Mastering Lambda Expressions In C#: A Guide to Essentials
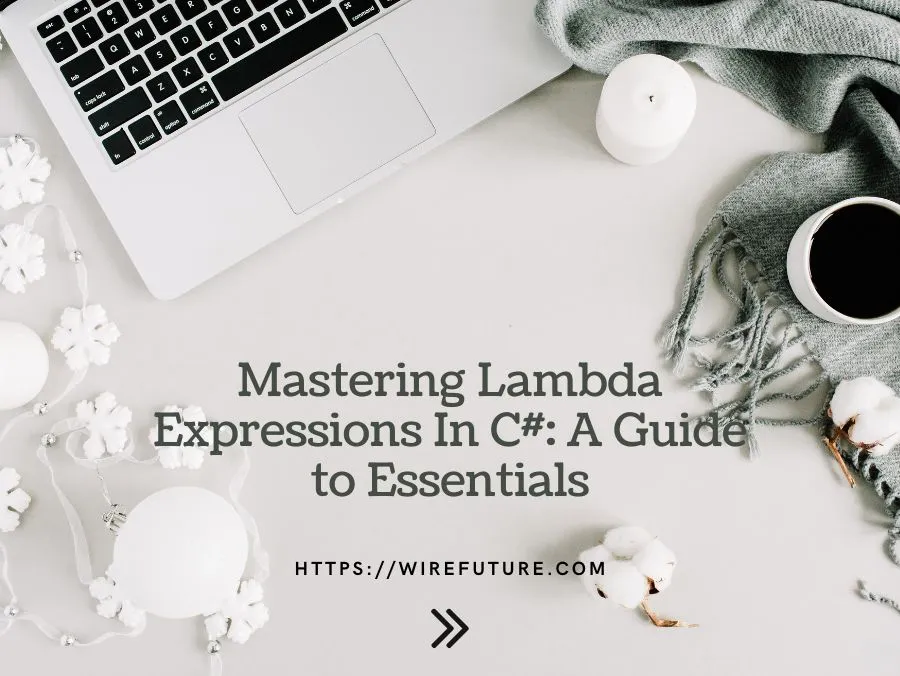
Modern programming has increasingly adopted lambda expressions as a basic structure for expressing functions or methods without requiring a formal method declaration. But what are lambda expressions, and exactly why can they be so prevalent amongst developers?
What Are Lambda Expressions?
Essentially a lambda expression is an anonymous function – it doesn’t have a name like standard functions. Rather, it’s defined where it’s used: typically to send a piece of code to a constructor or an algorithm in languages with higher level abstractions. Lambda expressions contain a set of parameters (optionally empty), a => symbol and a body that specifies the expression or statements to execute. For example, in C#, (x, y) => x + y just describes a function which takes 2 variables and also returns their value.
The Appeal of Lambda Expressions
Lambda expressions are much more than shorthand for writing less code; they are symbols for something more fundamental: they bring several advantages to the table:
Conciseness: Lambda expressions minimize boilerplate code for anonymous classes, making your code cleaner and more readable.
Functionality: They allow functional programming features in object oriented languages to be implemented, techniques being treated as first-class citizens, passed around and executed in a given context on demand.
Scalability: In languages such as Java and C#, lambda expressions enable parallel processing by decomposing the code necessary for concurrent processing. This is especially useful for collections and arrays.
Flexibility: Finally, lambdas expose final variables from the enclosing scope allowing more freedom in your code design.
Enhanced Iteration: They simplify and make iterating over collections easier and more readable.
Table of Contents
- The Anatomy of a Lambda Expressions
- Lambda Expressions in Action: Real-World Examples
- Lambda vs. Anonymous Functions: A Comparative Overview
- Optimizing Your Code with Lambda Expressions
- Common Pitfalls and How to Avoid Them
- Lambda Expressions: Under the Hood
- Advanced Techniques and Patterns Using Lambda Expressions
- Lambda Expressions in Multithreading and Asynchronous Programming
- Real-World Scenario: Customizable Reports for a Sales Dashboard
- Conclusion: Integrating Lambda Expressions into Your Development Workflow
- Further Resources and Learning Aids
The Anatomy of a Lambda Expressions
Lambda expressions are a crucial feature in C#, enabling developers to write more concise and readable code. Understanding the structure of a lambda expression is essential for harnessing its full potential. In this section, we will explore the key components that make up a lambda expression in C#. This knowledge is particularly valuable in an ASP.NET development company, where such skills can lead to more robust and scalable web applications.
Components of a Lambda Expression
A lambda expression in C# consists of three fundamental parts: the input parameters, the lambda operator (=>
), and the body. Let’s break down each component:
- Input Parameters: These are the variables passed into the lambda expression. They are defined inside parentheses, similar to parameters in a method declaration. The type of these parameters can often be inferred by the compiler, allowing for type omission in many scenarios.Example:
(x, y)
Here, x
and y
are parameters whose types are inferred based on the context in which the lambda is used.
- Lambda Operator (
=>
): This is the heart of a lambda expression. The lambda operator is read as “goes to” and separates the input parameters from the body of the lambda. It effectively says, “This input goes to this output.” - Body: The body of a lambda expression can be either a single expression or a block of statements enclosed in
{}
. In the case of a single expression, the result of the expression is automatically returned. If the body consists of a block of statements, it behaves like a standard method body, and a return statement must be explicitly used if a value is to be returned. Examples:
Expression Body:
(x, y) => x + y
- This lambda expression takes two parameters and returns their sum. There is no need for a
return
statement; the result ofx + y
is implicitly returned.
Statement Body:
(x, y) => { return x + y; }
Syntax Variations
The syntax of lambda expressions in C# can vary based on the complexity of the operation being performed and the types of the input parameters. Here are some variations to consider:
- No Parameters: If no parameters are required, you use empty parentheses:
() => "Hello World"
- Single Parameter: If there is only one parameter and its type can be inferred, parentheses can be omitted:
x => x * x
- Multiple Statements: For complex operations, you can expand the lambda body to include multiple statements:
(x, y) => {
int result = x + y;
return result;
}
Lambda Expressions in Action: Real-World Examples
Lambda expressions in C# are not just theoretical constructs but practical tools that can simplify complex coding tasks, make code more readable, and improve performance in some cases. Here, we’ll look at some common real-world applications of lambda expressions in C# to see just how versatile and powerful they can be.
Simplifying Data Filtering with LINQ
One of the most common uses of lambda expressions in C# is with the Language Integrated Query (LINQ) framework. LINQ allows for SQL-like query abilities directly in C#. Lambda expressions make these queries more concise and readable.
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6 };
var evenNumbers = numbers.Where(n => n % 2 == 0).ToList();
In the example above, the lambda expression n => n % 2 == 0
is used to filter out only even numbers from the list. This concise syntax replaces the need for loop constructs and conditional statements to achieve the same result.
Event Handling
Lambda expressions are extremely useful in event handling, where they allow you to inline event handler code instead of creating separate methods. This can make it easier to understand what an event is doing since the logic is right where the event is subscribed.
button.Click += (sender, e) => MessageBox.Show("Button clicked!");
Here, instead of defining a separate method for the click event handler, a lambda expression is used directly within the event subscription. This keeps the code compact and focused.
Sorting Collections
Lambda expressions can also be used to provide quick and easy ways to sort collections. They can define custom sorting criteria in a clear and concise manner.
List<string> names = new List<string> { "Anna", "John", "Bob", "Alice" };
var sortedNames = names.OrderBy(name => name.Length).ToList();
In this example, names
are sorted by the length of the name using a lambda expression name => name.Length
as the key selector. This line of code replaces what would traditionally require a custom comparer object.
Asynchronous Programming with Tasks
Lambda expressions shine in asynchronous programming patterns, particularly with the use of the Task
class in .NET. They allow you to define tasks in a straightforward, inline manner without cluttering your code with excessive method definitions.
Task.Run(() => {
Console.WriteLine("Running an asynchronous task.");
Thread.Sleep(1000); // Simulating work
Console.WriteLine("Work completed.");
});
Delegates and Functional Programming
Finally, lambda expressions are integral in defining inline delegates, enabling a more functional style of programming. They can be used to pass behavior around as data.
Func<int, int, int> add = (x, y) => x + y;
Console.WriteLine(add(5, 3)); // Outputs 8
Lambda vs. Anonymous Functions: A Comparative Overview
Lambda expressions and anonymous functions in C# serve similar purposes but are characterized by different syntax and capabilities. Understanding these differences is key to using each effectively.
What Are Lambda Expressions?
Lambda expressions are concise and typically used for short functions that are passed as arguments or used in LINQ queries.
Example of a Lambda Expression:
var numbers = new List<int> { 1, 2, 3, 4, 5 };
var evenNumbers = numbers.FindAll(x => x % 2 == 0);
Here, x => x % 2 == 0
is a lambda expression that identifies even numbers in a list.
What Are Anonymous Functions?
Anonymous functions can be more verbose and are suited for more complex tasks that require multiple statements.
Example of an Anonymous Function:
var numbers = new List<int> { 1, 2, 3, 4, 5 };
var evenNumbers = numbers.FindAll(delegate(int x) {
Console.WriteLine($"Evaluating {x}");
return x % 2 == 0;
});
This anonymous function does the same job as the lambda but includes additional logging.
Key Differences and Benefits
- Syntax: Lambda expressions use the
=>
operator and are generally more succinct. Anonymous functions use thedelegate
keyword and can accommodate more complex statements. - Flexibility: Lambda expressions are limited to a single expression or a statement block. Anonymous functions can contain multiple statements and offer more complex control flows.
- Readability: Lambdas are cleaner and usually easier to read, especially when the functionality is straightforward. Anonymous functions provide clarity when more detailed processing is necessary.
Additional Examples:
- Lambda for simple return:
Func<int, int> square = x => x * x;
- Anonymous function for multiple operations:
Func<int, int> factorial = delegate(int x) {
int result = 1;
for (int i = 1; i <= x; i++) {
result *= i;
}
return result;
};
Optimizing Your Code with Lambda Expressions
Lambda expressions in C# are not just syntactic sugar; they are powerful tools that can drastically improve the readability and efficiency of your code by making it more concise and focused. Here’s how you can optimize your code with lambda expressions:
Improving Readability
Lambda expressions reduce clutter and make the intent of your code clearer by removing unnecessary syntax.
Example: Filtering a list
Without lambda:
List<int> list = new List<int> { 1, 2, 3, 4, 5 };
List<int> evenNumbers = new List<int>();
foreach (int number in list) {
if (number % 2 == 0) {
evenNumbers.Add(number);
}
}
With lambda:
List<int> list = new List<int> { 1, 2, 3, 4, 5 };
List<int> evenNumbers = list.Where(x => x % 2 == 0).ToList();
Enhancing Efficiency
Lambda expressions can lead to more efficient code by leveraging high-performance features like LINQ and lazy evaluation.
Example: Streamlined data processing
Without lambda:
int[] numbers = { 1, 2, 3, 4, 5 };
int sum = 0;
foreach (int number in numbers) {
if (number > 2) {
int processed = number * 2;
sum += processed;
}
}
With lambda:
int[] numbers = { 1, 2, 3, 4, 5 };
int sum = numbers.Where(n => n > 2).Select(n => n * 2).Sum();
The lambda version uses LINQ methods to filter and process the data in a more streamlined and potentially more performant way, especially for larger data sets.
Simplifying Event Handling
Lambda expressions make event handling more straightforward by embedding the handler logic directly where the event is subscribed, improving the locality of reference.
Example: Event subscription
Without lambda:
button.Click += Button_Click;
private void Button_Click(object sender, EventArgs e) {
MessageBox.Show("Clicked!");
}
With lambda:
button.Click += (sender, e) => MessageBox.Show("Clicked!");
This not only makes the event handling clearer by reducing the amount of code but also keeps related code together.
Facilitating Asynchronous Programming
Lambdas work seamlessly with asynchronous programming, making it easier to write and maintain asynchronous code.
Example: Async operations
Without lambda:
public async Task LoadData() {
await Task.Run(() => {
// Code to load data
});
}
With lambda:
public async Task LoadData() {
await Task.Run(() => LoadDataImplementation());
}
Common Pitfalls and How to Avoid Them
Lambda expressions are powerful, but like any powerful tool, they must be used correctly to avoid common mistakes. Here are several typical pitfalls and how you can sidestep them:
Overusing Lambda Expressions
Pitfall: Using lambda expressions where a simple method would be more appropriate can lead to code that is harder to understand and debug.
Example of Overuse:
var numbers = new List<int> { 1, 2, 3, 4, 5 };
numbers.ForEach(x => Console.WriteLine(Math.Pow(x, 2) + Math.Log(x)));
Solution: Use lambda expressions when they enhance readability and maintainability, not just for their own sake. Refactoring to a named method can clarify intentions:
numbers.ForEach(PrintComplexCalculation);
void PrintComplexCalculation(int x) {
Console.WriteLine(Math.Pow(x, 2) + Math.Log(x));
}
Capturing Outer Variables
Pitfall: Lambdas capture variables from the enclosing scope, which can lead to unintended side effects, especially in loops or asynchronous code.
Example of Problematic Capturing:
List<Action> actions = new List<Action>();
for (int i = 0; i < 5; i++) {
actions.Add(() => Console.WriteLine(i));
}
foreach (var action in actions) {
action(); // Prints '5' five times, not 0 to 4
}
**Solution:** Capture loop variables by creating a local copy inside the loop:
```csharp
for (int i = 0; i < 5; i++) {
int loopScopedI = i;
actions.Add(() => Console.WriteLine(loopScopedI));
}
Ignoring Performance Implications
Pitfall: Inefficient use of lambda expressions, especially with LINQ, can lead to poor performance due to unnecessary processing.
Example of Inefficient LINQ Usage:
var result = numbers.Where(x => x > 2).ToList().Select(x => x * 2).ToList();
Solution: Streamline LINQ queries to minimize intermediate conversions and operations:
var result = numbers.Where(x => x > 2).Select(x => x * 2).ToList();
Handling Exceptions
Pitfall: Exception handling within lambda expressions can be tricky and obscure, particularly if the lambda is used as an event handler.
Example of Hidden Exception:
someEvent += (sender, args) => {
throw new Exception("Oops!");
};
Solution: Handle exceptions within lambdas carefully or ensure they are managed at a higher level to maintain clarity:
someEvent += (sender, args) => {
try {
// Potentially problematic code here
} catch (Exception ex) {
// Handle exception properly
}
};
Lambda Expressions: Under the Hood
Understanding how lambda expressions are implemented by the C# compiler can provide insights into their performance characteristics and help developers use them more effectively.
Compilation of Lambda Expressions
When you write a lambda expression in C#, the compiler doesn’t directly convert it into machine code. Instead, it’s transformed into one of two forms, depending on its usage: either as a delegate or as an expression tree.
As a Delegate
Most commonly, lambda expressions are compiled into anonymous delegate types. This is particularly true when they are used in a context that expects a delegate, such as event handlers or LINQ to Objects.
Func<int, int> square = x => x * x;
Here, the lambda expression x => x * x
is compiled into a delegate that takes an integer and returns its square.
Generated Code Insight:
class GeneratedClass {
public static int Square(int x) {
return x * x;
}
}
Func<int, int> square = new Func<int, int>(GeneratedClass.Square);
The compiler generates a method in a private class and creates a delegate pointing to this method.
As an Expression Tree
When lambda expressions are used in LINQ to Entities or any other API that operates over expression trees, they are compiled into data structures that represent the code as a tree of expressions (nodes).
Example:
Expression<Func<int, int>> exprTree = x => x * x;
This allows frameworks like Entity Framework to translate the code into SQL, optimizing database operations without executing them client-side.
Generated Code Insight:
// Simplified representation
var exprTree = Expression.Lambda<Func<int, int>>(
Expression.Multiply(
Expression.Parameter(typeof(int), "x"),
Expression.Parameter(typeof(int), "x")
),
new ParameterExpression[] { Expression.Parameter(typeof(int), "x") }
);
Debugging Challenges
One caveat with lambda expressions is that debugging can be challenging because the code you write is not exactly what’s running. It’s transformed, and that can obfuscate stack traces and error messages.
Example of Debugging Issue:
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
var result = numbers.Select(x => x / (x - 3)).ToList();
Advanced Techniques and Patterns Using Lambda Expressions
Lambda expressions are not just for simple operations; they can be integral to sophisticated design patterns and advanced programming techniques in C#. Here are some advanced scenarios where lambda expressions shine, often utilized by the best C# developers to create more elegant and efficient applications:
1. Using Lambdas with the Strategy Pattern
Lambda expressions can replace entire classes in the Strategy pattern, especially when the strategies are simple.
Example: Dynamic Sorting Strategy
Instead of creating multiple strategy classes for sorting, you can define strategies using lambda expressions:
var people = new List<Person> {
new Person("Alice", 25),
new Person("Bob", 30),
new Person("Charlie", 20)
};
Func<Person, object> ageStrategy = p => p.Age;
Func<Person, object> nameStrategy = p => p.Name;
people.Sort((p1, p2) => Comparer<object>.Default.Compare(ageStrategy(p1), ageStrategy(p2)));
people.ForEach(p => Console.WriteLine($"{p.Name}, {p.Age}"));
people.Sort((p1, p2) => Comparer<object>.Default.Compare(nameStrategy(p1), nameStrategy(p2)));
people.ForEach(p => Console.WriteLine($"{p.Name}, {p.Age}"));
This approach allows you to switch sorting strategies dynamically using lambda expressions.
2. Lambdas for Factory Methods
Use lambda expressions to encapsulate object creation logic, simplifying the Factory pattern, especially when dealing with complex object configurations.
Example: Factory Lambda
Func<int, int, Rectangle> rectangleFactory = (width, height) => new Rectangle(width, height);
var myRectangle = rectangleFactory(50, 20);
Console.WriteLine($"Rectangle with width: {myRectangle.Width} and height: {myRectangle.Height}");
This factory lambda creates a rectangle object, which simplifies object creation and reduces the need for a dedicated factory class.
3. Lazy Initialization with Lambdas
Lambda expressions can be used for lazy initialization, deferring the creation of an object until it is actually needed.
Example: Lazy Initialization
Lazy<ValueToBeCalculated> lazyValue = new Lazy<ValueToBeCalculated>(() => ComputeExpensiveValue());
ValueToBeCalculated value = lazyValue.Value; // Value computed here
This technique is useful for optimizing performance, especially when initialization is expensive and might not be needed during the runtime.
4. Decorator Pattern Simplification
Lambda expressions can streamline the implementation of the Decorator pattern by wrapping functionalities.
Example: Adding Functionality
Func<string, string> greet = name => $"Hello, {name}!";
Func<string, string> shout = message => message.ToUpper();
Func<string, string> loudGreeting = name => shout(greet(name));
Console.WriteLine(loudGreeting("Alice"));
Here, loudGreeting
uses lambdas to combine simple greeting and shouting behaviors, effectively decorating the original greet
function.
5. Combining Behaviors
Lambda expressions allow for the easy combination and chaining of behaviors, which can be particularly useful in event handling or when implementing middleware-like patterns.
Example: Middleware Pattern
Func<string, string> addHeader = text => $"Header, {text}";
Func<string, string> addFooter = text => $"{text}, Footer";
Func<string, string> completeMessage = text => addFooter(addHeader(text));
Console.WriteLine(completeMessage("Main Content"));
Lambda Expressions in Multithreading and Asynchronous Programming
Lambda expressions can greatly simplify writing multithreaded and asynchronous code in C#. They allow for inline definitions of tasks or actions, reducing boilerplate and improving readability. Here are a few scenarios where lambda expressions shine in concurrent and parallel programming:
Simplifying Asynchronous Operations
Lambda expressions are commonly used with the Task
class or asynchronous methods to encapsulate code that should run asynchronously.
Example: Using Task.Run
Task.Run(() => {
Console.WriteLine("Running on a separate thread");
Thread.Sleep(1000); // Simulate work
Console.WriteLine("Work completed");
});
In this example, a lambda expression defines a block of code that runs on a background thread, managing simple asynchronous operations neatly and clearly.
Parallel Processing
The Parallel
class in .NET uses lambda expressions to make parallel processing more straightforward. This can be used to perform loop operations concurrently.
Example: Parallel.For
int[] numbers = { 1, 2, 3, 4, 5 };
Parallel.For(0, numbers.Length, i => {
Console.WriteLine($"Processing number: {numbers[i]} on thread {Thread.CurrentThread.ManagedThreadId}");
});
Here, the lambda expression is used within Parallel.For
, allowing each iteration of the loop to run on different threads, enhancing performance for large-scale operations.
Event Handling in UI Applications
Lambda expressions streamline event handling, particularly in GUI applications, by allowing developers to easily manage UI updates and asynchronous calls from events.
Example: Updating UI from Another Thread
button.Click += async (sender, e) => {
var result = await Task.Run(() => {
// Simulate a task
Thread.Sleep(1000);
return "Task Complete";
});
label.Text = result; // Update UI after task completion
};
This example shows how a lambda expression facilitates asynchronous execution and UI updating, post-task completion, in response to a button click.
Capturing Variables in Lambdas
A common use case in multithreaded scenarios involves capturing local variables within lambda expressions. Special care must be taken to avoid unintended side effects.
Example: Capturing Variables
for (int i = 0; i < 5; i++) {
Task.Run(() => Console.WriteLine(i));
}
This could print unexpected results due to the loop variable i
being captured by the lambda. To fix this, capture a copy of i
inside the loop:
for (int i = 0; i < 5; i++) {
int localI = i;
Task.Run(() => Console.WriteLine(localI));
}
Real-World Scenario: Customizable Reports for a Sales Dashboard
Imagine you’re building a sales dashboard for a large retail company. The dashboard must provide dynamic reports from which managers can choose to filter and order sales data by region, salesperson, or date range. This ability is essential for managers to analyze trends and performance metrics effectively.
Expression trees are especially useful here since they enable very flexible and dynamic queries. This is crucial for a system where the user interface enables writing and modifying queries for a large number of parameters that are unknown at runtime. To effectively implement such advanced features, it is advisable to hire skilled C# developers who are proficient in leveraging expression trees to handle complex querying requirements. These developers can ensure that the dashboard is not only functional but also optimized for performance and scalability.
Below is an example of how you might implement this dynamic query building functionality using expression trees in a C# application:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Linq.Expressions;
public class Sale
{
public int Id { get; set; }
public DateTime Date { get; set; }
public string Region { get; set; }
public string SalesPerson { get; set; }
public decimal TotalAmount { get; set; }
}
public class DynamicQueryBuilder
{
public Expression<Func<Sale, bool>> BuildDynamicWhereClause(string region, string salesPerson, DateTime? startDate, DateTime? endDate)
{
var parameter = Expression.Parameter(typeof(Sale), "sale");
Expression expression = Expression.Constant(true); // Start with a true expression
if (!string.IsNullOrEmpty(region))
{
expression = Expression.AndAlso(
expression,
Expression.Equal(
Expression.Property(parameter, "Region"),
Expression.Constant(region)
)
);
}
if (!string.IsNullOrEmpty(salesPerson))
{
expression = Expression.AndAlso(
expression,
Expression.Equal(
Expression.Property(parameter, "SalesPerson"),
Expression.Constant(salesPerson)
)
);
}
if (startDate.HasValue)
{
expression = Expression.AndAlso(
expression,
Expression.GreaterThanOrEqual(
Expression.Property(parameter, "Date"),
Expression.Constant(startDate.Value)
)
);
}
if (endDate.HasValue)
{
expression = Expression.AndAlso(
expression,
Expression.LessThanOrEqual(
Expression.Property(parameter, "Date"),
Expression.Constant(endDate.Value)
)
);
}
return Expression.Lambda<Func<Sale, bool>>(expression, parameter);
}
public IQueryable<Sale> ApplyFilters(IQueryable<Sale> sales, Expression<Func<Sale, bool>> filter)
{
return sales.Where(filter);
}
}
class Program
{
static void Main()
{
var sales = new List<Sale>
{
new Sale { Id = 1, Date = new DateTime(2023, 1, 20), Region = "North", SalesPerson = "Alice", TotalAmount = 300 },
new Sale { Id = 2, Date = new DateTime(2023, 2, 15), Region = "West", SalesPerson = "Bob", TotalAmount = 200 },
new Sale { Id = 3, Date = new DateTime(2023, 1, 25), Region = "North", SalesPerson = "Charlie", TotalAmount = 450 }
}.AsQueryable();
var queryBuilder = new DynamicQueryBuilder();
var filter = queryBuilder.BuildDynamicWhereClause("North", null, new DateTime(2023, 1, 1), null);
var filteredSales = queryBuilder.ApplyFilters(sales, filter);
foreach (var sale in filteredSales)
{
Console.WriteLine($"Sale: {sale.Id}, Date: {sale.Date}, Region: {sale.Region}, SalesPerson: {sale.SalesPerson}, Total: {sale.TotalAmount}");
}
}
}
In this example, the DynamicQueryBuilder
class uses Expression.Parameter
and other Expression
methods to construct a complex Where
clause dynamically based on input parameters such as region, salesperson, and date range. This method begins with a base expression that always evaluates to true
and conditionally builds upon this expression by adding more conditions.
Conclusion: Integrating Lambda Expressions into Your Development Workflow
For any .NET development company looking to enhance the efficiency and readability of their codebase, adopting lambda expressions is a crucial step. Lambda expressions are not just a tool for reducing the verbosity of your code; they fundamentally transform how you handle data, events, and asynchronous processes, making your applications more scalable and maintainable.
Key Benefits:
- Increased Readability: By minimizing boilerplate and focusing on the essence of operations, lambda expressions make your code easier to understand at a glance.
- Enhanced Maintainability: Less code means fewer bugs and easier maintenance. Lambda expressions help streamline bug fixes and feature updates.
- Boosted Performance: In scenarios involving LINQ or asynchronous programming, lambda expressions can lead to significant performance optimizations.
Further Resources and Learning Aids
Microsoft’s official learning platform provides modules on C# programming, including lambda expressions. Explore their interactive tutorials to get hands-on experience. Microsoft Learn – C#
Dream big, because at WireFuture, no vision is too ambitious. Our team is passionate about turning your software dreams into reality, with custom solutions that exceed expectations.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.