Mastering .NET: A Deep Dive into Task Parallel Library
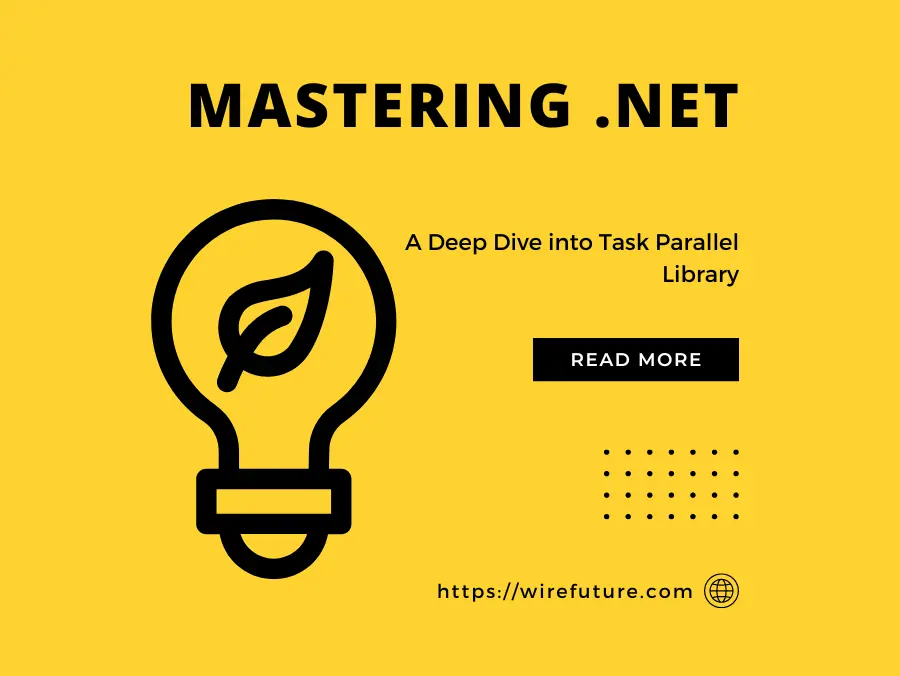
Task parallel Library (TPL) is an important component of the .NET framework for Parallel programming, allowing programmers to write fast, scalable and powerful multithreaded programs. As part of .NET’s parallel programming landscape, TPL makes it easy to add parallelism and concurrency to applications.
What is the Use of TPL in .NET for Parallel Programming?
TPL offers a higher-level abstraction for parallel execution; thus you don’t need to worry about the thread creation and synchronization issues directly. It automatically handles the work partitioning, task scheduling (on multiple threads) and thread management (across processors). This abstraction lets developers concentrate on the business logic rather than on thread management details.
Comparison with Other Parallel Programming Models in .NET
Although .NET provides various models for parallel execution including raw threads and thread pools, TPL is notable for its powerful and flexible API. Traditional threading involves tedious thread management and control of threads and race conditions, and can be tedious and inaccurate. Thread pools manage a collection of threads for repeated task execution, but lack fine-grained control over individual tasks. TPL extends these ideas with a task-based model that encapsulates data and code for more control and scalability. This makes TPL an essential tool in the arsenal of .NET development services, enabling more efficient and effective management of parallel tasks.
Pros of TPL
Simplicity: TPL makes parallel code simpler by letting you concentrate on what should be parallelized instead of the way it should be parallelized. The framework takes care of the ‘ how’, managing all aspects of task execution.
Scalability: With TPL, your applications can grow on multiple processors. The library partitions tasks among available computing resources and adjusts utilization based on workload and system capability.
Performance Enhancements: TPL applications can realize dramatic performance gains, especially for data and task parallelism. By reducing the thread overhead and maximizing parallel execution TPL can reduce execution time and improve responsiveness.
Table of Contents
- Core Concepts of Task Parallel Library (TPL)
- Using Task Factory in the Task Parallel Library (TPL)
- Task Schedulers in the Task Parallel Library (TPL)
- Parallel Loops and Aggregation in the Task Parallel Library (TPL)
- Exception Handling in TPL
- Cancellation Framework in TPL
- Advanced Topics in Task Parallel Library (TPL)
- Conclusion
Core Concepts of Task Parallel Library (TPL)
Knowledge of the fundamentals of TPL is essential to utilize its features in .NET applications. The library is organized around tasks that are the foundation of parallel execution, providing an extensible yet robust approach to asynchronous programming.
Tasks Explained in TPL
In TPL, a task is an asynchronous operation. In .NET’s TPL, a task is an object encapsulating the details of a work (a method that needs to be executed) and controlling its execution in a different thread pooled from the system. This abstraction enables developers to think about high level operations instead of low-level threading and synchronization details. Tasks may return results, and exceptions raised during task execution are handled in asynchronous code by propagating to the code that waits for the task results.
Task Class: Methods and Properties
The Task
class is central in TPL and provides several methods and properties that help in the creation, management, and synchronization of tasks. Key methods include:
Start()
: Begins task execution asynchronously.Wait()
: Blocks the calling thread until the task completes, which is useful for handling dependencies between tasks.ContinueWith()
: Allows for execution of a continuation task when the preceding task completes, which can be used to chain tasks together or handle tasks’ results or exceptions.
Properties such as Status
, IsCompleted
, and Result
offer insights into the task’s current state, completion status, and result value, respectively. These properties are crucial for monitoring and controlling task execution and integrating tasks with other application components.
Concept of Task Continuations
Task continuations are a powerful aspect of TPL that enables building complex workflows where tasks are connected to create a chain of operations. A continuation task can then start automatically when a task finishes, allowing sequential execution patterns in an otherwise parallel or asynchronous program structure. This is especially useful when the next task must process the results of the last task or deal with its exceptions. By calling ContinueWith(), developers can specify when the continuation task should start, for example, after the original task is finished successfully, failed, or canceled.
Continuations can also be nested to create more complex task dependencies and execution trees. This makes TPL extremely flexible for handling large asynchronous operations involving coordination of many steps or stages.
Using Task Factory in the Task Parallel Library (TPL)
Task Factory is a basic TPL component serving as a top-level task creator, which makes task instantiation and configuration a breeze. It proposes ways to create and control tasks more effectively, particularly for the more complex or repetitive task creation settings.
TaskFactory: An introduction to TaskFactory
The TaskFactory class is designed to produce various types of tasks, ranging from simple to complex, using customizable configurations. It stores default settings for the tasks it generates, allowing developers to map task creation parameters across an application. This is especially useful for applications where multiple tasks with similar configurations are required, minimizing recurrence of code and minimizing error potential.
Examples of Using TaskFactory
Here are a couple of examples to illustrate how TaskFactory can be used in real-world applications:
Creating a Simple Task: You can use TaskFactory for straightforward task creation without specifying many parameters. This is particularly useful for quick and easy task setup:
TaskFactory tf = new TaskFactory();
Task myTask = tf.StartNew(() => {
Console.WriteLine("Running a simple task");
});
Handling Task Continuations: TaskFactory can also simplify the process of setting up continuations, making it easier to manage complex workflows:
TaskFactory tf = new TaskFactory();
Task initial = tf.StartNew(() => PerformInitialTask());
Task continuation = initial.ContinueWith(prevTask => {
Console.WriteLine("Continuing after initial task");
}, TaskContinuationOptions.OnlyOnRanToCompletion);
Configuring Tasks with Common Settings: If you need multiple tasks with the same configuration, TaskFactory allows you to set these parameters once and use them repeatedly:
TaskFactory tf = new TaskFactory(CancellationToken.None, TaskCreationOptions.AttachedToParent,
TaskContinuationOptions.ExecuteSynchronously, TaskScheduler.Default);
Task task1 = tf.StartNew(() => DoWork(1));
Task task2 = tf.StartNew(() => DoWork(2));
Benefits of Using TaskFactory
- Simplified Task Configuration: TaskFactory allows for centralized task configuration, reducing redundancy and minimizing the chance of inconsistencies in task setup.
- Enhanced Code Readability and Maintenance: By abstracting the details of task creation, TaskFactory helps make the code more readable and easier to maintain. It’s especially beneficial in complex systems where tasks are frequently used and require consistent configurations.
- Efficient Task Management: With TaskFactory, managing dependencies between tasks through continuations becomes more streamlined. It provides built-in support for common patterns such as error handling and task chaining, which can significantly ease the complexity of managing task lifecycles.
Task Schedulers in the Task Parallel Library (TPL)
Task schedulers in TPL play a crucial role in how tasks are executed asynchronously. They are responsible for distributing tasks onto threads and managing how these tasks are prioritized and executed relative to one another.
Role of TaskScheduler in Controlling the Mapping of Tasks onto Threads
TaskScheduler is the component in TPL that determines which thread a task will run on, and when it will run. This allows for fine-tuned control over task execution, which can be crucial in applications where the timing and order of task execution are critical.
Code Example: Using the Default TaskScheduler
// Create a new task using the default scheduler
Task task1 = Task.Factory.StartNew(() => {
Console.WriteLine("Task 1 is running on a thread managed by the default scheduler.");
});
// Wait for the task to complete
task1.Wait();
Description of Default Task Scheduler and Scenarios for Custom Task Schedulers
The default Task Scheduler in .NET uses the ThreadPool to queue and execute tasks. This is generally sufficient for most scenarios where tasks are independent of each other and can be executed concurrently. However, there are scenarios where custom task schedulers might be necessary:
- Executing tasks on a single dedicated thread: Useful in scenarios where tasks need to be serialized or when tasks must not be run concurrently.
- Limiting concurrency to prevent resource saturation: When tasks consume intensive resources and running too many concurrently could lead to performance degradation.
Code Example: Implementing a Custom Task Scheduler
// Creating a custom task scheduler that schedules tasks on a single thread
public class SingleThreadTaskScheduler : TaskScheduler
{
private readonly BlockingCollection<Task> tasks = new BlockingCollection<Task>();
public SingleThreadTaskScheduler()
{
Thread dedicatedThread = new Thread(new ThreadStart(Execute));
dedicatedThread.Start();
}
private void Execute()
{
foreach (var task in tasks.GetConsumingEnumerable())
{
TryExecuteTask(task);
}
}
protected override IEnumerable<Task> GetScheduledTasks()
{
return tasks;
}
protected override void QueueTask(Task task)
{
tasks.Add(task);
}
protected override bool TryExecuteTaskInline(Task task, bool taskWasPreviouslyQueued)
{
return false; // Force all tasks to be processed by the dedicated thread
}
}
// Usage of the custom scheduler
TaskScheduler scheduler = new SingleThreadTaskScheduler();
Task task2 = new Task(() => Console.WriteLine("Task 2 executed with custom scheduler."), TaskCreationOptions.LongRunning);
task2.Start(scheduler);
Impact of Task Schedulers on Performance and Task Execution Order
Task schedulers have a significant impact on the performance and order of task execution. The choice of a task scheduler can affect how quickly tasks begin execution, their completion time, and how they are prioritized.
- Performance: The efficiency of task execution can be greatly influenced by how tasks are scheduled. A well-tuned task scheduler can reduce waiting times and make full use of the processor’s capabilities.
- Execution Order: Some applications require tasks to be executed in a specific order. Custom task schedulers can be created to enforce such order, ensuring that tasks are executed in the sequence required by the application logic.
Code Example: Task Scheduler Impact on Execution Order
// Demonstrating the effect of a custom scheduler on task execution order
Task.Factory.StartNew(() => Console.WriteLine("First Task"), CancellationToken.None, TaskCreationOptions.None, scheduler);
Task.Factory.StartNew(() => Console.WriteLine("Second Task"), CancellationToken.None, TaskCreationOptions.None, scheduler);
Parallel Loops and Aggregation in the Task Parallel Library (TPL)
Parallel loops are a core feature of the Task Parallel Library (TPL), which simplify the process of executing iterations in parallel by automatically distributing these iterations across multiple threads and processors. As a .NET development company, leveraging this feature is highly advantageous for tasks where operations can be performed independently on elements of a collection or range of numbers. This capability allows for significant improvements in performance and efficiency, making parallel loops an essential tool for optimizing various computing tasks within .NET applications.
Overview of Parallel Loops (Parallel.For and Parallel.ForEach)
- Parallel.For: Used to parallelize operations over a range of integer values. This is ideal for scenarios where you have a clear numeric range of operations.
- Parallel.ForEach: Used for iterating over any IEnumerable<T> collection in parallel, making it versatile for a variety of data structures.
Code Example: Using Parallel.For
// Using Parallel.For to perform a parallel operation on a range of integers
Parallel.For(0, 10, i =>
{
Console.WriteLine($"Processing {i} on thread {Thread.CurrentThread.ManagedThreadId}");
});
Code Example: Using Parallel.ForEach
// Using Parallel.ForEach to process elements of a list in parallel
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
Parallel.ForEach(numbers, number =>
{
Console.WriteLine($"Processing number {number} on thread {Thread.CurrentThread.ManagedThreadId}");
});
Techniques for Handling Loop Data Aggregation Safely
When performing data aggregation in parallel loops, it’s crucial to manage concurrent updates to shared data structures safely. TPL provides several options to handle aggregation without running into race conditions:
- Thread-local variables: Using local variables that are specific to each thread to store intermediate results.
- Concurrent collections: Utilizing thread-safe collections provided by .NET such as
ConcurrentBag<T>
orConcurrentDictionary<TKey, TValue>
. - Locks and synchronization: Applying locking mechanisms to protect shared data during updates.
Code Example: Thread-Local Aggregation with Parallel.For
// Using thread-local variables to safely aggregate data
int totalSum = 0;
Parallel.For(0, 1000,
() => 0, // Initialize the local state
(i, loopState, localSum) => localSum + i, // Accumulate in local state
localSum => Interlocked.Add(ref totalSum, localSum) // Aggregate local states safely
);
Console.WriteLine($"Total sum is {totalSum}");
Code Example: Using Concurrent Collections with Parallel.ForEach
// Using a ConcurrentBag to aggregate results safely
ConcurrentBag<int> sums = new ConcurrentBag<int>();
Parallel.ForEach(numbers, number =>
{
sums.Add(number * number); // Square each number and add to the concurrent bag
});
int total = sums.Sum();
Console.WriteLine($"Total of squares is {total}");
Examples to Demonstrate the Reduction of Execution Time with Parallel Loops
Using parallel loops can significantly reduce execution time compared to sequential execution, especially for CPU-intensive tasks.
Code Example: Comparing Parallel and Sequential Execution Times
// Measure execution time for sequential vs parallel processing
Stopwatch stopwatch = new Stopwatch();
// Sequential execution
stopwatch.Start();
List<int> data = Enumerable.Range(0, 100000).ToList();
int sum = 0;
foreach (int x in data) {
sum += x;
}
stopwatch.Stop();
Console.WriteLine($"Sequential sum: {sum} took {stopwatch.ElapsedMilliseconds} ms");
// Parallel execution
stopwatch.Restart();
int parallelSum = 0;
Parallel.ForEach(data, x => {
Interlocked.Add(ref parallelSum, x);
});
stopwatch.Stop();
Console.WriteLine($"Parallel sum: {parallelSum} took {stopwatch.ElapsedMilliseconds} ms");
Exception Handling in TPL
Exception handling in TPL is a critical aspect, as it ensures that your application can gracefully handle errors that occur in parallel tasks. This is especially challenging due to the nature of parallel execution where multiple tasks may throw exceptions simultaneously.
Challenges of Exception Handling in Asynchronous and Parallel Code
- Multiple Exceptions: Multiple tasks running in parallel can fail simultaneously, each throwing its own exception.
- Exception Propagation: In a sequential program, exceptions are thrown directly to the caller. In parallel programs, handling becomes complex as the context in which the error occurred may not be directly accessible.
- Synchronization Context: Exceptions need to be marshaled back to the calling thread, especially in UI applications, which adds another layer of complexity.
Code Example: Handling Multiple Exceptions
try
{
Parallel.Invoke(
() => throw new InvalidOperationException("Invalid operation!"),
() => throw new AccessViolationException("Access violation!")
);
}
catch (AggregateException ae)
{
ae.Handle(x =>
{
if (x is InvalidOperationException)
{
Console.WriteLine("Handled InvalidOperationException");
return true;
}
return false; // Unhandled, will re-throw
});
}
Strategies for Effective Exception Management in TPL
Effective exception management is essential to maintain the robustness and reliability of applications utilizing TPL.
- AggregateException: TPL wraps exceptions thrown by tasks in an
AggregateException
. This exception type can contain multiple inner exceptions that represent all the errors that occurred during the execution of parallel tasks.
Code Example: Handling AggregateException
Task<int> task = Task.Run(() => { throw new Exception("Error in task."); return 1; });
try
{
task.Wait();
}
catch (AggregateException ae)
{
foreach (var e in ae.InnerExceptions)
{
Console.WriteLine($"Handled exception: {e.Message}");
}
}
- Task Continuations for Error Handling: Use task continuations to handle exceptions in a clean, non-blocking way.
Code Example: Using Continuations for Exceptions
Task<int> task = Task.Run(() => { throw new Exception("Error!"); return 42; });
task.ContinueWith(t =>
{
if (t.IsFaulted)
{
Console.WriteLine($"Error: {t.Exception.InnerExceptions[0].Message}");
}
}, TaskContinuationOptions.OnlyOnFaulted);
Best Practices for Debugging and Diagnosing Task-Based Applications
- Using Debuggers: Modern IDEs like Visual Studio provide tools that can attach to multiple threads, helping to trace through parallel executions.
- Logging: Implement logging within tasks to record execution flow and capture exceptions as they occur.
- Task Status Checks: Regularly check task status (
Task.IsFaulted
,Task.IsCompleted
,Task.IsCanceled
) to monitor task health.
Code Example: Task Status Checks
Task task = Task.Run(() => throw new NullReferenceException());
task.ContinueWith(t =>
{
if (t.IsFaulted)
{
Console.WriteLine("Task failed with exception!");
}
}, TaskContinuationOptions.OnlyOnFaulted);
task.Wait();
Cancellation Framework in TPL
Cancellation in TPL is an essential mechanism that allows for responsive applications by giving tasks the ability to be cancelled gracefully. This is especially important in UI applications, long-running processes, or any scenario where conditions change dynamically requiring a halt in ongoing tasks.
Importance of Task Cancellation in Responsive Applications
- User Control: Users can cancel operations they initiate, which enhances the application’s usability and responsiveness.
- Resource Management: Cancellation helps free up resources that would otherwise be wasted on non-essential tasks.
- System Stability: By allowing tasks to be cancelled, systems can prevent unnecessary load, potentially improving stability and performance.
Usage of CancellationTokenSource and CancellationToken
The CancellationTokenSource
and CancellationToken
classes are the primary tools for managing task cancellations in .NET. CancellationTokenSource
generates a CancellationToken
that can be passed to tasks and used to handle cancellation requests.
Code Example: Basic CancellationToken Usage
var cts = new CancellationTokenSource();
var token = cts.Token;
Task task = Task.Run(() => {
while (!token.IsCancellationRequested)
{
Console.WriteLine("Task is running");
Thread.Sleep(1000); // Simulate work
}
token.ThrowIfCancellationRequested();
}, token);
try
{
Thread.Sleep(3000); // Let the task run for 3 seconds
cts.Cancel(); // Cancel the task
task.Wait(); // Optionally wait for the task to complete
}
catch (AggregateException ae)
{
if (ae.InnerExceptions[0] is OperationCanceledException)
{
Console.WriteLine("Task was cancelled.");
}
}
finally
{
cts.Dispose();
}
Examples Showing Graceful Task Cancellation
Implementing graceful task cancellation involves checking the cancellation token at appropriate points within the task’s execution and responding to cancellation requests by cleaning up resources and exiting cleanly. For a web development company, mastering this aspect of task management is crucial. It ensures that server resources are efficiently managed and that web applications remain responsive and stable, even when facing the need to halt operations. This capability is especially important in dynamic environments where user requests can be unpredictable and resource-intensive tasks may need to be stopped prematurely to preserve system performance.
Code Example: Graceful Cancellation in a Parallel.ForEach Loop
var numbers = Enumerable.Range(0, 100).ToList();
var cts = new CancellationTokenSource();
try
{
Parallel.ForEach(numbers, new ParallelOptions { CancellationToken = cts.Token }, number =>
{
if (number == 50) // Simulate condition to trigger cancellation
cts.Cancel();
cts.Token.ThrowIfCancellationRequested();
Console.WriteLine($"Processing number: {number}");
});
}
catch (OperationCanceledException)
{
Console.WriteLine("Operation was cancelled.");
}
finally
{
cts.Dispose();
}
Code Example: Integrating Cancellation with Async/Await
async Task ProcessDataAsync(CancellationToken token)
{
if (token.IsCancellationRequested)
token.ThrowIfCancellationRequested();
await Task.Run(() =>
{
for (int i = 0; i < 100; i++)
{
Thread.Sleep(100); // Simulate work
token.ThrowIfCancellationRequested();
}
}, token);
}
var cts = new CancellationTokenSource();
try
{
cts.CancelAfter(5000); // Automatically cancel after 5 seconds
await ProcessDataAsync(cts.Token);
}
catch (OperationCanceledException)
{
Console.WriteLine("Task cancelled by timeout.");
}
finally
{
cts.Dispose();
}
Advanced Topics in Task Parallel Library (TPL)
The Task Parallel Library provides advanced features that allow developers to handle more complex scenarios in parallel programming. These include creating tasks that do not directly map onto threads, improving code maintainability with async/await, and managing resources effectively in long-running tasks.
TaskCompletionSource for Creating Non-Thread-Based Tasks
TaskCompletionSource<T>
is a versatile mechanism in TPL that enables the creation of tasks which do not necessarily run on a new thread. Instead, it allows you to manually control the state of a task, including its start and completion, and its success or failure.
Code Example: Using TaskCompletionSource
TaskCompletionSource<int> tcs = new TaskCompletionSource<int>();
// This task can be set to complete from elsewhere in the application
void ProcessDataAndSetResult(int data)
{
try
{
// Simulate data processing
Thread.Sleep(2000);
tcs.SetResult(data * data); // Successfully set the result
}
catch (Exception ex)
{
tcs.SetException(ex); // Set the task to faulted state
}
}
// Start processing data
ProcessDataAndSetResult(5);
// The task associated with the TaskCompletionSource
Task<int> task = tcs.Task;
task.ContinueWith(t =>
{
if (t.IsFaulted)
Console.WriteLine("Task failed: " + t.Exception.InnerException.Message);
else
Console.WriteLine("Task completed successfully, result: " + t.Result);
});
// Await task completion
task.Wait();
Techniques for Using async/await with TPL
The integration of async/await syntax with TPL can greatly enhance code readability and maintainability. It provides a more declarative approach to handling asynchronous operations, making it easier to write and debug.
Code Example: Integrating async/await with TPL
async Task<int> ComputeDataAsync(int input)
{
// Simulate an asynchronous operation that computes a result
return await Task.Run(() =>
{
Thread.Sleep(1000); // Simulate delay
return input * input;
});
}
async Task RunComputation()
{
int result = await ComputeDataAsync(10);
Console.WriteLine($"Result: {result}");
}
RunComputation().Wait();
Considerations for Memory Management and Potential Leaks in Long-Running Tasks
Long-running tasks in TPL need careful management to prevent memory leaks and excessive resource consumption.
- Proper Task Disposal: Ensuring that tasks are properly disposed of after completion can help free up memory.
- Avoiding Closures in Long-Running Loops: Closures capture variables, which can lead to memory leaks if not handled carefully.
Code Example: Memory Management in Long-Running Tasks
async Task LongRunningTask()
{
int loopCounter = 0;
await Task.Run(() =>
{
for (int i = 0; i < int.MaxValue; i++)
{
loopCounter++;
// Simulate work
Thread.Sleep(10);
}
});
Console.WriteLine($"Loop completed: {loopCounter}");
}
// Start and forget a task (not recommended without proper monitoring or cancellation logic)
var task = LongRunningTask();
Conclusion
It’s clear that the Task Parallel Library (TPL) offers powerful tools for enhancing the performance and scalability of applications through parallel programming. It simplifies the complex process of managing multiple threads and tasks, providing a higher level of abstraction that makes concurrent code more accessible and maintainable.
As hardware continues to evolve with more cores and increased capabilities, .NET is poised to further enhance its parallel programming libraries. Future trends may include more integrated support for asynchronous programming, improvements in memory management, and more robust tools for debugging and performance profiling. Developers can look forward to advancements that make parallel operations even more efficient and easier to implement. For those looking to deepen their understanding of TPL and parallel programming in .NET, consider exploring Microsoft Docs
Looking to Take Your Project to the Next Level?
WireFuture understands modern software development, and the challenges it brings. Whether you need to optimize an existing application or develop a new one from scratch, hire expert C# developers from WireFuture. It can help you take advantage of .NET’s parallel programming features. Explore the future of software with WireFuture, where every line of code is built with innovation and precision in mind.
Our team of software development experts is here to transform your ideas into reality. Whether it's cutting-edge applications or revamping existing systems, we've got the skills, the passion, and the tech-savvy crew to bring your projects to life. Let's build something amazing together!
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.