Mastering Vue.js – A Comprehensive Guide
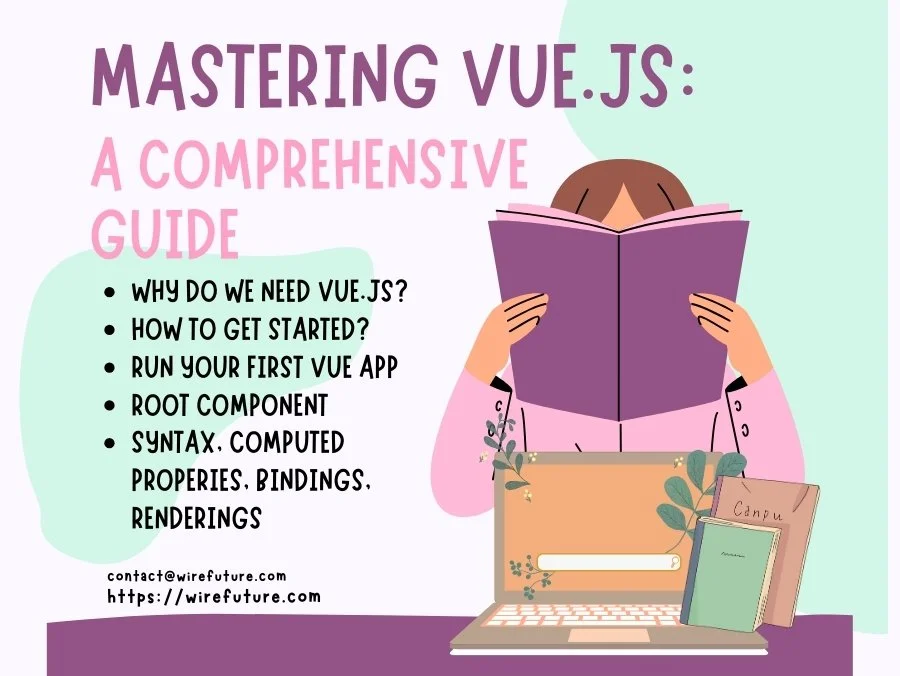
Vue.js is an open-source Model-View-ViewModel (MVVM) JavaScript framework that facilitates the creation of interactive web interfaces and single-page applications (SPAs). Because of its simplicity, ease of integration and fine balance between functionality and flexibility, Vue.js has rapidly gained popularity among developers since it was launched in 2014. Distinguished by its gentle learning curve, Vue.js enables developers to build powerful and dynamic web applications with minimal overhead.
The core library of Vue.js focuses on the view layer, allowing developers to efficiently manage the user interface with reactive data binding and composable view components. This design philosophy ensures that Vue.js can be seamlessly integrated into projects of any size, enhances existing web applications with minimal disruption or is used as a starting point for more complex Software Product Architectures.
Table of Contents
- Why Do We Even Need Vue.js?
- How To Get Started?
- Diving Deep into Vue.js Root Component
- Understanding Vue.js Template Syntax
- Diving into Reactivity Fundamentals
- Computed Properties
- Styling with Class and Style Bindings
- Implementing Conditional Rendering
- List Rendering
- Handling Events with Vue
- Navigating Component Lifecycle with Events
- API Calls in Vue with Axios
- Vue.js vs. Angular: A Developer’s Dilemma
- Wrapping Up
Why Do We Even Need Vue.js?
Now, why do we even need Vue.js? Think of it as your go to tool for creating a seamless and interactive web experience. Vue has taken the role of a superhero in an era where user experience can make or destroy your Web. It’s about simplifying the process of development, while offering a reactive and flexible framework that can deal with any size application.
For newcomers and experienced developers looking to improve their toolkit, Vue’s smooth learning curve, detailed documentation as well as active community support make it a no. 1 choice. In a complex web development process, it’s as if you’ve got a friendly guide to make sure you’re never lost.
Vue.js provides a comprehensive solution for professional web application development by offering an extensive ecosystem, including tools such as Vue Router for client side routing and Vuex for state management.
How To Get Started?
Vue.js, known for its simplicity and efficiency, offers a streamlined approach to building interactive web applications. From scratch, here’s how to quickly start the Vue application:
Install Vue CLI
First, ensure you have Node.js installed on your system. Then, install Vue CLI, Vue’s Command Line Interface, which facilitates the creation of new projects. Open your terminal and run:
npm install -g @vue/cli
Create a Vue Project
After installing Vue CLI, create a new project by executing:
vue create my-vue-app
Follow the prompts to select presets for your project. For beginners, the default preset is recommended.
Run Your Vue Application
Navigate into your project directory and start the development server:
cd my-vue-app
npm run serve
This command compiles and hot-reloads your project for development. You can see your new Vue app in action by opening the browser and going to http://localhost:8080.
Here’s a basic Vue component example to give you a taste of Vue’s simplicity:
<template>
<div id="app">
<h1>{{ message }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
message: "Hello, Vue World!"
}
}
}
</script>
This snippet specifies a Vue component that contains the data property message, and displays it in an h1 tag. The declarative rendering of Vue makes it easy to connect the HTML structure with JavaScript logic, which is a demonstration of its capabilities for building interactive web interfaces.
Diving Deep into Vue.js Root Component
The cornerstone of your application is a root component in Vue.js, which organises the hierarchy of child components and serves as an initial mounting point. To master the development of Vue.js, it is important to understand its structure and functions. With illustrative code samples, let’s see how to define and use the root component.
When you initiate a Vue instance, you essentially create the root component:
const app = new Vue({
el: '#app',
data: {
greeting: 'Welcome to Your Vue.js App'
}
});
This instance attaches to a DOM element (#app
) and serves as the root for your Vue application. The data
object contains properties accessible in the component’s template:
<div id="app">
<h1>{{ greeting }}</h1>
</div>
For more complex applications, the root component can include methods, computed properties, and lifecycle hooks, among others:
const app = new Vue({
el: '#app',
data: {
greeting: 'Welcome to Your Vue.js App'
},
methods: {
sayHello() {
alert('Hello, Vue.js!');
}
},
computed: {
capitalizedGreeting() {
return this.greeting.toUpperCase();
}
},
mounted() {
console.log('App is mounted and ready!');
}
});
The methods in this example contain functions that can be invoked from the template. Calculated properties calculate values based on the component’s data and are a lifecycle hook, which is triggered as soon as an element is inserted into this DOM. These features will allow the root component to manage and show the dynamic content in an efficient way.
Understanding Vue.js Template Syntax
Vue.js uses a straightforward template syntax to bind the rendered DOM to the underlying component instance’s data. Using mustache syntax {{ }}
, you can easily output data to the template:
<div id="app">
<p>{{ message }}</p>
</div>
data: {
message: 'Hello Vue!'
}
For directives (special tokens starting with v-
), Vue provides powerful functionalities like v-bind
for binding attributes and v-model
for creating two-way data bindings on form inputs:
<input v-model="message">
Diving into Reactivity Fundamentals With Vue.js
Vue’s reactivity system ensures that your UI updates automatically when your application state changes. It’s built on a simple but effective mechanism:
data: {
counter: 0
}
When counter
changes, any part of your application using it will update:
<div id="app">
<p>{{ counter }}</p>
<button @click="counter++">Increment</button>
</div>
Vue achieves this by tracking dependencies during render and automatically re-rendering when dependencies change.
Computed Properties In Vue.js
Computed properties in Vue are used for calculations that need to be cached and updated only when dependencies change:
computed: {
reversedMessage() {
return this.message.split('').reverse().join('');
}
}
Use it in your template like any property:
<p>{{ reversedMessage }}</p>
Styling with Class and Style Bindings
Vue simplifies dynamic styling with v-bind:class
and v-bind:style
. You can toggle classes based on component data:
<div v-bind:class="{ active: isActive }"></div>
And apply styles dynamically:
<div v-bind:style="{ color: activeColor, fontSize: fontSize + 'px' }"></div>
Implementing Conditional Rendering
Vue’s v-if
, v-else-if
, and v-else
directives make conditional rendering straightforward:
<p v-if="user === 'admin'">Admin Panel</p>
<p v-else>User Panel</p>
This renders elements conditionally based on the truthiness of the data property.
List Rendering in Vue.js
With v-for
, Vue.js can render a list of items based on an array:
<ul id="app">
<li v-for="item in items">{{ item.text }}</li>
</ul>
data: {
items: [{ text: 'Learn Vue' }, { text: 'Build something awesome' }]
}
Handling Events with Vue
Vue.js simplifies event handling with the v-on
directive or the shorthand @
, allowing you to listen to DOM events:
<button @click="greet">Greet</button>
methods: {
greet() {
alert('Hello Vue.js!');
}
}
Navigating Component Lifecycle with Events in Vue.js
Vue components go through a lifecycle, from creation to destruction, with hooks like created
, mounted
, updated
, and destroyed
:
created() {
console.log('Component is created!');
}
These hooks offer opportunities to perform actions at specific stages, ensuring fine-grained control over the component’s behavior throughout its lifecycle.
API Calls in Vue with Axios
Axios, a promise-based HTTP client, is the go-to library for making HTTP requests in Vue. You can use Axios to fetch data from an API and breathe new life into your Vue application here.
Installing Axios
First, you need to add Axios to your project. Run this command in your project directory:
npm install axios
Making an API Call
Once Axios is installed, you can import it into your Vue component and use it to make API calls. Here’s a simple example of fetching data from a JSON placeholder API and displaying it:
<template>
<div>
<ul>
<li v-for="post in posts" :key="post.id">
{{ post.title }}
</li>
</ul>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
posts: []
};
},
created() {
this.fetchPosts();
},
methods: {
fetchPosts() {
axios.get('https://wirefuture.com/get-data')
.then(response => {
this.posts = response.data;
})
.catch(error => {
console.log(error);
});
}
}
}
</script>
In this code, axios.get is used to send a GET request to the specified URL. You can assign the response data to your component’s posts data property using the then method to handle the Axios promise. To gracefully deal with any issues that may occur during the request, error handling is managed using a catch method.
Vue.js vs. Angular: A Developer’s Dilemma
Comparing Vue.js with Angular is like comparing a nimble speedboat to an enormous yacht, both of which have their place on the sea, but they’ve got different approaches. Angular, a fully functional MVC framework, is like a Swiss Army knife for web development; it’s robust, feature packed, and designed to build large scale applications right out of the box. At the same time, Vue chooses to take a minimal approach that focuses on being light and flexible. In projects where speed and ease of development are key, this simplicity and adaptability often makes Vue the preferred choice. Angular is the solution for enterprise level applications where complexity is an inherent condition, bringing structure and a full ecosystem together.
So, when’s the time to pick Vue instead of Angular? Vue could be your best bet if you’re starting a project with time to market which is critical, and value simplicity and flexibility. It’s particularly well-suited for projects that start small but might scale over time, thanks to its incremental adoptability. Vue’s low footprint and simple API may be a real game changer in the case of startups and projects where developer velocity and ease of maintenance is paramount.
For small projects or teams looking for a view layer magic that doesn’t require additional weight, Angular might be an overkill because of its hard learning curve and boilerplate code.
Basically, Vue.js shines when you want a combination of simplicity, speed and reactivity without having to commit to the complete complexity of something like Angular. It’s all about choosing the right tool for the job, and sometimes Vue’s focused, flexible approach is exactly what the project required.
Wrapping Up
As we’re about to finish our examination of Vue.js, it is clear that the dynamic JavaScript framework stands out in terms of simplicity, flexibility and effectiveness. For developers who want to create dynamic, responsive Web applications quickly and easily, Vue.js has proven itself as a valuable tool.
Vue.js offers a powerful set of features that meet the needs of many different projects, whether you’re new to web development or an experienced professional looking for efficiency in your workflow. Vue.js represents a balance of power and simplicity that accelerates the development process without sacrificing performance or scale, from its reactive data binding and component based architecture to the ease of integrating with other libraries and tools.
For those intrigued by the prospects of Vue.js for their next project and looking to hire Vue developers, navigating this journey with a seasoned partner can amplify your success. WireFuture stands ready to be that partner. Your digital vision can be brought to life by our team of Vue.js specialists, delivering custom solutions that match your project’s objectives perfectly. With WireFuture, you’ll be able to take advantage of a pool of talented developers dedicated to excellence in the development of Vue.js and ensure your project is not only completed but also unique. Considering a Vue.js project? Reach out to WireFuture. Let us show you how our Vue.js expertise can set your project apart and transform your digital ideas into tangible realities. Together, we can create something special.
WireFuture's team spans the globe, bringing diverse perspectives and skills to the table. This global expertise means your software is designed to compete—and win—on the world stage.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.