Implementing Azure Key Vault in C# for Secure Configuration
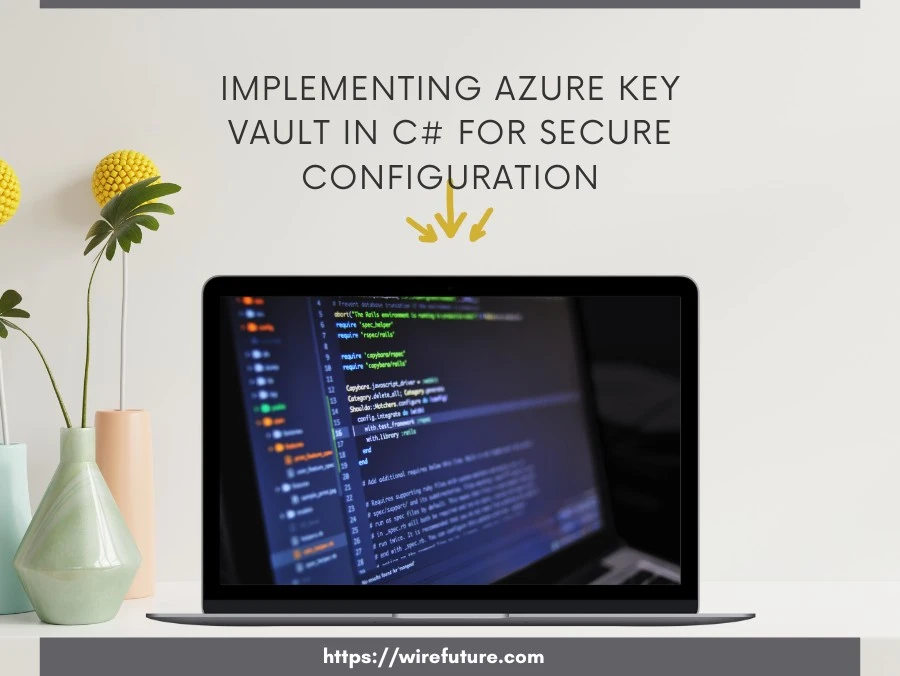
Securely managing application secrets and configurations is a common challenge in software development. Applications with hardcoding secrets in the codebase or storing them in plain text configurations expose them to security holes. Azure Key Vault provides a central location to store secrets, keys and certificates.
This article describes how to implement Azure Key Vault in C# using ASP.NET Core 8. How to create an Azure Key Vault, keep secrets and retrieve them through an ASP.NET Core application.
Why Use Azure Key Vault?
- Centralized Management: Azure Key Vault provides a centralized place to store secrets, keys, and certificates, making it easier to manage and rotate them.
- Enhanced Security: It offers robust security features, including access policies, role-based access control (RBAC), and auditing capabilities.
- Simplified Access: Integrates seamlessly with Azure services and applications, allowing secure access without exposing sensitive information in code.
- Compliance and Governance: Helps meet compliance requirements by providing detailed logs and security controls.
Creating an Azure Key Vault
Step 1: Create a Key Vault in Azure Portal
- Sign in to Azure Portal: Navigate to Azure Portal.
- Create a Key Vault:
- Go to “Create a resource”.
- Search for “Key Vault” and select it.
- Click “Create”.
- Configure Key Vault:
- Subscription: Select your Azure subscription.
- Resource Group: Choose an existing resource group or create a new one.
- Key Vault Name: Enter a unique name for the Key Vault.
- Region: Select the region closest to your application for lower latency.
- Pricing Tier: Choose the appropriate pricing tier (Standard or Premium).
- Review and Create: Click “Review + create”, then “Create” to deploy the Key Vault.
Step 2: Add Secrets to Key Vault
- Navigate to Key Vault: Once the Key Vault is created, go to it from the “All resources” or the “Resource Group” page.
- Add a Secret:
- Select “Secrets” from the left-hand menu.
- Click “Generate/Import”.
- Enter the secret name and value.
- Click “Create” to store the secret.
Accessing Secrets in C#
To access secrets stored in Azure Key Vault from a C# application, you’ll use the Azure SDK. Below are the steps to set up your C# project to interact with Azure Key Vault.
Step 1: Install Azure SDK
NuGet Package: Add the following NuGet packages to your project:
dotnet add package Azure.Identity
dotnet add package Azure.Security.KeyVault.Secrets
Step 2: Authenticate and Retrieve Secrets
1. Using DefaultAzureCredential: The DefaultAzureCredential
simplifies authentication by automatically using the best available credential.
using Azure.Identity;
using Azure.Security.KeyVault.Secrets;
var keyVaultName = "your-key-vault-name";
var kvUri = $"https://{keyVaultName}.vault.azure.net";
var client = new SecretClient(new Uri(kvUri), new DefaultAzureCredential());
KeyVaultSecret secret = client.GetSecret("YourSecretName");
Console.WriteLine($"Secret value: {secret.Value}");
2. Environment Setup: Ensure your environment is configured to provide the necessary credentials, such as setting environment variables or using managed identities for Azure resources.
Integrating Azure Key Vault with ASP.NET Core 8
Integrating Azure Key Vault with ASP.NET Core 8 allows for seamless access to secrets and configurations directly from your application settings.
Step 1: Update Configuration in appsettings.json
Add a placeholder for your secret in appsettings.json
:
{
"KeyVaultName": "your-key-vault-name",
"Secrets": {
"YourSecretName": ""
}
}
Step 2: Configure Key Vault in Program.cs
Update your Program.cs
to include Key Vault configuration:
using Azure.Extensions.AspNetCore.Configuration.Secrets;
using Azure.Identity;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.Hosting;
var builder = WebApplication.CreateBuilder(args);
var keyVaultName = builder.Configuration["KeyVaultName"];
var kvUri = $"https://{keyVaultName}.vault.azure.net";
// Add Azure Key Vault to configuration providers
builder.Configuration.AddAzureKeyVault(new Uri(kvUri), new DefaultAzureCredential());
var app = builder.Build();
// Remaining code...
Step 3: Use Secrets in Application
Retrieve secrets from configuration as you would with any other configuration value:
var secretValue = builder.Configuration["Secrets:YourSecretName"];
Console.WriteLine($"Retrieved secret value: {secretValue}");
Step 4: Handling Secret Changes
Azure Key Vault integration automatically handles changes in secrets without requiring redeployment. Your ASP.NET Core application will reflect updated secret values as soon as they are changed in Key Vault.
Benefits of Using Azure Key Vault with ASP.NET Core
- Improved Security: Secrets are stored securely and are never exposed in code or configuration files.
- Automatic Secret Management: Simplifies secret rotation and management, reducing the risk of outdated or compromised secrets.
- Seamless Integration: Works seamlessly with ASP.NET Core and other Azure services, providing a unified approach to security and configuration management.
- Compliance: Helps meet regulatory requirements by providing detailed logs and control over access to sensitive information.
Conclusion
Secure configuration management with Azure Key Vault in C# is an important step in making your applications secure. Sticking to the steps in this guide you can control and access your application secrets and configurations, embed them in your ASP.NET Core apps, and benefit from Azure Key Vault security features. Leveraging these techniques in your .NET development services improves security and also simplifies management of sensitive data, giving you a strong solution for clients who care about data protection and compliance.
WireFuture's team spans the globe, bringing diverse perspectives and skills to the table. This global expertise means your software is designed to compete—and win—on the world stage.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.