What is SignalR And How To Integrate it in ASP.NET Core
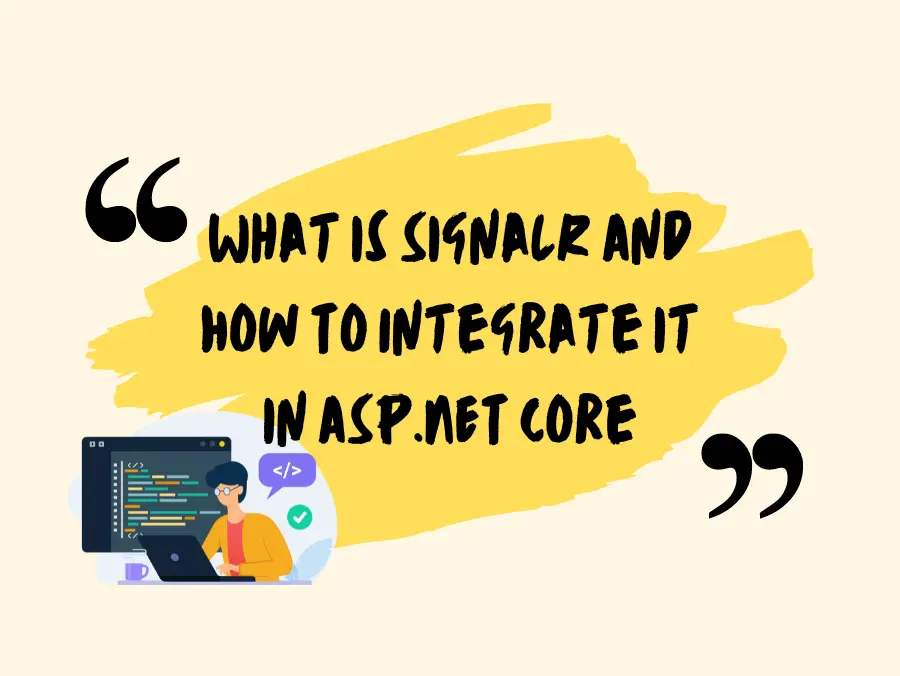
This blog post will help you discover what is SignalR and how to integrate it in ASP.NET Core. So, first we must ask what is SignalR? SignalR is a real time web library in ASP.NET Core. This means it allows an interactive session of interaction between the browser of the user and the server. What is unique about SignalR is that server side code can push content updates instantly to connected clients without having to hold out for the client to request it occasionally.
This is crucial when creating web applications which call for real-time data updates like chat applications, live content feeds or interactive gaming environments. With SignalR, developers can rest assured the application’s UI is responsive and fluid, delivering an experience as fast as desktop applications.
SignalR achieves this real time data push by abstracting many transport mechanisms. They are WebSockets, Server Sent Events (SSE) and Long Polling based on how much the client browser supports. This scalability enables SignalR to offer real-time functionality on a variety of platforms and devices – from contemporary web browsers to mobile devices and desktop applications – without needing to incorporate these advanced solutions right into the application code.
The necessity of SignalR in contemporary web development cannot be overstated. In an electronic world where users expect instantaneous interactions and updates, applications that do not provide real time communication can appear slow and old. SignalR resolves this by allowing applications to provide real time content updates, interactive experiences, and client-server communications. This makes it an invaluable resource for developers creating responsive and interactive web applications that react to present day user demands.
Table of Contents
- Why do we need SignalR?
- Benefits of using SignalR in ASP.NET Core
- How SignalR works
- Integrating SignalR into ASP.NET Core
- Use cases for SignalR
- Best practices for SignalR development
- Wrapping Up
Why do we need SignalR?
Before we integrate SignalR in ASP.NET Core we need to understand why do we need SignalR. SignalR is needed due to the limits of traditional web application architectures where information flow is mostly based on customer initiated requests, like AJAX. For this model the client should occasionally send requests to the server to check for new information. This particular approach, while functional, could cause data update delays, excessive server load because of frequent polling, and an overall less responsive user experience.
SignalR represents a paradigm shift by enabling servers to push updates to clients in real time, without the client having to request updates. This capability is particularly helpful for applications where timely information delivery is crucial. For instance, in chat applications, it delivers messages instantly; in live sports scoring applications, it updates scores whenever modifications occur ; What about collaborative tools it ensures all users see changes and edits in real time.
The impact of SignalR is not restricted to enhancing user experience. Additionally, it decreases server load time by staying away from unnecessary polling. This reduction in unnecessary network traffic and server load can result in more scalable applications that can support much more simultaneous connections without sacrificing performance.
Furthermore, SignalR is not just limited to web applications; additionally, it extends its usage to mobile devices and desktop applications. This cross-platform support means real time abilities can be embedded into any application, making SignalR a versatile tool in a developer’s toolbox.
Essentially, SignalR deals with the technical and experiential drawbacks of traditional web communication strategies, which makes it a crucial technology for developing contemporary, interactive, and efficient web applications which meet the expectations of modern users for immediate and dynamic content.
Benefits of using SignalR in ASP.NET Core
SignalR integration in ASP.NET Core offers numerous advantages for real time application development and performance. The benefits are outlined below :
Simplified Development of Real-Time Applications: SignalR abstracts the complexities of real time communication so developers can rapidly add instant messaging, live notifications in addition to real time dashboards with minimal code. It exposes abstract APIs to control underlying connections, groups and messaging, thereby minimizing development effort and time.
Improved User Experience with Instant Updates: SignalR – built applications can provide users with content updates in real time. This real time capability is crucial for applications which require time-sensitive information delivery, like chat applications, live sports feeds or financial trading platforms. It ensures users get updates immediately with no noticeable delay – leading to an immersive and interactive experience.
Reduced Network Traffic Compared to Polling or Constant Refreshes: Traditional content update methods including polling or periodic page refreshes consume network traffic and server load. SignalR performs all communications between server and client efficiently, sending only data when updates can be found. This dramatically decreases bandwidth use and server resources, leading to a far more scalable and performant application.
Scalable Architecture Supporting Thousands of Concurrent Connections: SignalR is a scalable solution, allowing thousands of concurrent connections on one server. This is accomplished by asynchronous programming patterns and efficient connection management. Furthermore, SignalR may be integrated with Azure SignalR service, a fully managed Service which scales to support any number of connections and is optimized for applications with variable load and high real time needs.
With these benefits in mind, developers can develop responsive, performant, and scalable web applications that deliver on the real time interaction and information demands of contemporary users. SignalR in ASP.NET Core thus represents an excellent enhancement to web applications which allows a wide range of real time features that were previously hard to implement.
How SignalR works
SignalR simplifies the creation of real time web applications by utilizing the most recent communication technologies and enabling bi-directional client-server communication. Here’s a deeper dive into how SignalR operates:
Adaptive Communication Protocols: From its core, SignalR dynamically picks the appropriate communication protocol – WebSockets, Server Sent Events (SSE) or Long Polling – based on the client and server abilities. This scalability delivers performance and interoperability for a variety of devices and network conditions. WebSockets offer a full duplex communication channel over a single, long-lived connection and are hence preferred for real time communications. In the absence of WebSockets, SignalR reverts to Long Polling or SSE, keeping real-time functionality.
Permanent Connection Establishment: SignalR keeps a persistent link between the client and server so that both can keep in contact. This persistent connection is the key to offer real-time updates and messages without the client having to request brand new data repeatedly, lowering latency and improving the user experience.
Comprehensive APIs for Server and Client Communication: SignalR offers a variety of APIs for both server-to-client push notifications and client-server messages. This bidirectional messaging capability enables real time interaction ranging from broadcasting messages to all connected clients to sending particular updates to specific users.
Sophisticated Connection Management: Among SignalR’s key features is its automatic connection management. This includes handling reconnections when connection loss happens so users are always up to date without having to do something manually. Furthermore, SignalR provides robust error handling to handle communication – related issues and empowers developers to develop robust applications.
These features combine to provide SignalR with a seamless, real time web application experience. By abstracting the specifics of real time communication, SignalR frees developers to concentrate on building fantastic experiences and new features, rather than examining the details of network protocols and connection management. This makes SignalR a crucial component of the ASP.NET Core framework to help developers to tackle today’s web application demands.
Integrating SignalR into ASP.NET Core
Integrating SignalR in ASP.NET Core involves a few essential steps that leverage both server-side and client-side configurations. Below, you’ll find a detailed guide complemented with code samples to help you set up a basic chat application using SignalR.
Step 1: Install the SignalR Package
First, add the SignalR package to your ASP.NET Core project. Open your terminal or command prompt, navigate to your project directory, and run:
dotnet add package Microsoft.AspNetCore.SignalR
Or, if you prefer using the NuGet Package Manager, search for Microsoft.AspNetCore.SignalR
and install it.
Step 2: Define SignalR Hubs
SignalR hubs serve as the core component in managing communication between clients and the server. Create a new class file named ChatHub.cs
in your project and define the hub like this:
using Microsoft.AspNetCore.SignalR;
using System.Threading.Tasks;
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync("ReceiveMessage", user, message);
}
}
This ChatHub
class inherits from Hub
and defines a SendMessage
method that sends messages to all connected clients.
Step 3: Configure Services and Middleware
In the Startup.cs
file of your ASP.NET Core application, update the ConfigureServices
method to include SignalR services:
public void ConfigureServices(IServiceCollection services)
{
services.AddSignalR();
}
Then, in the Configure
method, map the SignalR hub to a specific path:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapHub<ChatHub>("/chatHub");
});
}
Step 4: Implement Client-Side Code
On the client side, you’ll need to establish a connection to the SignalR hub and handle incoming messages. Assuming you’re working with an HTML page, include the SignalR JavaScript client library:
<script src="https://cdnjs.cloudflare.com/ajax/libs/microsoft-signalr/3.1.7/signalr.min.js"></script>
Here is the full page with SignalR integration:
<!DOCTYPE html>
<html>
<head>
<title>Chat App</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/microsoft-signalr/3.1.7/signalr.min.js"></script>
<style>
.container { max-width: 800px; margin: auto; }
input, button { padding: 10px; }
#messagesList { list-style-type: none; margin: 0; padding: 0; }
li { padding: 8px; margin-bottom: 2px; background-color: #f3f3f3; }
</style>
</head>
<body>
<div class="container">
<h2>Chat Application</h2>
<input type="text" id="userInput" placeholder="Your name..." />
<input type="text" id="messageInput" placeholder="Your message..." />
<button id="sendButton">Send</button>
<ul id="messagesList"></ul>
</div>
<script>
var connection = new signalR.HubConnectionBuilder().withUrl("/chatHub").build();
document.getElementById("sendButton").disabled = true;
connection.on("ReceiveMessage", function (user, message) {
var msg = user + " says " + message;
var li = document.createElement("li");
document.getElementById("messagesList").appendChild(li);
li.textContent = msg;
});
connection.start().then(function () {
document.getElementById("sendButton").disabled = false;
}).catch(function (err) {
return console.error(err.toString());
});
document.getElementById("sendButton").addEventListener("click", function (event) {
var user = document.getElementById("userInput").value;
var message = document.getElementById("messageInput").value;
connection.invoke("SendMessage", user, message).catch(function (err) {
return console.error(err.toString());
});
event.preventDefault();
});
</script>
</body>
</html>
This setup establishes a basic chat application where messages sent from one client are received and displayed to all connected clients in real-time. Integrating SignalR into your ASP.NET Core application not only enhances real-time communication capabilities but also provides a seamless and interactive user experience.
Use cases for SignalR
SignalR with its real time communication capabilities has numerous applications that can enhance interactivity and user experience. Below are in depth explanations of its use cases:
Chat Applications:
- SignalR is a leader in chat application development with instant messaging capabilities.
- It allows rich messaging applications where users can create rooms or groups and get messages in real time without having to refresh their internet browser.
- This is crucial for applications that need group chats, private messaging, and handling a high number of concurrent users.
Real-time Dashboards:
- SignalR’s real time data push capabilities are especially helpful for dashboards that show live data updates, like stock trading platforms, weather apps or sports scores.
- It allows the server to push updates to the dashboard when new data becomes available, enabling users to constantly have the up to date info without refreshing manually.
- This is crucial for decision making in dynamic environments where data changes rapidly.
Online Gaming:
- In online gaming, SignalR can be used to map game state between players to ensure that all players see the same game world.
- It enables the creation of real time, multiplayer games through rapid and secure server-client interaction.
- This synchronization is crucial for competitive and cooperative gaming where latency or game state discrepancies can have a large effect on gameplay.
Collaborative Editing:
- SignalR supports the development of applications where several users can edit documents or projects at the same time.
- SignalR facilitates collaborative work by making changes made by one user visible to all other users working on the document at the same time.
- This is especially crucial in academic, creative, and professional settings where teamwork and collaboration is crucial to productivity.
All these use cases illustrates SignalR’s versatility and strength to allow real time web functionalities that are essential for modern, interactive applications. Whether it’s improving collaboration, activity synchronization, live updates, or communications, SignalR provides a platform for building responsive and UI-friendly web applications.
Best practices for SignalR development
SignalR development, when done with best practices in mind, can significantly enhance the efficiency, reliability, and security of real-time applications. Here are some of these best practices expanded, including code samples where applicable:
Optimize Performance
Optimizing the performance of a SignalR application involves careful management of the data being transmitted to ensure responsiveness and scalability.
Minimize Message Size: Keep the data payloads as small as possible. This might involve sending only the necessary data or changes rather than full objects.
For example, if you’re updating a user’s status, send only the status change rather than the entire user object:
await Clients.All.SendAsync("UpdateStatus", userId, newStatus);
Reduce Frequency of Messages: Implement mechanisms to throttle the number of messages sent over a period, especially in scenarios with rapid state changes.
Consider a scenario where you’re tracking mouse movements in a collaborative drawing app. Instead of sending updates for every pixel change, you could send updates at regular intervals:
let lastUpdateTime = Date.now();
const updateInterval = 100; // milliseconds
document.onmousemove = function(event) {
let now = Date.now();
if (now - lastUpdateTime > updateInterval) {
lastUpdateTime = now;
connection.invoke("SendMousePosition", event.pageX, event.pageY);
}
};
Handle Connection Lifecycle Events Gracefully
Managing connection events is crucial for maintaining a robust and reliable application.
Reconnecting Clients: Implement client-side logic to automatically reconnect in case of disconnection.
connection.onclose(async () => {
await start();
});
async function start() {
try {
await connection.start();
console.log("SignalR Connected.");
} catch (err) {
console.log(err);
setTimeout(start, 5000);
}
}
start();
Secure Communication
Ensuring the security of your SignalR applications is critical to protect data and user privacy.
Authentication: SignalR can use ASP.NET Core’s authentication system. Ensure that your hub methods are accessible only to authenticated users by applying the [Authorize]
attribute.
[Authorize]
public class ChatHub : Hub
{
// Hub methods here
}
Authorization: For more granular control, you can implement custom authorization policies based on user roles or claims.
public class AdminHub : Hub
{
public override Task OnConnectedAsync()
{
if (!Context.User.IsInRole("Admin"))
{
Context.Abort();
}
return base.OnConnectedAsync();
}
}
Monitor and Scale Infrastructure
As your application grows, monitoring and scaling become critical to handle the increased load.
Logging: Implement logging to monitor connection density, message throughput, and error rates. ASP.NET Core’s built-in logging providers can be configured in Startup.cs
.
public void ConfigureServices(IServiceCollection services)
{
services.AddLogging(builder => builder
.AddConsole()
.AddDebug());
}
Scale-Out: For applications needing to support thousands of concurrent connections, consider using Azure SignalR Service or similar scale-out mechanisms.
To use Azure SignalR Service, add the service connection string to your appsettings.json
and modify the Startup.cs
to include:
services.AddSignalR().AddAzureSignalR(connectionString);
By adhering to these best practices, developers can build SignalR applications that are not only functional but also secure, scalable, and performant, ensuring a smooth and responsive user experience.
If you want to learn how to use JSON in T-SQL with SQL Server, refer to our detailed guide: Working with JSON in SQL Server.
Wrapping Up
In wrapping up our exploration of SignalR within the ASP.NET Core framework it is clear that SignalR is an effective tool for producing modern, real time web apps. Its ability to offer real-time, two way communication between server and client revolutionizes development of interactive elements like live chat, real time alerts, dynamic dashboards and collaborative editing. Understanding SignalR’s capabilities, applying best practices for scalability, security, and performance optimization, and taking advantage of its communication protocols flexibility can help developers enhance the user experience and performance of their applications.
For those looking to transform your digital presence, consider WireFuture. It is a leading asp.net development company specializing in bespoke .NET development services. From dynamic web applications to scalable enterprise systems, our expertise drives innovation and growth. Partner with WireFuture for unparalleled .NET excellence.
At WireFuture, we believe every idea has the potential to disrupt markets. Join us, and let's create software that speaks volumes, engages users, and drives growth.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.