What Is MongoDB And How To Use It In C#: A Developer’s Guide
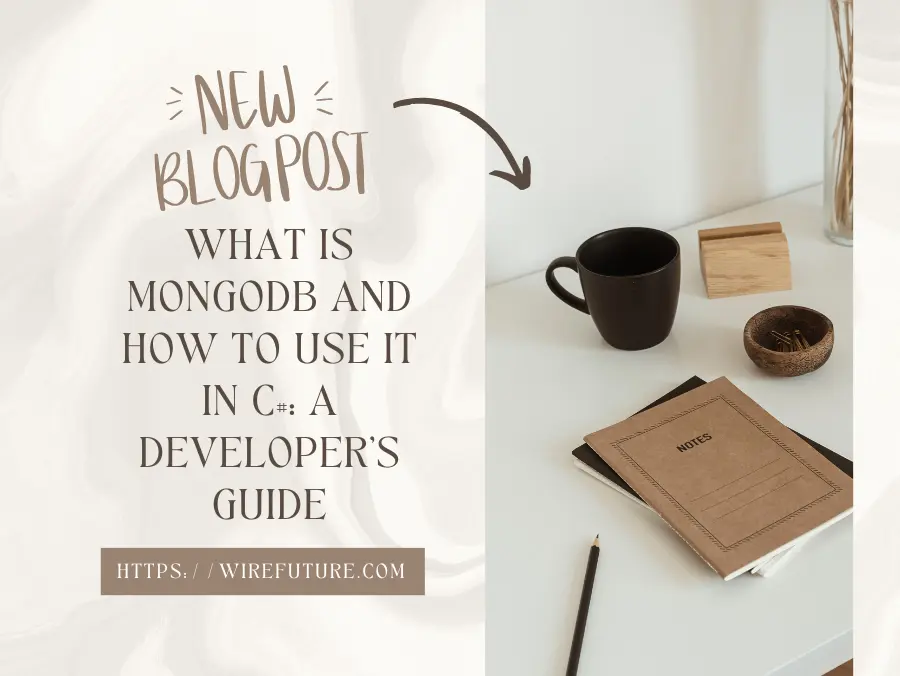
MongoDB is a high performance, high availability & scalable NoSQL database program. In contrast to traditional relational databases based on tables and rows, MongoDB is document oriented. This means it stores data in JSON-like files with dynamic schemas to facilitate and accelerate data integration in certain types of applications. Consequently, MongoDB is optimal for handling massive amounts of data whose structures are dynamic over time.
Integration with C#/.NET
MongoDB is easily integrated with C#/.NET applications by using the MongoDB.Driver library, a .NET driver that enables asynchronous MongoDB interaction. This integration allows .NET developers to easily manage their MongoDB databases from within the C# programming language. MongoDB integration with C#/.NET is facilitated by the driver supporting LINQ queries and its close encapsulation with C#’s object-oriented programming, making it a good choice for developers intending to utilize MongoDB’s schema-less and document-oriented features.
MongoDB enables developers to add features like full text search, real time data processing, and much more, while still keeping the freedom to handle data in how their software needs change. This combination is powerful for building any application type, from web / mobile to big data processing / real time analytics. The MongoDB ease of setup and scalability along with the type-safe environment provided by C# makes it an ideal foundation for developing performant and scalable applications.
Table of Contents
- Setting Up MongoDB with C#/.NET Core
- Key Features of MongoDB for C# Developers
- Building a Sample Application
- Advanced Features and Techniques
- Real-world Use Cases
- Best Practices for Using MongoDB with C#/.NET
- Challenges and Considerations
- Conclusion
Setting Up MongoDB with C#/.NET Core
Prerequisites
Before integrating MongoDB with a .NET Core application, you’ll need to ensure that you have the necessary tools and libraries installed. The primary library required is MongoDB.Driver
, which is the official MongoDB .NET driver. This driver allows .NET Core applications to interact with MongoDB using native .NET syntax and patterns. To install this library, you will need the .NET Core SDK installed on your machine and an IDE such as Visual Studio or Visual Studio Code for development.
Installation Guide
Here’s a step-by-step guide to setting up MongoDB in your .NET Core environment:
- Install MongoDB:
- If MongoDB isn’t installed on your system, download it from the MongoDB official site. Choose the version compatible with your operating system.
- Follow the installation instructions specific to your OS. This typically involves extracting the files and running the installer, which will set up MongoDB as a service that runs in the background.
- Set Up the MongoDB Environment:
- Ensure that MongoDB is running on your system. You can start MongoDB on Windows by navigating to your MongoDB installation folder (usually
C:\Program Files\MongoDB\Server\<version>\bin
) and runningmongod.exe
. - For macOS and Linux, you can usually start MongoDB with the
mongod
command in your terminal.
- Ensure that MongoDB is running on your system. You can start MongoDB on Windows by navigating to your MongoDB installation folder (usually
- Create a MongoDB Database and Collection:
- Use the MongoDB shell (
mongo.exe
in the bin directory for Windows) to interact with your MongoDB server. - Create a new database by using
use <databaseName>
, replacing<databaseName>
with your desired database name. - Create a collection within your database by executing
db.createCollection('<collectionName>')
.
- Use the MongoDB shell (
- Install MongoDB.Driver for .NET Core:
- Open your .NET Core project in your IDE and add the MongoDB.Driver package. This can be done via the NuGet Package Manager. You can either use the GUI in Visual Studio or run the following command in the terminal or Package Manager Console:
dotnet add package MongoDB.Driver
- This command adds the MongoDB.Driver to your project, allowing you to use MongoDB within your .NET Core application.
Configure Your .NET Core Application to Connect to MongoDB:
- In your .NET Core project, you’ll need to configure the connection to MongoDB. This typically involves adding a connection string in your
appsettings.json
file:
{
"MongoDB": {
"ConnectionString": "mongodb://localhost:27017",
"Database": "YourDatabaseName"
}
}
Create a MongoDB client in your application. You can do this in your startup configuration (e.g., Startup.cs
) or wherever you manage your application’s services and configuration:
services.AddSingleton<IMongoClient, MongoClient>(s =>
new MongoClient(Configuration.GetConnectionString("MongoDB")));
By following these steps, your .NET Core application will be equipped to interact with MongoDB, allowing you to perform CRUD operations and leverage the full capabilities of MongoDB in your applications.
Key Features of MongoDB for C# Developers
One of the most significant advantages MongoDB offers to C# developers, especially within a .NET Development Company working with .NET Core, is its schema-less data model. This model enables the storage of data in BSON (Binary JSON) documents, which can vary in structure. Unlike traditional relational databases that demand a predefined schema, MongoDB’s flexible schema approach permits developers to store documents in a collection without the uniformity of structure. This flexibility is particularly advantageous in scenarios where:
- Data Variability: Applications that handle data with variable structures, such as different types of user-generated content, can benefit significantly. Each document can store a unique set of fields, accommodating the diversity of data naturally.
- Agile Development: During the development process, especially in agile environments, requirements can change rapidly. For a .NET development agency, using MongoDB means that adapting to new data models does not necessitate extensive backend modifications or time-consuming database migrations. This flexibility significantly reduces development time and enhances the agency’s ability to respond swiftly to changing project needs, making MongoDB an ideal choice for agile and fast-paced development settings.
- Prototyping: Quick prototyping is facilitated as developers can modify the data model on the fly without worrying about schema migrations.
This flexibility can lead to more natural, intuitive, and efficient data handling within .NET Core applications, as it aligns well with dynamic object models often used in C# programming.
CRUD Operations
MongoDB.Driver for C#, the official MongoDB .NET driver, provides robust support for all CRUD (Create, Read, Update, Delete) operations, allowing .NET developers to interact with MongoDB in a way that is both idiomatic to C# and natural for those familiar with .NET’s LINQ queries. Here’s how these operations are typically performed:
Create: Adding new documents to a collection is straightforward. Developers can define a class that maps to the document structure, and then instances of this class can be added to the database using the InsertOne
or InsertMany
methods.
var database = client.GetDatabase("testdb");
var collection = database.GetCollection<BsonDocument>("testcollection");
var document = new BsonDocument { {"name", "New Document"}, {"value", 100} };
collection.InsertOne(document);
Read: Retrieving documents uses filters and projections, often constructed with fluent syntax or LINQ queries. The MongoDB.Driver library integrates seamlessly with .NET Core’s LINQ provider, making queries easy to write and understand.
var result = collection.Find(new BsonDocument("name", "New Document")).FirstOrDefault();
Update: Updating documents can be done using various methods such as UpdateOne
, UpdateMany
, or ReplaceOne
. These methods allow for precise modifications to documents based on specific conditions.
var filter = Builders<BsonDocument>.Filter.Eq("name", "New Document");
var update = Builders<BsonDocument>.Update.Set("value", 200);
collection.UpdateOne(filter, update);
Delete: Removing documents is similarly straightforward, with methods like DeleteOne
or DeleteMany
allowing for the removal of documents that match given criteria.
var deleteFilter = Builders<BsonDocument>.Filter.Eq("name", "New Document");
collection.DeleteOne(deleteFilter);
Building a Sample Application
Project Setup: Creating a New C# Project and Integrating MongoDB
To begin building a sample application that integrates MongoDB with C#, you’ll first need to set up a new project. Here’s how you can get started using .NET Core:
Create a New .NET Core Console Application:
- Open your command line interface (CLI) or Terminal.
- Navigate to the directory where you want your project to reside.
- Run the following command to create a new .NET Core console application:
dotnet new console -n MongoDbSampleApp
- This command creates a new folder named
MongoDbSampleApp
with a simple “Hello World” .NET Core console application.
Add MongoDB.Driver Package:
Change into the newly created project directory:
cd MongoDbSampleApp
Install the MongoDB.Driver package, which is necessary to interact with your MongoDB database, by executing:
dotnet add package MongoDB.Driver
This command downloads and adds the MongoDB.Driver library to your project, allowing you to write code that connects to and manipulates your MongoDB database.
Example Code: Connecting to MongoDB and Performing Basic Operations
Once your project setup is complete, you can start writing some basic code to interact with MongoDB. Below are simple examples that demonstrate how to connect to MongoDB from C# and perform CRUD operations.
Connecting to MongoDB:
- Open the
Program.cs
file in your project. - Add the following using directives at the top of your file to include necessary namespaces:
using MongoDB.Driver;
using MongoDB.Bson;
Inside the Main
method, add code to connect to a MongoDB instance:
var client = new MongoClient("mongodb://localhost:27017");
var database = client.GetDatabase("testdb");
var collection = database.GetCollection<BsonDocument>("testcollection");
Console.WriteLine("Connected to MongoDB.");
Inserting Documents:
- Add the following code to create and insert a new document into your collection:
var newDocument = new BsonDocument
{
{"name", "John Doe"},
{"age", 30},
{"isAlive", true}
};
collection.InsertOne(newDocument);
Console.WriteLine("Document inserted.");
Finding Documents:
- Retrieve and display the inserted document:
var filter = Builders<BsonDocument>.Filter.Eq("name", "John Doe");
var document = collection.Find(filter).FirstOrDefault();
Console.WriteLine("Found document: " + document.ToString());
Updating Documents:
- Modify the existing document by increasing the age:
var update = Builders<BsonDocument>.Update.Set("age", 31);
collection.UpdateOne(filter, update);
Console.WriteLine("Document updated.");
Deleting Documents:
- Remove the document from the collection:
collection.DeleteOne(filter);
Console.WriteLine("Document deleted.");
Advanced Features and Techniques
Aggregation Framework
MongoDB’s Aggregation Framework is a robust feature that facilitates complex data processing and analysis directly within the database. It enables developers to create pipelines consisting of multiple stages, each designed to transform the data progressively. By leveraging the Aggregation Framework through C#, companies can perform sophisticated operations like filtering, grouping, and sorting of data—essential for extracting insights and compiling reports from large datasets. Hiring a skilled .NET developers can further enhance the capability to utilize this framework effectively, ensuring that complex data tasks are handled efficiently and insights are derived with precision.
Here’s how you can use the Aggregation Framework in a C#/.NET application:
Basic Usage: To use the aggregation framework, you start by defining an aggregation pipeline, which is a list of processing stages. Here’s a simple example where we group documents by a category
field and calculate the total and average values of a price
field:
var collection = database.GetCollection<BsonDocument>("products");
var group = new BsonDocument
{
{ "_id", "$category" },
{ "totalPrice", new BsonDocument { { "$sum", "$price" } } },
{ "averagePrice", new BsonDocument { { "$avg", "$price" } } }
};
var pipeline = new[] { new BsonDocument { { "$group", group } } };
var results = collection.Aggregate<BsonDocument>(pipeline).ToList();
foreach (var result in results)
{
Console.WriteLine(result);
}
This code groups the products by category and calculates the total and average prices for each category.
Advanced Operations: More complex operations might include combining multiple stages such as $match
for filtering, $project
for selecting specific fields, and $sort
for ordering the results. The flexibility of the Aggregation Framework allows it to handle diverse requirements, such as multi-level grouping and conditional aggregations.
Data Indexing and Performance Tuning
Proper indexing is critical for enhancing the performance of MongoDB operations, especially in large-scale applications. Effective use of indexes can drastically reduce the amount of data the database needs to scan for queries, which improves response times and reduces load.
Creating Indexes: In C#, you can easily define indexes using the MongoDB.Driver library. For example, to create an ascending index on the username
field of a users
collection, you could use:
var collection = database.GetCollection<BsonDocument>("users");
var indexKeys = Builders<BsonDocument>.IndexKeys.Ascending("username");
collection.Indexes.CreateOne(new CreateIndexModel<BsonDocument>(indexKeys));
Index Management: Managing indexes involves more than just creating them. You should regularly review and optimize your indexes based on the actual usage patterns. Use the MongoDB query planner to analyze the effectiveness of your indexes and adjust as necessary.
Performance Tuning: Beyond indexing, performance can be tuned by optimizing query patterns and ensuring that operations are efficient. For instance, using projection to retrieve only the necessary fields can reduce the amount of data transferred over the network. Similarly, considering the use of capped collections for high-throughput operations can improve performance for scenarios such as logging and real-time data feeds.
Real-world Use Cases
Web Applications
MongoDB’s flexible schema and performance make it ideal for web applications, especially session management and user data storage. The very nature of web applications typically means handling a large volume of heterogeneous data types and structures – from user profiles to session data – with differing access patterns and life cycles.
Management of Session(s): In web applications, session data is often dynamic and tailored to individual users. MongoDB offers a flexible and scalable solution for session storage, supporting the nesting of session data as documents within a collection. Each session document can encompass not only session IDs and timestamps but also detailed user state information. This capability is crucial for handling the high read/write loads typical of session data operations while ensuring quick response times for user interactions. Additionally, adopting MongoDB for session management helps build secure software solutions, as it facilitates comprehensive and secure handling of sensitive session information, enhancing overall application security.
Storage of User Data: MongoDB is optimized for handling large sets of user data including profiles, user configurations and historical user activity logs. It is schema-less, so as application requirements evolve, the data structure can be reconfigured without significant downtime or tedious migrations. For example, a new feature that requires additional user preference storage can be added without rearranging the database schema.
IoT and Real-Time Analytics
MongoDB offers great potential in IoT and real-time analytics due to its massive size and query performance.
IoT Data Management: IoT devices create large amounts of data very quickly. MongoDB can efficiently store such time-series data, storing different data structures across different devices in a single database. Writing and reading data quickly is important for IoT applications where real-time response and analysis is often required.
Real-Time Analytics: MongoDB’s aggregation pipeline enables real-time data processing, which is necessary for actionable insights from distributed data streams. For instance, MongoDB can generate averages, sums, and other statistical information over time windows for dynamic decision-making. This capability is critical for environmental monitoring, real-time health data analysis or live financial trading environments.
These use cases demonstrate MongoDB features in use cases in real applications and prove that it is suitable for distributed web applications and IoT/realtime analytics. With MongoDB, programmers are able to be certain that their applications can meet today’s data demands efficiently with flexibility.
Best Practices for Using MongoDB with C#/.NET
Error Handling
Effective error handling is crucial for building robust applications using MongoDB and C#. MongoDB operations can fail due to a variety of reasons such as network issues, write conflicts, or schema validation errors. Here are some tips for handling these scenarios:
Use Try-Catch Blocks: Surround your MongoDB operations with try-catch blocks to handle exceptions gracefully. This approach helps in identifying the cause of the error and responding appropriately, whether it’s retrying the operation, logging the error, or notifying the user.
try
{
// MongoDB operation
var result = collection.Find(filter).FirstOrDefault();
}
catch (MongoException ex)
{
// Handle MongoDB-specific exceptions
Console.WriteLine("MongoDB error: " + ex.Message);
}
catch (Exception ex)
{
// Handle all other exceptions
Console.WriteLine("General error: " + ex.Message);
}
Understand Common MongoDB Errors: Familiarize yourself with common MongoDB errors such as DuplicateKeyException
, TimeoutException
, or NetworkException
. Knowing these can help you provide more specific error handling and recovery strategies.
Implement Robust Logging: Ensure that all exceptions are logged with detailed information about the context in which they occurred. This data is invaluable for diagnosing issues in production environments.
Security Practices
Securing your MongoDB database is critical, especially when dealing with sensitive or personal data. Here are key security practices to implement:
- Enable Authentication: Use MongoDB’s built-in support for authentication. Ensure that it is enabled and configure strong, role-based access control for your database users.
- Use TLS/SSL: Encrypt data in transit by enabling TLS/SSL between your C#/.NET application and MongoDB server. This prevents eavesdropping and man-in-the-middle attacks.
- Regular Updates: Keep your MongoDB server and .NET driver updated to the latest versions. Updates often include patches for security vulnerabilities.
- Secure Database Configuration: Minimize potential attack surfaces by disabling unused MongoDB services or interfaces, and ensure that the MongoDB instance is not exposed directly to the internet.
- Data Encryption: Consider encrypting sensitive fields within your documents using MongoDB’s encryption capabilities or client-side encryption in your .NET application for added security.
Optimal Schema Design
While MongoDB is schema-less, designing an effective document structure is crucial for performance and scalability:
- Align Documents with Application Needs: Design your document schema based on how your application accesses data. For instance, group data that is accessed together into the same document to minimize read operations.
- Avoid Large Arrays: Large arrays can degrade performance, especially if elements are frequently added or removed. If an array grows over time, consider an alternative design such as embedding sub-documents or using a separate collection.
- Use Indexes Wisely: Design your schema with indexing strategies in mind. Ensure that frequently queried fields are indexed but avoid over-indexing as it can slow down write operations.
- Consider Read/Write Patterns: Design your schema based on your application’s read and write patterns. For heavy read applications, schema design can be optimized for faster queries; for write-heavy applications, optimize the schema for quicker inserts and updates. Additionally, consider the need to hire backend developers who specialize in optimizing schema design for enhanced performance.
Challenges and Considerations
Transaction Support
While MongoDB offers transaction support, it comes with specific limitations and considerations that are particularly important when integrating with C#/.NET applications. Understanding these can help you better design and implement robust systems:
- ACID Transactions: As of MongoDB version 4.0 and later, ACID transactions are supported across multiple documents, collections, and databases. This is crucial for applications that require consistency across complex operations. However, using transactions can affect performance due to the increased overhead. It’s important for developers to balance the need for transactions with the performance implications.
- Scoped Usage: Transactions in MongoDB are best used selectively for operations that absolutely require atomicity. Overuse can lead to performance bottlenecks. They are ideal for scenarios where operations span multiple documents or collections and need to be atomic.
- Replica Set Requirements: To use transactions, MongoDB must be running in a replica set configuration, even if it is a single-node replica set. This setup is necessary for ensuring that data integrity and consistency are maintained across the distributed system.
- Error Handling: When working with transactions in MongoDB through C#, proper error handling becomes crucial. Transaction blocks should be carefully managed to handle exceptions and ensure that transactions are either committed or aborted correctly, to avoid hanging transactions or data inconsistencies.
Maintenance and Scaling
Maintaining and scaling a MongoDB database in a .NET application environment involves several strategies and considerations to ensure the database performs optimally as the application load increases:
- Monitoring: Regular monitoring of the MongoDB instance is crucial for understanding performance metrics and identifying potential bottlenecks. Tools like MongoDB Atlas offer built-in monitoring solutions that can alert developers to issues like slow-running queries or hardware resource limitations.
- Scaling Strategies: MongoDB provides horizontal scaling through sharding, which distributes data across multiple machines. Implementing sharding effectively requires careful planning of shard keys and understanding of the data distribution to prevent imbalances that can lead to performance issues.
- Backup and Recovery: Regular backups are essential for data recovery in case of a failure. MongoDB offers several backup solutions, including point-in-time backups and continuous backups with oplog access. Ensuring that these backups are configured correctly and tested regularly is crucial for data integrity.
- Capacity Planning: As the application scales, capacity planning becomes increasingly important. This involves estimating data growth and system load to proactively scale the MongoDB deployment before performance is impacted. This planning should consider read/write ratios, data growth rates, and query complexity.
- Index Management: As the database scales, managing indexes becomes more critical. Developers need to regularly review query performance and index usage, adding or removing indexes based on the current usage patterns to optimize query speed without compromising write performance.
Conclusion
Integrating MongoDB with C#/.NET offers numerous advantages that can significantly enhance your application’s performance, scalability, and maintainability. Some of the key benefits include:
- Flexible Data Modeling: The schema-less nature of MongoDB aligns perfectly with the dynamic and object-oriented features of C#, allowing developers to work more intuitively with complex data structures.
- High Performance: MongoDB’s powerful indexing and querying capabilities, along with support for aggregation and transactions, ensure that applications can handle large volumes of data efficiently.
- Scalability: With features like sharding and replication, MongoDB is designed to scale horizontally, making it an excellent choice for applications that anticipate growth in data volume and user load.
- Developer Productivity: The MongoDB.Driver for .NET provides a seamless integration that leverages LINQ and asynchronous programming features in C#, boosting developer productivity with familiar and powerful constructs.
Additional Learning Resources
To further enhance your understanding and skills in using MongoDB with C#, consider exploring the following resources:
- MongoDB University: Offers a range of free online courses that cover various aspects of MongoDB, from basic introductions to advanced usage and optimization techniques.
- Official MongoDB Blog: Provides updates, tutorials, and case studies that can give deeper insights into MongoDB’s capabilities and best practices.
- GitHub Repositories: Numerous community and official projects showcase MongoDB used in real-world C#/.NET applications, offering practical examples and codebases to learn from.
- Stack Overflow: A great resource for troubleshooting specific issues or learning from the challenges and solutions experienced by other MongoDB developers.
Expertise in Database Development
As you look to leverage MongoDB for your business applications, consider partnering with WireFuture. Our team includes expert database developers skilled in MongoDB, ready to help you design, implement, and optimize your databases. Whether you need to hire MongoDB developers or require broader database development expertise, WireFuture offers the professional services needed to ensure your projects are successful. Contact us to learn how our developers can enhance your data strategies and help you get the most out of MongoDB.
By taking advantage of these resources and considering professional assistance from teams like WireFuture, you can ensure that your MongoDB integration with C#/.NET not only meets but exceeds your application requirements.
Our team of software development experts is here to transform your ideas into reality. Whether it's cutting-edge applications or revamping existing systems, we've got the skills, the passion, and the tech-savvy crew to bring your projects to life. Let's build something amazing together!
No commitment required. Whether you’re a charity, business, start-up or you just have an idea – we’re happy to talk through your project.
Embrace a worry-free experience as we proactively update, secure, and optimize your software, enabling you to focus on what matters most – driving innovation and achieving your business goals.