContext(DbContextOptions<MyDbContext> options)
: base(options)
{
}
public DbSet<MyEntity> MyEntities { get; set; }
// Enable lazy loading proxies
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseLazyLoadingProxies();
}
}
// MyEntity.cs
public class MyEntity
{
public int Id { get; set; }
public string Name { get; set; }
// Navigation property for lazy loading
public virtual ICollection<RelatedEntity> RelatedEntities { get; set; }
}
By following these practices, you can ensure that your DbContext
is configured properly, leading to better performance and more manageable code.
Optimizing Queries for Performance
Optimizing your queries is essential for ensuring your application performs well, especially when dealing with large datasets or complex operations. Here are some best practices along with code samples to help you optimize your queries in Entity Framework Core.
1. Prefer Asynchronous Methods for I/O-bound Operations
Asynchronous methods help to avoid blocking the main thread, making your application more responsive and capable of handling more concurrent operations.
// Asynchronous method example
var data = await _context.MyEntities.ToListAsync();
// Other asynchronous query methods
var firstEntity = await _context.MyEntities.FirstOrDefaultAsync();
var singleEntity = await _context.MyEntities.SingleOrDefaultAsync(e => e.Id == 1);
var count = await _context.MyEntities.CountAsync();
var anyExist = await _context.MyEntities.AnyAsync();
2. Utilize Projection Queries to Fetch Only Required Columns
Projection queries allow you to select only the columns you need, which reduces the amount of data retrieved and improves performance.
// Fetch only specific columns using projection
var projectedData = await _context.MyEntities
.Select(e => new
{
e.Id,
e.Name
})
.ToListAsync();
3. Use Compiled Queries for Frequently Executed Queries
Compiled queries can significantly improve performance by caching the query execution plan. This is particularly useful for queries that are executed frequently with different parameters.
// Define a compiled query
private static readonly Func<MyDbContext, int, Task<MyEntity>> GetEntityByIdAsync =
EF.CompileAsyncQuery((MyDbContext context, int id) =>
context.MyEntities.FirstOrDefault(e => e.Id == id));
// Use the compiled query
var entity = await GetEntityByIdAsync(_context, 1);
// Another example of a compiled query with projection
private static readonly Func<MyDbContext, Task<List<MyEntityProjection>>> GetAllEntitiesProjectionAsync =
EF.CompileAsyncQuery((MyDbContext context) =>
context.MyEntities.Select(e => new MyEntityProjection
{
Id = e.Id,
Name = e.Name
}).ToListAsync());
var projectedEntities = await GetAllEntitiesProjectionAsync(_context);
Combined Example
Here’s an example combining these best practices in a realistic scenario:
public class MyService
{
private readonly MyDbContext _context;
public MyService(MyDbContext context)
{
_context = context;
}
// Asynchronous method using projection and compiled query
public async Task<List<MyEntityProjection>> GetEntitiesAsync()
{
// Use projection to fetch only required columns
return await _context.MyEntities
.Select(e => new MyEntityProjection
{
Id = e.Id,
Name = e.Name
})
.ToListAsync();
}
// Asynchronous method using a compiled query
public async Task<MyEntity> GetEntityByIdAsync(int id)
{
return await GetEntityByIdCompiledAsync(_context, id);
}
private static readonly Func<MyDbContext, int, Task<MyEntity>> GetEntityByIdCompiledAsync =
EF.CompileAsyncQuery((MyDbContext context, int id) =>
context.MyEntities.FirstOrDefault(e => e.Id == id));
}
// Entity and projection classes
public class MyEntity
{
public int Id { get; set; }
public string Name { get; set; }
}
public class MyEntityProjection
{
public int Id { get; set; }
public string Name { get; set; }
}
Efficient Change Tracking
Efficient change tracking is crucial for ensuring that your application performs well, especially when dealing with large datasets or complex object graphs. Entity Framework Core (EF Core) provides several features and methods to help manage change tracking efficiently. Here are some best practices along with code samples to help you optimize change tracking in EF Core.
1. Disable Change Tracking for Read-Only Queries with AsNoTracking
When you know that a query is read-only and you don’t need to track changes to the retrieved entities, you can use the AsNoTracking
method. This can significantly enhance performance by avoiding the overhead of tracking changes.
// Without AsNoTracking (default behavior)
var products = await _context.Products.ToListAsync();
// With AsNoTracking (better performance for read-only queries)
var productsNoTracking = await _context.Products.AsNoTracking().ToListAsync();
// Another example with a filter
var expensiveProducts = await _context.Products
.AsNoTracking()
.Where(p => p.Price > 1000)
.ToListAsync();
// Using AsNoTracking for a single entity
var product = await _context.Products
.AsNoTracking()
.FirstOrDefaultAsync(p => p.Id == 1);
2. Leverage TrackGraph
Method for Complex Object Graphs
The TrackGraph
method allows you to efficiently manage the state of complex object graphs, especially when dealing with disconnected entities. This method helps in setting the state of entities and their related entities in one go.
public async Task AddOrUpdateOrderAsync(Order order)
{
// Assume the order might be in a disconnected state
_context.ChangeTracker.TrackGraph(order, node =>
{
if (node.Entry.IsKeySet)
{
node.Entry.State = EntityState.Modified;
}
else
{
node.Entry.State = EntityState.Added;
}
});
await _context.SaveChangesAsync();
}
// Usage
var order = new Order
{
OrderDate = DateTime.UtcNow,
Customer = new Customer
{
Name = "John Doe"
},
OrderItems = new List<OrderItem>
{
new OrderItem
{
ProductId = 1,
Quantity = 2
},
new OrderItem
{
ProductId = 2,
Quantity = 1
}
}
};
await AddOrUpdateOrderAsync(order);
Combined Example
Here’s an example demonstrating both AsNoTracking
for read-only queries and TrackGraph
for handling complex object graphs:
public class ProductService
{
private readonly MyDbContext _context;
public ProductService(MyDbContext context)
{
_context = context;
}
// Method using AsNoTracking for read-only queries
public async Task<List<Product>> GetProductsAsync()
{
return await _context.Products
.AsNoTracking()
.ToListAsync();
}
// Method using TrackGraph for complex object graphs
public async Task AddOrUpdateOrderAsync(Order order)
{
_context.ChangeTracker.TrackGraph(order, node =>
{
if (node.Entry.IsKeySet)
{
node.Entry.State = EntityState.Modified;
}
else
{
node.Entry.State = EntityState.Added;
}
});
await _context.SaveChangesAsync();
}
}
// Entity classes
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
public class Order
{
public int Id { get; set; }
public DateTime OrderDate { get; set; }
public Customer Customer { get; set; }
public ICollection<OrderItem> OrderItems { get; set; }
}
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
}
public class OrderItem
{
public int Id { get; set; }
public int ProductId { get; set; }
public int Quantity { get; set; }
}
Batching and Transactions
Efficiently handling multiple database operations is key to improving the performance and reliability of your application. Batching operations can minimize database round-trips, while transactions ensure data consistency. Here are best practices for batching and using transactions in EF Core, along with detailed code samples.
1. Implement Batching for Multiple Database Operations
Batching allows you to perform multiple operations in a single round-trip to the database, reducing the overhead of multiple network calls.
// Example of batching multiple insert operations
public async Task AddProductsAsync(List<Product> products)
{
await _context.Products.AddRangeAsync(products);
await _context.SaveChangesAsync();
}
// Usage
var newProducts = new List<Product>
{
new Product { Name = "Product 1", Price = 100 },
new Product { Name = "Product 2", Price = 200 },
new Product { Name = "Product 3", Price = 300 }
};
await AddProductsAsync(newProducts);
// Example of batching multiple update operations
public async Task UpdateProductPricesAsync(List<Product> products)
{
_context.Products.UpdateRange(products);
await _context.SaveChangesAsync();
}
// Usage
var updatedProducts = await _context.Products.Where(p => p.Price < 500).ToListAsync();
foreach (var product in updatedProducts)
{
product.Price += 50;
}
await UpdateProductPricesAsync(updatedProducts);
2. Use Transactions (DbContextTransaction
) for a Group of Operations
Transactions ensure that a group of operations either all succeed or all fail, maintaining data consistency.
// Example of using a transaction for multiple operations
public async Task ProcessOrderAsync(Order order)
{
using var transaction = await _context.Database.BeginTransactionAsync();
try
{
// Add the order
await _context.Orders.AddAsync(order);
await _context.SaveChangesAsync();
// Update product inventory
foreach (var item in order.OrderItems)
{
var product = await _context.Products.FindAsync(item.ProductId);
if (product != null)
{
product.Stock -= item.Quantity;
_context.Products.Update(product);
}
}
await _context.SaveChangesAsync();
// Commit the transaction
await transaction.CommitAsync();
}
catch (Exception)
{
// Rollback the transaction in case of an error
await transaction.RollbackAsync();
throw;
}
}
// Usage
var order = new Order
{
OrderDate = DateTime.UtcNow,
CustomerId = 1,
OrderItems = new List<OrderItem>
{
new OrderItem { ProductId = 1, Quantity = 2 },
new OrderItem { ProductId = 2, Quantity = 1 }
}
};
await ProcessOrderAsync(order);
Combined Example
Here’s a comprehensive example that demonstrates both batching and transaction handling in a realistic scenario:
public class OrderService
{
private readonly MyDbContext _context;
public OrderService(MyDbContext context)
{
_context = context;
}
// Method to add products in batch
public async Task AddProductsAsync(List<Product> products)
{
await _context.Products.AddRangeAsync(products);
await _context.SaveChangesAsync();
}
// Method to process an order using a transaction
public async Task ProcessOrderAsync(Order order)
{
using var transaction = await _context.Database.BeginTransactionAsync();
try
{
// Add the order
await _context.Orders.AddAsync(order);
await _context.SaveChangesAsync();
// Update product inventory
foreach (var item in order.OrderItems)
{
var product = await _context.Products.FindAsync(item.ProductId);
if (product != null)
{
product.Stock -= item.Quantity;
_context.Products.Update(product);
}
}
await _context.SaveChangesAsync();
// Commit the transaction
await transaction.CommitAsync();
}
catch (Exception)
{
// Rollback the transaction in case of an error
await transaction.RollbackAsync();
throw;
}
}
}
// Entity classes
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public int Stock { get; set; }
}
public class Order
{
public int Id { get; set; }
public DateTime OrderDate { get; set; }
public int CustomerId { get; set; }
public ICollection<OrderItem> OrderItems { get; set; }
}
public class OrderItem
{
public int Id { get; set; }
public int ProductId { get; set; }
public int Quantity { get; set; }
}
// Usage
var newProducts = new List<Product>
{
new Product { Name = "Product 1", Price = 100, Stock = 10 },
new Product { Name = "Product 2", Price = 200, Stock = 20 },
new Product { Name = "Product 3", Price = 300, Stock = 30 }
};
var order = new Order
{
OrderDate = DateTime.UtcNow,
CustomerId = 1,
OrderItems = new List<OrderItem>
{
new OrderItem { ProductId = 1, Quantity = 2 },
new OrderItem { ProductId = 2, Quantity = 1 }
}
};
var orderService = new OrderService(_context);
await orderService.AddProductsAsync(newProducts);
await orderService.ProcessOrderAsync(order);
By following these practices and using the provided code samples, you can efficiently handle multiple database operations and ensure data consistency in your application.
Managing Relationships and Navigations In Entity Framework Core
Properly managing relationships and navigations in Entity Framework Core (EF Core) is essential for maintaining performance and ensuring that your data model is both efficient and easy to understand. Here are some best practices, expanded with detailed explanations and code samples.
1. Explicitly Configure Relationships Using Fluent API
Using the Fluent API to configure relationships between entities gives you more control and clarity over your data model. It ensures that relationships are clearly defined and makes your code more readable and maintainable.
Example: One-to-Many Relationship
public class Author
{
public int AuthorId { get; set; }
public string Name { get; set; }
public ICollection<Book> Books { get; set; }
}
public class Book
{
public int BookId { get; set; }
public string Title { get; set; }
public int AuthorId { get; set; }
public Author Author { get; set; }
}
public class MyDbContext : DbContext
{
public DbSet<Author> Authors { get; set; }
public DbSet<Book> Books { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Author>()
.HasMany(a => a.Books)
.WithOne(b => b.Author)
.HasForeignKey(b => b.AuthorId);
}
}
Example: Many-to-Many Relationship
public class Student
{
public int StudentId { get; set; }
public string Name { get; set; }
public ICollection<StudentCourse> StudentCourses { get; set; }
}
public class Course
{
public int CourseId { get; set; }
public string Title { get; set; }
public ICollection<StudentCourse> StudentCourses { get; set; }
}
public class StudentCourse
{
public int StudentId { get; set; }
public Student Student { get; set; }
public int CourseId { get; set; }
public Course Course { get; set; }
}
public class MyDbContext : DbContext
{
public DbSet<Student> Students { get; set; }
public DbSet<Course> Courses { get; set; }
public DbSet<StudentCourse> StudentCourses { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<StudentCourse>()
.HasKey(sc => new { sc.StudentId, sc.CourseId });
modelBuilder.Entity<StudentCourse>()
.HasOne(sc => sc.Student)
.WithMany(s => s.StudentCourses)
.HasForeignKey(sc => sc.StudentId);
modelBuilder.Entity<StudentCourse>()
.HasOne(sc => sc.Course)
.WithMany(c => c.StudentCourses)
.HasForeignKey(sc => sc.CourseId);
}
}
2. Avoid Excessive Eager Loading and Circular References
Eager loading is useful for loading related data, but it can lead to performance issues if overused. Circular references can cause infinite loops and stack overflow exceptions. To manage these, use eager loading judiciously and design your navigation properties carefully.
Example: Eager Loading
// Eager loading using Include
var authorsWithBooks = await _context.Authors
.Include(a => a.Books)
.ToListAsync();
// Avoiding excessive eager loading
var authorsWithSelectedBooks = await _context.Authors
.Include(a => a.Books.Where(b => b.Title.Contains("EF Core")))
.ToListAsync();
Handling Circular References
To avoid circular references, you can use the JsonIgnore
attribute or configure the serializer to handle circular references. If you want to learn how to use JSON in T-SQL with SQL Server, refer to our detailed guide: Working with JSON in SQL Server.
Example: Using JsonIgnore
public class Author
{
public int AuthorId { get; set; }
public string Name { get; set; }
[JsonIgnore]
public ICollection<Book> Books { get; set; }
}
public class Book
{
public int BookId { get; set; }
public string Title { get; set; }
public int AuthorId { get; set; }
[JsonIgnore]
public Author Author { get; set; }
}
Example: Configuring JSON Serializer
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers()
.AddJsonOptions(options =>
{
options.JsonSerializerOptions.ReferenceHandler = ReferenceHandler.Preserve;
});
}
Combined Example
Here’s an example demonstrating both explicit relationship configuration and careful management of eager loading:
public class LibraryService
{
private readonly MyDbContext _context;
public LibraryService(MyDbContext context)
{
_context = context;
}
// Method to get authors with their books using eager loading
public async Task<List<Author>> GetAuthorsWithBooksAsync()
{
return await _context.Authors
.Include(a => a.Books)
.ToListAsync();
}
// Method to add a new author and books with explicit relationship configuration
public async Task AddAuthorWithBooksAsync(Author author, List<Book> books)
{
foreach (var book in books)
{
book.Author = author;
}
await _context.Authors.AddAsync(author);
await _context.Books.AddRangeAsync(books);
await _context.SaveChangesAsync();
}
}
// Usage
var author = new Author { Name = "Jane Austen" };
var books = new List<Book>
{
new Book { Title = "Pride and Prejudice" },
new Book { Title = "Sense and Sensibility" }
};
var libraryService = new LibraryService(_context);
await libraryService.AddAuthorWithBooksAsync(author, books);
var authorsWithBooks = await libraryService.GetAuthorsWithBooksAsync();
Handling Migrations and Schema Changes In Entity Framework Core
Managing database schema changes effectively is crucial for maintaining the integrity and performance of your application. Entity Framework Core (EF Core) provides powerful tools to handle these changes through code-first migrations. Here’s how to utilize and apply migrations systematically, along with some best practices and code examples. Partnering with a company that provides ASP.NET development services can further enhance your ability to manage schema changes efficiently, ensuring your application remains robust and performant.
1. Utilize Code-First Migrations to Manage Database Schema Changes Systematically
Code-first migrations allow you to define and manage your database schema directly from your code. This makes it easier to track changes and maintain version control over your database schema.
Steps to Enable and Use Migrations:
Enable Migrations: Run the following command in the Package Manager Console to enable migrations:
Add-Migration InitialCreate
Update Database: Apply the migration to your database by running:
Update-Database
Example: Initial Migration
public class MyDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
public DbSet<Order> Orders { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Product>()
.HasKey(p => p.Id);
modelBuilder.Entity<Order>()
.HasKey(o => o.Id);
modelBuilder.Entity<Order>()
.HasMany(o => o.OrderItems)
.WithOne(oi => oi.Order)
.HasForeignKey(oi => oi.OrderId);
}
}
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public ICollection<OrderItem> OrderItems { get; set; }
}
public class Order
{
public int Id { get; set; }
public DateTime OrderDate { get; set; }
public ICollection<OrderItem> OrderItems { get; set; }
}
public class OrderItem
{
public int Id { get; set; }
public int ProductId { get; set; }
public int OrderId { get; set; }
public int Quantity { get; set; }
public Product Product { get; set; }
public Order Order { get; set; }
}
Adding New Fields or Entities:
When you need to add new fields or entities, you create a new migration to reflect these changes.
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
// Adding the new entity to the context
public class MyDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
public DbSet<Order> Orders { get; set; }
public DbSet<Customer> Customers { get; set; } // New entity
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Product>()
.HasKey(p => p.Id);
modelBuilder.Entity<Order>()
.HasKey(o => o.Id);
modelBuilder.Entity<Order>()
.HasMany(o => o.OrderItems)
.WithOne(oi => oi.Order)
.HasForeignKey(oi => oi.OrderId);
modelBuilder.Entity<Customer>()
.HasKey(c => c.Id); // Configuring new entity
}
}
// Running the migration
Add-Migration AddCustomerEntity
Update-Database
2. Apply Migrations Incrementally and Validate Them in a Staging Environment Before Production
Incremental migrations allow you to apply schema changes in a controlled manner. Validating migrations in a staging environment helps ensure that they do not introduce errors or performance issues in production.
Best Practices for Applying Migrations:
Create Incremental Migrations: Create a new migration for each set of changes. This makes it easier to track and manage changes.
Add-Migration AddEmailToCustomer
Review and Test Migrations: Review the generated migration code to ensure it accurately reflects the intended changes. Test the migration in a development or staging environment.
Update-Database
Deploy to Production: Once validated, apply the migration to the production database.
Update-Database -Environment Production
Example: Incremental Migration
// Adding a new field to the Customer entity
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string PhoneNumber { get; set; } // New field
}
// Creating and applying the migration
Add-Migration AddPhoneNumberToCustomer
Update-Database
Handling Migrations in a Staging Environment
- Set Up a Staging Environment: Configure a staging environment that mirrors your production setup.
- Apply Migrations in Staging: Apply the migrations to the staging database and run your tests to ensure everything works as expected.shellCopy code
Update-Database -Environment Staging
Update-Database -Environment Staging
3. Automate Testing: Automate your testing process to validate schema changes, ensuring that they do not break existing functionality.
Example: Automated Testing Script
public class MigrationTests
{
private readonly MyDbContext _context;
public MigrationTests()
{
var options = new DbContextOptionsBuilder<MyDbContext>()
.UseInMemoryDatabase(databaseName: "TestDatabase")
.Options;
_context = new MyDbContext(options);
}
[Fact]
public void CanAddCustomerWithPhoneNumber()
{
var customer = new Customer
{
Name = "John Doe",
Email = "john.doe@example.com",
PhoneNumber = "123-456-7890"
};
_context.Customers.Add(customer);
_context.SaveChanges();
var retrievedCustomer = _context.Customers.FirstOrDefault(c => c.Email == "john.doe@example.com");
Assert.NotNull(retrievedCustomer);
Assert.Equal("123-456-7890", retrievedCustomer.PhoneNumber);
}
}
Performance Profiling and Tuning
Optimizing the performance of your database access layer is crucial for ensuring that your application runs smoothly and efficiently. Here are some best practices for performance profiling and tuning in Entity Framework Core (EF Core), along with detailed explanations and code samples.
1. Use SQL Profiler or Similar Tools to Monitor and Analyze Generated SQL Queries
Monitoring and analyzing the SQL queries generated by EF Core can help identify performance bottlenecks and areas for improvement. Tools like SQL Profiler, EF Core logging, and other database monitoring tools can be very helpful.
Using EF Core Logging:
EF Core provides built-in logging capabilities that can be used to log the SQL queries generated by your application.
public class MyDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder
.UseSqlServer("YourConnectionString")
.LogTo(Console.WriteLine, new[] { DbLoggerCategory.Database.Command.Name }, LogLevel.Information)
.EnableSensitiveDataLogging();
}
}
// Example usage
var products = await _context.Products.ToListAsync();
Using SQL Profiler:
SQL Server Profiler is a tool provided by Microsoft for monitoring and analyzing SQL Server events, including the queries generated by your application.
- Start SQL Server Profiler: Open SQL Server Management Studio (SSMS), go to the Tools menu, and select SQL Server Profiler.
- Create a New Trace: Connect to your database server, and create a new trace. Select the events you want to monitor, such as SQL:BatchCompleted and SQL:BatchStarting.
- Run the Trace: Run your application and perform the operations you want to profile. SQL Server Profiler will capture the SQL queries and display them in real-time.
- Analyze the Results: Review the captured queries to identify slow or inefficient queries. Look for patterns such as long execution times, frequent executions, and missing indexes.
2. Optimize Database Schema and Indexes Based on Query Patterns
Analyzing the SQL queries generated by your application can help identify areas where your database schema and indexes can be optimized.
Adding Indexes:
Indexes can significantly improve query performance by allowing the database to quickly locate rows matching the query criteria.
// Define an index using Fluent API
public class MyDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Product>()
.HasIndex(p => p.Name)
.HasDatabaseName("IX_Product_Name");
}
}
// Example query that benefits from the index
var productsByName = await _context.Products
.Where(p => p.Name.Contains("EF Core"))
.ToListAsync();
Optimizing Schema:
Reviewing your database schema and making necessary adjustments can also improve performance. For example, you might need to normalize or denormalize tables based on your query patterns.
// Example of schema optimization by splitting a table into two related tables
public class Product
{
public int ProductId { get; set; }
public string Name { get; set; }
public ProductDetail ProductDetail { get; set; }
}
public class ProductDetail
{
public int ProductDetailId { get; set; }
public int ProductId { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
}
// Define the relationship using Fluent API
public class MyDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
public DbSet<ProductDetail> ProductDetails { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Product>()
.HasOne(p => p.ProductDetail)
.WithOne(pd => pd.Product)
.HasForeignKey<ProductDetail>(pd => pd.ProductId);
}
}
3. Implement Caching Strategies for Frequently Accessed Data to Reduce Database Load
Caching frequently accessed data can significantly reduce the load on your database and improve the performance of your application. There are several caching strategies you can use, including in-memory caching, distributed caching, and third-party caching solutions.
In-Memory Caching:
In-memory caching stores frequently accessed data in memory, making it available for quick retrieval.
// Register memory cache in the Startup class
public void ConfigureServices(IServiceCollection services)
{
services.AddMemoryCache();
services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
services.AddControllers();
}
// Use memory cache in a service
public class ProductService
{
private readonly MyDbContext _context;
private readonly IMemoryCache _cache;
public ProductService(MyDbContext context, IMemoryCache cache)
{
_context = context;
_cache = cache;
}
public async Task<List<Product>> GetProductsAsync()
{
const string cacheKey = "productsList";
if (!_cache.TryGetValue(cacheKey, out List<Product> products))
{
products = await _context.Products.ToListAsync();
var cacheEntryOptions = new MemoryCacheEntryOptions
{
AbsoluteExpirationRelativeToNow = TimeSpan.FromMinutes(10),
SlidingExpiration = TimeSpan.FromMinutes(2)
};
_cache.Set(cacheKey, products, cacheEntryOptions);
}
return products;
}
}
// Example usage
var productService = new ProductService(_context, _cache);
var products = await productService.GetProductsAsync();
Distributed Caching:
Distributed caching stores cached data in a distributed cache, such as Redis, which can be shared across multiple application instances.
// Register distributed cache (e.g., Redis) in the Startup class
public void ConfigureServices(IServiceCollection services)
{
services.AddStackExchangeRedisCache(options =>
{
options.Configuration = Configuration.GetConnectionString("RedisConnection");
options.InstanceName = "SampleInstance";
});
services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
services.AddControllers();
}
// Use distributed cache in a service
public class ProductService
{
private readonly MyDbContext _context;
private readonly IDistributedCache _cache;
public ProductService(MyDbContext context, IDistributedCache cache)
{
_context = context;
_cache = cache;
}
public async Task<List<Product>> GetProductsAsync()
{
const string cacheKey = "productsList";
var cachedProducts = await _cache.GetStringAsync(cacheKey);
if (!string.IsNullOrEmpty(cachedProducts))
{
return JsonSerializer.Deserialize<List<Product>>(cachedProducts);
}
var products = await _context.Products.ToListAsync();
var cacheEntryOptions = new DistributedCacheEntryOptions
{
AbsoluteExpirationRelativeToNow = TimeSpan.FromMinutes(10),
SlidingExpiration = TimeSpan.FromMinutes(2)
};
await _cache.SetStringAsync(cacheKey, JsonSerializer.Serialize(products), cacheEntryOptions);
return products;
}
}
// Example usage
var productService = new ProductService(_context, _cache);
var products = await productService.GetProductsAsync();
Dealing with Complex Queries In Entity Framework Core
Handling complex queries effectively is essential for maintaining both the performance and maintainability of your application. Here are some best practices for dealing with complex queries in Entity Framework Core (EF Core), along with detailed explanations and code samples.
1. Break Down Complex LINQ Queries into Smaller, Manageable Parts
Breaking down complex custom LINQ queries into smaller parts makes them more readable and easier to maintain. This approach also helps in debugging and optimizing individual parts of the query.
Example: Breaking Down a Complex Query
Suppose you have a complex query that filters products based on various criteria and then projects the results into a specific shape. Here’s how you can break it down:
// Step 1: Base query with initial filters
var baseQuery = _context.Products
.Where(p => p.IsActive && p.Price > 0);
// Step 2: Apply additional filters
var filteredQuery = baseQuery
.Where(p => p.Category == "Electronics" && p.Stock > 10);
// Step 3: Select specific columns or project to a new shape
var projectedQuery = filteredQuery
.Select(p => new
{
p.Id,
p.Name,
p.Price,
CategoryName = p.Category.Name
});
// Step 4: Execute the query
var result = await projectedQuery.ToListAsync();
Breaking down the query into smaller steps makes it easier to understand and maintain. Each step can be optimized independently if needed.
2. Use Raw SQL Queries for Complex Scenarios Where LINQ May Be Inefficient
Sometimes, LINQ queries can become too complex or inefficient. In such cases, using raw SQL queries can be more efficient and provide better performance.
Example: Using Raw SQL Queries
EF Core allows you to execute raw SQL queries directly against the database. This can be useful for complex queries or queries that require specific SQL features.
// Example of a raw SQL query
var sql = @"
SELECT p.Id, p.Name, p.Price, c.Name AS CategoryName
FROM Products p
INNER JOIN Categories c ON p.CategoryId = c.Id
WHERE p.IsActive = 1 AND p.Price > 0 AND c.Name = 'Electronics' AND p.Stock > 10";
var result = await _context.Products
.FromSqlRaw(sql)
.Select(p => new
{
p.Id,
p.Name,
p.Price,
CategoryName = p.Category.Name
})
.ToListAsync();
Using raw SQL queries can be more efficient in certain scenarios, but it’s important to ensure that the queries are safe from SQL injection attacks by using parameterized queries.
Example: Parameterized Raw SQL Query
var category = "Electronics";
var sql = @"
SELECT p.Id, p.Name, p.Price, c.Name AS CategoryName
FROM Products p
INNER JOIN Categories c ON p.CategoryId = c.Id
WHERE p.IsActive = 1 AND p.Price > 0 AND c.Name = {0} AND p.Stock > 10";
var result = await _context.Products
.FromSqlRaw(sql, category)
.Select(p => new
{
p.Id,
p.Name,
p.Price,
CategoryName = p.Category.Name
})
.ToListAsync();
3. Consider Stored Procedures for Highly Complex or Performance-Critical Operations
Stored procedures can be beneficial for highly complex queries or performance-critical operations. They allow you to encapsulate complex logic in the database, which can improve performance and reduce the amount of data transferred between the application and the database.
Example: Defining and Using Stored Procedures
Create a Stored Procedure in the Database:
CREATE PROCEDURE GetActiveProductsByCategory
@CategoryName NVARCHAR(50)
AS
BEGIN
SELECT p.Id, p.Name, p.Price, c.Name AS CategoryName
FROM Products p
INNER JOIN Categories c ON p.CategoryId = c.Id
WHERE p.IsActive = 1 AND p.Price > 0 AND c.Name = @CategoryName AND p.Stock > 10
END
Call the Stored Procedure from EF Core:
// Define a class to hold the results
public class ProductDto
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public string CategoryName { get; set; }
}
// Call the stored procedure
var categoryName = "Electronics";
var result = await _context.ProductDtos
.FromSqlRaw("EXEC GetActiveProductsByCategory @CategoryName = {0}", categoryName)
.ToListAsync();
Using the Result in the Application:
public async Task<List<ProductDto>> GetProductsByCategoryAsync(string categoryName)
{
return await _context.ProductDtos
.FromSqlRaw("EXEC GetActiveProductsByCategory @CategoryName = {0}", categoryName)
.ToListAsync();
}
// Usage
var products = await GetProductsByCategoryAsync("Electronics");
By following these practices for dealing with complex queries, you can improve the readability, maintainability, and performance of your EF Core queries. Breaking down complex queries, using raw SQL where necessary, and leveraging stored procedures for performance-critical operations will help you manage and optimize your data access layer effectively. Task Parallel Library is used for performance gain in the above code.
Error Handling and Logging In Entity Framework Core
Implementing robust error handling and logging in your Entity Framework Core (EF Core) applications is crucial for diagnosing issues, maintaining application health, and ensuring a smooth user experience. Here are some best practices for error handling and logging, along with detailed explanations and code samples.
1. Implement Robust Error Handling with Try-Catch Blocks Around Database Operations
Using try-catch blocks around your database operations helps to gracefully handle exceptions and provide meaningful error messages to users. This practice ensures that your application can recover from errors and maintain its stability.
Example: Basic Error Handling with Try-Catch
public async Task<List<Product>> GetProductsAsync()
{
try
{
return await _context.Products.ToListAsync();
}
catch (Exception ex)
{
// Handle the exception (e.g., log the error, return a default value, etc.)
Console.WriteLine($"An error occurred: {ex.Message}");
return new List<Product>();
}
}
Example: Detailed Error Handling
For more detailed error handling, you can catch specific types of exceptions and handle them accordingly.
public async Task<Product> GetProductByIdAsync(int id)
{
try
{
var product = await _context.Products.FindAsync(id);
if (product == null)
{
throw new KeyNotFoundException($"Product with ID {id} not found.");
}
return product;
}
catch (KeyNotFoundException ex)
{
// Handle not found exception
Console.WriteLine(ex.Message);
return null;
}
catch (DbUpdateException ex)
{
// Handle database update exception
Console.WriteLine($"A database update error occurred: {ex.Message}");
return null;
}
catch (Exception ex)
{
// Handle all other exceptions
Console.WriteLine($"An unexpected error occurred: {ex.Message}");
return null;
}
}
2. Log SQL Queries and Exceptions for Diagnostics and Monitoring Purposes
Logging SQL queries and exceptions is essential for diagnosing issues, monitoring application performance, and understanding the behavior of your application. EF Core provides built-in logging capabilities, and you can use various logging frameworks such as Serilog, NLog, or the built-in .NET Core logging.
Example: Logging SQL Queries
To log SQL queries, you can configure EF Core to use a logger.
public class MyDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder
.UseSqlServer("YourConnectionString")
.LogTo(Console.WriteLine, new[] { DbLoggerCategory.Database.Command.Name }, LogLevel.Information)
.EnableSensitiveDataLogging();
}
}
// Example usage
var products = await _context.Products.ToListAsync();
Example: Using a Logging Framework (Serilog)
Install Serilog: Install Serilog and the required packages via NuGet:
dotnet add package Serilog.AspNetCore
dotnet add package Serilog.Sinks.Console
dotnet add package Serilog.Sinks.File
Configure Serilog in Program.cs:
using Serilog;
var builder = WebApplication.CreateBuilder(args);
// Configure Serilog
Log.Logger = new LoggerConfiguration()
.WriteTo.Console()
.WriteTo.File("logs/myapp.txt", rollingInterval: RollingInterval.Day)
.CreateLogger();
builder.Host.UseSerilog();
builder.Services.AddDbContext<MyDbContext>(options =>
options.UseSqlServer(builder.Configuration.GetConnectionString("DefaultConnection"))
.LogTo(Log.Information, new[] { DbLoggerCategory.Database.Command.Name }, LogLevel.Information)
.EnableSensitiveDataLogging());
var app = builder.Build();
app.MapGet("/", async (MyDbContext context) =>
{
var products = await context.Products.ToListAsync();
return Results.Ok(products);
});
app.Run();
Log Exceptions in Services:
public class ProductService
{
private readonly MyDbContext _context;
private readonly ILogger<ProductService> _logger;
public ProductService(MyDbContext context, ILogger<ProductService> logger)
{
_context = context;
_logger = logger;
}
public async Task<List<Product>> GetProductsAsync()
{
try
{
return await _context.Products.ToListAsync();
}
catch (Exception ex)
{
_logger.LogError(ex, "An error occurred while retrieving products.");
return new List<Product>();
}
}
public async Task<Product> GetProductByIdAsync(int id)
{
try
{
var product = await _context.Products.FindAsync(id);
if (product == null)
{
_logger.LogWarning("Product with ID {ProductId} not found.", id);
throw new KeyNotFoundException($"Product with ID {id} not found.");
}
return product;
}
catch (KeyNotFoundException ex)
{
_logger.LogWarning(ex, ex.Message);
return null;
}
catch (DbUpdateException ex)
{
_logger.LogError(ex, "A database update error occurred while retrieving product with ID {ProductId}.", id);
return null;
}
catch (Exception ex)
{
_logger.LogError(ex, "An unexpected error occurred while retrieving product with ID {ProductId}.", id);
return null;
}
}
}
Conclusion
This article discusses best practices for data access optimization within Entity Framework Core. Techniques including DbContext configuration correctly, query performance optimization, relationship and navigation management, migration and schema change management, and error handling and logging are discussed. Additionally we covered security best practices to help safeguard your application against attacks.
Constant monitoring and tuning of your EF Core data access layer is vital for stability and performance. Profile your database activity frequently, test schema and queries, and also consider caching where needed.
Do not be scared to try new strategies to customize for your application. In case you require extra abilities, Hire ASP.NET Core developers with EF Core experience to carry out these best practices.
Additional Resources
To further enhance your understanding and expertise in Entity Framework Core (EF Core) and ensure you are applying the best practices effectively, here are some valuable additional resources: Entity Framework Core Documentation
- Stack Overflow: A great place to ask questions and get answers from the community. You can search for EF Core-related questions or post your own.
- Microsoft Q&A: A platform provided by Microsoft for asking technical questions and receiving answers from the community and Microsoft experts.
Step into the future with WireFuture at your side. Our developers harness the latest technologies to deliver solutions that are agile, robust, and ready to make an impact in the digital world.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.