Server Side Pagination using Angular and ASP.NET Core
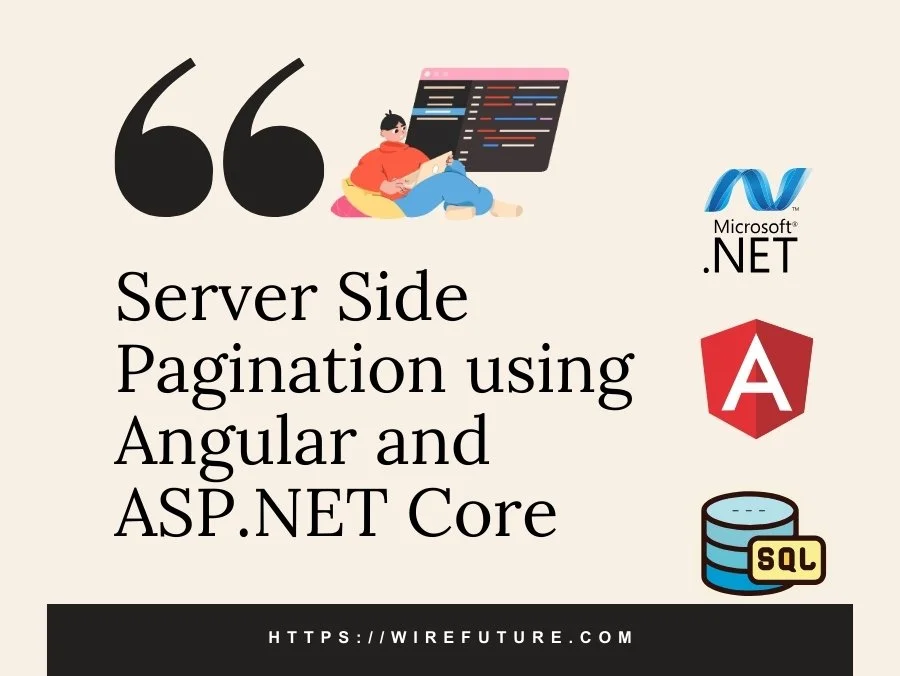
In modern web development, Angular is a strong framework offering solutions for building dynamic and responsive single page applications. A crucial element for just about any data-driven application is an effective grid system which works with large datasets. This article shows you how to implement a grid using the latest Angular, ng-bootstrap for user interface components and server side pagination and filtering with ASP.NET Core 8.
This approach takes advantage of both Angular and the slick and responsive design of ng-bootstrap. Further, ASP.NET Core 8 for backend operations makes your application performant and scalable even with huge datasets. Whether you are developing enterprise-level applications or sophisticated web solutions, this integration will lay the foundation for creating effective and – user-friendly data management interfaces.
At the conclusion of this tutorial, you ought to have a completely functioning Angular application with a grid with server-side pagination and filtering to deal with big datasets. This guide is particularly beneficial for any Angular development company looking to enhance their application’s data handling capabilities. See the process for achieving this integration.
Table of Contents
- Prerequisites
- Server Side Pagination: Setting Up the ASP.NET Core Backend
- Server Side Pagination: Setting Up the Angular Frontend with ng-bootstrap
- Running the Application
- Conclusion
Prerequisites
Before we begin, ensure you have the following installed:
- Node.js
- Angular CLI
- .NET SDK (version 8 or later)
- Visual Studio Code or any other code editor
For those interested in learning more about .NET development, check out our .NET Development blogs. Stay updated with the latest insights and best practices!
Server Side Pagination: Setting Up the ASP.NET Core Backend
1. Create a New ASP.NET Core Project Open your terminal and run the following commands to create a new ASP.NET Core Web API project. We’ll use Entity Framework Core for the purpose of data access:
dotnet new webapi -n GridDemo
cd GridDemo
2. Add Models
Create a Models
folder and add a Product.cs
file to represent your data model:
namespace GridDemo.Models
{
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
}
3. Add Data Context
In the Data
folder, create a ProductContext.cs
file:
using Microsoft.EntityFrameworkCore;
using GridDemo.Models;
namespace GridDemo.Data
{
public class ProductContext : DbContext
{
public ProductContext(DbContextOptions<ProductContext> options) : base(options) { }
public DbSet<Product> Products { get; set; }
}
}
4. Configure Database in appsettings.json
Add the connection string to your appsettings.json
:
{
"ConnectionStrings": {
"DefaultConnection": "YourConnectionStringHere"
},
// ... other settings
}
5. Register Data Context in Startup.cs
In Startup.cs
, register your data context:
services.AddDbContext<ProductContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
services.AddControllers();
6. Create a Controller
Add a ProductsController.cs
in the Controllers
folder to handle API requests:
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using GridDemo.Data;
using GridDemo.Models;
using System.Linq;
using System.Threading.Tasks;
namespace GridDemo.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ProductsController : ControllerBase
{
private readonly ProductContext _context;
public ProductsController(ProductContext context)
{
_context = context;
}
[HttpGet]
public async Task<IActionResult> GetProducts([FromQuery] int page = 1, [FromQuery] int pageSize = 10, [FromQuery] string filter = "")
{
var query = _context.Products.AsQueryable();
if (!string.IsNullOrEmpty(filter))
{
query = query.Where(p => p.Name.Contains(filter));
}
var totalRecords = await query.CountAsync();
var products = await query.Skip((page - 1) * pageSize).Take(pageSize).ToListAsync();
return Ok(new { Data = products, TotalRecords = totalRecords });
}
}
}
Server Side Pagination: Setting Up the Angular Frontend with ng-bootstrap
For those interested in learning more about Angular development, check out our Angular Development blogs. Stay updated with the latest insights and best practices!
1. Create a New Angular Project
If you haven’t already created an Angular project, run the following commands:
ng new grid-demo
cd grid-demo
2. Install Required Packages
Install ng-bootstrap
and Bootstrap:
npm install @ng-bootstrap/ng-bootstrap bootstrap
3. Configure Bootstrap
Add Bootstrap to your angular.json
file:
"styles": [
"node_modules/bootstrap/dist/css/bootstrap.min.css",
"src/styles.css"
],
4. Create a Product Service
Generate a new service to handle HTTP requests:
ng generate service services/product
Implement the service to fetch data from the API:
// src/app/services/product.service.ts
import { Injectable } from '@angular/core';
import { HttpClient, HttpParams } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class ProductService {
private apiUrl = 'https://localhost:5001/api/products';
constructor(private http: HttpClient) { }
getProducts(page: number, pageSize: number, filter: string = ''): Observable<any> {
let params = new HttpParams()
.set('page', page.toString())
.set('pageSize', pageSize.toString());
if (filter) {
params = params.set('filter', filter);
}
return this.http.get(this.apiUrl, { params });
}
}
5. Create a Product Component
Generate a new component to display the grid:
ng generate component components/product
Implement the component to fetch and display data:
// src/app/components/product/product.component.ts
import { Component, OnInit } from '@angular/core';
import { ProductService } from 'src/app/services/product.service';
@Component({
selector: 'app-product',
templateUrl: './product.component.html',
styleUrls: ['./product.component.css']
})
export class ProductComponent implements OnInit {
products = [];
totalRecords = 0;
pageSize = 10;
page = 1;
filter = '';
constructor(private productService: ProductService) { }
ngOnInit(): void {
this.loadProducts();
}
loadProducts(): void {
this.productService.getProducts(this.page, this.pageSize, this.filter).subscribe(data => {
this.products = data.data;
this.totalRecords = data.totalRecords;
});
}
onPageChange(page: number): void {
this.page = page;
this.loadProducts();
}
onFilterChange(filter: string): void {
this.filter = filter;
this.loadProducts();
}
}
6. Design the Product Component Template
Use ng-bootstrap
components to design the grid:
<!-- src/app/components/product/product.component.html -->
<div class="container mt-5">
<div class="mb-3">
<input type="text" class="form-control" placeholder="Filter" (input)="onFilterChange($event.target.value)">
</div>
<table class="table table-striped">
<thead>
<tr>
<th scope="col">ID</th>
<th scope="col">Name</th>
<th scope="col">Price</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let product of products">
<td>{{product.id}}</td>
<td>{{product.name}}</td>
<td>{{product.price}}</td>
</tr>
</tbody>
</table>
<ngb-pagination [collectionSize]="totalRecords" [(page)]="page" [pageSize]="pageSize" (pageChange)="onPageChange($event)"></ngb-pagination>
</div>
7. Add ng-bootstrap
Module
Import necessary ng-bootstrap
modules in your AppModule
:
// src/app/app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule } from '@angular/common/http';
import { NgbModule } from '@ng-bootstrap/ng-bootstrap';
import { AppComponent } from './app.component';
import { ProductComponent } from './components/product/product.component';
import { ProductService } from './services/product.service';
@NgModule({
declarations: [
AppComponent,
ProductComponent
],
imports: [
BrowserModule,
HttpClientModule,
NgbModule
],
providers: [ProductService],
bootstrap: [AppComponent]
})
export class AppModule { }
Running the Application
1. Run the ASP.NET Core Backend
dotnet run
2. Run the Angular Frontend
ng serve
Navigate to http://localhost:4200
in your browser to see the grid in action. You should now have a fully functional Angular grid with server-side paging and filtering powered by ng-bootstrap
.
Conclusion
This article demonstrated how to integrate a grid in an Angular application with ng-bootstrap for UI components and ASP.NET Core 8 for the backend. This setup supports efficient server side pagination and filtering and is a robust solution for large datasets in modern web applications.
Leveraging Angular’s powerful framework and ng-bootstrap’s flexible components, we built a simple grid interface. On the backend, ASP.NET Core 8’s API capabilities provided fast data retrieval and manipulation making the whole system performant and scalable.
This combination of technologies provides a single unified approach to developing feature rich applications that can handle large data requirements. Whether you create enterprise-level applications or smaller projects, this integration sets the groundwork for creating responsive, effective data-driven applications.
Success in the digital age requires strategy, and that's WireFuture's forte. We engineer software solutions that align with your business goals, driving growth and innovation.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.