Understanding SOLID Principles For Better Coding
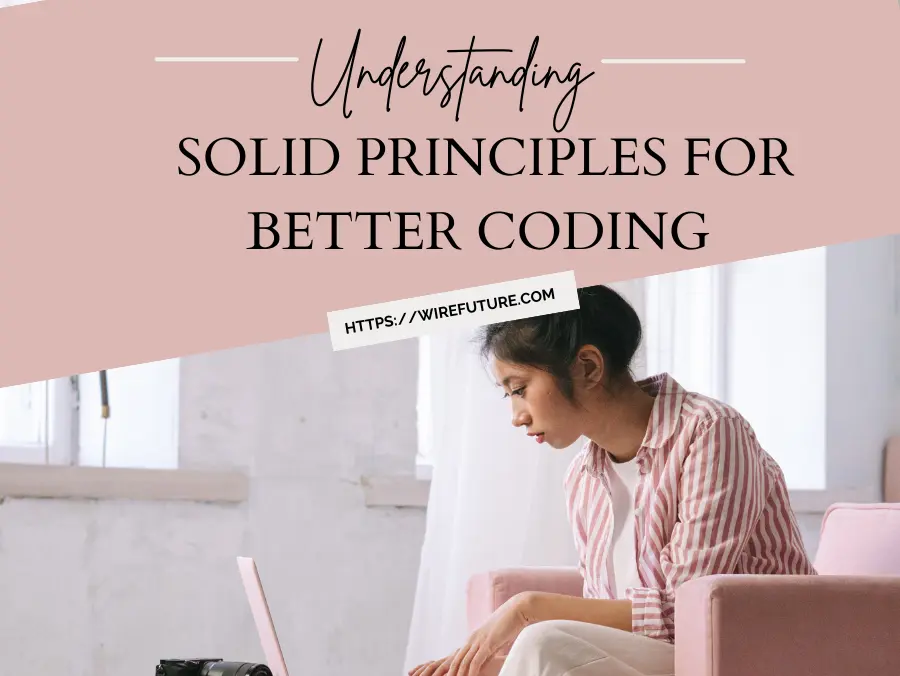
Clean and effective code is a universal goal in software development. Among the approaches and practices aimed at this goal, the SOLID principles are a primary foundation of modern software development, especially when using object oriented languages like C#. Established by Robert C. Martin in the early 2000s, these principles outline a methodology for writing simpler, easier to use and maintainable software.
Table of Contents
- Overview of SOLID Principles
- Importance of SOLID Principles in Software Development
- S – Single Responsibility Principle (SRP)
- O – Open/Closed Principle (OCP)
- L – Liskov Substitution Principle (LSP)
- I – Interface Segregation Principle (ISP)
- D – Dependency Inversion Principle (DIP)
- Conclusion
- Frequently Asked Questions about SOLID Principles
Overview of SOLID Principles
The SOLID acronym is a mnemonic device that helps developers remember five fundamental principles that aim to reduce dependencies, decouple components, and make software easier to modify and scale. These principles are:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Each principle addresses a specific aspect of software design and, when combined, they provide a comprehensive approach to building robust systems.
Importance of SOLID Principles in Software Development
The adoption of SOLID principles is important for several reasons. For starters, they inspire developers to write software that can be managed and scaled over time and can be readily adapted without a complete overhaul. This is especially critical in the agile world where agility to customer demands is critical. Second, these principles make code better, and the chance of bugs and issues which can happen in tightly coupled and interdependent systems are less. By fostering a design that encourages decoupling concerns developers can be more productive and save time debugging and maintaining code. If you want to hire full stack developers who expertly navigates both front-end and back-end technologies, ensuring your projects are built with efficiency and scalability, consider partnering with a team that stands at the forefront of modern development practices.
How SOLID Principles Enhance Maintainability, Flexibility, and Scalability of Code
- Maintainability: Each principle contributes to easier maintenance by isolating responsibilities, which simplifies understanding the system and making changes or updates. For instance, adhering to the Single Responsibility Principle means that each class in a system has only one reason to change, reducing the impact of modifications.
- Flexibility: By following the Open/Closed Principle, systems are built on abstractions that can be extended without modifying existing code, thus allowing for new features to be added with minimal disruption.
- Scalability: Dependency Inversion and Liskov Substitution principles play a direct role in ensuring that systems can grow. By relying on abstractions rather than concrete implementations, software is less brittle and more open to expansion.
S – Single Responsibility Principle (SRP)
Definition
Fundamentally, the Single Responsibility Principle (SRP) states that a class has only one reason to change. This is a fundamental principle of the SOLID principles and lessens complexity and dependencies in the code. By defining only one responsibility or functionality for each class, the SRP ensures that each individual piece of the software performs a single function.
Example In C#
Consider a class in a typical C# application named UserProfileManager that loads user data from the database, updates user information in the database and even formats user data for display. This design violates the SRP due to the fact that the class is given more than one operation.
Here’s how you could refactor this:
Original Class:
public class UserProfileManager
{
public User GetUser(int userId)
{
// Code to retrieve user from database
}
public void UpdateUser(User user)
{
// Code to update user in the database
}
public string DisplayUserInfo(User user)
{
// Code to format user info for display
}
}
Refactored Code:
Splitting UserProfileManager
into three separate classes, each with its own responsibility.
public class UserDataAccess
{
public User GetUser(int userId)
{
// Code to retrieve user from database
}
public void UpdateUser(User user)
{
// Code to update user in the database
}
}
public class UserInfoFormatter
{
public string DisplayUserInfo(User user)
{
// Code to format user info for display
}
}
In this refactored example, UserDataAccess
is responsible solely for database interactions, while UserInfoFormatter
takes care of formatting the user information for display. This separation makes each class simpler and adheres to the SRP.
Benefits Of Single Responsibility Principle
Adhering to the SRP offers several key benefits:
- Easier Maintenance: Changes in the way user information is displayed, for instance, would only require modifications to the
UserInfoFormatter
class, without impacting the data access code. - Reduced Impact of Changes: Since each class has only one reason to change, updates related to specific functionalities are less likely to introduce bugs in unrelated areas.
- Enhanced Modularity: With each class handling a single aspect of the system, the codebase becomes more modular, making it easier to read, understand, and manage.
O – Open/Closed Principle (OCP)
Definition
The Open/Closed Principle is one of the key tenets of the SOLID principles and states that software entities (such as classes, modules, functions, etc.) should be open for extension but closed for modification. This principle aims to promote software scalability and maintainability by allowing behaviors to be added without changing existing source code, thereby reducing the risk of breaking existing functionality.
Example in C#
To illustrate the OCP in C#, let’s consider a simple example involving a report generation system. Initially, the system can generate a standard text report, but as the application evolves, there’s a need to add functionality for generating reports in other formats like XML and JSON without altering the existing codebase.
Here’s how this can be accomplished using abstraction and inheritance:
Base Class:
public abstract class ReportGenerator
{
public abstract string GenerateReport();
}
Concrete Implementation:
public class TextReportGenerator : ReportGenerator
{
public override string GenerateReport()
{
return "Generating text report...";
}
}
Extending with New Formats:
public class XmlReportGenerator : ReportGenerator
{
public override string GenerateReport()
{
return "Generating XML report...";
}
}
public class JsonReportGenerator : ReportGenerator
{
public override string GenerateReport()
{
return "Generating JSON report...";
}
}
In this example, the ReportGenerator
class is designed to be extended without modification. When new report formats need to be added, new classes (XmlReportGenerator
and JsonReportGenerator
) are created inheriting from ReportGenerator
. This setup adheres to the OCP by allowing new functionalities (new report types) to be added without modifying the existing codebase.
Benefits Of Open/Closed Principle
- Enhanced System Flexibility: The system can easily adapt to new requirements or changes in the environment, as new functionalities can be added with minimal risk to the existing system operations.
- Reduced Risk of Bugs: Since existing code remains unmodified when extending functionality, there is a lower chance of introducing bugs into previously tested and proven code.
- Easier Maintenance and Scalability: Adding new features doesn’t require altering existing code, which simplifies maintenance and testing. The system can grow more seamlessly and sustainably.
L – Liskov Substitution Principle (LSP)
Definition
The Liskov Substitution Principle (LSP), named after Barbara Liskov, who introduced it in a 1987 conference keynote, posits that objects of a superclass should be replaceable with objects of its subclasses without affecting the functioning of the program. This principle is crucial for ensuring that a software system’s design remains consistent and predictable when extended through inheritance.
Example in C#
To illustrate LSP in action and highlight the consequences of violating it, consider a class hierarchy where a base class and several derived classes represent different types of birds:
Base Class:
public class Bird
{
public virtual void Fly()
{
Console.WriteLine("This bird flies");
}
}
Derived Class:
public class Sparrow : Bird
{
public override void Fly()
{
Console.WriteLine("Sparrow flies short distances");
}
}
Another Derived Class (Potentially Problematic):
public class Ostrich : Bird
{
public override void Fly()
{
throw new NotImplementedException("Ostriches cannot fly");
}
}
In this scenario, substituting a Sparrow
object with a Bird
object works seamlessly, adhering to the LSP. However, replacing a Bird
with an Ostrich
leads to runtime errors if the Fly
method is called, violating the LSP. The Ostrich
class should not inherit from Bird
if Bird
implies the ability to fly.
A better approach would be to restructure the inheritance hierarchy:
public abstract class Bird
{
// Common bird functionality
}
public interface IFlyingBird
{
void Fly();
}
public class Sparrow : Bird, IFlyingBird
{
public void Fly()
{
Console.WriteLine("Sparrow flies short distances");
}
}
public class Ostrich : Bird
{
// Ostrich-specific functionality
}
In this revised design, only birds that can fly implement the IFlyingBird
interface. This arrangement adheres to LSP by ensuring that Fly
is only expected where it is logically applicable.
Benefits Of Liskov Substitution Principle
- Code Robustness: By adhering to LSP, the software is less prone to runtime errors due to type incompatibilities, leading to more robust applications.
- Improved Code Correctness: LSP helps maintain the correctness of the program by ensuring that classes derived from the same base class are usable through interfaces of their base class without needing to know about their specific implementations.
- Enhanced Maintainability: Following LSP facilitates easier maintenance and expansion of the system since new subclasses can be added with confidence that they will integrate seamlessly without side effects.
I – Interface Segregation Principle (ISP)
Definition
The Interface Segregation Principle (ISP) dictates that no client should be forced to depend on methods it does not use. This principle aims to reduce the side effects and frequency of required changes by splitting large interfaces into smaller and more specific ones that clients will only need to know about the interfaces that are relevant to their operation. To implement Interface Segregation effectively and ensure your software architecture is optimally designed, hire from WireFuture, where our .NET development services specialize in creating clean, modular, and scalable solutions.
Example in C#
Consider a scenario in a software application where a multi-function printer needs to support operations like printing, scanning, and faxing. Initially, one might design a single interface to encompass all these functionalities. However, this design would force the implementation of all interface methods even if a particular model does not support all the functionalities.
Original Interface:
public interface IMultiFunctionDevice
{
void Print(Document d);
void Scan(Document d);
void Fax(Document d);
}
Segregated Interfaces:
Splitting the large interface into smaller, more specific interfaces helps adhere to ISP:
public interface IPrinter
{
void Print(Document d);
}
public interface IScanner
{
void Scan(Document d);
}
public interface IFax
{
void Fax(Document d);
}
Implementation:
For devices that support only specific functionalities, you can implement only those interfaces that are necessary:
public class SimplePrinter : IPrinter
{
public void Print(Document d)
{
Console.WriteLine("Print document");
}
}
public class PhotoCopier : IPrinter, IScanner
{
public void Print(Document d)
{
Console.WriteLine("Print document");
}
public void Scan(Document d)
{
Console.WriteLine("Scan document");
}
}
This design approach ensures that each device class only needs to implement the functionalities it requires, without being burdened by the unused methods of a fat interface.
Benefits Of Interface Segregation Principle
- Reduced Impact of Changes: By segregating interfaces, changes to a particular action (like updating scanning technology) do not affect classes that only implement printing.
- Enhances Class Cohesion: Classes that implement these smaller interfaces are more cohesive and easier to maintain since they only contain logic for the features they actually support.
- Easier to Understand and Implement: Developers can pick and choose which interfaces to implement based on the requirements of the specific device they are working on, making the system easier to understand and extend.
The Interface Segregation Principle plays a crucial role in developing maintainable software by ensuring that classes are not forced to implement interfaces they do not use, thus promoting system modularity and reducing the complexity of updates.
D – Dependency Inversion Principle (DIP)
Definition
The Dependency Inversion Principle is a fundamental tenet of the SOLID principles that addresses dependencies among the modules of a software system. According to DIP, high-level modules (those implementing business rules or logic) should not depend on low-level modules (those performing basic operations or interactions). Instead, both should depend on abstractions (e.g., interfaces or abstract classes). This principle serves to decouple the modules of the system, facilitating easier maintenance and scalability.
Example in C#
To illustrate DIP, consider a C# application where a service component needs to access a data repository. Initially, the high-level service class directly depends on a specific low-level repository class, leading to a tightly coupled system.
Highly Coupled Code:
public class CustomerService
{
private CustomerRepository repository = new CustomerRepository();
public Customer GetCustomer(int id)
{
return repository.GetCustomerById(id);
}
}
public class CustomerRepository
{
public Customer GetCustomerById(int id)
{
// Access database to retrieve customer
return new Customer();
}
}
Refactoring to Use Dependency Injection:
Transforming the design to use dependency injection involves defining an abstraction for the repository and injecting it into the service.
public interface ICustomerRepository
{
Customer GetCustomerById(int id);
}
public class CustomerService
{
private ICustomerRepository repository;
public CustomerService(ICustomerRepository repo)
{
repository = repo;
}
public Customer GetCustomer(int id)
{
return repository.GetCustomerById(id);
}
}
public class CustomerRepository : ICustomerRepository
{
public Customer GetCustomerById(int id)
{
// Access database to retrieve customer
return new Customer();
}
}
In this refactored example, CustomerService
no longer directly depends on the concrete CustomerRepository
. Instead, it interacts with the ICustomerRepository
interface, allowing for any suitable implementation to be injected. This design adheres to DIP and increases the modularity and testability of the application by allowing different repository implementations to be easily swapped or mocked for testing.
The Dependency Inversion Principle is crucial to designing maintainable and scalable systems. DIP enables systems to evolve more flexibly and autonomously by separating high-level business logic from the low-level implementation details. Thus software is simpler to maintain, extend and test, with components that can be independently developed and enhanced without affecting any others. This architectural pattern supports good software practices and is compatible with current development environments and frameworks that encourage modularity and dependency management.
Conclusion
Following the SOLID principles is not only a practice but a philosophy that drives good software development. By implementing Single Responsibility Principle, Open/Closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle developers can develop robust, maintainable and scalable systems. These principles help you write easier to debug, extend and manage software – an important role in today’s rapidly changing tech landscape.
For those looking to implement these practices within the .NET framework, choosing the right partner can make all the difference. WireFuture, a dedicated .NET Development Company, stands ready to bring these principles to life in your projects. Our expertise ensures that your software not only meets the highest standards of quality but also delivers sustainable business value. Let us help you transform your development approach with SOLID principles at the core.
Frequently Asked Questions about SOLID Principles
SOLID principles are a set of guidelines aimed at improving software design and architecture to make it more understandable, flexible, and maintainable. They consist of the Single Responsibility Principle (SRP), Open/Closed Principle (OCP), Liskov Substitution Principle (LSP), Interface Segregation Principle (ISP), and Dependency Inversion Principle (DIP).
Implementing SOLID principles helps in reducing code dependencies, enhancing code reusability, and making the system easier to refactor and scale. This not only increases the robustness and reliability of the software but also simplifies the management of future code changes and enhancements.
Yes, while examples often use object-oriented languages like C# or Java due to their inherent support for principles like inheritance and polymorphism, the concepts underlying the SOLID principles can be adapted to other programming paradigms with thoughtful design.
While the benefits are significant, over-implementation of SOLID principles can sometimes lead to increased complexity, with too many layers and abstractions that might complicate the system rather than simplify it. It’s crucial to balance the application of these principles based on the project’s needs.
WireFuture offers expert .NET development services that embrace SOLID principles to ensure your projects are robust, maintainable, and scalable. Our team specializes in crafting tailored solutions that adhere to these advanced design principles, helping your business stay agile and efficient in a competitive landscape.
Success in the digital age requires strategy, and that's WireFuture's forte. We engineer software solutions that align with your business goals, driving growth and innovation.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.