How to Write Test Cases in Angular with Karma and Jasmine
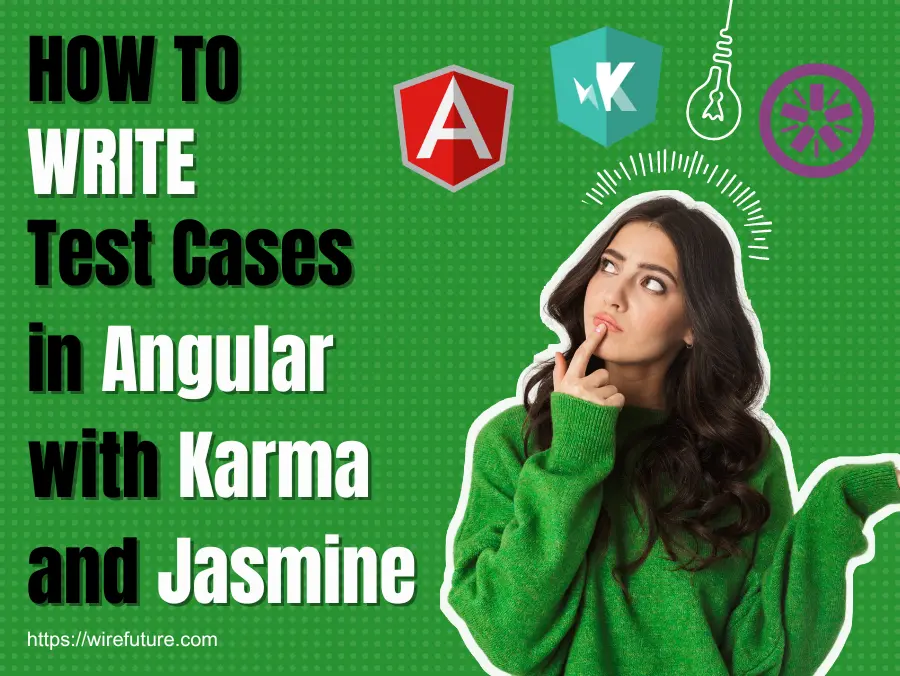
Join us in this blog post as we delve into the art of writing Test Cases in Angular, using the powerful duo of Karma and Jasmine to ensure your code stands the test of time. Ensuring your code’s reliability and robustness is of paramount importance in today’s rapidly evolving development environment. This is where Karma and Jasmine comes into play, offering you a smooth integration that will make your testing process much more efficient. This blog post is designed for developers who want to increase their test strategies to new heights by unravelling the mysteries of how Karma and Jasmine can create efficient testing cases.
The journey into automated testing can be both perplexing and daunting. However, this is a key step in ensuring the security of our code base. Karma, as a test runner, orchestrates the execution of written tests with different frameworks and when combined with Jasmine’s behavior driven development framework for testing JavaScript, it will become an extremely powerful tool to be used by any developer. This introduction will guide you through the fundamentals of leveraging Karma and Jasmine, ensuring that your tests not only run efficiently across multiple browsers but also contribute significantly to your project’s reliability.
You will gain insight into the design of a robust testing environment that embraces complexity and diversity in test situations. We want to demystify the writing of test cases that are not only comprehensive, but also maintain a high degree of creativity and adaptability. This blog post is your first step towards mastering the art of writing test cases in Angular, designed to inspire confidence in your code through a meticulously crafted testing regimen. So, let’s get started.
Table of Contents
- Writing Test Cases In Angular with Karma
- Writing Test Cases In Angular with Jasmine
- Running Your Test Cases In Angular with Karma
- Running Tests in Different Environments
- Debugging Tests
- Continuous Integration (CI)
- FAQ (Frequently Asked Questions)
- Conclusion
Writing Test Cases In Angular with Karma
Let’s delve into some code examples to illustrate how to write test cases in Angular with Karma, providing a practical guide to getting started.
After creating a new Angular project using the Angular CLI with the command:
ng new my-awesome-app
You are presented with a project structure including testing capability outside of the box. Both Jasmine and Karma are configured with the Angular CLI to write tests and execute them. For example, a karma.conf.js file is automatically created in your project’s root directory that contains the necessary configuration to run tests on Karma. A typical karma.conf.js file would look like this:
// Karma configuration file
module.exports = function(config) {
config.set({
// basePath: Specifies the base path that will be used to resolve all patterns (e.g., files, exclude).
basePath: '',
// frameworks: Specifies the testing frameworks you want to use. Typically, you would use 'jasmine' for Angular projects.
frameworks: ['jasmine', '@angular-devkit/build-angular'],
// plugins: Lists the Karma plugins to use. These usually include frameworks, launchers, and reporters.
plugins: [
require('karma-jasmine'),
require('karma-chrome-launcher'),
require('karma-jasmine-html-reporter'),
require('@angular-devkit/build-angular/plugins/karma')
],
// client: Configuration passed to the testing framework. Useful for configuring Jasmine's behavior in tests.
client: {
clearContext: false // Leave Jasmine Spec Runner output visible in the browser.
},
// jasmineHtmlReporter: Configuration for the jasmine-html-reporter plugin.
jasmineHtmlReporter: {
suppressAll: true // Optionally, remove the duplicated failures reported in the HTML report.
},
// reporters: Specifies what type of reporting should be generated by Karma. Common reporters include 'progress' and 'kjhtml'.
reporters: ['progress', 'kjhtml'],
// port: The port number Karma will use to launch the web server.
port: 9876,
// colors: Enable or disable colors in the output (reporters and logs).
colors: true,
// logLevel: Level of logging. Possible values are config.LOG_DISABLE, config.LOG_ERROR, config.LOG_WARN, config.LOG_INFO, config.LOG_DEBUG.
logLevel: config.LOG_INFO,
// autoWatch: Enable or disable watching files and executing the tests whenever one of these files changes.
autoWatch: true,
// browsers: Specifies the browsers to launch for testing. Chrome is typically used for development and ChromeHeadless for CI.
browsers: ['Chrome'],
// singleRun: If true, Karma will start and capture all configured browsers, run tests, and then exit with an exit code of 0 or 1 depending on whether all tests passed successfully.
singleRun: false,
// concurrency: How many browser instances should be started simultaneously. Infinity means unlimited.
concurrency: Infinity,
// customLaunchers: Allows defining custom browser configurations. Useful for setting up browsers for CI environments.
customLaunchers: {
ChromeHeadlessCI: {
base: 'ChromeHeadless',
flags: ['--no-sandbox', '--disable-gpu']
}
},
// restartOnFileChange: Specifies whether or not Karma should restart browsers if the files change.
restartOnFileChange: true
});
};
This configuration specifies the use of Jasmine as the testing framework and Chrome as the browser to run tests. Various plugins integrating Jasmine and Karma with Angular’s build tools are also part of this package.
For writing tests, Angular CLI generates a .spec.ts
file for each component, service, or class you create. These spec files are where you’ll write your tests using Jasmine’s syntax. For instance, when you generate a new component:
ng generate component my-component
A corresponding test file my-component.component.spec.ts
is created with a basic test suite:
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { MyComponent } from './my-component.component';
describe('MyComponent', () => {
let component: MyComponent;
let fixture: ComponentFixture<MyComponent>;
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [ MyComponent ]
})
.compileComponents();
});
beforeEach(() => {
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
This code snippet demonstrates how to set up and tear down each test case. The beforeEach
method is used to configure the testing module and create an instance of the component before each test. The it
function then defines a single test, with expect
statements to assert that the component initializes correctly.
Here is a more complex example that demonstrate how you would test your Login component by importing the necessary service in your test file:
// Import necessary Angular testing utilities and the component to be tested
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { LoginComponent } from './login.component';
import { FormsModule, ReactiveFormsModule } from '@angular/forms'; // Needed for forms
import { AuthService } from '../services/auth.service'; // Mocked authentication service
import { By } from '@angular/platform-browser'; // Used for querying DOM elements
// Create a mock AuthService with a spy on its login method
class MockAuthService {
login = jasmine.createSpy('login');
}
describe('LoginComponent', () => {
let component: LoginComponent;
let fixture: ComponentFixture<LoginComponent>;
let authService: AuthService; // To hold our service instance
beforeEach(async () => {
// Configure the testing module to setup the test environment
await TestBed.configureTestingModule({
declarations: [ LoginComponent ],
imports: [FormsModule, ReactiveFormsModule], // Import necessary modules
// Provide the mock service instead of the real AuthService
providers: [{ provide: AuthService, useClass: MockAuthService }]
})
.compileComponents(); // Compile the component's template and styles
});
beforeEach(() => {
// Create an instance of the component and the fixture
fixture = TestBed.createComponent(LoginComponent);
component = fixture.componentInstance;
fixture.detectChanges(); // Trigger initial data binding
// Retrieve the injected AuthService instance
authService = TestBed.inject(AuthService);
});
it('should create', () => {
// Verify that the component instance was created
expect(component).toBeTruthy();
});
it('should call AuthService.login on form submit', () => {
// Fill out the login form
component.loginForm.controls['email'].setValue('test@example.com');
component.loginForm.controls['password'].setValue('password123');
// Find the form element and trigger a submit event
const form = fixture.debugElement.query(By.css('form')).nativeElement;
form.dispatchEvent(new Event('submit'));
// Expect the AuthService login method to have been called with the form values
expect(authService.login).toHaveBeenCalledWith('test@example.com', 'password123');
});
it('should display an error message for invalid credentials', () => {
// Simulate the form submission with invalid credentials
component.loginForm.controls['email'].setValue('wrong@example.com');
component.loginForm.controls['password'].setValue('wrongPassword');
component.onSubmit(); // Directly call the submit handler for simplicity
// Simulate the AuthService returning an error
authService.login.and.returnValue(Promise.reject('Invalid credentials'));
// Manually trigger change detection to update the view
fixture.detectChanges();
// After async operations, check for the error message in the template
fixture.whenStable().then(() => {
const errorMessage = fixture.debugElement.query(By.css('.error-message')).nativeElement;
expect(errorMessage.innerText).toContain('Invalid credentials');
});
});
// Add more tests here to cover other functionalities and edge cases
});
It is simplicity and the ability of developers to write comprehensive tests that make this setup so attractive. For developers, the Angular CLI boilerplate code provides a fast way to get started by writing tests that focus on checking their application’s functionality rather than worrying about details of configuration. This foundational setup is the first step in building a robust testing strategy that ensures the quality and integrity of your Angular applications.
Writing Test Cases In Angular with Jasmine
The writing of test cases in Angular with Jasmine involves understanding its syntax and conventions, which are intended to make the tests easy to write, read or maintain. Jasmine is a behavior-driven development framework for JavaScript testing that provides a rich set of features to describe and test your application’s behavior in a readable format. Let’s dive into the details of writing test cases with Jasmine, accompanied by practical code examples.
Basic Structure
A Jasmine test suite begins with the describe
function, which defines a block of related tests. Inside this block, you use the it
function to define individual test cases. Each it
block represents a single unit test.
describe('MyComponent', () => {
it('should initialize correctly', () => {
expect(true).toBe(true);
});
it('should perform some action', () => {
expect(someFunction()).toEqual('expected result');
});
});
Setup and Teardown
Jasmine provides beforeEach
and afterEach
functions for setup and teardown tasks that need to run before and after each test case, respectively. Similarly, beforeAll
and afterAll
can run once before and after all tests in a suite.
describe('MyComponent', () => {
let myComponent;
beforeAll(() => {
// This runs once before all tests in the block.
myComponent = new MyComponent();
});
beforeEach(() => {
// This runs before each test.
myComponent.reset();
});
afterEach(() => {
// This runs after each test.
myComponent.cleanup();
});
afterAll(() => {
// This runs once after all tests in the block.
myComponent.destroy();
});
it('test case 1', () => {
expect(myComponent.property).toEqual('expected value');
});
// Additional test cases...
});
Testing Asynchronous Code
Jasmine supports testing asynchronous code using done callback, async/await, or returning a promise in your test cases.
Using done Callback:
it('should load data asynchronously', (done) => {
fetchData((data) => {
expect(data).toBeDefined();
done();
});
});
Using async/await:
it('should load data asynchronously', async () => {
const data = await fetchData();
expect(data).toBeDefined();
});
Spies
Jasmine spies are a powerful feature for testing interactions with functions or methods. Spies can track if and how a function was called, mock functions, or replace them with fake implementations.
describe('MyService', () => {
let service;
let dependency;
beforeEach(() => {
dependency = { fetchData: () => {} };
spyOn(dependency, 'fetchData').and.returnValue('mock data');
service = new MyService(dependency);
});
it('should fetch data using the dependency', () => {
service.getData();
expect(dependency.fetchData).toHaveBeenCalled();
});
});
Matchers
Jasmine provides a rich set of matchers that allow you to test your code in a readable and expressive way. Matchers are used with expect
function to assert different conditions.
describe('Matchers example', () => {
it('offers various ways to test values', () => {
expect(2 + 2).toEqual(4);
expect('hello world').toContain('hello');
expect([1, 2, 3]).toContain(2);
expect(() => { throw new Error('error'); }).toThrow();
expect(null).toBeNull();
expect(true).toBeTrue();
});
});
Writing test cases with Jasmine involves utilizing its suite of features to describe and test the behavior of your application in a clear, expressive manner. Jasmine will equip you with the tools necessary to ensure that your application is functioning as expected, such as creating and destroying test environments, testing async code or spying for mockery. The examples presented demonstrate the flexibility and strength of this framework, which serves as a starting point for building complete tests suites for your Angular applications.
Running Your Test Cases In Angular with Karma
To initiate your test runs with Karma, you generally start by invoking the test script defined in your package.json file, which is typically set up by the Angular CLI during project creation.
"scripts": {
"test": "karma start ./karma.conf.js"
}
Running the test script is as simple as executing the following command in your terminal:
npm test
The Karma command will automatically open the specified browsers and run the test suites, according to the configuration in karma.conf.js.
Running Tests in Different Environments
You can run tests in multiple browsers and environments with the flexibility of Karma. You can test your application in environments as diverse as Chrome, Firefox, Safari and even headless browsers like ChromeHeadless for continuous integration pipelines by configuring the browsers and customLaunchers options of karma.conf.js.
browsers: ['Chrome', 'Firefox', 'Safari'],
customLaunchers: {
ChromeHeadlessCI: {
base: 'ChromeHeadless',
flags: ['--no-sandbox', '--disable-gpu']
}
},
Debugging Tests
Here’s a deeper dive into how to effectively debug your Angular tests, complete with code samples to guide you.
Launching Karma in Debug Mode
To start debugging your tests, you first need to run Karma in a mode that keeps the browser open, allowing you to interact with the test runner. This is typically achieved through the configuration in your karma.conf.js:
// karma.conf.js
autoWatch: true,
singleRun: false,
These settings will open the specified browser and keep it open while running the npm test to display the test results. Then, in the browser window where the tests are running, you can go to the Debug page provided by Karma, which is usually accessible by clicking on the “Debug” button.
Using Browser Developer Tools
Once in the debug page, you can use the browser’s developer tools to set breakpoints, inspect variables, and step through your test code just like you would with your application code. For example, if you have a test that’s failing:
describe('AppComponent', () => {
it('should add two numbers correctly', () => {
const result = addNumbers(2, 3);
expect(result).toBe(5);
});
});
And you suspect the issue lies within the addNumbers function, you can open the developer tools in your browser (usually with F12 or right-click → “Inspect”) and set a breakpoint inside the function or directly in your test file.
Inspecting Variables and Call Stack
You can hover over variables to see their current values or use the console to evaluate expressions based on the current scope while paused at a breakpoint. In particular, it will give you an indication of the state of your application at the time when a test is performed.
Watching Expressions and Variables
Most browser developer tools allow you to add expressions or variables to a “watch” list, which is incredibly helpful for monitoring changes to specific data throughout the execution of your test.
// Adding to watch in browser developer tools
addNumbers(2, 3)
Debugging Asynchronous Code
Debugging tests with asynchronous code would require additional steps, such as using async and await with breakpoints or placing console.log statements to understand the sequence of operations.
it('should fetch data asynchronously', async () => {
const data = await fetchData();
console.log(data); // Use breakpoint here
expect(data).toBeDefined();
});
Continuous Integration (CI)
Continuous integration is a practice in which developers regularly integrate code into a shared repository, usually several times a day. Let’s delve deeper into how CI can be effectively implemented with Karma tests in Angular projects.
Setting Up CI for Angular Projects
You must set up your project and CI server for automatic test execution in order to integrate Karma testing into a Continuous Integration pipeline. To support headless testing modes and configure the CI server to execute a test command in your build process, you must modify karma.conf.js file.
Configuring Karma for CI
Karma supports running tests in headless browsers, which are ideal for CI environments. You can configure this by adding a custom launcher in your karma.conf.js:
browsers: ['ChromeHeadless'],
customLaunchers: {
ChromeHeadlessCI: {
base: 'ChromeHeadless',
flags: ['--no-sandbox', '--disable-gpu']
}
},
singleRun: true,
This configuration will enable Karma to use Chrome in a headless mode for server environments that do not have the visual interface. The singleRun: true option ensures that Karma runs the tests once and then exits, which is essential for CI processes.
Integrating with CI ToolsThe key is to ensure that your CI configuration file runs the Angular test command, whether you are using Jenkins, Travis CI, GitHub Actions, or any other CI service. For example, a.travis.yml file for Travis CI projects could contain the following:
language: node_js
node_js:
- "12"
addons:
chrome: stable
script:
- ng test --no-watch --no-progress --browsers=ChromeHeadlessCI
This configuration sets up the necessary environment, including installing a stable version of Chrome for headless testing, and then executes the ng test command with flags tailored for CI testing.
Benefits of CI in Angular Projects
- Early Detection of Errors: Running tests in CI helps catch integration and regression errors early in the development cycle, reducing the cost and effort required to fix them.
- Automated Testing Workflow: Automates the testing process, ensuring that tests are run consistently and results are reported back to the team promptly.
- Improved Code Quality: Continuous testing encourages developers to write more thorough tests, leading to higher code quality and more stable releases.
- Streamlined Development Process: CI integrates testing into the development workflow, making it easier for teams to collaborate and merge changes confidently.
Integration of CI can significantly improve the development process. You will ensure that every code commit is automatically tested, reduces errors and improves code quality by setting up your project and CI environment correctly.
FAQ (Frequently Asked Questions)
Karma serves as a test runner that allows developers to execute JavaScript tests in real browsers, providing an environment close to the end user’s experience. It’s essential for ensuring your application performs consistently across various browsers and platforms.
Jasmine is a behavior-driven development framework for testing JavaScript code. It complements Karma by providing a structured, readable syntax for writing test cases, enabling developers to define expected behaviors in a user-friendly manner.
Yes, Karma and Jasmine are versatile tools that can be integrated into the testing suite of any JavaScript application, regardless of its complexity or the framework it’s built with.
The primary prerequisites include having Node.js installed on your system, as well as a basic understanding of your project’s structure and the JavaScript testing ecosystem.
Writing a test case in Jasmine involves using describe and it blocks to define test suites and test specifications, respectively. Within an it block, you can use expect statements to assert expected outcomes.
Karma allows you to configure multiple browsers in your karma.conf.js file. By specifying different browsers, you can automatically run your tests across those browsers to ensure broad compatibility.
Yes, Karma and Jasmine can be integrated with various CI systems. Karma’s flexibility allows it to be easily incorporated into CI pipelines, ensuring tests are automatically run with every code commit.
Common challenges include browser compatibility issues, asynchronous code testing, and setting up a testing environment. Overcoming these challenges involves configuring Karma to use appropriate browser launchers, utilizing Jasmine’s done callback for asynchronous tests, and following best practices for test environment setup.
The official documentation for both Karma and Jasmine provides comprehensive guides and API references. Additionally, community forums, tutorials, and online courses can offer further insights and practical examples to enhance your testing skills.
Conclusion
For developers who want to deliver high quality, robust and reliable web applications, the complexities of writing test cases in Angular applications using Jasmine and Karma are essential. We’ve examined the foundations of effective testing strategies, such as creating a test environment and adjusting Karma, to write tests cases with Jasmine and integrating those processes in Continuous Integration with CI workflows. By sticking to these practices, developers can ensure their applications perform as expected across different environments, thereby enhancing user satisfaction and trust in the product.
The importance of a qualified development team cannot be underestimated in organizations seeking to develop or improve their Angular applications. WireFuture is at the forefront of Angular development, offering expertise services covering all aspects of application development, including design, construction, testing and maintenance. With a team of experienced Angular developers, WireFuture is the ideal partner for businesses seeking build Angular development team to harness the power of Angular for their web development projects. Hire Angular developers from WireFuture and transform your development journey into a path of success.
Success in the digital age requires strategy, and that's WireFuture's forte. We engineer software solutions that align with your business goals, driving growth and innovation.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.