What Are Design Patterns? Practical Guide With Examples
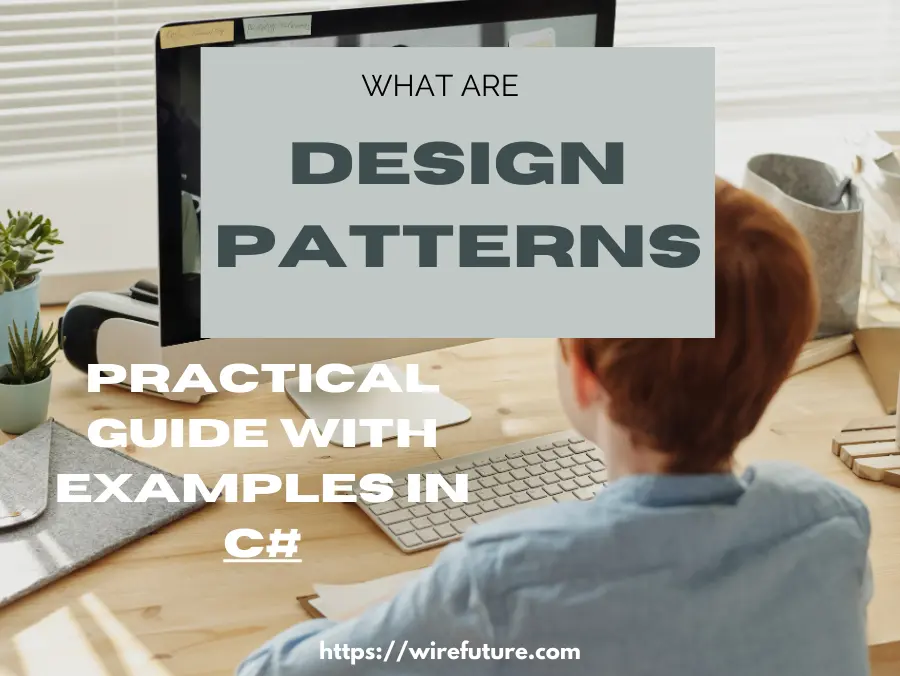
In this blog post we will aim to uncover what are design patterns with practical examples in C#. So let’s get started.
Design patterns are fundamental to software engineering because they standardize common problems developers will run into when developing software applications. Following the proven practices can help developers avoid common mistakes and ensure that their code follows sound principles of software design. These patterns enable reliable and scalable software development while also improving code readability and maintainability.
What are Design Patterns?
Design patterns are reused solutions to commonly encountered problems in a given context in software design. They can be viewed as templates to be applied to fix recurring design problems. Importantly, design patterns are not finished designs that can be directly translated into code. instead, they are descriptions or templates of how to solve a problem, customized to your specific software development project. Using design patterns helps developers draw on the collective knowledge of experienced software engineers to solve challenging design issues efficiently and effectively. One such design pattern is Command Query Responsibility Segregation (CQRS), which can be explored in more detail in our comprehensive blog post on implementing CQRS and Event Sourcing in .NET Core.
There are many design patterns so let’s study each of the popular ones with examples.
Table of Contents
- Creational Patterns
- Structural Patterns
- Behavioral Patterns
- Real-World Applications
- Best Practices and Considerations
- Conclusion
Creational Patterns
Creational design patterns are fundamentally about the creation of objects, specifically how these objects are created, composed, and represented. They help in making a system independent of how its objects are created, composed, and represented. Here, we delve into some of the primary creational patterns in C#, providing practical examples for each.
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to this instance. It is particularly useful when exactly one object is needed to coordinate actions across the system.
Example in C#:
public class DatabaseConnection
{
private static DatabaseConnection instance;
// Lock synchronization object
private static readonly object lockObject = new object();
// Private constructor to prevent instantiation outside
private DatabaseConnection() {}
public static DatabaseConnection Instance
{
get
{
lock (lockObject)
{
if (instance == null)
{
instance = new DatabaseConnection();
}
return instance;
}
}
}
}
In this example, the DatabaseConnection
class ensures that a database connection is opened only once throughout the application. The lock ensures that the instance is thread-safe.
Factory Method Pattern
The Factory Method pattern defines an interface for creating an object but lets subclasses decide which class to instantiate. This design is often used to build scalable software solutions. This pattern lets a class defer instantiation to subclasses.
Example in C#:
public abstract class VehicleFactory
{
// Factory Method
public abstract IVehicle CreateVehicle();
public class CarFactory : VehicleFactory
{
public override IVehicle CreateVehicle()
{
return new Car();
}
}
public class TruckFactory : VehicleFactory
{
public override IVehicle CreateVehicle()
{
return new Truck();
}
}
}
public interface IVehicle { }
public class Car : IVehicle { }
public class Truck : IVehicle { }
Here, VehicleFactory
is an abstract class that defines a method CreateVehicle
. Different factories (CarFactory
and TruckFactory
) override this method to create specific types of vehicles.
Builder Pattern
The Builder pattern allows constructing complex objects step by step, providing control over the construction process. This pattern separates the construction of a complex object from its representation, allowing the same construction process to create various representations.
Example in C#:
public class CarBuilder
{
private Car car = new Car();
public CarBuilder SetWheels(int number)
{
car.Wheels = number;
return this;
}
public CarBuilder SetColor(string color)
{
car.Color = color;
return this;
}
public Car Build()
{
return car;
}
}
public class Car
{
public int Wheels { get; set; }
public string Color { get; set; }
}
In this example, CarBuilder
provides methods to configure a Car
object’s properties step by step, and Build
method to finally construct the object.
Prototype Pattern
The Prototype pattern involves copying existing objects without making code dependent on their classes. It offers a mechanism for copying an object into a new object and modifying the new object to suit new requirements.
Example in C#:
public interface ICloneable
{
ICloneable Clone();
}
public class Prototype : ICloneable
{
public int Property1 { get; set; }
public string Property2 { get; set; }
public ICloneable Clone()
{
return this.MemberwiseClone() as Prototype;
}
}
This example shows how an object can be cloned using the ICloneable
interface. MemberwiseClone
is used to create a shallow copy of the object, which can then be modified without affecting the original object.
Structural Patterns
Structural patterns are crucial in software design as they help to establish how classes and objects can be composed to form larger structures. These patterns simplify the design by identifying a simple way to realize relationships between entities.
Adapter Pattern
The Adapter pattern allows objects with incompatible interfaces to work together by converting the interface of one class into another expected by the clients. This pattern is often used when new components need to be integrated and work with existing code in an application.
Example in C#:
// Existing interface in use
public interface ITarget
{
string GetRequest();
}
// Class with incompatible interface
public class Adaptee
{
public string GetSpecificRequest()
{
return "Specific request.";
}
}
// Adapter class
public class Adapter : ITarget
{
private readonly Adaptee _adaptee;
public Adapter(Adaptee adaptee)
{
_adaptee = adaptee;
}
public string GetRequest()
{
return $"This is '{_adaptee.GetSpecificRequest()}'";
}
}
In this example, Adapter
makes Adaptee
’s interface compatible with the ITarget
interface.
Decorator Pattern
The Decorator pattern allows adding new functionalities to objects dynamically by placing them inside special wrapper objects that contain these functionalities. This pattern provides a flexible alternative to subclassing for extending functionality.
Example in C#:
public interface IComponent
{
string Operation();
}
public class ConcreteComponent : IComponent
{
public string Operation()
{
return "I am walking";
}
}
public class Decorator : IComponent
{
protected IComponent component;
public Decorator(IComponent component)
{
this.component = component;
}
public virtual string Operation()
{
return component.Operation();
}
}
public class ConcreteDecorator : Decorator
{
public ConcreteDecorator(IComponent component) : base(component) {}
public override string Operation()
{
return $"[{base.Operation()} and singing]";
}
}
Here, ConcreteDecorator
adds functionality to ConcreteComponent
dynamically.
Facade Pattern
The Facade pattern simplifies interaction with a complex subsystem by providing a single simplified interface, making the subsystem easier to use. This is particularly useful for custom software solutions providers who need to offer simple interfaces to complex systems.
Example in C#:
public class SubsystemA
{
public string OperationA1() => "Subsystem A, Method A1";
public string OperationA2() => "Subsystem A, Method A2";
}
public class SubsystemB
{
public string OperationB1() => "Subsystem B, Method B1";
public string OperationB2() => "Subsystem B, Method B2";
}
public class Facade
{
protected SubsystemA _subsystemA;
protected SubsystemB _subsystemB;
public Facade(SubsystemA subsystemA, SubsystemB subsystemB)
{
_subsystemA = subsystemA;
_subsystemB = subsystemB;
}
public string Operation()
{
return $"Facade coordinates: {_subsystemA.OperationA1()}, then {_subsystemB.OperationB1()}";
}
}
This example shows how Facade
provides a simple interface to the underlying subsystems, streamlining their complexities.
Proxy Pattern
The Proxy pattern provides a surrogate or placeholder for another object to control access to it. This might be for security reasons, cost implications, or managing the lifecycle of the resource.
Example in C#:
public interface ISubject
{
void Request();
}
public class RealSubject : ISubject
{
public void Request()
{
Console.WriteLine("Request made");
}
}
public class Proxy : ISubject
{
private RealSubject _realSubject;
public void Request()
{
if (_realSubject == null)
{
_realSubject = new RealSubject();
}
_realSubject.Request();
}
}
In this scenario, Proxy
controls access to RealSubject
, potentially adding additional behaviors such as lazy initialization or access controls.
Behavioral Patterns
Behavioral design patterns are all about improving communication between disparate objects in a system. They help in managing algorithms, relationships, and responsibilities among objects, particularly useful for a .NET development company that aims to build software with efficient and dynamic inter-object communication.
Observer Pattern
The Observer pattern is used to establish a subscription mechanism to notify multiple objects about any events that happen to the object they’re observing, without creating a tight coupling between them.
Example in C#:
public interface IObserver
{
void Update(ISubject subject);
}
public interface ISubject
{
void Attach(IObserver observer);
void Detach(IObserver observer);
void Notify();
}
public class ConcreteSubject : ISubject
{
public int State { get; set; }
private List<IObserver> _observers = new List<IObserver>();
public void Attach(IObserver observer)
{
Console.WriteLine("Subject: Attached an observer.");
_observers.Add(observer);
}
public void Detach(IObserver observer)
{
_observers.Remove(observer);
Console.WriteLine("Subject: Detached an observer.");
}
public void Notify()
{
Console.WriteLine("Subject: Notifying observers...");
foreach (var observer in _observers)
{
observer.Update(this);
}
}
}
public class ConcreteObserverA : IObserver
{
public void Update(ISubject subject)
{
if ((subject as ConcreteSubject).State < 3)
{
Console.WriteLine("ConcreteObserverA: Reacted to the event.");
}
}
}
In this scenario, ConcreteObserverA
reacts to changes in ConcreteSubject
‘s state, demonstrating how the Observer pattern can facilitate reactive behaviors within software.
Strategy Pattern
The Strategy pattern allows defining a family of algorithms, encapsulating each one, and making them interchangeable. Strategy lets the algorithm vary independently from clients that use it, a principle that enhances flexibility in scenarios where multiple variations of a method are required.
Example in C#:
public interface IStrategy
{
object DoAlgorithm(object data);
}
public class ConcreteStrategyA : IStrategy
{
public object DoAlgorithm(object data)
{
var list = data as List<string>;
list.Sort();
return list;
}
}
public class Context
{
private IStrategy _strategy;
public Context() { }
public Context(IStrategy strategy)
{
this._strategy = strategy;
}
public void SetStrategy(IStrategy strategy)
{
this._strategy = strategy;
}
public void DoSomeBusinessLogic()
{
Console.WriteLine("Context: Sorting data using the strategy (not sure how it'll do it)");
var result = _strategy.DoAlgorithm(new List<string> { "a", "e", "c", "b", "d" });
string resultStr = string.Join(", ", result as List<string>);
Console.WriteLine(resultStr);
}
}
Here, different Strategy
instances can be swapped in Context
at runtime depending on the requirements, demonstrating flexibility and dynamic behavior.
Command Pattern
The Command pattern turns a request into a stand-alone object that contains all information about the request. This transformation allows parameterizing methods with different requests, delay or queue a request’s execution, and support undoable operations.
Example in C#:
public interface ICommand
{
void Execute();
}
public class SimpleCommand : ICommand
{
private string _payload;
public SimpleCommand(string payload)
{
this._payload = payload;
}
public void Execute()
{
Console.WriteLine($"SimpleCommand: See, I can do simple things like printing ({_payload})");
}
}
public class ComplexCommand : ICommand
{
private Receiver _receiver;
private string _a;
private string _b;
public ComplexCommand(Receiver receiver, string a, string b)
{
this._receiver = receiver;
this._a = a;
this._b = b;
}
public void Execute()
{
Console.WriteLine("ComplexCommand: Complex stuff should be done by a receiver object.");
_receiver.DoSomething(_a);
_receiver.DoSomethingElse(_b);
}
}
public class Receiver
{
public void DoSomething(string a)
{
Console.WriteLine($"Receiver: Working on ({a}).");
}
public void DoSomethingElse(string b)
{
Console.WriteLine($"Receiver: Also working on ({b}).");
}
}
This example showcases how commands encapsulate all the necessary details and are executed by various handler or receiver classes.
Iterator Pattern
The Iterator pattern provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. It supports variations in the traversal of a collection without changing the underlying data structure.
Example in C#:
public interface IIterator
{
bool HasNext();
object Next();
}
public interface IAggregate
{
IIterator CreateIterator();
}
public class ConcreteAggregate : IAggregate
{
private List<string> _items = new List<string>();
public IIterator CreateIterator()
{
return new ConcreteIterator(this);
}
public int Count
{
get { return _items.Count; }
}
public object this[int index]
{
get { return _items[index]; }
set { _items.Insert(index, (string)value); }
}
}
public class ConcreteIterator : IIterator
{
private ConcreteAggregate _aggregate;
private int _current = 0;
public ConcreteIterator(ConcreteAggregate aggregate)
{
this._aggregate = aggregate;
}
public bool HasNext()
{
return _current < _aggregate.Count;
}
public object Next()
{
return _aggregate[_current++] as object;
}
}
This pattern is especially useful for collections within software systems, allowing for efficient traversal without exposing internal details.
Real-World Applications
Delving into specific case studies where design patterns have resolved significant problems can provide deep insights:
Singleton Pattern in a Configuration Manager: In a large enterprise application example, many parts need access to the same configuration settings. By using the Singleton pattern, these components access the configuration from one instance of a configuration manager, allowing a single view of settings across the application. This avoids the overhead and possible inconsistencies of having each component load its own copy of the settings.
Integration of Facade Pattern with Payment Gateway: The Facade pattern could be used by a .NET development company to simplify interactions with a complex payment gateway. Using a minimal UI that hides the internal complexity of the payment processing API will make payments simpler and error-free. For example, a PaymentFacade class might offer one API call to accept payments while abstracting out the details of different payment types and API calls.
UI Components: Decorator Pattern: In a graphical user interface program, features could be added to UI elements dynamically using the decorator pattern. For instance, scrolling or border functionality can be added to a text box component without affecting the textbox’s core functionality, allowing code reuse and flexibility.
Command Pattern in Undo-Redo Operations: The command pattern may be useful in a document editor for undo and redo operations. Any action on the document (such as typing text, deleting, formatting) can be abstracted away as a command object with execute and undo capabilities. This simplifies managing a history of actions and reversing them when needed.
Best Practices and Considerations
When to Use Which Pattern
Choosing the right design pattern depends significantly on the specific problem context and the particular needs of your application. Here are some considerations for selecting appropriate patterns:
- Understanding the Problem: Clearly define what problem you are trying to solve. For instance, use creational patterns like Singleton when you need to ensure that only one instance of a class is created or use the Observer pattern to allow a subscription mechanism for event handling.
- Simplification vs. Complexity: Opt for a pattern that simplifies your system’s architecture. If a pattern does not clearly make the codebase simpler, more maintainable, or more scalable, it might not be the right choice.
- Future of the Application: Consider the future scalability and maintenance of the application. Structural patterns, like Adapter or Facade, are useful when the software needs to be adaptable to changing environments or when integration with other software systems is required.
- Performance Considerations: Some patterns, although providing excellent solutions architecturally, might introduce overhead that impacts performance. Analyze the performance implications of a pattern before deciding.
Common Pitfalls
While design patterns are powerful tools, their misuse can lead to increased complexity, making code harder to understand and maintain. Here are some common pitfalls to avoid:
- Overusing Patterns: Just because a pattern exists, doesn’t mean it should always be used. Applying a pattern where it is not necessary can lead to unnecessary complexity. For example, using a Factory pattern when a simple constructor would suffice can overcomplicate the code needlessly.
- Forcing a Fit: Avoid trying to force the use of a pattern if the requirements of the problem do not align with what the pattern is designed to solve. This can lead to awkward and inefficient solutions that are hard to maintain.
- Not Considering Simpler Solutions: Before applying a complex pattern, explore if there are simpler solutions available. Often, a more straightforward approach might be more effective and easier to implement and maintain.
- Ignoring the Implications: Every pattern has its trade-offs. For instance, Singleton can restrict flexibility and make testing difficult. Always weigh the benefits against the potential downsides in the context of your specific project.
Conclusion
As we look towards the future of software development, particularly in the context of C# and the .NET framework, it’s evident that design patterns will continue to play a critical role. However, the way these patterns are applied and evolved will likely adapt to new challenges and technologies in the software development landscape. As a leading .NET development services provider, WireFuture is ideally positioned to leverage the evolving landscape of design patterns in C#. Our expertise in custom software solutions and commitment to staying at the forefront of technology trends enable us to provide innovative, efficient, and scalable software solutions. We understand the critical role that well-implemented design patterns play in ensuring the success of complex software projects.
From initial concept to final deployment, WireFuture is your partner in software development. Our holistic approach ensures your project not only launches successfully but also thrives in the competitive digital ecosystem.
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.