Mastering KnockoutJS: A Comprehensive Guide for Dynamic Web Apps
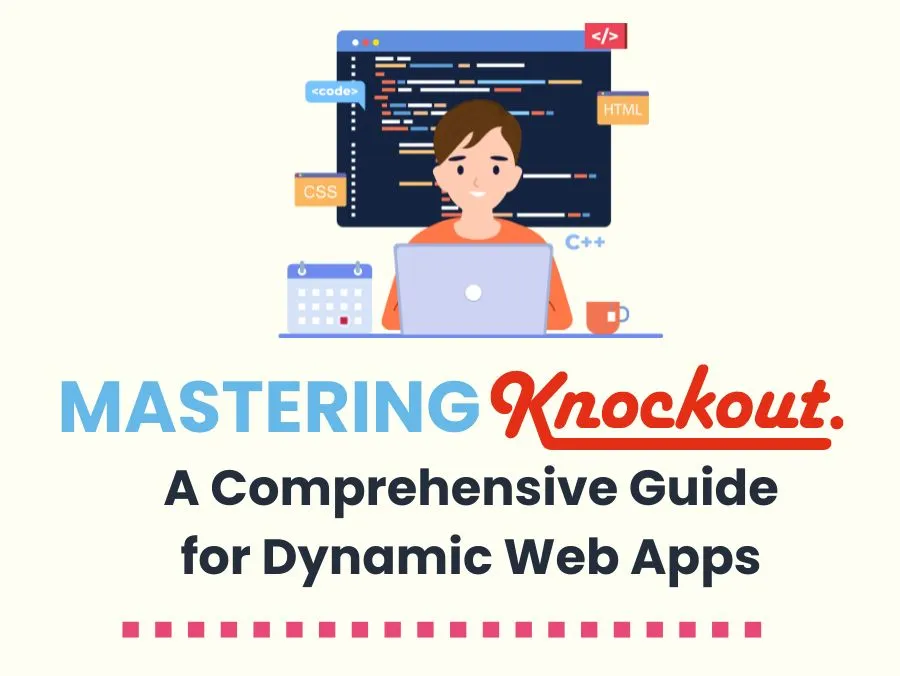
In the ever-evolving landscape of web development, where the demand for rich, interactive user interfaces meets the complexity of dynamic data models, one hero emerges to bridge the gap with elegance and efficiency: KnockoutJS. The way developers approach developing responsive and sophisticated web applications has been redefined by this powerful JavaScript library.
KnockoutJS provides a seamless connection between your data UI and application, ensuring that applications are not only scalable but also usable, by adopting the ModelViewModelVMML pattern. Understanding KnockoutJS’s capabilities can change the way you think about and build web applications, whether you are an experienced JavaScript developer or are just beginning to use the vast range of JavaScript frameworks.
We’re going to go through the basic concepts, benefits and extensive features of KnockoutJS in this comprehensive guide. We will examine KnockoutJS’s position as a testament to contemporary Web development practices, from the simplicity of observables to the fluid nature of computed functions and its intricate component life cycle.
We’re going to unravel KnockoutJS’s magic, complete with in depth examples, code samples, and best practices that will give you the knowledge to take full advantage of its potential in your next project. This is the final guide to mastering KnockoutJS.
- What is KnockoutJS?
- Why KnockoutJS over jQuery?
- Benefits of KnockoutJS
- Performance
- Setup, Downloading, and Installing
- Creating Observables and View Models
- Binding Observables and ViewModels to the UI
- Computed Observables
- Visible and Hidden Bindings
- Text and HTML Bindings
- CSS Binding
- For Each Loop
- Component Lifecycle
- Click, Event, Submit Binding
- Value, HasFocus, Data-Bind Syntax
- Creating Custom Binding Handlers in KnockoutJS
- Conclusion
What is KnockoutJS?
KnockoutJS is a standalone JavaScript implementation of the Model-View-ViewModel (MVVM) pattern with templates. If you are interested in exploring other frameworks we highly encourage you to read our article on best frontend frameworks. The main idea of KnockoutJS is to update your data model dynamically and take these changes into account in the UI without any effort at all. It’s as if you have a magical mirror in your UI, reflecting changes to the model at any moment.
Why KnockoutJS over jQuery?
jQuery is a wonderful tool to manipulate and manage events directly from the DOM. However, jQuery can lead to a lot of boilerplate code to manually synchronize your UI with your data model when developing applications with complex data models and dynamic UI updates. KnockoutJS provides a more complex approach to UI data binding by means of its MVVM pattern. This doesn’t just reduce the amount of code you write but also makes it more readable and maintainable by separating the concerns of data (Model), UI (View), and the logic that connects the two (ViewModel).
Benefits of KnockoutJS
Declaration Bindings: KnockoutJS is using HTML5 dataattribute bindings to connect the UI to the data model. That way, you don’t have to go into complex JavaScript code to understand and manage the relationship of your UI components with their source data.
Automatic UI refresh: If the data model changes, KnockoutJS will automatically refresh the UI. This twoway data binding ensures that your UI always reflects the model’s current state, without any further effort.
Dependency Tracking: KnockoutJS’s computed observables can automatically update themselves and the UI based on changes in other observables. This allows complex dependencies to be managed in your application state more easily.
Flexibility and Extensibility: KnockoutJS enables developers to build their own binding for specific needs, which makes it very flexible in terms of different projects’ requirements.
Performance
KnockoutJS has been designed with performance in mind. An efficient dependency tracking system is used to minimize unnecessary DOM updates through its observable and computed observable mechanisms. That means KnockoutJS will intelligently update only the parts of your UI that need to be changed based on Data Model updates, resulting in faster rendering times and a more pleasant user experience.
Setup, Downloading, and Installing
First, let’s start by adding KnockoutJS to your project:
<!-- Adding KnockoutJS via CDN -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/knockout/3.5.1/knockout-min.js"></script>
Creating Observables and View Models
What is an Observable?
An observable in KnockoutJS is essentially a JavaScript object that allows subscribers to be notified of changes. This type of object is designed to be reactive; KnockoutJS automatically propagates changes to the UI or any other dependent observables when its value changes, ensuring that the application’s data model and UI are always in sync. Observables are the core components of KnockoutJS, enabling twoway data binding, which makes the KnockoutJS library so powerful and efficient for dynamic web applications.
Creating an observable is straightforward:
var myObservable = ko.observable('Initial Value');
When the value of myObservable
changes, any part of your UI bound to this observable will automatically update to reflect the new value.
What is a ViewModel?
The ViewModel in KnockoutJS is a middleman between the model’s data and your UI view. This is where the UI data and behavior can be defined. The ViewModel will interact with the model, process data and present elements that a view may be bound to. It defines the application’s data model, converting the model properties into observables or computed observables, and defines the functions that the view can use, effectively decoupling the model from the view, and simplifying the management of the view.
Here’s a simple ViewModel:
function AppViewModel() {
this.firstName = ko.observable('John');
this.lastName = ko.observable('Doe');
this.fullName = ko.computed(function() {
return this.firstName() + " " + this.lastName();
}, this);
}
In this example, firstName
and lastName
are observables, while fullName
is a computed observable, which automatically updates its value when any of its dependencies (firstName
or lastName
) change.
Binding Observables and ViewModels to the UI
The real power of observables and ViewModels comes into play when you bind them to your HTML UI, enabling automatic updates and synchronization between the UI and your data model:
<p>First name: <strong data-bind="text: firstName"></strong></p>
<p>Last name: <strong data-bind="text: lastName"></strong></p>
<p>Full name: <strong data-bind="text: fullName"></strong></p>
<script type="text/javascript">
ko.applyBindings(new AppViewModel());
</script>
To demonstrate KnockoutJS’s dynamic capabilities, changes to the firstName or lastName of ViewModel will automatically update corresponding strong>] elements in HTML.
To fully exploit KnockoutJS’s full potential, it is essential to understand observables and view model. They are the foundation to build powerful, interactive web applications that allow for effective data management and user interface synchronization.
Computed Observables
Computed observables are functions that are automatically re-evaluated when observables they depend on change, perfect for calculating values on the fly.
function AppViewModel() {
this.firstName = ko.observable('Jane');
this.lastName = ko.observable('Doe');
this.fullName = ko.computed(function() {
return this.firstName() + " " + this.lastName();
}, this);
}
Visible and Hidden Bindings
Control the visibility of elements effortlessly with KnockoutJS’s visible and hidden bindings.
<!-- Toggles visibility based on the `shouldBeVisible` observable -->
<div data-bind="visible: shouldBeVisible">Visible when true</div>
Text and HTML Bindings
Easily bind the inner text or HTML of an element to your ViewModel.
<!-- Binding with `text` and `html` -->
<span data-bind="text: userName"></span>
<!-- Any HTML content bound will be rendered -->
<div data-bind="html: userBio"></div>
CSS Binding
Dynamically assign CSS classes based on your model’s data.
<!-- The `active` class is applied based on the `isActive` observable -->
<div data-bind="css: { active: isActive }">Active User</div>
For Each Loop
Iterate over arrays and render lists seamlessly.
<!-- Rendering a list of items using `foreach` -->
<ul data-bind="foreach: userList">
<li data-bind="text: $data"></li>
</ul>
function ViewModel() {
this.userList = ko.observableArray(['User 1', 'User 2', 'User 3']);
}
ko.applyBindings(new ViewModel());
Component Lifecycle
KnockoutJS components provide hooks for each stage of a component’s lifecycle, including when all the child components or descendants have been processed.
ko.components.register('my-component', {
viewModel: function(params) {
// Component logic
},
template: '<div>Component Content</div>',
synchronous: true
});
ko.bindingHandlers.myCustomHandler = {
init: function(element, valueAccessor, allBindings, viewModel, bindingContext) {
// Initialization code
},
update: function(element, valueAccessor, allBindings, viewModel, bindingContext) {
// Update logic
}
};
To listen for the childrenComplete
and descendantsComplete
events:
ko.bindingHandlers.childrenComplete = {
init: function(element, valueAccessor, allBindings, viewModel, bindingContext) {
// Code to run when all child components are complete
}
};
ko.bindingHandlers.descendantsComplete = {
init: function(element, valueAccessor, allBindings, viewModel, bindingContext) {
// Code to run when all descendant components are complete
}
};
Click, Event, Submit Binding
Handle user interactions with ease using KnockoutJS bindings for events.
<!-- Handling click events -->
<button data-bind="click: incrementCounter">Click me</button>
function ViewModel() {
this.counter = ko.observable(0);
this.incrementCounter = () => {
this.counter(this.counter() + 1);
};
}
ko.applyBindings(new ViewModel());
Value, HasFocus, Data-Bind Syntax
Manage form elements, focus, and apply complex bindings succinctly.
<!-- Binding `value` and `hasFocus` -->
<input data-bind="value: email, hasFocus: emailFocused" />
function ViewModel() {
this.email = ko.observable('');
this.emailFocused = ko.observable(false);
}
ko.applyBindings(new ViewModel());
Creating Custom Binding Handlers in KnockoutJS
KnockoutJS is flexible and extensible, in particular through custom binding handlers. You will be able to customize your own way of binding data to the DOM elements, enabling you to generate mutandisable and embedded DOM element behavior.
When you need to implement complex UI components or integrate third party libraries that require specific initialization or lifecycle management, custom binding handlers are particularly useful. You can keep your HTML clean and declarative while encapsulating the essential logic in a binding handler, by defining custom binding handlers.
Use the ko.bindingHandlers object to specify your custom binding’s name and create an init or update function when creating a custom binding handler. The init function is called when the binding has been applied to an element, perfect for onetime initialization. Whenever the bound observable changes, an update function is called that allows you to react to changing data.
Here’s a simple example of a custom binding handler that applies a jQuery UI datepicker to an input element:
ko.bindingHandlers.datepicker = {
init: function(element, valueAccessor) {
$(element).datepicker({
onSelect: function(date) {
var observable = valueAccessor();
observable(date);
}
});
},
update: function(element, valueAccessor) {
var value = ko.unwrap(valueAccessor());
$(element).datepicker("setDate", value);
}
};
In this example, the init function initializes the jQuery UI datepicker on the bound element and updates the observable with the selected date. The update function ensures that the datepicker’s display date remains in sync when the observable changes elsewhere in your application.
The creation of custom binding handlers in KnockoutJS opens up a world of possibilities, allowing you to create highly interactive and responsive web applications that stand out. This is a testament to KnockoutJS’s power and flexibility for developers who want to challenge the limits of what can be done with web UI development.
Conclusion
Through this expanded exploration of KnockoutJS, we’ve seen how it simplifies the creation of dynamic, responsive web applications by providing a robust set of tools for two-way data binding, automatic UI updates, and much more. This is a powerful library that will substantially enhance the quality and stability of your web projects once it’s mastered.
To provide cutting edge web solutions, hire KnockoutJS developers from WireFuture who specialize in developing backend applications using .Net, PHP, Python and uses modern JavaScript Library such as KnockoutJS. Our team of dedicated KnockoutJS developers are ready to bring your projects to life with the efficiency, scalability, and performance that KnockoutJS offers. Together, we can create something great.
Dream big, because at WireFuture, no vision is too ambitious. Our team is passionate about turning your software dreams into reality, with custom solutions that exceed expectations.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.