TypeScript vs JavaScript: Which One Should You Use?
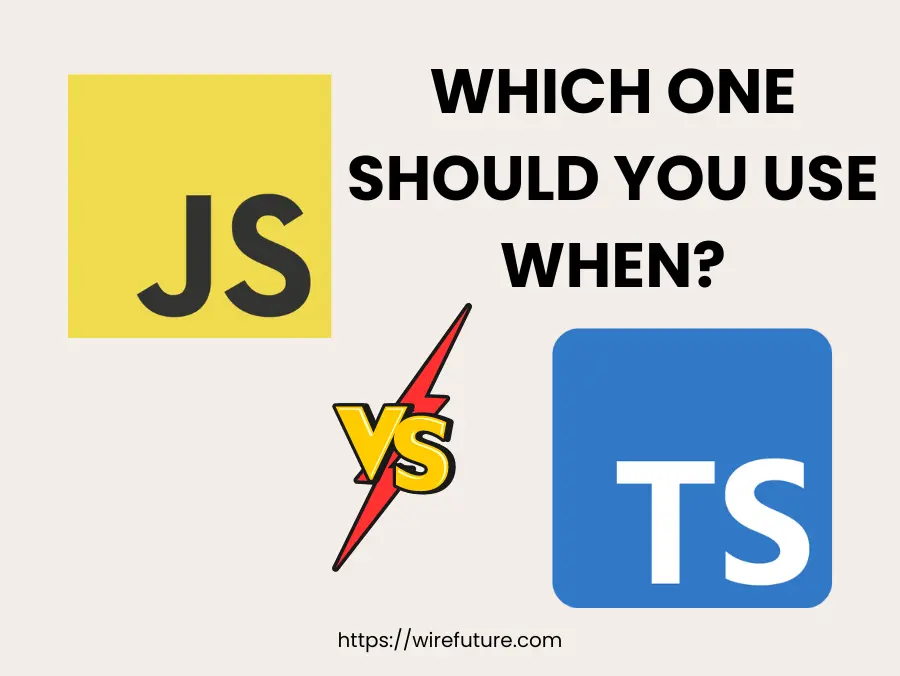
In this piece, we’ll dive into a side-by-side comparison of TypeScript vs JavaScript, exploring their relationship, key differences, and the unique benefits each language offers. From its beginnings in the 1990s to these days, JavaScript remains the basis for interactive web development. It powers everything from simple webpage animations to large web applications and is the essential tool for all developers worldwide, being the fundamental scripting language of the internet. Its portability and fluidity have helped create immersive and dynamic web experiences that have evolved alongside the internet.
But as web projects became more complex and scalable, developers struggled with managing massive codebases and maintaining code reliability. Recognising these difficulties, Microsoft introduced TypeScript in the early 2010s. TypeScript extends JavaScript, maintaining the language’s core features but adding a static type system and other improvements for code quality and developer productivity. With built-in error checking and debugging features and powerful tools to work with large programs, TypeScript is now a favorite for enterprise projects and offers developers navigating today’s web development challenges.
Table of Contents
- TypeScript vs JavaScript: More Like Cousins Than Rivals
- TypeScript vs JavaScript: The Differences
- TypeScript: Sharpening the Arrow in Your Quiver
- JavaScript: The Nimble Warrior of Web Development
- TypeScript vs JavaScript Use Cases: Picking the Right Tool for the Job
- Community and Ecosystem: Thriving and Evolving
- TypeScript vs JavaScript: Conclusion
- TypeScript vs. JavaScript FAQ
TypeScript vs JavaScript: More Like Cousins Than Rivals
Diving deeper into the kinship between TypeScript and JavaScript, it becomes clear that these two languages share a foundational relationship. We demonstrate their similarity with code samples that highlight their shared capabilities in front-and back-end environments, courtesy of the Node.js runtime. Here is picture showing how TypeScript works:
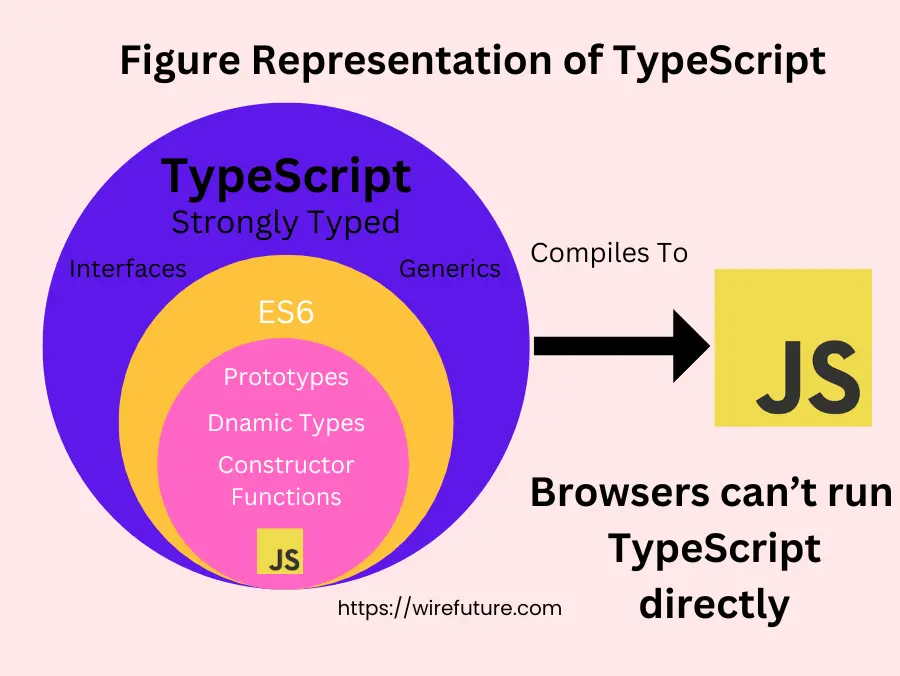
Shared Front-end Possibilities
JavaScript Example: A simple function to fetch data from an API
function fetchData(url) {
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Fetching error:', error));
}
TypeScript Equivalent
function fetchData(url: string): void {
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Fetching error:', error));
}
In both snippets, the functionality remains the same, demonstrating how TypeScript and JavaScript can be used interchangeably for front-end tasks. TypeScript adds type annotations, enhancing code readability and reliability.
Unified Back-end Development with Node.js
JavaScript Example: Creating a simple server
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => console.log('Server running on port 3000'));
TypeScript Equivalent
import express, { Application, Request, Response } from 'express';
const app: Application = express();
app.get('/', (req: Request, res: Response) => {
res.send('Hello World!');
});
app.listen(3000, () => console.log('Server running on port 3000'));
Here we configure a minimal server with Express in a Node.js environment using both JavaScript and TypeScript. TypeScript adds type types for requests and responses, improving the development experience with type checks and autocompletion.
These examples highlight that TypeScript and JavaScript are cousins rather than competitors. They both are highly configurable front-and back-end development tools. The superset TypeScript provides JavaScript type safety and additional features, making it a good choice for developers needing high scalability without leaving the JavaScript ecosystem.
TypeScript vs JavaScript: The Differences
When comparing TypeScript and JavaScript, their divergent paths are apparent in several important respects: development tools, the type system, learning curve and the learning curve. We’ll examine these differences using detailed code samples to show you what each language actually is.
Type System: Static vs. Dynamic
JavaScript Dynamic Typing Example
let myVariable = 'Hello, world!';
console.log(myVariable); // 'Hello, world!'
myVariable = 23;
console.log(myVariable); // 23
In JavaScript, a variable’s type can change at runtime, reflecting its dynamic type system. This flexibility allows for rapid development but can lead to unexpected bugs.
TypeScript Static Typing Example
let myVariable: string = 'Hello, world!';
console.log(myVariable); // 'Hello, world!'
myVariable = 23; // Error: Type 'number' is not assignable to type 'string'.
TypeScript’s static typing system enforces type consistency, catching type mismatches at compile time, thus preventing a class of runtime errors.
Learning Curve: Simplicity vs. Rigor
JavaScript Simplicity
// JavaScript's flexibility allows for simple, straightforward function definitions.
function add(a, b) {
return a + b;
}
console.log(add(5, 3)); // 8
JavaScript’s less strict nature makes it more accessible to beginners but can lead to issues with type safety and scalability in complex applications.
TypeScript Additional Syntax
// TypeScript requires types for function parameters and output.
function add(a: number, b: number): number {
return a + b;
}
console.log(add(5, 3)); // 8
The additional syntax in TypeScript (type annotations, interfaces, etc.) contributes to a steeper learning curve but pays off in larger, more complex applications through enhanced code safety and readability.
Development Tools: Basic vs. Advanced
JavaScript Tooling Example In JavaScript, development tools can catch some errors, but the dynamic nature of the language limits the ability to perform comprehensive checks before runtime.
TypeScript Enhanced Tooling Example
interface IEmployee {
name: string;
title: string;
}
function hireEmployee(employee: IEmployee) {
// ...
}
// TypeScript's IDE support and tooling would catch errors here at compile time.
hireEmployee({ namee: "John Doe", title: "Developer" }); // Error: Object literal may only specify known properties, and 'namee' does not exist in type 'IEmployee'.
TypeScript supports more advanced tooling through autocompletion, interface definition, and debugging capabilities via its static type system, improving the developer experience by catching errors early in the development process.
These differences highlight the complementary contributions of TypeScript and JavaScript to the development ecosystem. Whereas JavaScript provides simple entry and flexibility, TypeScript provides the structure and tools to support larger, more complex projects with greater reliability and maintainability.
TypeScript: Sharpening the Arrow in Your Quiver
In fact, TypeScript has totally transformed the way programmers create and maintain huge applications by providing a comprehensive set of features for enhancing code quality, scalability and readability. We’ll examine these benefits in more detail with code samples that highlight TypeScript’s strengths.
Enhanced Code Quality and Readability
Static Typing for Early Error Detection
// TypeScript static typing
let message: string = 'Hello, TypeScript';
console.log(message);
message = 123; // Error: Type 'number' is not assignable to type 'string'.
In this example, TypeScript’s static type system flags a type mismatch error during development, preventing a potential runtime error that could occur in JavaScript due to its dynamic typing.
Function Parameter and Return Types
function greet(name: string): string {
return `Hello, ${name}!`;
}
const greeting = greet('Alice');
console.log(greeting); // "Hello, Alice!"
// greet(42); // Error: Argument of type 'number' is not assignable to parameter of type 'string'.
By specifying parameter and return types, TypeScript ensures that functions are called with the correct types, enhancing code readability and reliability.
Powerful Tools for Large Projects
Interfaces for Structured Data
interface User {
id: number;
name: string;
isLoggedIn: boolean;
}
const user: User = {
id: 1,
name: 'John Doe',
isLoggedIn: true
};
// Using the interface to enforce structure on an object.
console.log(user);
Interfaces in TypeScript define the structure for objects, ensuring that data adheres to a specified format, which is invaluable in large projects where consistency is key.
Generics for Reusable Code
function getArray<T>(items : T[]) : T[] {
return new Array<T>().concat(items);
}
let numberArray = getArray<number>([1, 2, 3]);
let stringArray = getArray<string>(['Hello', 'World']);
console.log(numberArray); // [1, 2, 3]
console.log(stringArray); // ['Hello', 'World']
Generics allow for the creation of reusable components by providing a way to use types as variables in other types, functions, or interfaces. This flexibility ensures that functions and components can work with any data type without losing type safety.
Namespaces for Organizing Code
namespace Validation {
export interface StringValidator {
isValid(s: string): boolean;
}
export class LettersOnlyValidator implements StringValidator {
isValid(s: string) {
return /^[A-Za-z]+$/.test(s);
}
}
export class ZipCodeValidator implements StringValidator {
isValid(s: string) {
return /^\d{5}$/.test(s);
}
}
}
let validator = new Validation.ZipCodeValidator();
console.log(validator.isValid('12345')); // true
TypeScript namespaces group related functionalities, classes, interfaces, and other identifiers into a named scope, which is useful for organizing code in a large codebase.
With these examples, TypeScript provides programmers with the way to create far better code, read it, and keep it, particularly when creating huge applications. Static typing, in addition to its enhancements like interfaces, namespaces and generics, makes TypeScript an effective tool for any programmer, sharpening the arrow to produce more precise code.
JavaScript: The Nimble Warrior of Web Development
Known as the agile hacker of web development, JavaScript remains strong in today’s digital landscape. Its versatility and universal browser support make it a must for developers almost everywhere. We will examine more details of the attributes that make JavaScript so important for web development.
Flexibility and Dynamism
Quick Prototyping
JavaScript supports rapid prototyping, which is critical for early iteration and ideation in the development cycle. Its loose typing system enables developers to write less code and iterate on the fly without being constrained by strict type constraints.
// Quickly define and use an object without predefining its structure
const user = { name: "Alice", age: 25 };
user.location = "Wonderland"; // Easily add properties on the fly
console.log(user); // { name: "Alice", age: 25, location: "Wonderland" }
This example demonstrates how effortlessly new properties can be added to objects, showcasing JavaScript’s adaptability in prototyping phases.
Flexibility in Function Use
JavaScript functions can be passed around as variables, allowing for flexible code structures that can adapt to various programming patterns, such as callbacks and promises for handling asynchronous operations.
// Callback function to handle asynchronous operation
function fetchData(callback) {
setTimeout(() => {
callback("Data fetched after 2 seconds");
}, 2000);
}
fetchData(data => console.log(data)); // "Data fetched after 2 seconds"
The ease of creating and passing callback functions underscores JavaScript’s dynamic capabilities, facilitating non-blocking code execution which is essential in web development.
Universal Support
No Compilation Required
A major strength of JavaScript is its native support across all web browsers. The code is written in JavaScript format and executed directly in the browser without compiling. This rapidity is both a benefit to development speed and allows JavaScript applications to reach a wide audience without concerns about compatibility.
<!DOCTYPE html>
<html>
<body>
<script>
// Simple JavaScript code that runs in any browser
alert('Hello, world!');
</script>
</body>
</html>
This snippet exemplifies the ease with which JavaScript integrates into a web page, making it accessible instantly to users across different browsers and devices.
The Universal Language of the Web
Thanks to its native support in browsers, JavaScript has become the de facto language of the web. It powers interactive elements on websites, from simple animations to complex single-page applications, without requiring any additional software or plugins.
// Manipulating web page content directly
document.getElementById("demo").innerHTML = "Welcome to JavaScript!";
JavaScript enriches user interfaces by manipulating DOM elements directly, and provides dynamic content and interactivity to the web site.
Ultimately, JavaScript’s portability for rapid prototyping and its decentralized support across browsers make it fundamentally part of modern web development. Its fast development times and its cross-platform execution make JavaScript the agile warrior that can help solve the challenges of building rich, responsive and accessible web applications.
TypeScript vs JavaScript Use Cases: Picking the Right Tool for the Job
Technology selection is a crucial decision when starting a brand new project. This particular choice may significantly influence the development time, scalability and maintainability of the project. For web development, TypeScript and JavaScript are two dominant tools with different benefits for different project requirements. We’ll explore the use cases wherein each is better suited, and when one ought to be chosen over the other.
TypeScript: The Architect of Large-scale Applications
Large-scale Enterprise Applications
TypeScript’s static typing and robust tooling shine in the context of large-scale applications. These applications typically involve complex business logic, extensive codebases, and a large team of developers. TypeScript’s type system facilitates early detection of errors, making the codebase easier to refactor and maintain.
interface User {
id: number;
name: string;
}
// Static typing ensures that all user objects adhere to the User interface structure, reducing errors in large codebases.
const getUser = (userId: number): User => {
// Functionality to fetch user details
return { id: userId, name: 'John Doe' };
}
Scalability and Maintainability
For projects expected to grow significantly, TypeScript offers features like interfaces, generics, and namespaces, which help organize code and ensure it remains scalable and maintainable.
// Using generics for scalable and reusable code components
function mergeObjects<T, U>(obj1: T, obj2: U): T & U {
return { ...obj1, ...obj2 };
}
const mergedObj = mergeObjects({ name: 'Alice' }, { id: 1 });
console.log(mergedObj); // Output: { name: 'Alice', id: 1 }
JavaScript: The Swift Blade for Smaller Projects and Prototypes
Smaller Projects and Quick Prototypes
JavaScript’s dynamic nature and ease of use make it particularly well-suited for smaller projects and rapid prototyping. Developers can quickly write and test ideas without being bogged down by strict type constraints, speeding up the iteration process.
// Quick prototyping with JavaScript
let product = { name: 'Book', price: 9.99 };
// Easily add new properties or change existing ones
product.category = 'Education';
console.log(product); // Output: { name: 'Book', price: 9.99, category: 'Education' }
Dynamic Development Environments
In environments where requirements change frequently or projects that require a high degree of flexibility, JavaScript’s dynamic typing and the vast ecosystem of libraries and frameworks make it a perfect fit.
// Leveraging JavaScript's flexibility in dynamic environments
function calculateDiscount(price, discount) {
return price * (1 - discount);
}
// The same function can be used for different types of values
console.log(calculateDiscount(100, 0.1)); // Output: 90
console.log(calculateDiscount('100', '0.1')); // Output: 90
Finally, the decision between TypeScript vs JavaScript depends on your project requirements and goals. TypeScript is preferred for large scale applications that need strong scalability and maintainability. Conversely, JavaScript is incredibly fast and flexible and is therefore best for smaller projects, quick prototypes, or even complex development environments. By picking out the best tool for the job, developers can anticipate a smoother, quicker development process based on their project needs.
Community and Ecosystem: Thriving and Evolving
TypeScript and JavaScript have extensive ecosystems; they are rich. They are living, breathing things which are developing and expanding due to the work and creativity of their communities. These languages are the basis of web development, accompanied by a maze of libraries, tools and frameworks for any possible requirement a developer may have. We will explore more deeply the ecosystems and communities that drive TypeScript and JavaScript.
Community Engagement and Support
TypeScript and JavaScript Communities: A Synergy of Talent
The TypeScript and JavaScript communities include a wide variety of enthusiastic developers: hobbyists and open-source contributors to enterprise developers building high-end applications. These communities are the lifeblood of both languages and offer support through forums and social media sites, conferences and local meetups.
- Online Forums and Platforms: Places like Stack Overflow, GitHub, and Reddit serve as vibrant forums for discussion, problem-solving, and sharing of ideas and resources.
- Conferences and Meetups: Annual conferences such as JSConf, TypeScriptConf, and local meetups around the world offer opportunities for learning, networking, and sharing the latest in JavaScript and TypeScript development.
Libraries, Frameworks, and Tools
A Rich Ecosystem for Developers
Both TypeScript and JavaScript are supported by an extensive array of libraries and frameworks, making it easier for developers to implement complex features, optimize performance, and ensure security.
- JavaScript Libraries and Frameworks: With libraries like React, Vue, and Angular, developers can build dynamic user interfaces and single-page applications more efficiently. Node.js allows JavaScript to run on the server side, enabling full-stack development with the same language.
- TypeScript Enhancements: TypeScript enhances these JavaScript libraries and frameworks by adding type definitions, available through the DefinitelyTyped project, improving developer productivity and code quality.
Continuous Evolution and Updates
Keeping Pace with the Digital World
Both TypeScript and JavaScript are under constant development, with new features, improvements, and bug fixes being regularly introduced. This ensures that they remain at the forefront of web development technology, capable of meeting the demands of modern web applications.
- ECMAScript Standards: JavaScript evolves through ECMAScript (ES) standards, with new specifications bringing in syntactic sugar, new features, and optimizations to make the language more powerful and easier to use.
- TypeScript Releases: TypeScript updates often include improvements to the type system, compiler optimizations, and support for the latest ECMAScript features, ensuring that TypeScript developers can leverage the strengths of JavaScript while benefiting from strong typing and advanced tooling.
The communities and ecosystems for TypeScript and JavaScript drive innovation in the web development field and ensure that both languages are prepared to meet the requirements of modern web application development. From the developer forums and meetups to the regular feature releases and bug fixes that make the languages fresh and powerful, the communities and evolving ecosystems around TypeScript and JavaScript are what have made them successful and popular.
TypeScript vs JavaScript: Conclusion
Our study of the TypeScript vs JavaScript landscapes has shown that the real power is not in choosing one over the other but in understanding and leveraging both strengths. These two languages emerge not as rivals but as allies which each contribute to the web development process in their very own distinctive way. Static typing in TypeScript provides structure and scalability to large projects and is a strong recommendation for enterprise-level applications. JavaScript, long praised for its fluidity and dynamism, is good for fast prototyping and small projects.
Whether you make use of JavaScript vs TypeScript is dependent upon the project requirements, the skills of your development group, and your organizational or personal tastes. Picking out the language that best fits the job can make an impact in how your development projects go.
At this time, it must also be mentioned that having a team prepared to navigate both languages can be life changing. And that’s where WireFuture comes in. Whether you need to hire JavaScript developer who creates compelling user experiences or hire TypeScript developer who adds order and precision to complex applications, WireFuture can connect you to top talent. Our developers are native to both languages and know how TypeScript and JavaScript can complement one another to power your project.
TypeScript vs. JavaScript FAQ
TypeScript is built on top of JavaScript. It includes all JavaScript features and adds its own syntax for type declarations and annotations. This means any valid JavaScript code is also valid TypeScript code.
The key difference is that TypeScript offers static typing, while JavaScript is dynamically typed. This means TypeScript allows developers to define the types of their variables, function parameters, and return values, providing compile-time type checking and error detection.
TypeScript is ideal for developers working on large-scale applications where codebase maintainability and scalability are critical. Its type system helps catch errors early, simplifying debugging and improving code quality.
JavaScript is perfect for smaller projects, quick prototypes, or projects where the development environment is highly dynamic. Its flexibility and the fact that it’s supported natively by all modern web browsers make it a universal choice for web development.
Yes, TypeScript compiles down to JavaScript, allowing you to use both languages within the same project. This can be particularly useful for gradually migrating a JavaScript project to TypeScript or for incorporating TypeScript into parts of a project where static typing is beneficial.
For JavaScript, popular frameworks include React, Vue.js, and Angular.
TypeScript is often used with these same frameworks, thanks to its interoperability with JavaScript. React, Angular, and Vue.js all have TypeScript definitions, allowing for seamless integration.
Dream big, because at WireFuture, no vision is too ambitious. Our team is passionate about turning your software dreams into reality, with custom solutions that exceed expectations.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.