Unlocking the Power of C# Generics: A Comprehensive Guide
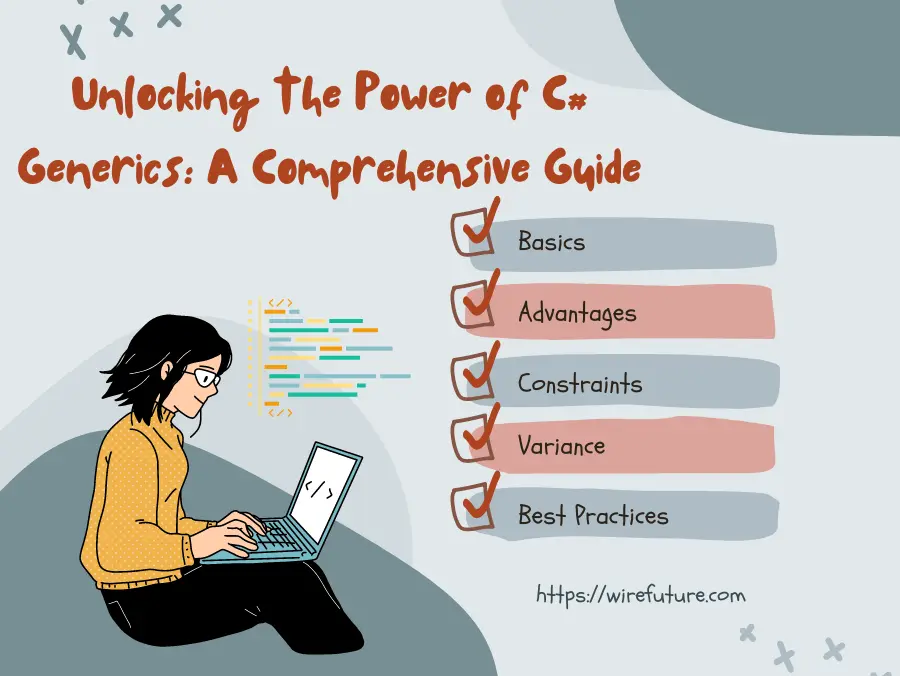
C# generics provide a powerful functionality enabling developers to define classes, methods, delegates and interfaces that contain placeholders for one or more types. This capability enables you to produce a class or method which is typesafe while working on any type of data.
What Is The Purpose Of Generics: Generics provide type safety by preventing runtime errors due to type mismatches at compile time, increasing application robustness. They also optimize performance by avoiding boxing and unboxing operations for value types. Furthermore, generics make code reuseable and clearer since developers do not have to write type-specific implementations but rather more generic methods and classes. This approach is particularly beneficial in a professional setting where you plan to hire .NET developers which can further leverage the power of generics to produce efficient and reliable software solutions.
A Short Introduction to Generics in.NET: With .NET 2.0 and C# 2.0, the community requested more flexible collections than those in .NET 1.x. In the absence of generics, developers had to use collections such as ArrayList to store elements as objects, which incurred performance overhead when boxing/unboxing operations. Generics introduced a massive new feature in .NET that allowed developers to create type-safe data structures. This shift is particularly important in the context of converting legacy systems, where updating to generics can significantly enhance performance and maintainability by replacing outdated data structures with more efficient, type-safe alternatives.
In this blog post we’ll explore C# generics in depth with lots of code samples and how it is beneficial to developers. So let’s get started.
Table of Contents
- Basics of Using C# Generics
- Advantages of C# Generics
- Constraints in C# Generics
- Generic Collections in .NET
- Variance in Generics
- Best Practices and Common Uses
- Real-World Examples of C# Generics in .NET
- Advanced Topics in Generics
- Conclusion
Basics of Using C# Generics
- Syntax Overview: Generics in C# are denoted by the angle brackets
<T>
, whereT
stands for type. This can be applied to classes, methods, interfaces, and delegates. For example, a generic class might be defined aspublic class GenericClass<T> { }
, and a generic method within could be defined aspublic T DoSomething(T input) { return input; }
. Interfaces and delegates are similarly defined, for instance,public interface IGenericInterface<T>
andpublic delegate T GenericDelegate<T>(T item);
. - The Role of the
T
Placeholder:T
is a placeholder that represents a type that will be specified by the client code when it instantiates a type or invokes a method. UsingT
allows you to write a single generic class or method that can work with any data type. You can define multiple type parameters, such as<T, U, V>
, to handle multiple unknown types in the same class or method. - Type Parameters in Action: When using generics, the specific type that replaces the placeholder can be almost any class, struct, interface, or even another generic. This allows for extensive flexibility and reusability of code. For instance,
GenericClass<int>
creates an instance of theGenericClass
that operates specifically with integers, whileGenericClass<string>
would operate with strings, both using the same underlying class structure but working with different data types.
Advantages of C# Generics
Safety of Type: The main advantage of generics is improved type safety. Generics impose compile-time type checking, requiring that only the correct type of data is used with the generic classes or methods. This prevents runtime problems such as type mismatches that would normally be caught only at runtime, thereby minimizing runtime errors and making the code overall safer and stronger.
Performance Benefits: Generics perform better because there is no boxing and unboxing involved when working with value types. In non-generic collections such as ArrayList value types are treated as objects meaning they should be boxed (converted out of a value type to an object type) when put into the collection along with unboxed (converted to the value type) when retrieved. This operation is computationally expensive. As generics typically have the type known at compile time, no boxing is required since operations are type-specific and thus much faster. This efficiency is particularly advantageous in the context of a .NET development company, where performance and resource optimization are crucial.
Reusability: Generics make code much easier to reuse. By type parameters, a single generic class, method, interface or delegate might have any number of data types. This enables developers to design a type – independent function or data structure without worrying about overloading methods or creating multiple variants of the same class for different types. This not just makes the codebase easier but also causes it to be simpler to keep less code and also increases applicability.
Constraints in C# Generics
Purpose and Utility of Constraints: Constraints in generics specify the types that can be used as arguments for type parameters in a generic class, method, or interface. They are essential because they enable the generic types to utilize the properties, methods, or interfaces of the constrained type, thus ensuring more specific behavior and safer code. Constraints help to enforce additional rules on the types used with generics, providing compile-time type safety and preventing the use of inappropriate types.
Different Types of Constraints:
Class Constraint (where T : class
): This constraint restricts the type parameter to reference types. It ensures that the generic type will only work with classes.
public class MyClass<T> where T : class
{
public T Data { get; set; }
}
Struct Constraint (where T : struct
): Conversely, this constrains the type parameter to value types. This is useful when you need to ensure that your generic type deals only with structures.
public class MyValueContainer<T> where T : struct
{
public T Value { get; set; }
}
New Constraint (where T : new()
): This constraint forces any type argument to have a public parameterless constructor. This is particularly useful when creating new instances of T
internally within the class or method.
public class MyCreator<T> where T : new()
{
public T CreateInstance()
{
return new T();
}
}
Base Class Constraint (where T : BaseClass
): This allows the generic type to accept any type that is or is derived from the specified base class. This is useful for creating generic methods that need to operate on a specific hierarchy of objects.
public class MyBaseClass { }
public class MyDerived : MyBaseClass { }
public class MyGenericClass<T> where T : MyBaseClass
{
public void PrintType()
{
Console.WriteLine(typeof(T).Name);
}
}
Interface Constraint (where T : IInterface
): This constraint ensures that the type argument must implement a particular interface. It allows the generic type to safely call the interface’s methods or properties.
public interface IMyInterface
{
void DoWork();
}
public class MyGenericWorker<T> where T : IMyInterface
{
public void ExecuteWork(T instance)
{
instance.DoWork();
}
}
Generic Collections in .NET
Overview of System.Collections.Generic Namespace: This namespace includes a variety of generic collections that are designed to store objects in different ways depending on the requirements of the application. Each collection type is designed to offer specific advantages for different operations, such as adding elements, searching elements, or removing elements.
- List<T>: A list of objects that can be accessed by index. Provides methods to search, sort, and manipulate lists.
List<int> numbers = new List<int>() { 1, 2, 3, 4, 5 };
numbers.Add(6); // Adding an element
numbers.Remove(2); // Removing an element
int x = numbers[2]; // Accessing by index
- Dictionary<TKey, TValue>: A collection of keys and values with fast lookups using keys.
Dictionary<string, int> ages = new Dictionary<string, int>();
ages.Add("Alice", 28);
ages["Bob"] = 25; // Adding using index
int aliceAge = ages["Alice"]; // Accessing by key
- Queue<T>: Represents a first-in, first-out collection of objects.
Queue<string> queue = new Queue<string>();
queue.Enqueue("First");
queue.Enqueue("Second");
string first = queue.Dequeue(); // Removes and returns 'First'
- Stack<T>: Represents a last-in, first-out collection of objects.
Stack<string> stack = new Stack<string>();
stack.Push("Last");
stack.Push("First");
string top = stack.Pop(); // Removes and returns 'First'
Comparison with Non-Generic Collections: Comparing these generic collections to their non-generic counterparts highlights several benefits primarily related to performance and type safety.
- Type Safety: Generic collections enforce type safety. You declare the type of elements they store, so there’s no risk of runtime errors due to type mismatches.
// Generic
List<int> genericList = new List<int>();
genericList.Add(10); // Only integers allowed
// Non-Generic
ArrayList nonGenericList = new ArrayList();
nonGenericList.Add(10); // Any type allowed
nonGenericList.Add("string"); // Potential runtime errors later
- Performance: Generic collections provide better performance. They do not require boxing of value types, which is needed in non-generic collections like
ArrayList
orHashtable
. This saves CPU time and memory.
// Boxing in non-generic collections
ArrayList arrayList = new ArrayList();
arrayList.Add(45); // 45 is boxed into an object
// No boxing in generic collections
List<int> intList = new List<int>();
intList.Add(45); // No boxing, 45 remains an int
Variance in Generics
Understanding Covariance and Contravariance:
- Covariance: Allows a method to return a more derived type than that defined by the generic type parameter. In C#, covariance is used in generic interfaces and delegates to enable implicit conversion of classes that implement these interfaces from a base class to a derived class. It is specified using the
out
keyword.
public interface ICovariant<out T>
{
T GetT();
}
class Animal { }
class Cat : Animal { }
class AnimalGetter : ICovariant<Animal>
{
public Animal GetT() => new Animal();
}
class CatGetter : ICovariant<Cat>
{
public Cat GetT() => new Cat();
}
// Covariance allows this assignment
ICovariant<Animal> animalGetter = new CatGetter();
- Contravariance: Allows a method to accept arguments of less derived types than that specified by the generic type parameter. Contravariance is applicable to parameters in generic interfaces and delegates. It uses the
in
keyword.
public interface IContravariant<in T>
{
void DoSomething(T t);
}
class AnimalTrainer : IContravariant<Animal>
{
public void DoSomething(Animal animal) { }
}
class CatTrainer : IContravariant<Cat>
{
public void DoSomething(Cat cat) { }
}
// Contravariance allows this assignment
IContravariant<Cat> catTrainer = new AnimalTrainer();
Best Practices and Common Uses
Effective Use of Generics:
- Use Generics for Code Reusability and Maintenance: Generics are designed to maximize code reusability. Utilizing generic classes, methods, and interfaces allows you to create more adaptable and maintainable code, which can handle various data types without redundant code.
public class DataStore<T>
{
private T[] items = new T[10];
private int count = 0;
public void Add(T item)
{
items[count++] = item;
}
public T Get(int index)
{
return items[index];
}
}
This generic DataStore
class can be used to store any type of data, ensuring type safety without duplication of logic.
- Type-Safe Collections: Generics provide a type-safe way to work with collections that store elements of a specific type, reducing the need for casting and the associated runtime errors.
List<string> names = new List<string>();
names.Add("Alice");
names.Add("Bob");
// names.Add(123); // Compile-time error
Common Pitfalls and How to Avoid Them:
- Overusing Generics: While generics are powerful, they should not be used where simple type parameters will suffice. Overusing generics can make the code unnecessarily complex and difficult to understand.
// Overuse of generics
public class Printer<T>
{
public void Print(T message)
{
Console.WriteLine(message);
}
}
// Simpler approach without generics
public class Printer
{
public void Print(string message)
{
Console.WriteLine(message);
}
}
The generic version is not justified if you are only going to use Printer
with string messages.
- Ignoring Constraints: Not using constraints when needed can lead to misuse of your generics, as they might be used with inappropriate types.
public class Repository<T> where T : IEntity
{
public void Add(T item) { /* Add item to database */ }
}
// Usage without constraints might lead to errors, e.g., trying to add types that aren't entities to a database
- Complexity in Debugging: Debugging generic code can become complex due to type abstraction. Keeping generics simple and well-documented can mitigate this issue.
// Good practice
public class Repository<T> where T : class, new()
{
public T FindById(int id)
{
// Debugging this generic method is easier when T is constrained and predictable.
T item = new T();
// Retrieve item from the database using id
return item;
}
}
Real-World Examples of C# Generics in .NET
Generic Repositories in Data Access Layers:
- A common use case for generics is in the implementation of a repository pattern, where generics are used to create a flexible data access layer that can handle various types of entities.
public interface IRepository<T> where T : class
{
T FindById(int id);
void Save(T entity);
void Delete(T entity);
}
public class GenericRepository<T> : IRepository<T> where T : class, new()
{
private DbContext context = new DbContext();
public T FindById(int id)
{
return context.Set<T>().Find(id);
}
public void Save(T entity)
{
context.Set<T>().Add(entity);
context.SaveChanges();
}
public void Delete(T entity)
{
context.Set<T>().Remove(entity);
context.SaveChanges();
}
}
Performance Optimization in Collection Operations:
- Generics can be used to improve performance in operations involving collections by avoiding boxing and unboxing, which is especially beneficial in high-performance scenarios.
List<int> numbers = new List<int>();
for (int i = 0; i < 100000; i++)
{
numbers.Add(i); // No boxing occurs here, which is efficient for memory and speed
}
int sum = 0;
foreach (int number in numbers)
{
sum += number; // Direct access to integers without unboxing
}
Custom Generic Methods for Utilities:
- Generic methods can be useful in utility classes that perform operations such as comparison, swapping, or searching, making them applicable to a wide range of data types.
public static class Utils
{
// Generic method to swap two items
public static void Swap<T>(ref T lhs, ref T rhs)
{
T temp = lhs;
lhs = rhs;
rhs = temp;
}
// Generic method to find the maximum item
public static T Max<T>(T a, T b) where T : IComparable<T>
{
return a.CompareTo(b) > 0 ? a : b;
}
}
int a = 5, b = 10;
Utils.Swap(ref a, ref b); // Works with any data type
string firstName = "Alice", lastName = "Bob";
string maxName = Utils.Max(firstName, lastName);
Case Study: System Design Enhancement:
- Generics have been used to redesign system components such as caching mechanisms and message queues, which significantly improve system performance and code quality.
public class GenericCache<T>
{
private Dictionary<string, T> cache = new Dictionary<string, T>();
public T GetItem(string key)
{
if (cache.ContainsKey(key))
{
return cache[key];
}
return default(T);
}
public void SetItem(string key, T value)
{
cache[key] = value;
}
}
// Usage of generic cache for different data types
var stringCache = new GenericCache<string>();
stringCache.SetItem("greeting", "Hello, World!");
var userCache = new GenericCache<User>();
userCache.SetItem("user1", new User { Name = "Alice", Age = 30 });
Advanced Topics in Generics
Generic Methods in Non-Generic Classes:
- It’s possible to define generic methods within non-generic classes. This approach allows the method to be flexible and handle different types without making the entire class generic.
public class UtilityClass
{
// A generic method in a non-generic class
public static void Print<T>(T message)
{
Console.WriteLine(message);
}
public static T Max<T>(T x, T y) where T : IComparable<T>
{
return x.CompareTo(y) > 0 ? x : y;
}
}
// Usage
UtilityClass.Print("Hello, World!"); // Prints a string
UtilityClass.Print(123); // Prints an integer
Console.WriteLine(UtilityClass.Max(10, 20)); // Finds and prints the maximum of two integers
Reflection with Generics:
- Reflection can be used with generics to inspect and manipulate generic types and methods dynamically. This can be complex due to type erasure and the need to handle generic type parameters correctly.
// Using reflection to investigate generic types
Type genericListType = typeof(List<>);
Type constructedListType = genericListType.MakeGenericType(typeof(int));
object listInstance = Activator.CreateInstance(constructedListType);
MethodInfo addMethod = constructedListType.GetMethod("Add");
addMethod.Invoke(listInstance, new object[] { 42 });
Console.WriteLine(listInstance); // Outputs: List of int with one element: 42
Limitations and Workarounds in the Use of Generics:
- Limitations:
- Generics cannot be used with some base type features in C#, such as static fields or methods, constructors, and non-public members when using reflection.
- Generic methods cannot infer their type parameters from the method arguments if called within another generic method without explicitly specifying the type parameters.
- Workarounds:
- For issues with base type features, consider designing your generic structures to ensure that any needed functionality does not rely on such features.
- When dealing with method type inference limitations, you can explicitly specify type arguments when calling generic methods, or refactor your code to better suit generic usage.
public class GenericHelper
{
public static T CreateInstance<T>() where T : new()
{
return new T();
}
public static void InvokeGenericMethod<T>()
{
MethodInfo method = typeof(GenericHelper).GetMethod(nameof(CreateInstance));
MethodInfo genericMethod = method.MakeGenericMethod(typeof(T));
object result = genericMethod.Invoke(null, null);
Console.WriteLine(result);
}
}
// Explicitly calling a generic method
GenericHelper.InvokeGenericMethod<int>(); // Works by explicitly specifying the type
Conclusion
C# generics are the foundation of lightweight, robust, and maintainable code in .NET applications. From performance tuning by avoiding unnecessary boxing to strong typing for code safety, generics have plenty of benefits. As we’ve seen, whether by writing Generic data structures with Generic Collections, type safety with Constraints, or more advanced techniques such as Reflection and Variance handling, generics enable cleaner, scalable code.
As C# evolves in the future, we can expect more powerful features to help generics. These advancements will unquestionably offer exciting possibilities for developers to redefine what software development can do.
Want to use C# generics to its fullest in your projects? Hire C# developers from WireFuture, where expertise meets innovation. Contact us today to learn how our expert developers can transform your business with revolutionary apps.
Our team of software development experts is here to transform your ideas into reality. Whether it's cutting-edge applications or revamping existing systems, we've got the skills, the passion, and the tech-savvy crew to bring your projects to life. Let's build something amazing together!
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.