What is Reflection in C#? Unlocking Dynamic Capabilities
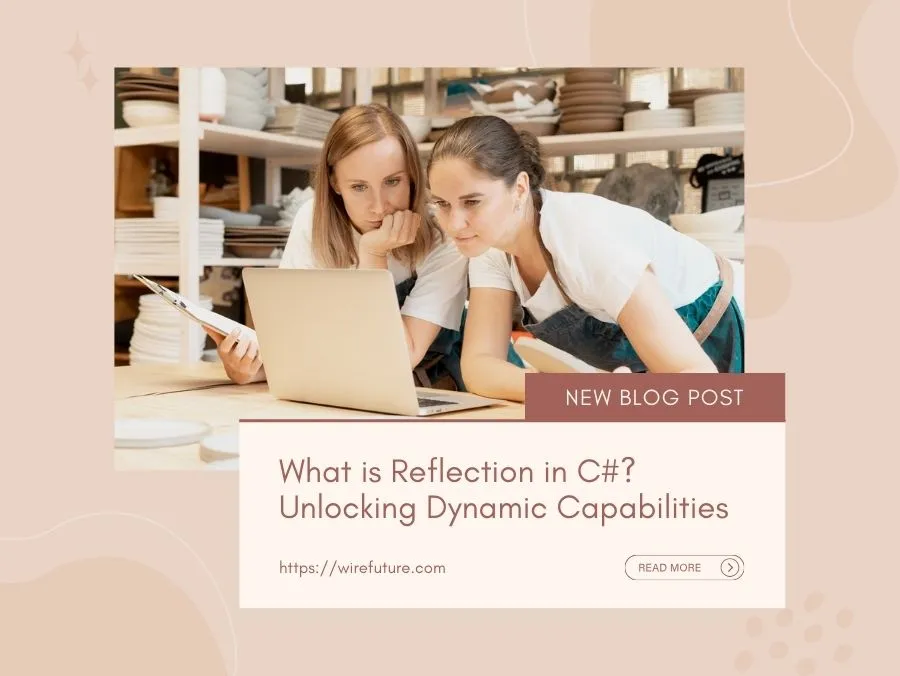
Reflection is a powerful runtime mechanism for inspecting and manipulating a program’s own structure. Reflection enables dynamic access to metadata and the type system, and is the backbone of many advanced .NET features such as serialization, dependency injection, and dynamic proxies.
Brief Definition of What Is Reflection:
Reflection allows for programmatic manipulation of the assembly metadata and types, methods, properties and fields. This involves creating objects, calling methods, and accessing fields and properties at runtime without knowing the assembly structure.
The Role of Reflection in Dynamic Code Execution:
For situations where code flexibility and reusability are critical, Reflection is critical. It allows programmers to write applications that load and execute new code paths depending on an external configuration or plugin without recompiling the application. This makes Reflection an indispensable tool for designing dynamically reconfigurable, extensible, and modular applications that respond to dynamically changing requirements or environment. Any proficient Web Development Company will utilize these capabilities of Reflection to ensure that their clients’ applications are not only robust but also versatile and forward-thinking in their design.
With Reflection developers can abstract their applications deeply while maintaining a low level of tight coupling between components, thereby making their applications more maintainable and scalable. Let us understand Reflection in C# in depth with several examples.
Table of Contents
- Core Concepts of Reflection
- Using Reflection to Inspect Assemblies
- Manipulating Objects Through Reflection
- Practical Applications of Reflection
- Performance Considerations
- Security Implications of Using Reflection
- Reflection in C# Advanced Techniques
- Conclusion
Core Concepts of Reflection
Reflection in C# revolves around several key concepts and classes that are part of the System.Reflection
namespace. This namespace contains types that provide a way to obtain information about assemblies, modules, members, parameters, and other entities in managed code.
Understanding the System.Reflection
Namespace: The System.Reflection
namespace plays a vital role in accessing and manipulating the types stored in a managed assembly. It enables the inspection of an assembly’s metadata to discover classes, interfaces, structures, methods, and fields at runtime.
Key Classes Involved:
Type – Represents type declarations: class types, interface types, array types, value types, enumeration types, type parameters, generic type definitions, and open or closed constructed generic types.
- Example: Using
Type.GetType("System.Int32")
retrieves the type object representing theint
data type in C#.
MethodInfo – Represents a method of a class. This class can be used to invoke methods or get information about method parameters.
- Example:
Type type = typeof(String);
MethodInfo substringMethod = type.GetMethod("Substring", new[] { typeof(int), typeof(int) });
string result = (string)substringMethod.Invoke("Hello, world!", new object[] { 7, 5 });
PropertyInfo – Provides access to property metadata. You can use this class to get or set property values, as well as to get the attributes of a property.
- Example:
Type type = typeof(DateTime);
PropertyInfo dayProperty = type.GetProperty("Day");
DateTime today = DateTime.Now;
int day = (int)dayProperty.GetValue(today);
FieldInfo – Allows access to fields of a class. Using this class, one can retrieve or set values directly on fields.
- Example:
Type type = typeof(ConsoleColor);
FieldInfo blackFieldInfo = type.GetField("Black");
object value = blackFieldInfo.GetValue(null); // Since 'Black' is a static field
Each of these classes provides methods that allow developers to create instances of types, call methods, get or set property values, and access fields dynamically, all of which can be done without knowing the types at compile time.
Using Reflection to Inspect Assemblies
Reflection provides mechanisms to load and inspect assemblies, which are collections of types and resources built as a single unit of deployment in a .NET application. This capability is crucial for applications that need to load modules at runtime or analyze their contents programmatically. Utilizing these features is a fundamental aspect of .NET development services, enabling developers to offer highly customizable and scalable solutions tailored to meet diverse and evolving business needs.
How to Load and Examine Assemblies: Assemblies can be loaded into an application domain from different sources, such as from a file on disk or from a byte array. The Assembly
class in the System.Reflection
namespace provides methods to load assemblies.
// Loading an assembly from a file
Assembly assembly = Assembly.LoadFile(@"path\to\your\assembly.dll");
// Displaying basic information about the assembly
Console.WriteLine($"Fully Qualified Name: {assembly.FullName}");
This loaded assembly can then be examined to retrieve information about its contents, such as metadata about the types defined in the assembly, the resources it includes, and other attributes.
Exploring Types Within an Assembly: Once an assembly is loaded, you can explore its types. This includes examining classes, interfaces, enums, and other components defined in the assembly.
// Enumerating all types in the loaded assembly
Type[] types = assembly.GetTypes();
foreach (Type type in types) {
Console.WriteLine($"Type: {type.Name}");
}
This functionality is essential for applications that need to discover and utilize types dynamically, such as when creating plugin systems where the plugins are not known at compile time.
Accessing Attributes and Their Values: Attributes in .NET provide a powerful way to add metadata to assemblies, types, methods, and other code elements. Reflection allows you to inspect these attributes.
// Accessing custom attributes of an assembly
object[] attributes = assembly.GetCustomAttributes(false);
foreach (object attribute in attributes) {
Console.WriteLine($"Attribute: {attribute.GetType().Name}");
}
// Example of retrieving a specific attribute
if (assembly.IsDefined(typeof(AssemblyTitleAttribute), false)) {
AssemblyTitleAttribute titleAttribute = (AssemblyTitleAttribute)assembly.GetCustomAttribute(typeof(AssemblyTitleAttribute));
Console.WriteLine($"Assembly Title: {titleAttribute.Title}");
}
Manipulating Objects Through Reflection
Reflection not only allows you to inspect the types and their components in an assembly, but it also provides the capability to dynamically create instances, invoke methods, and modify properties or fields. This is particularly useful in scenarios where the type information is not available until runtime.
Creating Instances of Types Dynamically: Using Reflection, you can instantiate classes dynamically by obtaining the Type
object of the class and then calling the Activator.CreateInstance
method.
// Dynamically creating an instance of a class
Type listType = typeof(List<>);
Type specificListType = listType.MakeGenericType(typeof(string));
object listInstance = Activator.CreateInstance(specificListType);
Console.WriteLine($"Created instance type: {listInstance.GetType().Name}"); // Outputs: List`1
This method is crucial for creating objects when their types are determined at runtime, such as loading types from external assemblies or implementing factory patterns.
Invoking Methods and Accessing Properties at Runtime: Reflection allows you to invoke methods and access properties on objects without knowing their types at compile time.
// Invoking a method
MethodInfo addMethod = specificListType.GetMethod("Add");
addMethod.Invoke(listInstance, new object[] { "Hello Reflection!" });
// Accessing a property
PropertyInfo countProperty = specificListType.GetProperty("Count");
int count = (int)countProperty.GetValue(listInstance);
Console.WriteLine($"Items in list: {count}"); // Outputs: Items in list: 1
Modifying Field Values Without Knowing the Types at Compile Time: Reflection enables you to modify the field values of an object dynamically, which is particularly useful for applications that need to alter their behavior based on runtime conditions.
// Modifying fields
Type randomType = typeof(Random);
Random randomInstance = new Random();
FieldInfo seedArrayField = randomType.GetField("_seedArray", BindingFlags.NonPublic | BindingFlags.Instance);
if (seedArrayField != null) {
int[] seedArray = (int[])seedArrayField.GetValue(randomInstance);
seedArray[0] = 123456; // Altering the private field value
seedArrayField.SetValue(randomInstance, seedArray);
Console.WriteLine($"Modified field value");
}
Practical Applications of Reflection
Reflection is more than a theoretical construct, it is an actual utility that provides a great flexibility and functionality to .NET development company that develop applications in .NET: Here are some of the key practical applications:
Use Cases for Dependency Injection Frameworks:
Dependency injection (DI) frameworks such as ASP.NET Core’s native DI container leverage Reflection. These frameworks leverage Reflection to discover service types and their dependencies automatically without hard coding the objects that need to be instantiated.
For example:
Reflection in DI frameworks scans assemblies, finds classes annotated with specified interfaces or attributes and creates dynamically instance of those classes at runtime. This is fundamental for app dependencies management so that code can be written that is cleaner, more modular and testable.
Dynamic Type Usage Examples: Plugins/Script Engines:
In applications where design approaches are modular, such as plugin architectures or script engines, reflection is essential. It enables the main application to load, inspect and execute code from external assemblies dynamically.
For example:
Choose a graphics editor that lets you add plugins for additional image effects. Reflection enables the software to load plugin assemblies at runtime, find classes that implement a specific plugin interface, and instantiate these dynamically, allowing the software to expand its capabilities without changing its core.
Designing Flexible and Extensive Applications:
Reflection allows lightweight and extensible applications to be built. It enables features such as runtime configuration changes or condition-based behavior adjustments.
For example:
In enterprise applications, you might have to load extra behaviors or configurations depending on the deployment environment (development, production, staging, etc.). Reflection can dynamically load components or settings from external configuration files or databases to make the application more flexible and less likely to require multiple builds. This adaptability is particularly beneficial in Cloud and DevOps services, where automated deployment processes and environment-specific configurations are critical for maintaining continuous integration and continuous deployment (CI/CD) workflows.
Building Better Development Tools and Frameworks:
Building development tools and frameworks also requires reflection. As an example, object relational mapping (ORM) frameworks like Entity Framework use Reflection to map database data to domain items without having the developer writing boilerplate code.
For example:
Reflection is an ORM tool that finds properties of domain model classes and maps those properties to corresponding columns in a database. This makes it easier for developers to interact with database entities by treating them like regular C # objects.
Unit Tests – Dynamic Testing & Mocking:
In unit testing frameworks, reflection is widely used to call methods dynamically, access internal members, and modify class behavior during testing. This is important when testing private methods or properties without making them public.
For example:
Reflection is also used by testing frameworks such as NUnit or Moq to generate mock objects and to call private or protected members during tests. This is critical for thorough testing, allowing developers to keep encapsulation while still maintaining code robustness.
Performance Considerations
While Reflection is a powerful feature in C#, it comes with certain performance implications that developers need to be aware of. Understanding these can help in optimizing applications that rely on Reflection.
Understanding the Impact of Using Reflection on Performance: Reflection can be slower than direct code execution because it involves investigating metadata and dynamically invoking members, which can introduce overhead. This overhead is primarily due to the extra steps the runtime has to take to resolve type information, access members, and ensure security checks.
- Example: Comparing the time taken to directly invoke a method versus using Reflection shows that Reflection can be significantly slower. For instance, directly calling a method might take a few nanoseconds, whereas invoking the same method via Reflection might take several microseconds.
Best Practices for Minimizing Performance Overhead: To mitigate the performance overhead associated with Reflection, several best practices can be followed:
- Cache Reflective Results: Frequently accessed type information, such as
MethodInfo
orType
objects, should be cached to avoid repeated costly lookup operations.- Example: Store the
MethodInfo
objects in a dictionary if you need to repeatedly invoke the same method via Reflection.
- Example: Store the
- Use Lightweight Reflection Alternatives: For some operations, lighter alternatives are available. For instance,
TypeDescriptor
can be used for property access instead ofPropertyInfo
, as it is optimized for component-based designs and might cache information more aggressively. - Limit Scope and Frequency: Restrict the use of Reflection to initialization or infrequent operations instead of regular use within performance-critical loops.
When to Use and When to Avoid Reflection: Knowing when to employ Reflection and when to seek alternatives is crucial for maintaining good performance and clean code architecture.
- When to Use Reflection:
- Dynamic Behavior: When building applications that require dynamic behavior that cannot be determined at compile time, such as plugin systems or dynamically loaded modules.
- Complex Configurations: In scenarios where application behavior needs to be extensively configurable at runtime beyond what standard configuration files offer.
- When to Avoid Reflection:
- Performance-Critical Paths: Avoid using Reflection in loops or performance-critical sections of code where execution speed is paramount.
- Simple Tasks: For simple tasks where direct code paths are available and sufficient, avoid Reflection to keep the code simpler and more maintainable.
- Example of Avoiding Reflection: Instead of using Reflection to call a method that is known at compile time, directly call the method or use delegates. For instance, using delegates to invoke methods can be nearly as fast as direct calls and much faster than Reflection.
Security Implications of Using Reflection
Reflection, by allowing dynamic code execution and manipulation, can introduce several security risks and vulnerabilities. Understanding these risks and how to mitigate them is crucial for developing secure applications.
Potential Security Risks and Vulnerabilities: Reflection increases the attack surface of an application by enabling dynamic execution paths that could be exploited if not properly secured. The risks include:
- Exposure of Sensitive Data: Reflection can be used to access private fields and methods, potentially exposing sensitive data that should not be accessible outside of the class.
- Unintended Code Execution: Malicious use of Reflection can lead to execution of unintended code paths or methods, which can compromise application integrity.
- Bypassing Access Controls: Since Reflection can modify virtually any aspect of a program’s execution state, it can be used to bypass security checks or access controls, leading to privilege escalation.
Mitigating Security Issues While Using Reflection: To protect against the risks posed by Reflection, developers should adopt the following security practices:
- Restrict Reflection Usage: Limit the use of Reflection to the necessary parts of the application and avoid its use in exposed or security-critical areas.
- Use Strong Access Controls: Implement runtime checks to ensure that only authorized users are allowed to perform operations that involve Reflection.
- Validate Inputs Rigorously: Since Reflection can dynamically execute code based on input, ensure that all inputs are validated to prevent injection attacks.
- Use Security Annotations: Leverage security annotations (like
[SecuritySafeCritical]
) to mark safe Reflection operations, helping maintain clear security boundaries.
Reflection and Code Access Security: Code Access Security (CAS) in .NET allows for specifying what resources a program is allowed to access. Reflection can interact with CAS by demanding certain permissions before executing, thus providing a layer of security.
- Using Permission Demands: Before performing sensitive operations via Reflection, demand the necessary permissions using CAS. This ensures that only code with appropriate rights can use potent Reflection capabilities.
MethodBase method = typeof(SecretClass).GetMethod("SensitiveMethod");
// Demand that the caller has the permission to call this method
method.Invoke(new PermissionSet(PermissionState.Unrestricted), null);
Audit and Monitoring: Given the potential for abuse, it’s advisable to audit and monitor the use of Reflection in your applications. This involves logging Reflection access patterns and reviewing them for any unusual or unauthorized activities.
Reflection in C# Advanced Techniques
Reflecting on Generic Types and Methods: Working with generics via Reflection can be tricky due to the need to handle type parameters and constraints dynamically.
- Example: Creating a Generic Type Instance Dynamically:
// Create a generic type of List<>
Type genericListType = typeof(List<>);
// Specify the type parameter
Type specificListType = genericListType.MakeGenericType(typeof(string));
// Create an instance of this type
object listInstance = Activator.CreateInstance(specificListType);
Console.WriteLine(listInstance.GetType()); // Outputs: System.Collections.Generic.List`1[System.String]
- Example: Invoking a Generic Method:
// Define a method that takes a generic type parameter
MethodInfo openGenericMethod = typeof(Program).GetMethod("GenericMethod");
// Make the method generic with a specific type
MethodInfo closedGenericMethod = openGenericMethod.MakeGenericMethod(typeof(int));
// Invoke the generic method
closedGenericMethod.Invoke(null, new object[] { 42 });
Handling Exceptions and Errors in Reflection Operations: Errors in Reflection can arise from incorrect type handling, missing members, security issues, etc. Proper exception handling is critical.
- Example: Safely Invoking a Method with Exception Handling:
try {
Type type = typeof(SampleClass);
object instance = Activator.CreateInstance(type);
MethodInfo method = type.GetMethod("MethodThatMightFail");
method.Invoke(instance, null);
} catch (TargetInvocationException tie) {
Console.WriteLine($"Exception thrown by called method: {tie.InnerException.Message}");
} catch (Exception ex) {
Console.WriteLine($"Reflection operation failed: {ex.Message}");
}
Using Expression Trees to Optimize Reflection Tasks: Expression Trees can be used to compile parts of what would be a Reflection operation into directly executable code, drastically reducing overhead in repeated operations.
- Example: Using Expression Trees to Set a Property Value:
// Target property and value
var target = new SampleClass();
string propertyName = "Name";
string valueToSet = "John Doe";
// Get property info
PropertyInfo propertyInfo = target.GetType().GetProperty(propertyName);
// Create the expression to set the property
var parameter = Expression.Parameter(typeof(string), "value");
var property = Expression.Property(Expression.Constant(target), propertyInfo);
var assignment = Expression.Assign(property, parameter);
// Compile and run the expression
var setter = Expression.Lambda<Action<string>>(assignment, parameter).Compile();
setter(valueToSet);
Console.WriteLine(target.Name); // Outputs: John Doe
- Example: Optimizing Method Invocation Using Expression Trees:
MethodInfo methodInfo = typeof(SampleClass).GetMethod("Echo");
var instance = Expression.Constant(new SampleClass());
var argument = Expression.Constant("Hello, world!");
// Create method call expression
var callExpression = Expression.Call(instance, methodInfo, argument);
// Compile and execute
var echo = Expression.Lambda<Func<string>>(callExpression).Compile();
Console.WriteLine(echo()); // Outputs: Hello, world!
Conclusion
Reflection in C# is a unique combination of power and agility allowing developers to inspect, modify and interact with their code in a dynamic and runtime-efficient manner. It enables applications that are resilient and scalable to changing requirements while also being able to easily leverage external code and configurations. From the examples and discussions given herein, Reflection is a must for any advanced .NET development, supporting everything from simple dynamic type generation to more advanced operations such as dependency injection and runtime metadata access.
If your projects need such advanced, flexible .NET solutions, partner with a team with expertise in this area. Hire ASP.NET Core developers from WireFuture that can help you implement these advanced features in your applications quickly and effectively. Our team is experienced in leveraging Reflection for customized, future-ready solutions.
Our team of software development experts is here to transform your ideas into reality. Whether it's cutting-edge applications or revamping existing systems, we've got the skills, the passion, and the tech-savvy crew to bring your projects to life. Let's build something amazing together!
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.