Introduction To Cross-Platform Apps with .NET MAUI in .NET 8
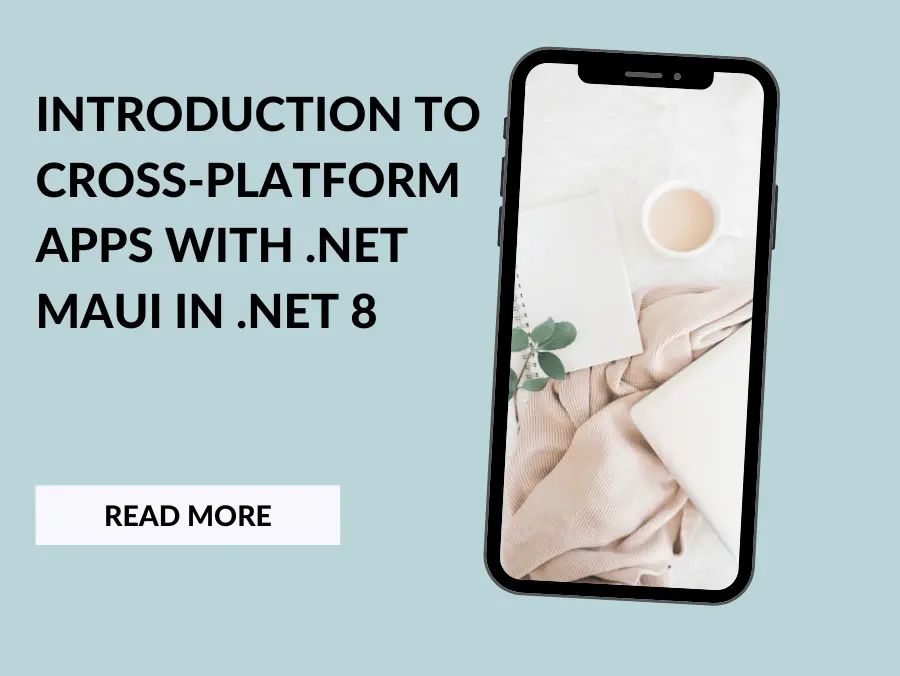
As software development expands, applications that run on multiple platforms with one codebase become more prevalent. Enter .NET Multi-platform App UI (MAUI): a framework that lets developers develop apps for Android, macOS, iOS, along with Windows from one codebase. With .NET 8, .NET MAUI delivers enhanced performance, improved tooling and more powerful features that make cross-platform development easier and faster than ever before.
In this article we’ll demonstrate the capability of .NET MAUI with a particular focus on .NET 8 and show you how to develop strong, cross platform applications with one codebase. We’ll cover the fundamental concepts, walk through setting up your development environment, and provide detailed code examples to help you start building your first .NET MAUI app.
For those interested in learning more about .NET development, check out our .NET Development blogs. Stay updated with the latest insights and best practices!
Table of Contents
- What is .NET MAUI in .NET 8?
- Key Features of .NET MAUI in .NET 8
- Setting Up Your .NET MAUI Development Environment
- Building a Simple .NET MAUI Application in .NET 8
- Leveraging Platform-Specific Code in .NET MAUI
- Integrating Blazor with .NET MAUI in .NET 8
- Advanced Features in .NET MAUI
- Conclusion
What is .NET MAUI in .NET 8?
.NET MAUI (Multi-platform App UI) is a framework that allows developers create native user interfaces for multiple platforms from one codebase. As the successor to Xamarin.Forms, .NET MAUI provides developers with one API that works on Android, iOS, Windows and macOS. With .NET 8, .NET MAUI has matured with new features and improvements to improve its capability and performance.
Key Features of .NET MAUI in .NET 8
.NET 8 has brought several advancements to .NET MAUI, making it an even more powerful tool for cross-platform development. Let’s explore some of the key features that make .NET MAUI stand out:
- Unified Project Structure: .NET MAUI in .NET 8 continues to use a single project structure that consolidates platform-specific code and resources, making it easier to manage and share code across different platforms.
- Enhanced Performance: With .NET 8, .NET MAUI benefits from runtime improvements that enhance the performance of applications, particularly in areas like startup time and memory usage.
- Advanced Native API Access: .NET 8 expands the capabilities of .NET MAUI by providing deeper access to native APIs across all supported platforms, allowing developers to create richer, more native experiences.
- Improved Hot Reload: The XAML and .NET Hot Reload features have been further refined in .NET 8, offering an even smoother development experience by allowing instant feedback on code changes without requiring a full rebuild or restart.
- MVU and Blazor Integration: .NET MAUI continues to support the Model-View-Update (MVU) pattern and tight integration with Blazor, enabling developers to build interactive UIs using a declarative approach while reusing Blazor components across web and native apps.
- Optimized for the Cloud: .NET 8 enhances .NET MAUI’s ability to integrate with cloud services, making it easier to build applications that leverage cloud-based APIs, databases, and authentication services.
Setting Up Your .NET MAUI Development Environment
To get started with .NET MAUI in .NET 8, you’ll need to set up your development environment. Here’s how you can do it:
- Install Visual Studio 2022: Make sure you have the latest version of Visual Studio 2022 installed, as it provides the best tooling support for .NET MAUI.
- Install .NET 8 SDK: Download and install the .NET 8 SDK from the official .NET website.
- Enable MAUI Workload: Open the Visual Studio Installer, select your Visual Studio instance, and modify it to include the “.NET Multi-platform App UI development” workload. This will ensure that you have all the necessary tools to work with .NET MAUI.
- Create a New MAUI Project: Launch Visual Studio, select “Create a new project,” and choose the “.NET MAUI App” template. This will create a cross-platform project that targets Android, iOS, macOS, and Windows from a single codebase.
Building a Simple .NET MAUI Application in .NET 8
Let’s walk through building a simple .NET MAUI application that runs on both mobile and desktop platforms. We’ll cover the basics of creating the UI and handling platform-specific code.
Step 1: Creating the Project
After setting up your development environment, the first step is to create a new .NET MAUI project. Open Visual Studio and follow these steps:
- Create a New Project: Select “Create a new project” from the Visual Studio start screen.
- Choose the MAUI App Template: Search for “MAUI” in the project templates, select “.NET MAUI App”, and click “Next”.
- Configure Your Project: Enter a name and location for your project, then click “Create”.
Visual Studio will generate a new .NET MAUI project with the following structure:
- Platforms: Contains platform-specific code for Android, iOS, macOS, and Windows.
- Resources: Includes shared resources like images, fonts, and styles.
- MainPage.xaml: The main UI file for your application.
- App.xaml: The global resource dictionary and application lifecycle management.
Step 2: Designing the UI
Let’s start by creating a simple UI in MainPage.xaml
that displays a welcome message and a button. When the button is clicked, the app will display a platform-specific message.
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp.MainPage">
<StackLayout Padding="20" VerticalOptions="Center">
<Label Text="Welcome to .NET MAUI!"
FontSize="24"
HorizontalOptions="Center" />
<Button Text="Click Me"
FontSize="18"
HorizontalOptions="Center"
Clicked="OnButtonClicked" />
</StackLayout>
</ContentPage>
Step 3: Handling Button Clicks
Next, we’ll add some code in the MainPage.xaml.cs
file to handle the button click event. The goal is to display a different message depending on the platform the app is running on.
using Microsoft.Maui.Controls;
using System;
namespace MauiApp
{
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
private void OnButtonClicked(object sender, EventArgs e)
{
string platformMessage = DeviceInfo.Platform switch
{
DevicePlatform.Android => "Hello from Android!",
DevicePlatform.iOS => "Hello from iOS!",
DevicePlatform.macOS => "Hello from macOS!",
DevicePlatform.WinUI => "Hello from Windows!",
_ => "Hello from .NET MAUI!"
};
DisplayAlert("Platform Message", platformMessage, "OK");
}
}
}
This simple switch expression uses the DeviceInfo.Platform
property to determine which platform the app is running on and displays an appropriate message.
Step 4: Running the Application
You can now run the application on different platforms directly from Visual Studio:
- Select the Target Platform: Use the drop-down menu in Visual Studio to select the platform you want to target (e.g., Android, iOS, Windows).
- Run the App: Click the “Run” button (or press
F5
) to build and deploy the application to the selected platform.
You should see a simple UI with a welcome message and a button. Clicking the button will display a platform-specific message, demonstrating how .NET MAUI allows you to target multiple platforms with the same codebase.
Leveraging Platform-Specific Code in .NET MAUI
While .NET MAUI provides a unified API for most common scenarios, there will be times when you need to implement platform-specific functionality. This can be achieved using platform-specific code, also known as partial classes or conditional compilation.
Example: Accessing Device-Specific Features
Suppose you want to access a device-specific feature, such as the battery level on Android and iOS. Here’s how you can do it using platform-specific code in .NET MAUI.
1. Create an Interface for Battery Level Access
First, define an interface in the shared project that will be implemented differently on each platform.
public interface IBatteryService
{
double GetBatteryLevel();
}
2. Implement the Interface on Android
In the Platforms/Android
folder, create a new class that implements the IBatteryService
interface.
using Android.OS;
using MauiApp;
[assembly: Dependency(typeof(BatteryService))]
namespace MauiApp.Platforms.Android
{
public class BatteryService : IBatteryService
{
public double GetBatteryLevel()
{
BatteryManager batteryManager = (BatteryManager)Android.App.Application.Context.GetSystemService(Android.Content.Context.BatteryService);
return batteryManager.GetIntProperty((int)BatteryProperty.Capacity) / 100.0;
}
}
}
3. Implement the Interface on iOS
Similarly, implement the interface in the Platforms/iOS
folder.
using UIKit;
using MauiApp;
[assembly: Dependency(typeof(BatteryService))]
namespace MauiApp.Platforms.iOS
{
public class BatteryService : IBatteryService
{
public double GetBatteryLevel()
{
UIDevice.CurrentDevice.BatteryMonitoringEnabled = true;
return UIDevice.CurrentDevice.BatteryLevel * 100;
}
}
}
Integrating Blazor with .NET MAUI in .NET 8
One of the most exciting features of .NET MAUI is its seamless integration with Blazor, enabling you to build interactive UIs using web technologies like HTML and CSS, and embed them within your native apps. This allows you to reuse existing Blazor components or create new ones that can run on both the web and native platforms.
Example: Embedding a Blazor Component in a MAUI App
Let’s walk through an example of how you can embed a Blazor component in a .NET MAUI application.
1. Create a Blazor Component
First, create a new Blazor component that will display a simple counter. In the Shared
folder, add a new Razor component named Counter.razor
.
<h3>Counter</h3>
<p>Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
}
2. Add BlazorWebView to MAUI Project
To use this Blazor component in your .NET MAUI app, add a BlazorWebView
control to your MainPage.xaml
.
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:blazor="clr-namespace:Microsoft.AspNetCore.Components.WebView.Maui;assembly=Microsoft.AspNetCore.Components.WebView.Maui"
x:Class="MauiApp.MainPage">
<StackLayout>
<Label Text="Welcome to .NET MAUI with Blazor!"
FontSize="24"
HorizontalOptions="Center" />
<blazor:BlazorWebView HostPage="wwwroot/index.html">
<blazor:BlazorWebView.RootComponents>
<blazor:RootComponent Selector="#app" ComponentType="{x:Type local:Counter}" />
</blazor:BlazorWebView.RootComponents>
</blazor:BlazorWebView>
</StackLayout>
</ContentPage>
3. Configure the Blazor WebView
In the MauiProgram.cs
file, register the Blazor services and set up the BlazorWebView
.
using Microsoft.Maui.Hosting;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.AspNetCore.Components.WebView.Maui;
public static class MauiProgram
{
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
});
builder.Services.AddMauiBlazorWebView();
#if DEBUG
builder.Services.AddBlazorWebViewDeveloperTools();
#endif
return builder.Build();
}
}
4. Run the Application
Now, when you run the application, you’ll see the Blazor component embedded within your .NET MAUI app, displaying a simple counter that increments every time you click the button. This demonstrates how easily Blazor components can be integrated into native applications using .NET MAUI.
Advanced Features in .NET MAUI
Beyond the basics, .NET MAUI in .NET 8 offers advanced features that can take your cross-platform applications to the next level.
1. Handling Device Orientation and Layouts
.NET MAUI provides a responsive design system that adapts to different screen sizes and orientations. You can use the VisualStateManager
to define different UI states based on device orientation.
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp.MainPage">
<StackLayout>
<VisualStateManager.VisualStateGroups>
<VisualStateGroup x:Name="OrientationStates">
<VisualState x:Name="Portrait">
<VisualState.Setters>
<Setter Property="Orientation" Value="Vertical" />
</VisualState.Setters>
</VisualState>
<VisualState x:Name="Landscape">
<VisualState.Setters>
<Setter Property="Orientation" Value="Horizontal" />
</VisualState.Setters>
</VisualState>
</VisualStateGroup>
</VisualStateManager.VisualStateGroups>
<Label Text="Responsive Layout" FontSize="24" HorizontalOptions="Center" />
<Button Text="Button 1" />
<Button Text="Button 2" />
</StackLayout>
</ContentPage>
This simple example changes the orientation of the StackLayout
based on whether the device is in portrait or landscape mode.
2. Using Custom Renderers for Platform-Specific Customization
While .NET MAUI abstracts most platform-specific details, there are times when you need to implement custom controls. You can do this by creating custom renderers.
For example, let’s say you want to create a custom renderer for a button on Android:
using Android.Content;
using Android.Graphics;
using Android.Widget;
using MauiApp;
using MauiApp.Platforms.Android;
using Microsoft.Maui.Controls.Compatibility;
using Microsoft.Maui.Controls.Compatibility.Platform.Android;
[assembly: ExportRenderer(typeof(CustomButton), typeof(CustomButtonRenderer))]
namespace MauiApp.Platforms.Android
{
public class CustomButtonRenderer : ButtonRenderer
{
public CustomButtonRenderer(Context context) : base(context)
{
}
protected override void OnElementChanged(ElementChangedEventArgs<Button> e)
{
base.OnElementChanged(e);
if (Control != null)
{
// Customizing the button
Control.SetBackgroundColor(Android.Graphics.Color.LightGreen);
Control.SetTextColor(Android.Graphics.Color.Black);
Control.SetTypeface(null, TypefaceStyle.Bold);
}
}
}
}
This custom renderer changes the appearance of a button on Android, giving it a light green background, black text, and bold font style.
Conclusion
.NET MAUI in.NET 8 provides developers with a consistent, cross-platform development framework. With features like a single project structure, improved native API access, and powerful integrations with Blazor. .NET MAUI makes it easier than ever to develop high-quality, responsive apps for mobile and desktop.
This article covered the basics of .NET MAUI, setting up your development environment, and building and customizing a simple cross-platform application. We also delved into advanced features like handling device orientation, using platform-specific code, and integrating Blazor components.
As you find out more about .NET MAUI, you will see it makes cross-platform development easy, and it lets you create richer, much more interactive apps that take full advantage of every platform. With the tools and knowledge in this guide, you are now ready to create your next cross-platform masterpiece with .NET MAUI in .NET 8, whether you are an individual developer or part of a .NET development company.
At WireFuture, we believe every idea has the potential to disrupt markets. Join us, and let's create software that speaks volumes, engages users, and drives growth.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.