Complete Guide to Regex in C#: Patterns, Groups & More
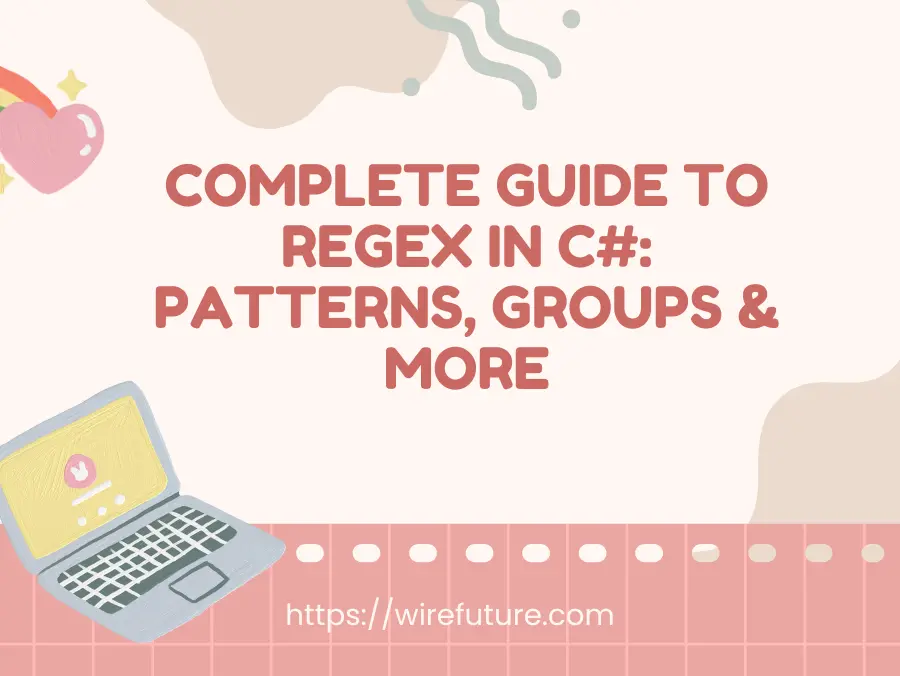
Regular expressions (Regex) are crucial for effective string manipulation and validation in software development. They allow developers to match, search, and manipulate strings with precision and efficiency.
Overview of Regex and Its Importance in Pattern Matching
Regex provides a method to define search patterns for text, enabling functionalities like email verification, data parsing, or password validation. This makes Regex a fundamental tool in any developer’s toolkit.
Brief Introduction to the System.Text.RegularExpressions Namespace in C#
In C#, Regex capabilities are housed within the System.Text.RegularExpressions
namespace, offering essential classes like Regex
for operations such as matching and replacing, and Match
and Group
for capturing specific segments of text. These tools simplify complex text-processing tasks, allowing for cleaner, more efficient code.
Table of Contents
- Basic Concepts of Regex
- Using Regex Class in C#
- Patterns and Metacharacters
- Quantifiers in Regex
- Advanced Matching Using Lookahead and Lookbehind
- Captured Groups and Backreferences
- Practical Examples of Regex in C#
- Performance Considerations
- Tools and Resources
- Conclusion
Basic Concepts of Regex
A regular expression (Regex) is a string of letters defining a search pattern. This pattern returns a way to find, match, and manipulate text in a string. Regex patterns are not only about finding text that matches the pattern exactly but also text that fits some format (specific numbers, letters, or a mixture of both). This makes Regex useful for everything from basic string searches to more complex text manipulations.
Given the complexity and potential of Regex in applications, particularly those developed with C#, it can be highly beneficial to hire C# developers who are proficient in these techniques.
Common Characters Used in Regex
Regex uses a variety of characters to define patterns. Here are some of the most commonly used characters in Regex expressions:
- \d: Matches any digit. Equivalent to
[0-9]
. - \w: Matches any word character (alphanumeric plus underscore). Equivalent to
[A-Za-z0-9_]
. - \s: Matches any whitespace character (spaces, tabs, line breaks).
- ^: Asserts the position at the start of a line.
- $: Asserts the position at the end of a line.
- .: Matches any character except newline, unless specified otherwise.
Each of these characters serves a unique purpose, enabling the creation of versatile and powerful search patterns.
The Concept of Literals and Special Characters
In Regex, literals are characters that represent themselves in a search and do not hold any special meaning. For example, the letter a
is a literal and will match the character ‘a’ in a string. On the other hand, special characters, like the ones listed above (\d
, \w
, \s
, etc.), perform specific functions and are part of the Regex syntax that helps define complex patterns.
- Literals: Characters like
a
,b
,1
, or@
that match directly. - Special Characters: Characters that, when combined, form the powerful syntax of Regex. To use a special character as a literal, it must be escaped with a backslash (
\
). For example,\.
matches a literal period.
Using Regex Class in C#
The Regex
class in C# provides a suite of methods to apply regular expressions to strings. Understanding how to utilize this class effectively is crucial for performing complex text manipulations and validations.
How to Create a Regex Object
To use the Regex capabilities, you first need to create an instance of the Regex
class. This is typically done by passing a regex pattern as a string to the constructor of the class. For example:
Regex myRegex = new Regex(@"\d+");
This example creates a Regex object that matches one or more digits in a string. You can also pass RegexOptions as a second parameter to modify the behavior of the pattern matching.
Overview of Key Methods in the Regex Class
The Regex
class includes several methods that allow you to perform different operations related to regular expressions:
- Match(): This method searches the specified input string for the first occurrence of the regular expression specified in the Regex constructor. It returns a
Match
object containing details about the match found, or it returnsMatch.None
if no match was found. - Matches(): Unlike
Match()
, which only finds the first match,Matches()
returns aMatchCollection
containing all the matches of the regular expression found in the input string. - Replace(): This method replaces the text in the input string that matches the regex pattern with a specified replacement string. It is highly useful for tasks such as formatting strings.
- Split(): This method splits an input string into an array of substrings at the positions defined by a regular expression pattern. It is often used to parse text.
Here’s a brief code example illustrating these methods:
string input = "123 to 456";
Regex regex = new Regex(@"\d+");
// Match
Match match = regex.Match(input);
Console.WriteLine("First Match: " + match.Value);
// Matches
MatchCollection matches = regex.Matches(input);
foreach(Match m in matches) {
Console.WriteLine("Found Match: " + m.Value);
}
// Replace
string replaced = regex.Replace(input, "number");
Console.WriteLine("Replaced String: " + replaced);
// Split
string[] split = regex.Split(input);
Console.WriteLine("Split Array: " + string.Join(", ", split));
Discussing the RegexOptions Enum for Controlling Regex Behavior
RegexOptions
is an enumeration that provides options to modify the behavior of the pattern matching. Some of the most commonly used options include:
- RegexOptions.IgnoreCase: Makes the pattern matching case-insensitive.
- RegexOptions.Multiline: Changes the meaning of
^
and$
so they match at the beginning and end of any line, not just the beginning and end of the string. - RegexOptions.Compiled: Compiles the regular expression to an assembly, which speeds up its execution.
Using RegexOptions
can significantly alter how patterns are matched and should be chosen based on the specific requirements of the application.
Patterns and Metacharacters
In regular expressions, patterns and metacharacters are the building blocks that allow developers to craft complex search criteria for string manipulation and analysis. Understanding these elements is critical for effectively utilizing Regex in any programming environment, including C#.
Detailed Explanation of Character Classes
Character classes are fundamental components of regular expressions that define sets of characters to match within a string. Here are some of the most commonly used character classes in Regex:
- [abc]: Matches any single character listed within the brackets. In this example, it matches ‘a’, ‘b’, or ‘c’.
- [^abc]: Matches any single character not listed within the brackets. This example matches any character except ‘a’, ‘b’, or ‘c’.
- [a-z]: Matches any single character in the range from ‘a’ to ‘z’.
- \d: A shorthand character class that matches any digit, equivalent to [0-9].
- \w: Matches any word character (alphanumeric or underscore), equivalent to [A-Za-z0-9_].
- \s: Matches any whitespace character (spaces, tabs, newlines).
Using character classes allows for flexible and powerful string matching capabilities, making them indispensable in data validation, extraction, and transformation tasks.
Anchors and Position Metacharacters
Anchors and position metacharacters do not match characters themselves but instead match positions within the string. They are crucial for asserting whether a certain pattern occurs at the beginning, middle, or end of a string. Common anchors include:
- ^: Matches the start of a string or line, depending on the mode (single-line or multi-line) the regex is operating in.
- $: Matches the end of a string or line.
- \b: Matches a word boundary, which is the position between a word character and a non-word character.
These anchors are used to ensure that a pattern appears in a specific context within the text, which is particularly useful in tasks like validating the structure of strings.
Grouping Constructs and How They Alter Processing
Grouping constructs in Regex allow you to combine a sequence of pattern elements into a single unit, which can be captured, quantified, or referenced later in the pattern. The use of parentheses ()
is the most common way to create groups. Here’s how they can alter the processing of regex patterns:
- Capturing Groups: Parentheses capture the text matched by the pattern inside them. This allows the programmer to extract or manipulate specific parts of the string. For example,
(\d+)-(\d+)
could be used to match and capture two sets of digits separated by a hyphen. - Non-Capturing Groups: Sometimes, you might want to use groups for applying quantifiers or logical conditions without capturing the matched text. You can create a non-capturing group by starting it with
?:
, like so:(?:\d+)
. - Named Groups: You can also give names to the groups, which makes the regex more readable and the code easier to maintain. Named groups are defined by
(?<name>pattern)
, wherename
is the label for the group.
Grouping constructs are incredibly powerful for managing complex matching logic, enabling developers to build sophisticated pattern-matching functionality that can capture, reuse, and manipulate subpatterns within a string.
Quantifiers in Regex
Quantifiers in regular expressions are powerful tools that specify how many instances of a character, group, or character class must be present in the input for a match to occur. They are essential for creating flexible patterns that can match varying amounts of text. Understanding and implementing these quantifiers effectively can greatly enhance the functionality of applications, particularly in environments that utilize .NET development services
Describing Different Quantifiers
Quantifiers can broadly adjust how a pattern matches text. Here’s a breakdown of the most commonly used quantifiers in Regex:
- *: The asterisk indicates that the preceding element should be matched zero or more times. For example,
ab*
would match ‘a’, ‘ab’, ‘abb’, ‘abbb’, etc. - +: The plus sign dictates that the preceding element must appear one or more times. For instance,
ab+
would match ‘ab’, ‘abb’, ‘abbb’, etc., but not ‘a’. - ?: The question mark means the preceding element may appear zero or one time. Therefore,
ab?
matches either ‘a’ or ‘ab’. - {n}: This quantifier matches exactly n occurrences of the preceding element. For example,
a{3}
matches exactly ‘aaa’. - {n,}: Matches n or more occurrences of the preceding element.
a{2,}
would match ‘aa’, ‘aaa’, ‘aaaa’, and so on. - {n,m}: Matches between n and m occurrences of the preceding element, inclusive.
a{2,4}
matches ‘aa’, ‘aaa’, or ‘aaaa’.
Greedy vs. Lazy vs. Possessive Quantifiers
Quantifiers in Regex can be classified into three types based on their matching strategy: greedy, lazy, and possessive. Understanding the difference between these is crucial for writing efficient Regex patterns.
- Greedy Quantifiers: These quantifiers try to match as much text as possible. They are the default behavior for quantifiers like
*
,+
,{n,}
and{n,m}
. For example, in the string “aaaaaa”,a+
will match all ‘a’s. - Lazy (or Reluctant) Quantifiers: Lazy quantifiers, on the other hand, match as little text as possible. They are denoted by appending a
?
to a greedy quantifier. For instance, in the string “aaaaaa”,a+?
will match only the first ‘a’. - Possessive Quantifiers: These are like greedy quantifiers but without the backtracking. They are denoted by appending a
+
to a greedy quantifier. If a match fails after a possessive quantifier consumes characters, the regex engine does not backtrack to attempt other potential matches. For example, in the string “aaaaaab”,a++b
will not match because the possessivea++
consumes all ‘a’s and leaves nothing for matching ‘b’.
Here’s an example illustrating the differences:
string text = "aaab";
Regex greedyRegex = new Regex("a+"); // Matches 'aaa'
Regex lazyRegex = new Regex("a+?"); // Matches 'a'
Regex possessiveRegex = new Regex("a++"); // Matches 'aaa', but would fail if followed by 'b' as it leaves no 'a' for 'b'
Match greedyMatch = greedyRegex.Match(text);
Match lazyMatch = lazyRegex.Match(text);
// Note: .NET does not directly support possessive quantifiers, and the above is an illustration.
Console.WriteLine("Greedy Match: " + greedyMatch.Value); // Outputs: aaa
Console.WriteLine("Lazy Match: " + lazyMatch.Value); // Outputs: a
Advanced Matching Using Lookahead and Lookbehind
- Lookahead Assertions: These allow you to check for the presence or absence of a pattern following your main pattern without including it in the match. There are two types:
- Positive Lookahead (
(?=...)
): Asserts that what immediately follows the current position in the string must match the pattern inside the lookahead. - Negative Lookahead (
(?!...)
): Asserts that what immediately follows the current position in the string must not match the pattern inside the lookahead.
- Positive Lookahead (
- Lookbehind Assertions: Similar to lookahead assertions, but they check the text preceding the current position:
- Positive Lookbehind (
(?<=...)
): Asserts that the text preceding the current position must match the pattern inside the lookbehind. - Negative Lookbehind (
(?<!...)
): Asserts that the text preceding the current position must not match the pattern inside the lookbehind.
- Positive Lookbehind (
Practical Examples Where These Can Be Particularly Useful
Lookahead and lookbehind are extremely useful for extracting or validating strings where you need to consider context without capturing it. Here are some practical examples:
Validating Passwords: Suppose you need to validate that a password contains at least one digit, one uppercase letter, and is between 8 and 15 characters long.
Regex regex = new Regex(@"^(?=.*\d)(?=.*[A-Z]).{8,15}$");
Here, (?=.*\d)
is a positive lookahead to ensure there is at least one digit, and (?=.*[A-Z])
ensures there is at least one uppercase letter anywhere in the string.
Excluding Specific Terms: If you want to find instances of “file” that are not immediately followed by “.txt”:
Regex regex = new Regex(@"file(?!\.txt)");
The negative lookahead (?!\.txt)
asserts that “file” is not followed by “.txt”.
Matching Lines Not Starting with Specific Words: For instance, match lines that do not start with “Error” or “Warning”:
Regex regex = new Regex(@"^(?!Error|Warning).*$", RegexOptions.Multiline);
This uses a negative lookahead combined with the multiline mode to apply the pattern at the start of each line.
Extracting Text Within Brackets Without Including Brackets: If you need to extract text inside brackets:
Regex regex = new Regex(@"(?<=\[).+?(?=\])");
Here, (?<=\[)
is a positive lookbehind ensuring the text is preceded by [
, and (?=\])
is a positive lookahead ensuring the text is followed by ]
.
Conditionally Adding or Replacing Based on Context: For instance, adding a unit to numbers only if they are not already followed by a unit like “cm”:
Regex regex = new Regex(@"\d+(?!cm)");
This pattern matches digits (\d+
) only if they are not followed by “cm”, allowing for context-sensitive manipulation.
Captured Groups and Backreferences
Captured groups and backreferences are powerful features in regular expressions that enhance the flexibility and functionality of pattern matching. They allow you to capture parts of the matched text and reuse them within the regex or in subsequent replacements and operations.
What are Captured Groups and How to Use Them in C#
Captured groups are parts of the regex enclosed in parentheses, which not only apply the regex logic to control the pattern matching but also save the matched text for later use. These groups can be referred to by their index number, starting at 1.
Example in C#:
string text = "John Smith 1990";
Regex regex = new Regex(@"(\w+) (\w+) (\d+)");
Match match = regex.Match(text);
if (match.Success) {
Console.WriteLine("First Name: " + match.Groups[1].Value); // John
Console.WriteLine("Last Name: " + match.Groups[2].Value); // Smith
Console.WriteLine("Year: " + match.Groups[3].Value); // 1990
}
This example captures three groups: first name, last name, and year, each accessible through the Groups
property of the Match
object.
Named Captured Groups Using (?<name>...)
Named captured groups enhance the readability and manageability of regex patterns by allowing you to assign names to each group instead of using numeric indexes.
Example in C#:
string text = "John Smith 1990";
Regex regex = new Regex(@"(?<firstName>\w+) (?<lastName>\w+) (?<year>\d+)");
Match match = regex.Match(text);
if (match.Success) {
Console.WriteLine("First Name: " + match.Groups["firstName"].Value); // John
Console.WriteLine("Last Name: " + match.Groups["lastName"].Value); // Smith
Console.WriteLine("Year: " + match.Groups["year"].Value); // 1990
}
This approach is particularly useful in complex expressions where tracking group numbers can be confusing.
Utilizing Backreferences to Refer to Previous Groups Within a Pattern
Backreferences in a regex allow you to refer back to previously captured groups and match the same text again later in the same pattern. This is useful for patterns where the input should repeat in a specific way.
Example in C#:
string text = "go go";
Regex regex = new Regex(@"(\w+) \1");
Match match = regex.Match(text);
if (match.Success) {
Console.WriteLine("Repeated Word: " + match.Groups[1].Value); // go
}
Here, \1
refers to the first captured group, ensuring that the second word matches exactly the first word captured.
Backreferences can also be used in replacements:
string text = "Hello 123";
string result = Regex.Replace(text, @"(\d+)", "#$1#");
Console.WriteLine(result); // Hello #123#
This example inserts #
characters before and after the sequence of digits captured by the group.
Practical Examples of Regex in C#
Regular expressions in C# are indispensable for various tasks that involve pattern matching and string manipulation. Here, we’ll explore some practical applications of Regex, specifically for validating user input, searching within strings and extracting data, and handling complex string manipulation scenarios. This expertise is crucial in custom software development, where tailored solutions often require precise and efficient data handling capabilities.
Validating User Input
Regex is extremely useful for ensuring that user input conforms to expected formats, such as email addresses, phone numbers, and other structured data.
Email Validation:
string email = "example@domain.com";
Regex regex = new Regex(@"^[^@\s]+@[^@\s]+\.[^@\s]+$");
bool isValidEmail = regex.IsMatch(email);
Console.WriteLine("Is the email valid? " + isValidEmail); // True
This pattern checks for a typical structure of an email address: characters followed by an @
symbol, more characters, a period, and then more characters.
Phone Number Validation:
string phone = "+1-800-555-0199";
Regex regex = new Regex(@"^\+\d{1,3}-\d{3}-\d{3}-\d{4}$");
bool isValidPhone = regex.IsMatch(phone);
Console.WriteLine("Is the phone number valid? " + isValidPhone); // True
This regex validates international phone numbers with a country code prefix, ensuring the number is properly formatted.
Searching Within Strings and Extracting Data
Regex can be used to locate specific data within a string or extract various parts of the string based on a pattern.
Extract Dates:
string text = "The event will happen on 12/25/2022.";
Regex regex = new Regex(@"\b\d{1,2}/\d{1,2}/\d{4}\b");
Match match = regex.Match(text);
if (match.Success) {
Console.WriteLine("Found date: " + match.Value); // 12/25/2022
}
This example finds dates formatted as MM/DD/YYYY in a text.
Search for Keywords
string content = "Learn more about C#, Java, and Python.";
Regex regex = new Regex(@"\b(C#|Java|Python)\b", RegexOptions.IgnoreCase);
MatchCollection matches = regex.Matches(content);
foreach (Match m in matches) {
Console.WriteLine("Found language: " + m.Value);
}
This pattern matches specific programming language names, useful for syntax highlighting or feature tagging in texts.
Complex String Manipulation Scenarios
Regex also excels in complex string manipulations that involve conditional replacements or formatting.
Conditional Text Replacement:
string text = "Replace the word 'cat' with 'dog' but not 'catalog' or 'caterpillar'.";
Regex regex = new Regex(@"\bcat\b");
string modifiedText = regex.Replace(text, "dog");
Console.WriteLine(modifiedText);
This replaces “cat” only when it appears as a whole word, not as part of another word like “catalog”.
Reformatting Data:
string data = "Name: John, Age: 30; Name: Jane, Age: 25;";
Regex regex = new Regex(@"Name: (?<name>\w+), Age: (?<age>\d+);");
string reformatted = regex.Replace(data, "User: ${name}, Years: ${age}; ");
Console.WriteLine(reformatted);
This example uses named groups to extract and reformat parts of the string into a new structure, which is useful for data transformation tasks such as preparing data for reports.
Performance Considerations
Best Practices for Optimizing Regex Performance in C#
Use Compiled Regex:
- The
RegexOptions.Compiled
option in C# compiles the regular expression to MSIL (Microsoft Intermediate Language), which can significantly improve performance for regular expressions that are used frequently. - This practice is particularly effective in long-running applications but involves a higher initialization cost.
Regex regex = new Regex(@"\b\w+\b", RegexOptions.Compiled);
Be Specific with Your Patterns:
- The more specific the pattern, the fewer paths the engine has to explore. Avoid using overly broad patterns such as
.*
which can lead to excessive backtracking. - Use character classes and quantifiers judiciously to tighten the scope of the match.
Avoid Unnecessary Capturing:
- If you do not need to capture groups for later reference, use non-capturing groups
(?:...)
instead of capturing groups. This reduces the overhead associated with storing captured substrings.
Opt for Non-Greedy Quantifiers:
Non-greedy quantifiers (*?
, +?
, ??
, {n,m}?
) match the minimum number of characters necessary and can often be more efficient than their greedy counterparts.
Regex regex = new Regex(@"<title>.*?</title>");
Use Anchors:
Anchors like ^
(start of string) and $
(end of string) can help reduce the workload by anchoring the regex engine to specific positions in the input string.
Regex regex = new Regex(@"^\d+");
Common Pitfalls and How to Avoid Them
- Catastrophic Backtracking:
- This occurs when the regex engine spends an excessive amount of time backtracking through various permutations of the input, especially with nested quantifiers.
- To avoid this, simplify the regex pattern, limit the use of nested quantifiers, and be more specific with your character classes and quantifiers.
- Overusing the Dot (
.
) Character:- The dot is often overused in patterns where more specific character classes would be more appropriate. It matches almost any character, which can lead to performance issues and unintended matches.
- Instead of using the dot, define a more specific set of characters that you expect in that position.
- Misusing Lookaround Assertions:
- While powerful, lookarounds can be costly in terms of performance. Use them sparingly and only when necessary.
- Optimize the inside of the lookaround to be as specific and restrictive as possible to minimize performance hits.
- Frequent Recompilation of Regex Objects:
- Recompiling the same regex pattern multiple times can lead to unnecessary overhead. To avoid this, store and reuse
Regex
objects when the same pattern is applied multiple times.
- Recompiling the same regex pattern multiple times can lead to unnecessary overhead. To avoid this, store and reuse
static readonly Regex emailRegex = new Regex(@"^[^@\s]+@[^@\s]+\.[^@\s]+$", RegexOptions.Compiled);
Tools and Resources
Recommended Tools for Testing and Debugging Regex Patterns
- RegexBuddy:
- RegexBuddy is a popular tool that allows you to create, test, and debug regular expressions in a user-friendly environment. It supports many programming languages, including C#. While it is a paid tool, its comprehensive features make it a worthwhile investment for those who work with regex extensively.
- Link: RegexBuddy
- Regex101:
- Regex101 is a powerful online regex testing tool that supports multiple languages, including PCRE (Perl Compatible Regular Expressions), which closely resemble C# syntax. It provides a detailed explanation of your regex pattern, making it easier to understand how it works. This tool is particularly useful for learning and debugging complex regex expressions.
- Link: Regex101
- Microsoft’s Official Documentation:
- Microsoft provides comprehensive documentation on the
System.Text.RegularExpressions
namespace, offering insights into all the classes, methods, and properties available for Regex in C#. This is a great resource for understanding how regex is implemented specifically in the .NET framework. - Link: Microsoft Docs on Regular Expressions
- Microsoft provides comprehensive documentation on the
Conclusion
Regular expressions are a powerful tool in any programmer’s toolkit, especially for those working with C#. The versatility and efficiency of Regex make it an indispensable resource for parsing, validating, and manipulating text data. While the concepts and syntax of Regex can initially seem daunting, mastery of this tool can significantly enhance your capabilities in handling complex string operations.
At WireFuture, a .NET Development Company, we pride ourselves on leveraging cutting-edge technologies and methodologies to deliver exceptional software solutions. Regular expressions are just one of the many tools we employ to ensure our applications are robust, secure, and performant. If you’re looking to boost your .NET applications or need expert guidance and services in software development, consider reaching out to WireFuture. Let’s build powerful solutions together.
WireFuture stands for precision in every line of code. Whether you're a startup or an established enterprise, our bespoke software solutions are tailored to fit your exact requirements.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.